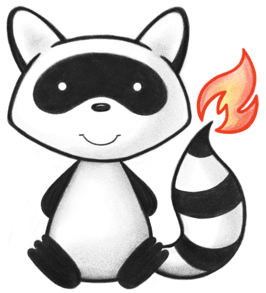
001package org.hl7.fhir.r5.utils; 002 003import java.io.File; 004import java.io.FileInputStream; 005import java.io.FileOutputStream; 006import java.io.IOException; 007import java.util.HashSet; 008import java.util.Set; 009 010import org.hl7.fhir.exceptions.FHIRException; 011import org.hl7.fhir.r5.formats.IParser.OutputStyle; 012import org.hl7.fhir.r5.formats.JsonParser; 013import org.hl7.fhir.r5.formats.XmlParser; 014import org.hl7.fhir.r5.model.CodeSystem; 015import org.hl7.fhir.r5.model.Enumerations.CodeSystemContentMode; 016import org.hl7.fhir.r5.model.CodeSystem.CodeSystemHierarchyMeaning; 017import org.hl7.fhir.r5.model.CodeSystem.ConceptDefinitionComponent; 018import org.hl7.fhir.r5.model.Resource; 019import org.hl7.fhir.utilities.MarkedToMoveToAdjunctPackage; 020import org.hl7.fhir.utilities.filesystem.ManagedFileAccess; 021 022@MarkedToMoveToAdjunctPackage 023public class ResourceFixer { 024 025 026 public static void main(String[] args) throws IOException { 027 new ResourceFixer().vistAllResources(args[0]); 028 029 } 030 031 private Set<String> refs = new HashSet<>(); 032 033 private void vistAllResources(String folder) throws IOException { 034 035 for (File f : ManagedFileAccess.file(folder).listFiles()) { 036 if (f.isDirectory()) { 037 vistAllResources(f.getAbsolutePath()); 038 } else if (f.getName().endsWith(".json")) { 039 Resource r = null; 040 try { 041 r = new JsonParser().parse(ManagedFileAccess.inStream(f)); 042 } catch (Throwable e) { 043 // nothing at all 044 } 045 if (r != null) { 046 try { 047 if (visitResource(r)) { 048 new JsonParser().setOutputStyle(OutputStyle.PRETTY).compose(ManagedFileAccess.outStream(f), r); 049 } 050 } catch (Exception e) { 051 System.out.println("Error processing "+f.getAbsolutePath()+": "+e.getMessage()); 052// e.printStackTrace(); 053 } 054 } 055 } else if (f.getName().endsWith(".xml")) { 056 Resource r = null; 057 try { 058 r = new XmlParser().parse(ManagedFileAccess.inStream(f)); 059 } catch (Throwable e) { 060 // nothing at all 061 } 062 if (r != null) { 063 try { 064 if (visitResource(r)) { 065 new XmlParser().setOutputStyle(OutputStyle.PRETTY).compose(ManagedFileAccess.outStream(f), r); 066 } 067 } catch (Exception e) { 068 System.out.println("Error processing "+f.getAbsolutePath()+": "+e.getMessage()); 069// e.printStackTrace(); 070 } 071 } 072 } 073 } 074 } 075 076 private boolean visitResource(Resource r) { 077 if (r.hasId()) { 078 String ref = r.fhirType()+"/"+r.getId(); 079 if (refs.contains(ref)) { 080 throw new FHIRException("Duplicate resource "+ref); 081 } 082 refs.add(ref); 083 } 084 if (r instanceof CodeSystem) { 085 return visitCodeSystem((CodeSystem) r); 086 } 087 return false; 088 } 089 090 private boolean visitCodeSystem(CodeSystem cs) { 091 if (!cs.hasContent()) { 092 System.out.println("Setting content = complete for CodeSystem/"+cs.getId()); 093 cs.setContent(CodeSystemContentMode.COMPLETE); 094 return true; 095 } else if (!cs.hasHierarchyMeaning() && hasHierarchy(cs)) { 096 System.out.println("Setting hierarchyMeaning = is-a for CodeSystem/"+cs.getId()); 097 cs.setHierarchyMeaning(CodeSystemHierarchyMeaning.ISA); 098 return true; 099 } else { 100 return false; 101 } 102 } 103 104 private boolean hasHierarchy(CodeSystem cs) { 105 for (ConceptDefinitionComponent c : cs.getConcept()) { 106 if (c.hasConcept()) { 107 return true; 108 } 109 } 110 return false; 111 } 112 113}