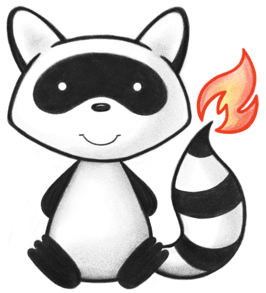
Interface IValidationSupport
- All Known Implementing Classes:
DefaultProfileValidationSupport
This interface is invoked directly by internal parts of the HAPI FHIR API, including the Validator and the FHIRPath evaluator. It is used to supply artifacts required for validation (e.g. StructureDefinition resources, ValueSet resources, etc.) and also to provide terminology functions such as code validation, ValueSet expansion, etc.
Implementations are not required to implement all of the functions
in this interface; in fact it is expected that most won't. Any
methods which are not implemented may simply return null
and calling code is expected to be able to handle this. Generally, a
series of implementations of this interface will be joined together using
the
ValidationSupportChain
class.
See Validation Support Modules
for information on how to assemble and configure implementations of this interface. See also
the org.hl7.fhir.common.hapi.validation.support
package summary
in the hapi-fhir-validation
module for many implementations of this interface.
- Since:
- 5.0.0
-
Nested Class Summary
Nested ClassesModifier and TypeInterfaceDescriptionstatic class
static class
static class
This is a hapi-fhir internal version agnostic object holding information about a validation issue.static class
Defines codes in system http://hl7.org/fhir/issue-type.static class
Defines codes that can be part of the details of an issue.static class
Holds information about the details of aIValidationSupport.CodeValidationIssue
.static class
This is a hapi-fhir internal version agnostic object holding information about the validation result.static class
static class
static class
static enum
Defines codes in system http://hl7.org/fhir/issue-severity.static class
static class
static class
static class
-
Field Summary
Fields -
Method Summary
Modifier and TypeMethodDescriptionexpandValueSet
(ValidationSupportContext theValidationSupportContext, ValueSetExpansionOptions theExpansionOptions, String theValueSetUrlToExpand) Expands the given portion of a ValueSet by canonical URL.expandValueSet
(ValidationSupportContext theValidationSupportContext, ValueSetExpansionOptions theExpansionOptions, IBaseResource theValueSetToExpand) Expands the given portion of a ValueSetdefault List
<IBaseResource> Load and return all conformance resources associated with this validation support module.default <T extends IBaseResource>
List<T> Load and return all possible structure definitions aside from resource definitions themselvesdefault <T extends IBaseResource>
List<T> Load and return all possible search parametersdefault <T extends IBaseResource>
List<T> Load and return all possible structure definitionsdefault byte[]
fetchBinary
(String binaryKey) Fetch the given binary data by key.default IBaseResource
fetchCodeSystem
(String theSystem) Fetch a code system by IDdefault <T extends IBaseResource>
TfetchResource
(Class<T> theClass, String theUri) Loads a resource needed by the validation (a StructureDefinition, or a ValueSet)default IBaseResource
fetchStructureDefinition
(String theUrl) default IBaseResource
fetchValueSet
(String theValueSetUrl) Fetch the given ValueSet by URL, or returns null if one can't be found for the given URLdefault IBaseResource
generateSnapshot
(ValidationSupportContext theValidationSupportContext, IBaseResource theInput, String theUrl, String theWebUrl, String theProfileName) Generate a snapshot from the given differential profile.Returns the FHIR Context associated with this moduledefault String
getName()
This field is used by the Terminology Troubleshooting Log to log which validation support module was used for the operation being logged.default void
This method clears any temporary caches within the validation support.default boolean
When validating a CodeableConcept containing multiple codings, this method can be used to control whether the validator requires all codings in the CodeableConcept to be valid in order to consider the CodeableConcept valid.default boolean
isCodeSystemSupported
(ValidationSupportContext theValidationSupportContext, String theSystem) Returnstrue
if codes in the given code system can be expanded or validateddefault boolean
Returnstrue
if a Remote Terminology Service is currently configureddefault boolean
isValueSetSupported
(ValidationSupportContext theValidationSupportContext, String theValueSetUrl) Returnstrue
if the given ValueSet can be validated by the given validation support modulelookupCode
(ValidationSupportContext theValidationSupportContext, LookupCodeRequest theLookupCodeRequest) Look up a code using the system, code and other parameters captured inLookupCodeRequest
.lookupCode
(ValidationSupportContext theValidationSupportContext, String theSystem, String theCode) Deprecated.This method has been deprecated in HAPI FHIR 7.0.0.lookupCode
(ValidationSupportContext theValidationSupportContext, String theSystem, String theCode, String theDisplayLanguage) Deprecated.This method has been deprecated in HAPI FHIR 7.0.0.default TranslateConceptResults
Attempt to translate the given concept from one code system to anothervalidateCode
(ValidationSupportContext theValidationSupportContext, ConceptValidationOptions theOptions, String theCodeSystem, String theCode, String theDisplay, String theValueSetUrl) Validates that the given code exists and if possible returns a display name.validateCodeInValueSet
(ValidationSupportContext theValidationSupportContext, ConceptValidationOptions theOptions, String theCodeSystem, String theCode, String theDisplay, IBaseResource theValueSet) Validates that the given code exists and if possible returns a display name.
