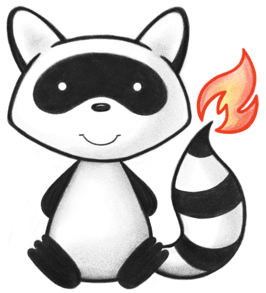
Package ca.uhn.fhir.util
Class BundleBuilder
java.lang.Object
ca.uhn.fhir.util.BundleBuilder
This class can be used to build a Bundle resource to be used as a FHIR transaction. Convenience methods provide
support for setting various bundle fields and working with bundle parts such as metadata and entry
(method and search).
This is not yet complete, and doesn't support all FHIR features. USE WITH CAUTION as the API may change.
- Since:
- 5.1.0
-
Nested Class Summary
Nested ClassesModifier and TypeClassDescriptionclass
class
class
class
class
class
-
Constructor Summary
Constructors -
Method Summary
Modifier and TypeMethodDescriptionvoid
addCollectionEntry
(IBaseResource theResource) Adds an entry for a Collection bundle typevoid
addDocumentEntry
(IBaseResource theResource) Adds an entry for a Document bundle typeaddEntry()
Creates new entry and adds it to the bundleCreates new search instance for the specified entry.void
addToEntry
(IBase theEntry, String theEntryChildName, IBase theValue) Sets the specified entry field.void
addToSearch
(IBase theSearch, String theSearchFieldName, IBase theSearchFieldValue) Sets the specified search field.addTransactionCreateEntry
(IBaseResource theResource) Adds an entry containing an create (POST) request.addTransactionCreateEntry
(IBaseResource theResource, String theFullUrl) Adds an entry containing an create (POST) request.void
addTransactionCreateEntryIdOnly
(IBaseResource theResource) Adds an entry containing a create (POST) request without the body of the resource.addTransactionDeleteConditionalEntry
(String theCondition) Adds an entry containing a delete (DELETE) request.addTransactionDeleteEntry
(String theResourceType, String theIdPart) Adds an entry containing a delete (DELETE) request.addTransactionDeleteEntry
(IBaseResource theResource) Adds an entry containing a delete (DELETE) request.addTransactionDeleteEntry
(IIdType theResourceId) Adds an entry containing a delete (DELETE) request.addTransactionDeleteEntryConditional
(String theMatchUrl) Adds an entry containing a delete (DELETE) request.addTransactionFhirPatchEntry
(IBaseParameters thePatch) Adds a FHIRPatch patch bundle to the transaction.addTransactionFhirPatchEntry
(IIdType theTarget, IBaseParameters thePatch) Adds a FHIRPatch patch bundle to the transactionaddTransactionUpdateEntry
(IBaseResource theResource) Adds an entry containing an update (PUT) request.void
addTransactionUpdateIdOnlyEntry
(IBaseResource theResource) Adds an entry containing an update (UPDATE) request without the body of the resource.<T extends IBaseBundle>
TConvenience method which auto-casts the results ofgetBundle()
<T> IPrimitiveType
<T> newPrimitive
(String theTypeName) Creates a new primitive.<T> IPrimitiveType
<T> newPrimitive
(String theTypeName, T theInitialValue) Creates a new primitive instance of the specified element type.setBundleField
(String theFieldName, String theFieldValue) Sets the specified primitive field on the bundle with the value provided.void
setIdentifier
(String theSystem, String theValue) Adds an identifier toBundle.identifier
setMetaField
(String theFieldName, IBase theFieldValue) Note that this method does not work for DSTU2 model classes, it will only work on DSTU3+.setSearchField
(IBase theSearch, String theFieldName, String theFieldValue) Sets the specified primitive field on the search entry with the value provided.setSearchField
(IBase theSearch, String theFieldName, IPrimitiveType<?> theFieldValue) void
setTimestamp
(IPrimitiveType<Date> theTimestamp) Sets the timestamp inBundle.timestamp
void
Sets a value forBundle.type
.
-
Constructor Details
-
BundleBuilder
Constructor
-
-
Method Details
-
setBundleField
Sets the specified primitive field on the bundle with the value provided.- Parameters:
theFieldName
- Name of the primitive field.theFieldValue
- Value of the field to be set.
-
setSearchField
Sets the specified primitive field on the search entry with the value provided.- Parameters:
theSearch
- Search part of the entrytheFieldName
- Name of the primitive field.theFieldValue
- Value of the field to be set.
-
setSearchField
public BundleBuilder setSearchField(IBase theSearch, String theFieldName, IPrimitiveType<?> theFieldValue) -
addTransactionFhirPatchEntry
public BundleBuilder.PatchBuilder addTransactionFhirPatchEntry(IIdType theTarget, IBaseParameters thePatch) Adds a FHIRPatch patch bundle to the transaction- Parameters:
theTarget
- The target resource ID to patchthePatch
- The FHIRPath Parameters resource- Since:
- 6.3.0
-
addTransactionFhirPatchEntry
Adds a FHIRPatch patch bundle to the transaction. This method is intended for conditional PATCH operations. If you know the ID of the resource you wish to patch, useaddTransactionFhirPatchEntry(IIdType, IBaseParameters)
instead.- Parameters:
thePatch
- The FHIRPath Parameters resource- Since:
- 6.3.0
- See Also:
-
addTransactionUpdateEntry
Adds an entry containing an update (PUT) request. Also sets the Bundle.type value to "transaction" if it is not already set.- Parameters:
theResource
- The resource to update
-
addTransactionUpdateIdOnlyEntry
Adds an entry containing an update (UPDATE) request without the body of the resource. Also sets the Bundle.type value to "transaction" if it is not already set.- Parameters:
theResource
- The resource to update.
