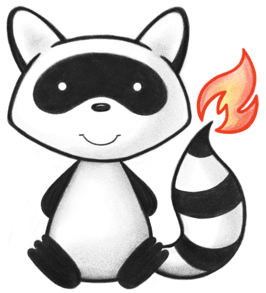
001package org.hl7.fhir.r5.utils.sql; 002 003import java.util.List; 004 005import org.hl7.fhir.exceptions.FHIRException; 006import org.hl7.fhir.r5.model.Base; 007import org.hl7.fhir.r5.utils.sql.Validator.TrueFalseOrUnknown; 008import org.hl7.fhir.utilities.MarkedToMoveToAdjunctPackage; 009import org.hl7.fhir.utilities.json.model.JsonArray; 010import org.hl7.fhir.utilities.json.model.JsonBoolean; 011import org.hl7.fhir.utilities.json.model.JsonElement; 012import org.hl7.fhir.utilities.json.model.JsonNull; 013import org.hl7.fhir.utilities.json.model.JsonNumber; 014import org.hl7.fhir.utilities.json.model.JsonObject; 015import org.hl7.fhir.utilities.json.model.JsonString; 016 017@MarkedToMoveToAdjunctPackage 018public class StorageJson implements Storage { 019 020 private String name; 021 private JsonArray rows; 022 023 @Override 024 public TrueFalseOrUnknown supportsArrays() { 025 return TrueFalseOrUnknown.TRUE; 026 } 027 028 @Override 029 public Store createStore(String name, List<Column> columns) { 030 this.name = name; 031 this.rows = new JsonArray(); 032 return new Store(name); // we're not doing anything with this 033 } 034 035 @Override 036 public void addRow(Store store, List<Cell> cells) { 037 JsonObject row = new JsonObject(); 038 rows.add(row); 039 for (Cell cell : cells) { 040 if (cell.getColumn().isColl() || cell.getValues().size() > 1) { 041 JsonArray arr = new JsonArray(); 042 row.add(cell.getColumn().getName(), arr); 043 for (Value value : cell.getValues()) { 044 arr.add(makeJsonNode(value)); 045 } 046 } else if (cell.getValues().size() == 0) { 047 row.add(cell.getColumn().getName(), new JsonNull()); 048 } else { 049 row.add(cell.getColumn().getName(), makeJsonNode(cell.getValues().get(0))); 050 } 051 } 052 } 053 054 private JsonElement makeJsonNode(Value value) { 055 if (value == null) { 056 return new JsonNull(); 057 } else if (value.getValueInt() != null) { 058 return new JsonNumber(value.getValueInt().intValue()); 059 } 060 if (value.getValueBoolean() != null) { 061 return new JsonBoolean(value.getValueBoolean().booleanValue()); 062 } 063 if (value.getValueDecimal() != null) { 064 return new JsonNumber(value.getValueDecimal().toPlainString()); 065 } 066 return new JsonString(value.getValueString()); 067 } 068 069 @Override 070 public void finish(Store store) { 071 // nothing 072 } 073 074 public String getName() { 075 return name; 076 } 077 078 public JsonArray getRows() { 079 return rows; 080 } 081 082 @Override 083 public TrueFalseOrUnknown supportsComplexTypes() { 084 return TrueFalseOrUnknown.TRUE; 085 } 086 087 @Override 088 public TrueFalseOrUnknown needsName() { 089 return TrueFalseOrUnknown.FALSE; 090 } 091 092 @Override 093 public String getKeyForSourceResource(Base res) { 094 return res.fhirType()+"/"+res.getIdBase(); 095 } 096 097 @Override 098 public String getKeyForTargetResource(Base res) { 099 return res.fhirType()+"/"+res.getIdBase(); 100 } 101 102}