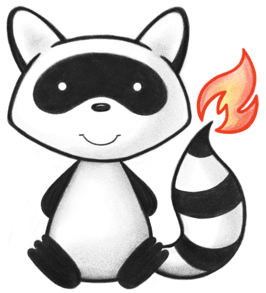
Class ValidationSupportChain
- All Implemented Interfaces:
ca.uhn.fhir.context.support.IValidationSupport
The following chaining logic is used:
-
Calls to fetchAll... methods such as
fetchAllConformanceResources()
andfetchAllStructureDefinitions()
will call every method in the chain in order, and aggregate the results into a single list to return. -
Calls to fetch or validate codes, such as
validateCode(ValidationSupportContext, ConceptValidationOptions, String, String, String, String)
andlookupCode(ValidationSupportContext, LookupCodeRequest)
will first test each module in the chain using theisCodeSystemSupported(ValidationSupportContext, String)
orisValueSetSupported(ValidationSupportContext, String)
methods (depending on whether a ValueSet URL is present in the method parameters) and will invoke any methods in the chain which return that they can handle the given CodeSystem/ValueSet URL. The first non-null value returned by a method in the chain that can support the URL will be returned to the caller. - All other methods will invoke each method in the chain in order, and will stop processing and return immediately as soon as the first non-null value is returned.
The following caching logic is used if caching is enabled using ValidationSupportChain.CacheConfiguration
.
You can use ValidationSupportChain.CacheConfiguration.disabled()
if you want to disable caching.
-
Calls to fetch StructureDefinitions including
fetchAllStructureDefinitions()
andfetchStructureDefinition(String)
are cached in a non-expiring cache. This is because theFhirInstanceValidator
module makes assumptions that these objects will not change for the lifetime of the validator for performance reasons. -
Calls to all other fetchAll... methods including
fetchAllConformanceResources()
andfetchAllSearchParameters()
cache their results in an expiring cache, but will refresh that cache asynchronously. -
Results of
generateSnapshot(ValidationSupportContext, IBaseResource, String, String, String)
are not cached, since this method is generally called in contexts where the results are cached. - Results of all other methods are stored in an expiring cache.
Note that caching functionality used to be provided by a separate provider called CachingValidationSupport but that functionality has been moved into this class as of HAPI FHIR 8.0.0, because it is possible to provide a more efficient chain when these functions are combined.
-
Nested Class Summary
Nested ClassesNested classes/interfaces inherited from interface ca.uhn.fhir.context.support.IValidationSupport
ca.uhn.fhir.context.support.IValidationSupport.BaseConceptProperty, ca.uhn.fhir.context.support.IValidationSupport.CodeValidationIssue, ca.uhn.fhir.context.support.IValidationSupport.CodeValidationIssueCode, ca.uhn.fhir.context.support.IValidationSupport.CodeValidationIssueCoding, ca.uhn.fhir.context.support.IValidationSupport.CodeValidationIssueDetails, ca.uhn.fhir.context.support.IValidationSupport.CodeValidationResult, ca.uhn.fhir.context.support.IValidationSupport.CodingConceptProperty, ca.uhn.fhir.context.support.IValidationSupport.ConceptDesignation, ca.uhn.fhir.context.support.IValidationSupport.GroupConceptProperty, ca.uhn.fhir.context.support.IValidationSupport.IssueSeverity, ca.uhn.fhir.context.support.IValidationSupport.LookupCodeResult, ca.uhn.fhir.context.support.IValidationSupport.StringConceptProperty, ca.uhn.fhir.context.support.IValidationSupport.TranslateCodeRequest, ca.uhn.fhir.context.support.IValidationSupport.ValueSetExpansionOutcome
-
Field Summary
FieldsModifier and TypeFieldDescriptionstatic final ca.uhn.fhir.context.support.ValueSetExpansionOptions
Fields inherited from interface ca.uhn.fhir.context.support.IValidationSupport
TYPE_CODING, TYPE_GROUP, TYPE_STRING, URL_PREFIX_VALUE_SET
-
Constructor Summary
ConstructorsConstructorDescriptionConstructor which initializes the chain with no modules (modules must subsequently be registered usingaddValidationSupport(IValidationSupport)
).ValidationSupportChain
(ca.uhn.fhir.context.support.IValidationSupport... theValidationSupportModules) Constructor which initializes the chain with the given modules.ValidationSupportChain
(List<ca.uhn.fhir.context.support.IValidationSupport> theValidationSupportModules) Constructor which initializes the chain with the given modules.ValidationSupportChain
