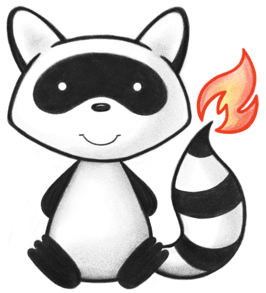
001package org.hl7.fhir.r5.utils.structuremap; 002 003import org.hl7.fhir.r5.elementmodel.Property; 004import org.hl7.fhir.r5.fhirpath.TypeDetails; 005import org.hl7.fhir.utilities.CommaSeparatedStringBuilder; 006import org.hl7.fhir.utilities.MarkedToMoveToAdjunctPackage; 007 008import java.util.ArrayList; 009import java.util.List; 010 011@MarkedToMoveToAdjunctPackage 012public class VariablesForProfiling { 013 private final StructureMapUtilities structureMapUtilities; 014 private List<VariableForProfiling> list = new ArrayList<VariableForProfiling>(); 015 private boolean optional; 016 private boolean repeating; 017 018 public VariablesForProfiling(StructureMapUtilities structureMapUtilities, boolean optional, boolean repeating) { 019 this.structureMapUtilities = structureMapUtilities; 020 this.optional = optional; 021 this.repeating = repeating; 022 } 023 024 public void add(VariableMode mode, String name, String path, Property property, TypeDetails types) { 025 add(mode, name, new PropertyWithType(path, property, null, types)); 026 } 027 028 public void add(VariableMode mode, String name, String path, Property baseProperty, Property profileProperty, TypeDetails types) { 029 add(mode, name, new PropertyWithType(path, baseProperty, profileProperty, types)); 030 } 031 032 public void add(VariableMode mode, String name, PropertyWithType property) { 033 VariableForProfiling vv = null; 034 for (VariableForProfiling v : list) 035 if ((v.getMode() == mode) && v.getName().equals(name)) 036 vv = v; 037 if (vv != null) 038 list.remove(vv); 039 list.add(new VariableForProfiling(mode, name, property)); 040 } 041 042 public VariablesForProfiling copy(boolean optional, boolean repeating) { 043 VariablesForProfiling result = new VariablesForProfiling(structureMapUtilities, optional, repeating); 044 result.list.addAll(list); 045 return result; 046 } 047 048 public VariablesForProfiling copy() { 049 VariablesForProfiling result = new VariablesForProfiling(structureMapUtilities, optional, repeating); 050 result.list.addAll(list); 051 return result; 052 } 053 054 public VariableForProfiling get(VariableMode mode, String name) { 055 if (mode == null) { 056 for (VariableForProfiling v : list) 057 if ((v.getMode() == VariableMode.OUTPUT) && v.getName().equals(name)) 058 return v; 059 for (VariableForProfiling v : list) 060 if ((v.getMode() == VariableMode.INPUT) && v.getName().equals(name)) 061 return v; 062 } 063 for (VariableForProfiling v : list) 064 if ((v.getMode() == mode) && v.getName().equals(name)) 065 return v; 066 return null; 067 } 068 069 public String summary() { 070 CommaSeparatedStringBuilder s = new CommaSeparatedStringBuilder(); 071 CommaSeparatedStringBuilder t = new CommaSeparatedStringBuilder(); 072 for (VariableForProfiling v : list) 073 if (v.getMode() == VariableMode.INPUT) 074 s.append(v.summary()); 075 else 076 t.append(v.summary()); 077 return "source variables [" + s.toString() + "], target variables [" + t.toString() + "]"; 078 } 079}