-
addTransactionCreateEntry
Adds an entry containing an create (POST) request. Also sets the Bundle.type value to "transaction" if it is not already set.- Parameters:
theResource
- The resource to create
-
addTransactionCreateEntry
public BundleBuilder.CreateBuilder addTransactionCreateEntry(IBaseResource theResource, @Nullable String theFullUrl) Adds an entry containing an create (POST) request. Also sets the Bundle.type value to "transaction" if it is not already set.- Parameters:
theResource
- The resource to createtheFullUrl
- The fullUrl to attach to the entry. If null, will default to the resource ID.
-
addTransactionCreateEntryIdOnly
Adds an entry containing a create (POST) request without the body of the resource. Also sets the Bundle.type value to "transaction" if it is not already set.- Parameters:
theResource
- The resource to create
-
addTransactionDeleteConditionalEntry
Adds an entry containing a delete (DELETE) request. Also sets the Bundle.type value to "transaction" if it is not already set.Note that the resource is only used to extract its ID and type, and the body of the resource is not included in the entry,
- Parameters:
theCondition
- The conditional URL, e.g. "Patient?identifier=foo|bar"- Since:
- 6.8.0
-
addTransactionDeleteEntry
Adds an entry containing a delete (DELETE) request. Also sets the Bundle.type value to "transaction" if it is not already set.Note that the resource is only used to extract its ID and type, and the body of the resource is not included in the entry,
- Parameters:
theResource
- The resource to delete.
-
addTransactionDeleteEntry
Adds an entry containing a delete (DELETE) request. Also sets the Bundle.type value to "transaction" if it is not already set.Note that the resource is only used to extract its ID and type, and the body of the resource is not included in the entry,
- Parameters:
theResourceId
- The resource ID to delete.- Returns:
-
addTransactionDeleteEntry
public BundleBuilder.DeleteBuilder addTransactionDeleteEntry(String theResourceType, String theIdPart) Adds an entry containing a delete (DELETE) request. Also sets the Bundle.type value to "transaction" if it is not already set.- Parameters:
theResourceType
- The type resource to delete.theIdPart
- the ID of the resource to delete.
-
addTransactionDeleteEntryConditional
Adds an entry containing a delete (DELETE) request. Also sets the Bundle.type value to "transaction" if it is not already set.- Parameters:
theMatchUrl
- The match URL, e.g.Patient?identifier=http://foo|123
- Since:
- 6.3.0
-
addCollectionEntry
Adds an entry for a Collection bundle type -
addDocumentEntry
Adds an entry for a Document bundle type -
addEntry
Creates new entry and adds it to the bundle- Returns:
- Returns the new entry.
-
addSearch
Creates new search instance for the specified entry. Note that this method does not work for DSTU2 model classes, it will only work on DSTU3+.- Parameters:
entry
- Entry to create search instance for- Returns:
- Returns the search instance
-
addEntryAndReturnRequest
-
getBundle
-
getBundleTyped
Convenience method which auto-casts the results ofgetBundle()
- Since:
- 6.3.0
-
setMetaField
Note that this method does not work for DSTU2 model classes, it will only work on DSTU3+. -
addToEntry
Sets the specified entry field.- Parameters:
theEntry
- The entry instance to set values ontheEntryChildName
- The child field name of the entry instance to be settheValue
- The field value to set
-
addToSearch
Sets the specified search field.- Parameters:
theSearch
- The search instance to set values ontheSearchFieldName
- The child field name of the search instance to be settheSearchFieldValue
- The field value to set
-
newPrimitive
Creates a new primitive.- Type Parameters:
T
- Actual type of the parameterized primitive type interface- Parameters:
theTypeName
- The element type for the primitive- Returns:
- Returns the new empty instance of the element definition.
-
newPrimitive
Creates a new primitive instance of the specified element type.- Type Parameters:
T
- Actual type of the parameterized primitive type interface- Parameters:
theTypeName
- Element type to createtheInitialValue
- Initial value to be set on the new instance- Returns:
- Returns the newly created instance
-
setType
Sets a value forBundle.type
. That this is a coded field so theType must be an actual valid value for this field or aDataFormatException
will be thrown. -
setIdentifier
Adds an identifier toBundle.identifier
- Parameters:
theSystem
- The systemtheValue
- The value- Since:
- 6.4.0
-
setTimestamp
Sets the timestamp inBundle.timestamp
- Since:
- 6.4.0
-