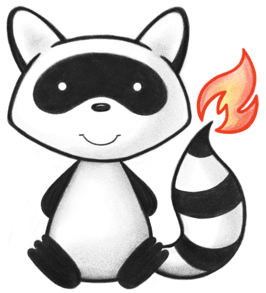
001package org.hl7.fhir.r5.utils.validation; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030 */ 031 032 033import org.hl7.fhir.exceptions.FHIRException; 034import org.hl7.fhir.r5.context.IWorkerContext; 035import org.hl7.fhir.r5.elementmodel.Manager.FhirFormat; 036import org.hl7.fhir.r5.model.Coding; 037import org.hl7.fhir.r5.model.StructureDefinition; 038import org.hl7.fhir.r5.model.UsageContext; 039import org.hl7.fhir.r5.utils.validation.constants.BestPracticeWarningLevel; 040import org.hl7.fhir.r5.utils.validation.constants.CheckDisplayOption; 041import org.hl7.fhir.r5.utils.validation.constants.IdStatus; 042import org.hl7.fhir.utilities.MarkedToMoveToAdjunctPackage; 043import org.hl7.fhir.utilities.json.model.JsonObject; 044import org.hl7.fhir.utilities.validation.ValidationMessage; 045 046import java.io.IOException; 047import java.io.InputStream; 048import java.util.List; 049 050/** 051 * Interface to the instance validator. This takes a resource, in one of many forms, and 052 * checks whether it is valid 053 * 054 * @author Grahame Grieve 055 * 056 */ 057@MarkedToMoveToAdjunctPackage 058public interface IResourceValidator { 059 060 IWorkerContext getContext(); 061 062 /** 063 * how much to check displays for coded elements 064 */ 065 CheckDisplayOption getCheckDisplay(); 066 void setCheckDisplay(CheckDisplayOption checkDisplay); 067 068 /** 069 * whether the resource must have an id or not (depends on context) 070 */ 071 IdStatus getResourceIdRule(); 072 void setResourceIdRule(IdStatus resourceIdRule); 073 074 /** 075 * whether the validator should enforce best practice guidelines 076 * as defined by various HL7 committees 077 */ 078 BestPracticeWarningLevel getBestPracticeWarningLevel(); 079 IResourceValidator setBestPracticeWarningLevel(BestPracticeWarningLevel value); 080 081 IValidatorResourceFetcher getFetcher(); 082 IResourceValidator setFetcher(IValidatorResourceFetcher value); 083 084 IValidationPolicyAdvisor getPolicyAdvisor(); 085 void setPolicyAdvisor(IValidationPolicyAdvisor advisor); 086 087 IValidationProfileUsageTracker getTracker(); 088 IResourceValidator setTracker(IValidationProfileUsageTracker value); 089 090 boolean isNoBindingMsgSuppressed(); 091 IResourceValidator setNoBindingMsgSuppressed(boolean noBindingMsgSuppressed); 092 093 boolean isNoInvariantChecks(); 094 IResourceValidator setNoInvariantChecks(boolean value) ; 095 096 boolean isWantInvariantInMessage(); 097 IResourceValidator setWantInvariantInMessage(boolean wantInvariantInMessage); 098 099 boolean isNoTerminologyChecks(); 100 IResourceValidator setNoTerminologyChecks(boolean noTerminologyChecks); 101 102 boolean isNoExtensibleWarnings(); 103 IResourceValidator setNoExtensibleWarnings(boolean noExtensibleWarnings); 104 105 boolean isNoUnicodeBiDiControlChars(); 106 void setNoUnicodeBiDiControlChars(boolean noUnicodeBiDiControlChars); 107 108 boolean isForPublication(); 109 IResourceValidator setForPublication(boolean forPublication); 110 111 boolean isExample(); 112 IResourceValidator setExample(boolean example); 113 114 // used to decide whether additional bindings, constraints etc apply 115 public List<UsageContext> getUsageContexts(); 116 117 public boolean isWarnOnDraftOrExperimental(); 118 119 public IResourceValidator setWarnOnDraftOrExperimental(boolean warnOnDraftOrExperimental); 120 121 /** 122 * Whether being unable to resolve a profile in found in Resource.meta.profile or ElementDefinition.type.profile or targetProfile is an error or just a warning 123 */ 124 boolean isErrorForUnknownProfiles(); 125 void setErrorForUnknownProfiles(boolean errorForUnknownProfiles); 126 127 boolean isShowMessagesFromReferences(); 128 void setShowMessagesFromReferences(boolean value); 129 130 /** 131 * this is used internally in the publishing stack to ensure that everything is water tight, but 132 * this check is not necessary or appropriate at run time when the validator is hosted in HAPI 133 * @return 134 */ 135 boolean isWantCheckSnapshotUnchanged(); 136 void setWantCheckSnapshotUnchanged(boolean wantCheckSnapshotUnchanged); 137 138 /** 139 * It's common to see references such as Patient/234234 - these usually mean a reference to a Patient resource. 