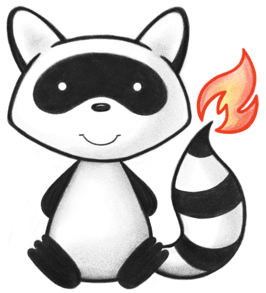
Package ca.uhn.fhir.rest.api
Class MethodOutcome
java.lang.Object
ca.uhn.fhir.rest.api.MethodOutcome
- All Implemented Interfaces:
IHasHeaders
-
Constructor Summary
ConstructorsConstructorDescriptionConstructorMethodOutcome
(IBaseOperationOutcome theOperationOutcome) ConstructorMethodOutcome
(IIdType theId) ConstructorMethodOutcome
(IIdType theId, Boolean theCreated) ConstructorMethodOutcome
(IIdType theId, IBaseOperationOutcome theBaseOperationOutcome) ConstructorMethodOutcome
(IIdType theId, IBaseOperationOutcome theBaseOperationOutcome, Boolean theCreated) Constructor -
Method Summary
Modifier and TypeMethodDescriptionvoid
Fires callbacks registered toregisterResourceViewCallback(Runnable)
and then clears the list of registered callbacks.This will be set toBoolean.TRUE
for instance of MethodOutcome which are returned to client instances, if the server has responded with an HTTP 201 Created.getFirstResponseHeader
(String theHeader) getId()
Returns theIBaseOperationOutcome
resource to return to the client ornull
if none.From a client response: If the method returned an actual resource body (e.g. a create/update with "Prefer: return=representation") this field will be populated with the resource itself.Gets the headers for the HTTP responseint
protected boolean
boolean
void
registerResourceViewCallback
(Runnable theCallback) Registers a callback to be invoked before the resource in this object gets returned to the client.setCreated
(Boolean theCreated) If not null, indicates whether the resource was created (as opposed to being updated).void
setCreatedUsingStatusCode
(int theResponseStatusCode) setOperationOutcome
(IBaseOperationOutcome theBaseOperationOutcome) Sets theIBaseOperationOutcome
resource to return to the client.setResource
(IBaseResource theResource) In a server response: This field may be populated in server code with the final resource for operations where a resource body is being created/updated.void
setResponseHeaders
(Map<String, List<String>> theResponseHeaders) Sets the headers for the HTTP responsevoid
setResponseStatusCode
(int theResponseStatusCode)
-
Constructor Details
-
MethodOutcome
public MethodOutcome()Constructor -
MethodOutcome
Constructor- Parameters:
theId
- The ID of the created/updated resourcetheCreated
- If not null, indicates whether the resource was created (as opposed to being updated). This is generally not needed, since the server can assume based on the method being called whether the result was a creation or an update. However, it can be useful if you are implementing an update method that does a create if the ID doesn't already exist.
-
MethodOutcome
Constructor- Parameters:
theId
- The ID of the created/updated resourcetheBaseOperationOutcome
- The operation outcome to return with the response (or null for none)
-
MethodOutcome
public MethodOutcome(IIdType theId, IBaseOperationOutcome theBaseOperationOutcome, Boolean theCreated) Constructor- Parameters:
theId
- The ID of the created/updated resourcetheBaseOperationOutcome
- The operation outcome to return with the response (or null for none)theCreated
- If not null, indicates whether the resource was created (as opposed to being updated). This is generally not needed, since the server can assume based on the method being called whether the result was a creation or an update. However, it can be useful if you are implementing an update method that does a create if the ID doesn't already exist.
-
MethodOutcome
Constructor- Parameters:
theId
- The ID of the created/updated resource
-
MethodOutcome
Constructor- Parameters:
theOperationOutcome
- The operation outcome resource to return
-
-
Method Details
-
getCreated
This will be set toBoolean.TRUE
for instance of MethodOutcome which are returned to client instances, if the server has responded with an HTTP 201 Created. -
setCreated
If not null, indicates whether the resource was created (as opposed to being updated). This is generally not needed, since the server can assume based on the method being called whether the result was a creation or an update. However, it can be useful if you are implementing an update method that does a create if the ID doesn't already exist.Users of HAPI should only interact with this method in Server applications
- Parameters:
theCreated
- If not null, indicates whether the resource was created (as opposed to being updated). This is generally not needed, since the server can assume based on the method being called whether the result was a creation or an update. However, it can be useful if you are implementing an update method that does a create if the ID doesn't already exist.- Returns:
- Returns a reference to
this
for easy method chaining
-
getId
-
setId
- Parameters:
theId
- The ID of the created/updated resource- Returns:
- Returns a reference to
this
for easy method chaining
-
getOperationOutcome
Returns theIBaseOperationOutcome
resource to return to the client ornull
if none.- Returns:
- This method will return null, unlike many methods in the API.
-
setOperationOutcome
Sets theIBaseOperationOutcome
resource to return to the client. Set tonull
(which is the default) if none.- Returns:
- Returns a reference to
this
for easy method chaining
-
getResource
From a client response: If the method returned an actual resource body (e.g. a create/update with "Prefer: return=representation") this field will be populated with the resource itself. -
setResource
In a server response: This field may be populated in server code with the final resource for operations where a resource body is being created/updated. E.g. for an update method, this field could be populated with the resource after the update is applied, with the new version ID, lastUpdate time, etc.This field is optional, but if it is populated the server will return the resource body if requested to do so via the HTTP Prefer header.
- Returns:
- Returns a reference to
this
for easy method chaining - See Also:
-
getResponseHeaders
Gets the headers for the HTTP response- Specified by:
getResponseHeaders
in interfaceIHasHeaders
-
setResponseHeaders
Sets the headers for the HTTP response -
getFirstResponseHeader
-
registerResourceViewCallback
Registers a callback to be invoked before the resource in this object gets returned to the client. Note that this is an experimental API and may change.- Parameters:
theCallback
- The callback- Since:
- 4.0.0
-
fireResourceViewCallbacks
Fires callbacks registered toregisterResourceViewCallback(Runnable)
and then clears the list of registered callbacks.- Since:
- 4.0.0
-
setCreatedUsingStatusCode
-
hasResource
-
setResponseStatusCode
-
getResponseStatusCode
-
isResponseStatusCodeSet
-