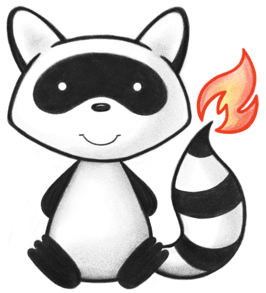
Package ca.uhn.fhir.model.api
Class Include
java.lang.Object
ca.uhn.fhir.model.api.Include
- All Implemented Interfaces:
Serializable
Represents a FHIR resource path specification, e.g.
Patient:name
Note on equality: This class uses value
and the recurse
properties to test
equality. Prior to HAPI 1.2 (and FHIR DSTU2) the recurse property did not exist, so this may merit consideration when
upgrading servers.
Note on thread safety: This class is not thread safe.
- See Also:
-
Constructor Summary
Constructors -
Method Summary
Modifier and TypeMethodDescriptionCreates a copy of this include with non-recurse behaviourCreates a copy of this include with recurse behaviourboolean
See the note on equality on theclass documentation
Returns the portion of the value after the first colon but before the second colonReturns the portion of the string after the second colon, or null if there are not two colons in the value.Returns the portion of the value before the first colongetValue()
int
hashCode()
See the note on equality on theclass documentation
boolean
isLocked()
Is this objectlocked
?boolean
setRecurse
(boolean theRecurse) Should this include recursevoid
toLocked()
Return a newtoString()
Creates and returns a new copy of this Include with the given type.
-
Constructor Details
-
Include
Constructor for non-recursive include- Parameters:
theValue
- The_include
value, e.g. "Patient:name"
-
Include
Constructor for an include- Parameters:
theValue
- The_include
value, e.g. "Patient:name"theIterate
- Should the include recurse
-
Include
Constructor for an include- Parameters:
theValue
- The_include
value, e.g. "Patient:name"theIterate
- Should the include recurse
-
-
Method Details
-
asNonRecursive
Creates a copy of this include with non-recurse behaviour -
asRecursive
Creates a copy of this include with recurse behaviour -
equals
See the note on equality on theclass documentation
-
getParamType
Returns the portion of the value before the first colon -
getParamName
Returns the portion of the value after the first colon but before the second colon -
getParamTargetType
Returns the portion of the string after the second colon, or null if there are not two colons in the value. -
getValue
-
hashCode
See the note on equality on theclass documentation
-
isLocked
Is this objectlocked
? -
isRecurse
-
setRecurse
Should this include recurse- Returns:
- Returns a reference to
this
for easy method chaining
-
setValue
-
toLocked
Return a new -
toString
-
withType
Creates and returns a new copy of this Include with the given type. The following table shows what will be returned:Initial Contents theResourceType Output Patient:careProvider Organization Patient:careProvider:Organization Patient:careProvider:Practitioner Organization Patient:careProvider:Organization Patient (any) IllegalStateException
- Parameters:
theResourceType
- The resource type (e.g. "Organization")- Returns:
- A new copy of the include. Note that if this include is
locked
, the returned include will be too
-