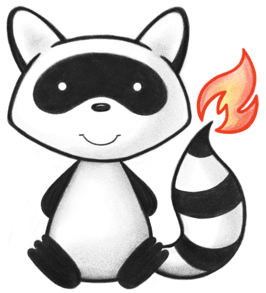
001package org.hl7.fhir.r5.utils.client; 002 003 004 005 006 007/* 008 Copyright (c) 2011+, HL7, Inc. 009 All rights reserved. 010 011 Redistribution and use in source and binary forms, with or without modification, 012 are permitted provided that the following conditions are met: 013 014 * Redistributions of source code must retain the above copyright notice, this 015 list of conditions and the following disclaimer. 016 * Redistributions in binary form must reproduce the above copyright notice, 017 this list of conditions and the following disclaimer in the documentation 018 and/or other materials provided with the distribution. 019 * Neither the name of HL7 nor the names of its contributors may be used to 020 endorse or promote products derived from this software without specific 021 prior written permission. 022 023 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 024 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 025 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 026 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 027 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 028 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 029 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 030 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 031 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 032 POSSIBILITY OF SUCH DAMAGE. 033 034*/ 035 036import java.util.ArrayList; 037import java.util.List; 038 039import org.hl7.fhir.r5.model.OperationOutcome; 040 041/** 042 * FHIR client exception. 043 * 044 * FHIR API exception will be wrapped in FHIR client exceptions. OperationOutcome errors 045 * resulting from the server can be access by calling: 046 * <pre><code> 047 * if(e.hasServerErrors()) { 048 * List<OperationOutcome> errors = e.getServerErrors(); 049 * //process errors... 050 * } 051 * </code></pre> 052 * 053 * 054 * 055 * @author Claude Nanjo 056 * 057 */ 058public class EFhirClientException extends RuntimeException { 059 private static final long serialVersionUID = 1L; 060 private OperationOutcome error = null; 061 private int code; 062 063 public EFhirClientException(int code, String message) { 064 super(message); 065 this.code = code; 066 } 067 068 public EFhirClientException(Exception cause) { 069 super(cause); 070 } 071 072 public EFhirClientException(int code, String message, Exception cause) { 073 super(message, cause); 074 this.code = code; 075 } 076 077 /** 078 * Generate EFhirClientException which include a message indicating the cause of the exception 079 * along with any OperationOutcome server error that may have resulted. 080 * 081 * @param message 082 * @param serverError 083 */ 084 public EFhirClientException(int code, String message, OperationOutcome serverError) { 085 super(message); 086 this.code = code; 087 error = serverError; 088 } 089 090 091 /** 092 * Generate EFhirClientException which include a message indicating the cause of the exception 093 * along with any OperationOutcome server error that may have resulted. 094 * 095 * @param message 096 * @param serverError 097 */ 098 public EFhirClientException(int code, String message, OperationOutcome serverError, Exception cause) { 099 super(message, cause); 100 this.code = code; 101 error = serverError; 102 } 103 104 /** 105 * Generate EFhirClientException indicating the cause of the exception 106 * along with any OperationOutcome server error the server may have generated. 107 * 108 * A default message of "One or more server side errors have occurred during this operation. Refer to e.getServerErrors() for additional details." 109 * will be returned to users. 110 * 111 * @param serverError 112 */ 113 public EFhirClientException(int code, OperationOutcome serverError) { 114 super("Error on the server: "+serverError.getText().getDiv().allText()+". Refer to e.getServerErrors() for additional details."); 115 this.code = code; 116 error = serverError; 117 } 118 119 public OperationOutcome getServerError() { 120 return error; 121 } 122 123 /** 124 * Method returns true if exception contains server OperationOutcome errors in payload. 125 * 126 * @return 127 */ 128 public boolean hasServerError() { 129 return error != null; 130 } 131 132 public int getCode() { 133 return code; 134 } 135 136}