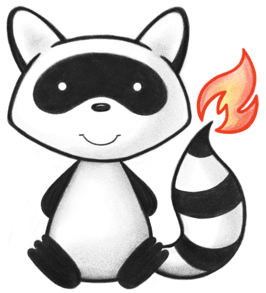
001package org.hl7.fhir.common.hapi.validation.validator; 002 003import ca.uhn.fhir.context.BaseRuntimeElementDefinition; 004import ca.uhn.fhir.context.FhirContext; 005import ca.uhn.fhir.context.RuntimeCompositeDatatypeDefinition; 006import ca.uhn.fhir.context.RuntimePrimitiveDatatypeDefinition; 007import ca.uhn.fhir.context.RuntimeResourceDefinition; 008import ca.uhn.fhir.i18n.Msg; 009import org.apache.commons.lang3.Validate; 010import org.hl7.fhir.exceptions.FHIRException; 011import org.hl7.fhir.r5.conformance.profile.BindingResolution; 012import org.hl7.fhir.r5.conformance.profile.ProfileKnowledgeProvider; 013import org.hl7.fhir.r5.model.ElementDefinition; 014import org.hl7.fhir.r5.model.Resource; 015import org.hl7.fhir.r5.model.StructureDefinition; 016 017public class ProfileKnowledgeWorkerR5 implements ProfileKnowledgeProvider { 018 private final FhirContext myCtx; 019 020 public ProfileKnowledgeWorkerR5(FhirContext theCtx) { 021 myCtx = theCtx; 022 } 023 024 @Override 025 public boolean isDatatype(String typeSimple) { 026 BaseRuntimeElementDefinition<?> def = myCtx.getElementDefinition(typeSimple); 027 Validate.notNull(typeSimple); 028 return (def instanceof RuntimePrimitiveDatatypeDefinition) 029 || (def instanceof RuntimeCompositeDatatypeDefinition); 030 } 031 032 @Override 033 public boolean isPrimitiveType(String typeSimple) { 034 BaseRuntimeElementDefinition<?> def = myCtx.getElementDefinition(typeSimple); 035 Validate.notNull(typeSimple); 036 return (def instanceof RuntimePrimitiveDatatypeDefinition); 037 } 038 039 @Override 040 public boolean isResource(String typeSimple) { 041 BaseRuntimeElementDefinition<?> def = myCtx.getElementDefinition(typeSimple); 042 Validate.notNull(typeSimple); 043 return def instanceof RuntimeResourceDefinition; 044 } 045 046 @Override 047 public boolean hasLinkFor(String typeSimple) { 048 return false; 049 } 050 051 @Override 052 public String getLinkFor(String corePath, String typeSimple) { 053 return null; 054 } 055 056 @Override 057 public BindingResolution resolveBinding( 058 StructureDefinition theStructureDefinition, 059 ElementDefinition.ElementDefinitionBindingComponent theElementDefinitionBindingComponent, 060 String theS) 061 throws FHIRException { 062 return null; 063 } 064 065 @Override 066 public BindingResolution resolveBinding(StructureDefinition theStructureDefinition, String theS, String theS1) 067 throws FHIRException { 068 return null; 069 } 070 071 @Override 072 public String getLinkForProfile(StructureDefinition theStructureDefinition, String theS) { 073 return null; 074 } 075 076 @Override 077 public boolean prependLinks() { 078 return false; 079 } 080 081 @Override 082 public String getLinkForUrl(String corePath, String url) { 083 throw new UnsupportedOperationException(Msg.code(693)); 084 } 085 086 @Override 087 public String getCanonicalForDefaultContext() { 088 return null; 089 } 090 091 @Override 092 public String getDefinitionsName(Resource r) { 093 return null; 094 } 095}