140 * But there's no actual technical rule that it does, so the validator doesn't enforce that unless this setting is 141 * set to true 142 * 143 * @return 144 */ 145 boolean isAssumeValidRestReferences(); 146 void setAssumeValidRestReferences(boolean value); 147 148 /** 149 * if this is true, the validator will accept extensions and references to example.org and acme.com as 150 * valid, on the basis that they are understood to be references to content that could exist in priniple but can't in practice 151 */ 152 boolean isAllowExamples(); 153 void setAllowExamples(boolean value) ; 154 155 boolean isNoCheckAggregation(); 156 void setNoCheckAggregation(boolean value); 157 158 /** 159 * CrumbTrail - whether the validator creates hints to 160 * @return 161 */ 162 boolean isCrumbTrails(); 163 void setCrumbTrails(boolean crumbTrails); 164 165 boolean isValidateValueSetCodesOnTxServer(); 166 void setValidateValueSetCodesOnTxServer(boolean value); 167 168 public Coding getJurisdiction(); 169 public IResourceValidator setJurisdiction(Coding jurisdiction); 170 171 /** 172 * Bundle validation rules allow for requesting particular entries in a bundle get validated against particular profiles 173 * Typically this is used from the command line to avoid having to construct profile just to validate a particular resource 174 * in a bundle against a particular profile 175 * 176 * @return 177 */ 178 List<BundleValidationRule> getBundleValidationRules(); 179 180 /** 181 * Validate suite 182 * 183 * you can validate one of the following representations of resources: 184 * 185 * stream - provide a format - this is the preferred choice 186 * 187 * Use one of these two if the content is known to be valid XML/JSON, and already parsed 188 * - a DOM element or Document 189 * - a Json Object 190 * 191 * In order to use these, the content must already be parsed - e.g. it must syntactically valid 192 * - a native resource 193 * - a elementmodel resource 194 * 195 * in addition, you can pass one or more profiles ti validate beyond the base standard - as structure definitions or canonical URLs 196 * @throws IOException 197 */ 198 void validate(Object Context, List<ValidationMessage> errors, String initialPath, org.hl7.fhir.r5.elementmodel.Element element) throws FHIRException; 199 void validate(Object Context, List<ValidationMessage> errors, String initialPath, org.hl7.fhir.r5.elementmodel.Element element, String profile) throws FHIRException; 200 void validate(Object Context, List<ValidationMessage> errors, String initialPath, org.hl7.fhir.r5.elementmodel.Element element, List<StructureDefinition> profiles) throws FHIRException; 201 202 org.hl7.fhir.r5.elementmodel.Element validate(Object Context, List<ValidationMessage> errors, InputStream stream, FhirFormat format) throws FHIRException; 203 org.hl7.fhir.r5.elementmodel.Element validate(Object Context, List<ValidationMessage> errors, InputStream stream, FhirFormat format, String profile) throws FHIRException; 204 org.hl7.fhir.r5.elementmodel.Element validate(Object Context, List<ValidationMessage> errors, InputStream stream, FhirFormat format, List<StructureDefinition> profiles) throws FHIRException; 205 206 org.hl7.fhir.r5.elementmodel.Element validate(Object Context, List<ValidationMessage> errors, org.hl7.fhir.r5.model.Resource resource) throws FHIRException; 207 org.hl7.fhir.r5.elementmodel.Element validate(Object Context, List<ValidationMessage> errors, org.hl7.fhir.r5.model.Resource resource, String profile) throws FHIRException; 208 org.hl7.fhir.r5.elementmodel.Element validate(Object Context, List<ValidationMessage> errors, org.hl7.fhir.r5.model.Resource resource, List<StructureDefinition> profiles) throws FHIRException; 209 210 org.hl7.fhir.r5.elementmodel.Element validate(Object Context, List<ValidationMessage> errors, org.w3c.dom.Element element) throws FHIRException; 211 org.hl7.fhir.r5.elementmodel.Element validate(Object Context, List<ValidationMessage> errors, org.w3c.dom.Element element, String profile) throws FHIRException; 212 org.hl7.fhir.r5.elementmodel.Element validate(Object Context, List<ValidationMessage> errors, org.w3c.dom.Element element, List<StructureDefinition> profile) throws FHIRException; 213 214 org.hl7.fhir.r5.elementmodel.Element validate(Object Context, List<ValidationMessage> errors, org.w3c.dom.Document document) throws FHIRException; 215 org.hl7.fhir.r5.elementmodel.Element validate(Object Context, List<ValidationMessage> errors, org.w3c.dom.Document document, String profile) throws FHIRException; 216 org.hl7.fhir.r5.elementmodel.Element validate(Object Context, List<ValidationMessage> errors, org.w3c.dom.Document document, List<StructureDefinition> profile) throws FHIRException; 217 218 org.hl7.fhir.r5.elementmodel.Element validate(Object Context, List<ValidationMessage> errors, JsonObject object) throws FHIRException; 219 org.hl7.fhir.r5.elementmodel.Element validate(Object Context, List<ValidationMessage> errors, JsonObject object, String profile) throws FHIRException; 220 org.hl7.fhir.r5.elementmodel.Element validate(Object Context, List<ValidationMessage> errors, JsonObject object, List<StructureDefinition> profile) throws FHIRException; 221 222 223}