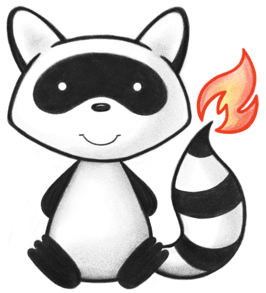
001package org.hl7.fhir.r5.utils.sql; 002 003import java.math.BigDecimal; 004import java.util.Date; 005 006import org.hl7.fhir.r5.model.Base; 007import org.hl7.fhir.utilities.MarkedToMoveToAdjunctPackage; 008 009 010/** 011 * String value is always provided, and a more specific value may also be provided 012 */ 013 014@MarkedToMoveToAdjunctPackage 015public class Value { 016 017 private String valueString; 018 private Boolean valueBoolean; 019 private Date valueDate; 020 private Integer valueInt; 021 private BigDecimal valueDecimal; 022 private byte[] valueBinary; 023 private Base valueComplex; 024 025 private Value() { 026 super(); 027 } 028 029 public static Value makeString(String s) { 030 Value v = new Value(); 031 v.valueString = s; 032 return v; 033 } 034 035 public static Value makeBoolean(String s, Boolean b) { 036 Value v = new Value(); 037 v.valueString = s; 038 v.valueBoolean = b; 039 return v; 040 } 041 042 public static Value makeDate(String s, Date d) { 043 Value v = new Value(); 044 v.valueString = s; 045 v.valueDate = d; 046 return v; 047 } 048 049 public static Value makeInteger(String s, Integer i) { 050 Value v = new Value(); 051 v.valueString = s; 052 v.valueInt = i; 053 return v; 054 } 055 056 057 public static Value makeDecimal(String s, BigDecimal bigDecimal) { 058 Value v = new Value(); 059 v.valueString = s; 060 v.valueDecimal = bigDecimal; 061 return v; 062 } 063 064 public static Value makeBinary(String s, byte[] b) { 065 Value v = new Value(); 066 v.valueString = s; 067 v.valueBinary = b; 068 return v; 069 } 070 071 public static Value makeComplex(Base b) { 072 Value v = new Value(); 073 v.valueComplex = b; 074 return v; 075 } 076 public String getValueString() { 077 return valueString; 078 } 079 080 public Date getValueDate() { 081 return valueDate; 082 } 083 084 public Integer getValueInt() { 085 return valueInt; 086 } 087 088 public BigDecimal getValueDecimal() { 089 return valueDecimal; 090 } 091 092 public byte[] getValueBinary() { 093 return valueBinary; 094 } 095 096 public Boolean getValueBoolean() { 097 return valueBoolean; 098 } 099 100 public Base getValueComplex() { 101 return valueComplex; 102 } 103 104 public boolean hasValueString() { 105 return valueString != null; 106 } 107 108 public boolean hasValueDate() { 109 return valueDate != null; 110 } 111 112 public boolean hasValueInt() { 113 return valueInt != null; 114 } 115 116 public boolean hasValueDecimal() { 117 return valueDecimal != null; 118 } 119 120 public boolean hasValueBinary() { 121 return valueBinary != null; 122 } 123 124 public boolean hasValueBoolean() { 125 return valueBoolean != null; 126 } 127 128 public boolean hasValueComplex() { 129 return valueComplex != null; 130 } 131}