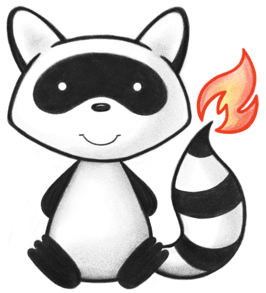
001package org.hl7.fhir.r5.utils.formats; 002 003import java.io.File; 004import java.io.FileInputStream; 005import java.io.FileOutputStream; 006import java.io.IOException; 007import java.util.ArrayList; 008import java.util.List; 009 010import javax.sound.sampled.FloatControl.Type; 011 012import org.hl7.fhir.r5.formats.JsonParser; 013import org.hl7.fhir.r5.formats.XmlParser; 014import org.hl7.fhir.r5.formats.IParser.OutputStyle; 015import org.hl7.fhir.r5.model.Resource; 016import org.hl7.fhir.utilities.filesystem.ManagedFileAccess; 017 018public class ResourceFolderVisitor { 019 020 public interface IResourceObserver { 021 public boolean visitResource(String filename, Resource resource); 022 } 023 024 private IResourceObserver observer; 025 private List<String> types = new ArrayList<>(); 026 027 public ResourceFolderVisitor(IResourceObserver observer) { 028 super(); 029 this.observer = observer; 030 } 031 032 public ResourceFolderVisitor(IResourceObserver observer, String... types) { 033 super(); 034 this.observer = observer; 035 for (String t : types) { 036 this.types.add(t); 037 } 038 } 039 040 041 public void visit(String folder) throws IOException { 042 visit(ManagedFileAccess.file(folder)); 043 } 044 045 public void visit(File file) { 046 for (File f : file.listFiles()) { 047 if (f.isDirectory()) { 048 visit(f); 049 } else if (f.getName().endsWith(".xml")) { 050 try { 051 Resource res = new XmlParser().parse(ManagedFileAccess.inStream(f)); 052 if (types.isEmpty() || types.contains(res.fhirType())) { 053 if (observer.visitResource(f.getAbsolutePath(), res)) { 054 new XmlParser().setOutputStyle(OutputStyle.PRETTY).compose(ManagedFileAccess.outStream(f), res); 055 } 056 } 057 } catch (Exception e) { 058 } 059 } else if (f.getName().endsWith(".json")) { 060 try { 061 Resource res = new JsonParser().parse(ManagedFileAccess.inStream(f)); 062 if (types.isEmpty() || types.contains(res.fhirType())) { 063 if (observer.visitResource(f.getAbsolutePath(), res)) { 064 new JsonParser().setOutputStyle(OutputStyle.PRETTY).compose(ManagedFileAccess.outStream(f), res); 065 } 066 } 067 } catch (Exception e) { 068 } 069 } 070 } 071 } 072 073 074}