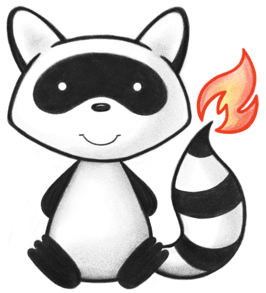
001package org.hl7.fhir.common.hapi.validation.support; 002 003import ca.uhn.fhir.context.FhirContext; 004import ca.uhn.fhir.context.support.ConceptValidationOptions; 005import ca.uhn.fhir.context.support.IValidationSupport; 006import ca.uhn.fhir.context.support.LookupCodeRequest; 007import ca.uhn.fhir.context.support.TranslateConceptResults; 008import ca.uhn.fhir.context.support.ValidationSupportContext; 009import ca.uhn.fhir.context.support.ValueSetExpansionOptions; 010import jakarta.annotation.Nonnull; 011import jakarta.annotation.Nullable; 012import org.apache.commons.lang3.Validate; 013import org.hl7.fhir.instance.model.api.IBaseResource; 014 015import java.util.List; 016 017/** 018 * This class is a wrapper for an existing {@link @IContextValidationSupport} object, intended to be 019 * subclassed in order to layer functionality on top of the existing validation support object. 020 * 021 * @since 5.0.0 022 */ 023public abstract class BaseValidationSupportWrapper extends BaseValidationSupport { 024 private final IValidationSupport myWrap; 025 026 /** 027 * Constructor 028 * 029 * @param theFhirContext The FhirContext object (must be initialized for the appropriate FHIR version) 030 * @param theWrap The validation support object to wrap 031 */ 032 public BaseValidationSupportWrapper(FhirContext theFhirContext, IValidationSupport theWrap) { 033 super(theFhirContext); 034 Validate.notNull(theWrap, "theWrap must not be null"); 035 036 myWrap = theWrap; 037 } 038 039 @Override 040 public List<IBaseResource> fetchAllConformanceResources() { 041 return myWrap.fetchAllConformanceResources(); 042 } 043 044 @Nullable 045 @Override 046 public <T extends IBaseResource> List<T> fetchAllNonBaseStructureDefinitions() { 047 return myWrap.fetchAllNonBaseStructureDefinitions(); 048 } 049 050 @Override 051 public <T extends IBaseResource> List<T> fetchAllStructureDefinitions() { 052 return myWrap.fetchAllStructureDefinitions(); 053 } 054 055 @Nullable 056 @Override 057 public <T extends IBaseResource> List<T> fetchAllSearchParameters() { 058 return myWrap.fetchAllSearchParameters(); 059 } 060 061 @Override 062 public <T extends IBaseResource> T fetchResource(Class<T> theClass, String theUri) { 063 return myWrap.fetchResource(theClass, theUri); 064 } 065 066 @Override 067 public byte[] fetchBinary(String theBinaryKey) { 068 return myWrap.fetchBinary(theBinaryKey); 069 } 070 071 @Override 072 public boolean isCodeSystemSupported(ValidationSupportContext theValidationSupportContext, String theSystem) { 073 return myWrap.isCodeSystemSupported(theValidationSupportContext, theSystem); 074 } 075 076 @Override 077 public CodeValidationResult validateCode( 078 @Nonnull ValidationSupportContext theValidationSupportContext, 079 @Nonnull ConceptValidationOptions theOptions, 080 String theCodeSystem, 081 String theCode, 082 String theDisplay, 083 String theValueSetUrl) { 084 return myWrap.validateCode( 085 theValidationSupportContext, theOptions, theCodeSystem, theCode, theDisplay, theValueSetUrl); 086 } 087 088 @Override 089 public IValidationSupport.CodeValidationResult validateCodeInValueSet( 090 ValidationSupportContext theValidationSupportContext, 091 ConceptValidationOptions theValidationOptions, 092 String theCodeSystem, 093 String theCode, 094 String theDisplay, 095 @Nonnull IBaseResource theValueSet) { 096 return myWrap.validateCodeInValueSet( 097 theValidationSupportContext, theValidationOptions, theCodeSystem, theCode, theDisplay, theValueSet); 098 } 099 100 @Override 101 public LookupCodeResult lookupCode( 102 ValidationSupportContext theValidationSupportContext, @Nonnull LookupCodeRequest theLookupCodeRequest) { 103 return myWrap.lookupCode(theValidationSupportContext, theLookupCodeRequest); 104 } 105 106 @Override 107 public boolean isValueSetSupported(ValidationSupportContext theValidationSupportContext, String theValueSetUrl) { 108 return myWrap.isValueSetSupported(theValidationSupportContext, theValueSetUrl); 109 } 110 111 @Override 112 public IValidationSupport.ValueSetExpansionOutcome expandValueSet( 113 ValidationSupportContext theValidationSupportContext, 114 ValueSetExpansionOptions theExpansionOptions, 115 @Nonnull IBaseResource theValueSetToExpand) { 116 return myWrap.expandValueSet(theValidationSupportContext, theExpansionOptions, theValueSetToExpand); 117 } 118 119 @Override 120 public IBaseResource fetchCodeSystem(String theSystem) { 121 return myWrap.fetchCodeSystem(theSystem); 122 } 123 124 @Override 125 public IBaseResource fetchValueSet(String theUri) { 126 return myWrap.fetchValueSet(theUri); 127 } 128 129 @Override 130 public IBaseResource fetchStructureDefinition(String theUrl) { 131 return myWrap.fetchStructureDefinition(theUrl); 132 } 133 134 @Override 135 public IBaseResource generateSnapshot( 136 ValidationSupportContext theValidationSupportContext, 137 IBaseResource theInput, 138 String theUrl, 139 String theWebUrl, 140 String theProfileName) { 141 return myWrap.generateSnapshot(theValidationSupportContext, theInput, theUrl, theWebUrl, theProfileName); 142 } 143 144 @Override 145 public TranslateConceptResults translateConcept(TranslateCodeRequest theRequest) { 146 return myWrap.translateConcept(theRequest); 147 } 148}