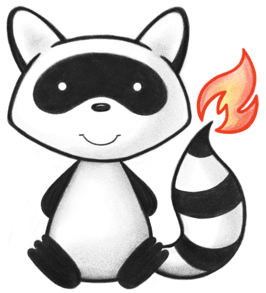
001package org.hl7.fhir.common.hapi.validation.support; 002 003import ca.uhn.fhir.context.support.IValidationSupport; 004import org.apache.commons.lang3.time.DateUtils; 005 006/** 007 * @deprecated This should no longer be used, caching functionality is provided by {@link ValidationSupportChain} 008 */ 009@Deprecated(since = "8.0.0", forRemoval = true) 010public class CachingValidationSupport extends BaseValidationSupportWrapper implements IValidationSupport { 011 012 private final boolean myIsEnabledValidationForCodingsLogicalAnd; 013 014 /** 015 * Constructor with default timeouts 016 * 017 * @param theWrap The validation support module to wrap 018 */ 019 public CachingValidationSupport(IValidationSupport theWrap) { 020 this(theWrap, CacheTimeouts.defaultValues(), false); 021 } 022 023 public CachingValidationSupport(IValidationSupport theWrap, boolean theIsEnabledValidationForCodingsLogicalAnd) { 024 this(theWrap, CacheTimeouts.defaultValues(), theIsEnabledValidationForCodingsLogicalAnd); 025 } 026 027 public CachingValidationSupport(IValidationSupport theWrap, CacheTimeouts theCacheTimeouts) { 028 this(theWrap, theCacheTimeouts, false); 029 } 030 031 /** 032 * Constructor with configurable timeouts 033 * 034 * @param theWrap The validation support module to wrap 035 * @param theCacheTimeouts The timeouts to use 036 */ 037 public CachingValidationSupport( 038 IValidationSupport theWrap, 039 CacheTimeouts theCacheTimeouts, 040 boolean theIsEnabledValidationForCodingsLogicalAnd) { 041 super(theWrap.getFhirContext(), theWrap); 042 myIsEnabledValidationForCodingsLogicalAnd = theIsEnabledValidationForCodingsLogicalAnd; 043 } 044 045 /** 046 * @since 5.4.0 047 * @deprecated 048 */ 049 @Deprecated 050 public static class CacheTimeouts { 051 052 public CacheTimeouts setExpandValueSetMillis(long theExpandValueSetMillis) { 053 // nothing 054 return this; 055 } 056 057 public CacheTimeouts setTranslateCodeMillis(long theTranslateCodeMillis) { 058 // nothing 059 return this; 060 } 061 062 public CacheTimeouts setLookupCodeMillis(long theLookupCodeMillis) { 063 // nothibng 064 return this; 065 } 066 067 public CacheTimeouts setValidateCodeMillis(long theValidateCodeMillis) { 068 // nothing 069 return this; 070 } 071 072 public CacheTimeouts setMiscMillis(long theMiscMillis) { 073 // nothing 074 return this; 075 } 076 077 public static CacheTimeouts defaultValues() { 078 return new CacheTimeouts() 079 .setLookupCodeMillis(10 * DateUtils.MILLIS_PER_MINUTE) 080 .setExpandValueSetMillis(DateUtils.MILLIS_PER_MINUTE) 081 .setTranslateCodeMillis(10 * DateUtils.MILLIS_PER_MINUTE) 082 .setValidateCodeMillis(10 * DateUtils.MILLIS_PER_MINUTE) 083 .setMiscMillis(10 * DateUtils.MILLIS_PER_MINUTE); 084 } 085 } 086 087 @Override 088 public boolean isCodeableConceptValidationSuccessfulIfNotAllCodingsAreValid() { 089 return myIsEnabledValidationForCodingsLogicalAnd; 090 } 091}