-
Field Details
-
URL_PREFIX_VALUE_SET
- See Also:
-
TYPE_STRING
- See Also:
-
TYPE_BOOLEAN
- See Also:
-
TYPE_CODING
- See Also:
-
TYPE_GROUP
- See Also:
-
-
Method Details
-
expandValueSet
@Nullable default IValidationSupport.ValueSetExpansionOutcome expandValueSet(ValidationSupportContext theValidationSupportContext, @Nullable ValueSetExpansionOptions theExpansionOptions, @Nonnull IBaseResource theValueSetToExpand) Expands the given portion of a ValueSet- Parameters:
theValidationSupportContext
- The validation support module will be passed in to this method. This is convenient in cases where the operation needs to make calls to other method in the support chain, so that they can be passed through the entire chain. Implementations of this interface may always safely ignore this parameter.theExpansionOptions
- If provided (can benull
), contains options controlling the expansiontheValueSetToExpand
- The valueset that should be expanded- Returns:
- The expansion, or null
-
expandValueSet
@Nullable default IValidationSupport.ValueSetExpansionOutcome expandValueSet(ValidationSupportContext theValidationSupportContext, @Nullable ValueSetExpansionOptions theExpansionOptions, @Nonnull String theValueSetUrlToExpand) throws ResourceNotFoundException Expands the given portion of a ValueSet by canonical URL.- Parameters:
theValidationSupportContext
- The validation support module will be passed in to this method. This is convenient in cases where the operation needs to make calls to other method in the support chain, so that they can be passed through the entire chain. Implementations of this interface may always safely ignore this parameter.theExpansionOptions
- If provided (can benull
), contains options controlling the expansiontheValueSetUrlToExpand
- The valueset that should be expanded- Returns:
- The expansion, or null
- Throws:
ResourceNotFoundException
- If no ValueSet can be found with the given URL- Since:
- 6.0.0
-
fetchAllConformanceResources
Load and return all conformance resources associated with this validation support module. This method may return null if it doesn't make sense for a given module. -
fetchAllSearchParameters
Load and return all possible search parameters- Since:
- 6.6.0
-
fetchAllStructureDefinitions
Load and return all possible structure definitions -
fetchAllNonBaseStructureDefinitions
Load and return all possible structure definitions aside from resource definitions themselves -
fetchCodeSystem
Fetch a code system by ID- Parameters:
theSystem
- The code system- Returns:
- The valueset (must not be null, but can be an empty ValueSet)
-
fetchResource
@Nullable default <T extends IBaseResource> T fetchResource(@Nullable Class<T> theClass, String theUri) Loads a resource needed by the validation (a StructureDefinition, or a ValueSet)Note: Since 5.3.0, theClass can be null
- Parameters:
theClass
- The type of the resource to load, ornull
to return any resource with the given canonical URItheUri
- The resource URI- Returns:
- Returns the resource, or
null
if no resource with the given URI can be found
-
fetchStructureDefinition
-
isCodeSystemSupported
default boolean isCodeSystemSupported(ValidationSupportContext theValidationSupportContext, String theSystem) Returnstrue
if codes in the given code system can be expanded or validated- Parameters:
theValidationSupportContext
- The validation support module will be passed in to this method. This is convenient in cases where the operation needs to make calls to other method in the support chain, so that they can be passed through the entire chain. Implementations of this interface may always safely ignore this parameter.theSystem
- The URI for the code system, e.g."http://loinc.org"
- Returns:
- Returns
true
if codes in the given code system can be validated
-
isRemoteTerminologyServiceConfigured
Returnstrue
if a Remote Terminology Service is currently configured- Returns:
- Returns
true
if a Remote Terminology Service is currently configured
-
fetchValueSet
Fetch the given ValueSet by URL, or returns null if one can't be found for the given URL -
fetchBinary
Fetch the given binary data by key.- Parameters:
binaryKey
-- Returns:
-
validateCode
@Nullable default IValidationSupport.CodeValidationResult validateCode(ValidationSupportContext theValidationSupportContext, ConceptValidationOptions theOptions, String theCodeSystem, String theCode, String theDisplay, String theValueSetUrl) Validates that the given code exists and if possible returns a display name. This method is called to check codes which are found in "example" binding fields (e.g.Observation.code
) in the default profile.- Parameters:
theValidationSupportContext
- The validation support module will be passed in to this method. This is convenient in cases where the operation needs to make calls to other method in the support chain, so that they can be passed through the entire chain. Implementations of this interface may always safely ignore this parameter.theOptions
- Provides options controlling the validationtheCodeSystem
- The code system, e.g. "http://loinc.org
"theCode
- The code, e.g. "1234-5
"theDisplay
- The display name, if it should also be validated- Returns:
- Returns a validation result object
-
validateCodeInValueSet
@Nullable default IValidationSupport.CodeValidationResult validateCodeInValueSet(ValidationSupportContext theValidationSupportContext, ConceptValidationOptions theOptions, String theCodeSystem, String theCode, String theDisplay, @Nonnull IBaseResource theValueSet) Validates that the given code exists and if possible returns a display name. This method is called to check codes which are found in "example" binding fields (e.g.Observation.code
