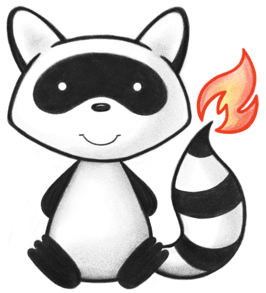
001package org.hl7.fhir.r5.utils.structuremap; 002 003import org.apache.commons.lang3.NotImplementedException; 004import org.hl7.fhir.exceptions.FHIRException; 005import org.hl7.fhir.exceptions.PathEngineException; 006import org.hl7.fhir.r5.elementmodel.Element; 007import org.hl7.fhir.r5.fhirpath.FHIRPathEngine; 008import org.hl7.fhir.r5.fhirpath.TypeDetails; 009import org.hl7.fhir.r5.fhirpath.FHIRPathUtilityClasses.FunctionDetails; 010import org.hl7.fhir.r5.model.Base; 011import org.hl7.fhir.r5.model.Resource; 012import org.hl7.fhir.r5.model.ValueSet; 013import org.hl7.fhir.r5.utils.validation.IResourceValidator; 014import org.hl7.fhir.utilities.MarkedToMoveToAdjunctPackage; 015import org.hl7.fhir.utilities.validation.ValidationMessage; 016 017import java.util.ArrayList; 018import java.util.List; 019 020@MarkedToMoveToAdjunctPackage 021public class FHIRPathHostServices implements FHIRPathEngine.IEvaluationContext { 022 023 private final StructureMapUtilities structureMapUtilities; 024 025 public FHIRPathHostServices(StructureMapUtilities structureMapUtilities) { 026 this.structureMapUtilities = structureMapUtilities; 027 } 028 029 public List<Base> resolveConstant(FHIRPathEngine engine, Object appContext, String name, boolean beforeContext, boolean explicitConstant) throws PathEngineException { 030 Variables vars = (Variables) appContext; 031 Base res = vars.get(VariableMode.INPUT, name); 032 if (res == null) 033 res = vars.get(VariableMode.OUTPUT, name); 034 List<Base> result = new ArrayList<Base>(); 035 if (res != null) 036 result.add(res); 037 return result; 038 } 039 040 @Override 041 public TypeDetails resolveConstantType(FHIRPathEngine engine, Object appContext, String name, boolean explicitConstant) throws PathEngineException { 042 if (!(appContext instanceof VariablesForProfiling)) 043 throw new Error("Internal Logic Error (wrong type '" + appContext.getClass().getName() + "' in resolveConstantType)"); 044 VariablesForProfiling vars = (VariablesForProfiling) appContext; 045 VariableForProfiling v = vars.get(null, name); 046 if (v == null) 047 throw new PathEngineException("Unknown variable '" + name + "' from variables " + vars.summary()); 048 return v.getProperty().getTypes(); 049 } 050 051 @Override 052 public boolean log(String argument, List<Base> focus) { 053 throw new Error("Not Implemented Yet"); 054 } 055 056 @Override 057 public FunctionDetails resolveFunction(FHIRPathEngine engine, String functionName) { 058 return null; // throw new Error("Not Implemented Yet"); 059 } 060 061 @Override 062 public TypeDetails checkFunction(FHIRPathEngine engine, Object appContext, String functionName, TypeDetails focus, List<TypeDetails> parameters) throws PathEngineException { 063 throw new Error("Not Implemented Yet"); 064 } 065 066 @Override 067 public List<Base> executeFunction(FHIRPathEngine engine, Object appContext, List<Base> focus, String functionName, List<List<Base>> parameters) { 068 throw new Error("Not Implemented Yet"); 069 } 070 071 @Override 072 public Base resolveReference(FHIRPathEngine engine, Object appContext, String url, Base refContext) throws FHIRException { 073 if (structureMapUtilities.getServices() == null) 074 return null; 075 return structureMapUtilities.getServices().resolveReference(appContext, url); 076 } 077 078 private boolean noErrorValidationMessages(List<ValidationMessage> valerrors) { 079 boolean ok = true; 080 for (ValidationMessage v : valerrors) 081 ok = ok && !v.getLevel().isError(); 082 return ok; 083 } 084 085 @Override 086 public boolean conformsToProfile(FHIRPathEngine engine, Object appContext, Base item, String url) throws FHIRException { 087 IResourceValidator val = structureMapUtilities.getWorker().newValidator(); 088 List<ValidationMessage> valerrors = new ArrayList<ValidationMessage>(); 089 if (item instanceof Resource) { 090 val.validate(appContext, valerrors, (Resource) item, url); 091 return noErrorValidationMessages(valerrors); 092 } 093 if (item instanceof Element) { 094 val.validate(appContext, valerrors, null, (Element) item, url); 095 return noErrorValidationMessages(valerrors); 096 } 097 throw new NotImplementedException("Not done yet (FHIRPathHostServices.conformsToProfile), when item is not element or not resource"); 098 } 099 100 @Override 101 public ValueSet resolveValueSet(FHIRPathEngine engine, Object appContext, String url) { 102 return structureMapUtilities.getWorker().findTxResource(ValueSet.class, url); 103 } 104 105 @Override 106 public boolean paramIsType(String name, int index) { 107 return false; 108 } 109}