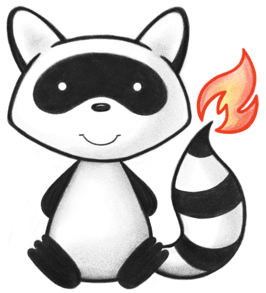
001package org.hl7.fhir.r5.utils.sql; 002 003import org.hl7.fhir.utilities.MarkedToMoveToAdjunctPackage; 004 005@MarkedToMoveToAdjunctPackage 006public class Column { 007 008 private String name; 009 private int length; 010 private String type; 011 private ColumnKind kind; 012 private Boolean isColl; 013 private boolean duplicateReported; 014 private String notes; 015 016 protected Column() { 017 super(); 018 } 019 020 public Column(String name, Boolean isColl, String type, ColumnKind kind) { 021 super(); 022 this.name = name; 023 this.isColl = isColl; 024 this.type = type; 025 this.kind = kind; 026 } 027 028 public String getName() { 029 return name; 030 } 031 public int getLength() { 032 return length; 033 } 034 public ColumnKind getKind() { 035 return kind; 036 } 037 038 public void setName(String name) { 039 this.name = name; 040 } 041 042 public void setLength(int length) { 043 this.length = length; 044 } 045 046 public void setKind(ColumnKind kind) { 047 this.kind = kind; 048 } 049 050 public String getType() { 051 return type; 052 } 053 054 public void setType(String type) { 055 this.type = type; 056 } 057 058 public Boolean isColl() { 059 return isColl; 060 } 061 062 public void setColl(Boolean isColl) { 063 this.isColl = isColl; 064 } 065 066 public String diff(Column other) { 067 if (!name.equals(other.name)) { 068 return "Names differ: '"+name+"' vs '"+other.name+"'"; 069 } 070 if (kind != ColumnKind.Null && other.kind != ColumnKind.Null) { 071 if (length != other.length) { 072 return "Lengths differ: '"+length+"' vs '"+other.length+"'"; 073 } 074 if (kind != other.kind) { 075 return "Kinds differ: '"+kind+"' vs '"+other.kind+"'"; 076 } 077 if (isColl != other.isColl) { 078 return "Collection status differs: '"+isColl+"' vs '"+other.isColl+"'"; 079 } 080 } else if (kind == ColumnKind.Null) { 081 kind = other.kind; 082 length = other.length; 083 isColl = other.isColl; 084 } 085 return null; 086 } 087 088 public boolean isDuplicateReported() { 089 return duplicateReported; 090 } 091 092 public void setDuplicateReported(boolean duplicateReported) { 093 this.duplicateReported = duplicateReported; 094 } 095 096 @Override 097 public String toString() { 098 return "Column [name=" + name + ", length=" + length + ", type=" + type + ", kind=" + kind + ", isColl=" + isColl 099 + "]"; 100 } 101 102 public String getNotes() { 103 return notes; 104 } 105 106 public void setNotes(String notes) { 107 this.notes = notes; 108 } 109 110 111 public void addNote(String note) { 112 this.notes = notes == null ? note : notes+"; "+note; 113 } 114 115}