) in the default profile.- Parameters:
theValidationSupportContext
- The validation support module will be passed in to this method. This is convenient in cases where the operation needs to make calls to other method in the support chain, so that they can be passed through the entire chain. Implementations of this interface may always safely ignore this parameter.theCodeSystem
- The code system, e.g. "http://loinc.org
"theCode
- The code, e.g. "1234-5
"theDisplay
- The display name, if it should also be validatedtheValueSet
- The ValueSet to validate against. Must not be null, and must be a ValueSet resource.- Returns:
- Returns a validation result object, or
null
if this validation support module can not handle this kind of request
-
lookupCode
@Deprecated @Nullable default IValidationSupport.LookupCodeResult lookupCode(ValidationSupportContext theValidationSupportContext, String theSystem, String theCode, String theDisplayLanguage) Deprecated.This method has been deprecated in HAPI FHIR 7.0.0. UselookupCode(ValidationSupportContext, LookupCodeRequest)
instead.Look up a code using the system and code value.- Parameters:
theValidationSupportContext
- The validation support module will be passed in to this method. This is convenient in cases where the operation needs to make calls to other method in the support chain, so that they can be passed through the entire chain. Implementations of this interface may always safely ignore this parameter.theSystem
- The CodeSystem URLtheCode
- The codetheDisplayLanguage
- Used to filter out the designation by the display language. To return all designation, set this value tonull
.
-
lookupCode
@Deprecated @Nullable default IValidationSupport.LookupCodeResult lookupCode(ValidationSupportContext theValidationSupportContext, String theSystem, String theCode) Deprecated.This method has been deprecated in HAPI FHIR 7.0.0. UselookupCode(ValidationSupportContext, LookupCodeRequest)
instead.Look up a code using the system and code value- Parameters:
theValidationSupportContext
- The validation support module will be passed in to this method. This is convenient in cases where the operation needs to make calls to other method in the support chain, so that they can be passed through the entire chain. Implementations of this interface may always safely ignore this parameter.theSystem
- The CodeSystem URLtheCode
- The code
-
lookupCode
@Nullable default IValidationSupport.LookupCodeResult lookupCode(ValidationSupportContext theValidationSupportContext, @Nonnull LookupCodeRequest theLookupCodeRequest) Look up a code using the system, code and other parameters captured inLookupCodeRequest
.- Parameters:
theValidationSupportContext
- The validation support module will be passed in to this method. This is convenient in cases where the operation needs to make calls to other method in the support chain, so that they can be passed through the entire chain. Implementations of this interface may always safely ignore this parameter.theLookupCodeRequest
- The parameters used to perform the lookup, including system and code.- Since:
- 7.0.0
-
isValueSetSupported
default boolean isValueSetSupported(ValidationSupportContext theValidationSupportContext, String theValueSetUrl) Returnstrue
if the given ValueSet can be validated by the given validation support module- Parameters:
theValidationSupportContext
- The validation support module will be passed in to this method. This is convenient in cases where the operation needs to make calls to other method in the support chain, so that they can be passed through the entire chain. Implementations of this interface may always safely ignore this parameter.theValueSetUrl
- The ValueSet canonical URL
-
generateSnapshot
@Nullable default IBaseResource generateSnapshot(ValidationSupportContext theValidationSupportContext, IBaseResource theInput, String theUrl, String theWebUrl, String theProfileName) Generate a snapshot from the given differential profile.- Parameters:
theValidationSupportContext
- The validation support module will be passed in to this method. This is convenient in cases where the operation needs to make calls to other method in the support chain, so that they can be passed through the entire chain. Implementations of this interface may always safely ignore this parameter.- Returns:
- Returns null if this module does not know how to handle this request
-
getFhirContext
Returns the FHIR Context associated with this module -
invalidateCaches
This method clears any temporary caches within the validation support. It is mainly intended for unit tests, but could be used in non-test scenarios as well. -
translateConcept
@Nullable default TranslateConceptResults translateConcept(IValidationSupport.TranslateCodeRequest theRequest) Attempt to translate the given concept from one code system to another -
getName
This field is used by the Terminology Troubleshooting Log to log which validation support module was used for the operation being logged. -
isCodeableConceptValidationSuccessfulIfNotAllCodingsAreValid
When validating a CodeableConcept containing multiple codings, this method can be used to control whether the validator requires all codings in the CodeableConcept to be valid in order to consider the CodeableConcept valid.See VersionSpecificWorkerContextWrapper#validateCode in hapi-fhir-validation, and the refer to the values below for the behaviour associated with each value.
- If
false
(default setting) the validation for codings will return a positive result only if ALL codings are valid. - If
true
the validation for codings will return a positive result if ANY codings are valid.
- Returns:
- true or false depending on the desired coding validation behaviour.
- If
-