(ValidationSupportChain.CacheConfiguration theCacheConfiguration, ca.uhn.fhir.context.support.IValidationSupport... theValidationSupportModules) ConstructorValidationSupportChain
(ValidationSupportChain.CacheConfiguration theCacheConfiguration, List<ca.uhn.fhir.context.support.IValidationSupport> theValidationSupportModules) Constructor -
Method Summary
Modifier and TypeMethodDescriptionvoid
addValidationSupport
(int theIndex, ca.uhn.fhir.context.support.IValidationSupport theValidationSupport) Add a validation support module to the chain at the given index.void
addValidationSupport
(ca.uhn.fhir.context.support.IValidationSupport theValidationSupport) Add a validation support module to the chain.ca.uhn.fhir.context.support.IValidationSupport.ValueSetExpansionOutcome
expandValueSet
(ca.uhn.fhir.context.support.ValidationSupportContext theValidationSupportContext, ca.uhn.fhir.context.support.ValueSetExpansionOptions theExpansionOptions, String theValueSetUrlToExpand) ca.uhn.fhir.context.support.IValidationSupport.ValueSetExpansionOutcome
expandValueSet
(ca.uhn.fhir.context.support.ValidationSupportContext theValidationSupportContext, ca.uhn.fhir.context.support.ValueSetExpansionOptions theExpansionOptions, org.hl7.fhir.instance.model.api.IBaseResource theValueSetToExpand) List
<org.hl7.fhir.instance.model.api.IBaseResource> List
<org.hl7.fhir.instance.model.api.IBaseResource> <T extends org.hl7.fhir.instance.model.api.IBaseResource>
List<T> List
<org.hl7.fhir.instance.model.api.IBaseResource> byte[]
fetchBinary
(String theKey) org.hl7.fhir.instance.model.api.IBaseResource
fetchCodeSystem
(String theSystem) <T extends org.hl7.fhir.instance.model.api.IBaseResource>
TfetchResource
(Class<T> theClass, String theUri) org.hl7.fhir.instance.model.api.IBaseResource
fetchStructureDefinition
(String theUrl) org.hl7.fhir.instance.model.api.IBaseResource
fetchValueSet
(String theUrl) org.hl7.fhir.instance.model.api.IBaseResource
generateSnapshot
(ca.uhn.fhir.context.support.ValidationSupportContext theValidationSupportContext, org.hl7.fhir.instance.model.api.IBaseResource theInput, String theUrl, String theWebUrl, String theProfileName) ca.uhn.fhir.context.FhirContext
getName()
List
<ca.uhn.fhir.context.support.IValidationSupport> Returns a view of theIValidationSupport
modules within this chain.void
void
Invalidate the expiring cache, but not the permanent StructureDefinition cacheboolean
boolean
isCodeSystemSupported
(ca.uhn.fhir.context.support.ValidationSupportContext theValidationSupportContext, String theSystem) boolean
boolean
isValueSetSupported
(ca.uhn.fhir.context.support.ValidationSupportContext theValidationSupportContext, String theValueSetUrl) void
ca.uhn.fhir.context.support.IValidationSupport.LookupCodeResult
lookupCode
(ca.uhn.fhir.context.support.ValidationSupportContext theValidationSupportContext, ca.uhn.fhir.context.support.LookupCodeRequest theLookupCodeRequest) void
removeValidationSupport
(ca.uhn.fhir.context.support.IValidationSupport theValidationSupport) Removes an item from the chain.setCodeableConceptValidationSuccessfulIfNotAllCodingsAreValid
(boolean theEnabledValidationForCodingsLogicalAnd) When validating a CodeableConcept containing multiple codings, this method can be used to control whether the validator requires all codings in the CodeableConcept to be valid in order to consider the CodeableConcept valid.void
Sets a name for this chain.void
start()
void
stop()
ca.uhn.fhir.context.support.TranslateConceptResults
translateConcept
(ca.uhn.fhir.context.support.IValidationSupport.TranslateCodeRequest theRequest) ca.uhn.fhir.context.support.IValidationSupport.CodeValidationResult
validateCode
(ca.uhn.fhir.context.support.ValidationSupportContext theValidationSupportContext, ca.uhn.fhir.context.support.ConceptValidationOptions theOptions, String theCodeSystem, String theCode, String theDisplay, String theValueSetUrl) ca.uhn.fhir.context.support.IValidationSupport.CodeValidationResult
validateCodeInValueSet
(ca.uhn.fhir.context.support.ValidationSupportContext theValidationSupportContext, ca.uhn.fhir.context.support.ConceptValidationOptions theOptions, String theCodeSystem, String theCode, String theDisplay, org.hl7.fhir.instance.model.api.IBaseResource theValueSet) Methods inherited from class java.lang.Object
clone, equals, finalize, getClass, hashCode, notify, notifyAll, toString, wait, wait, wait
Methods inherited from interface ca.uhn.fhir.context.support.IValidationSupport
lookupCode, lookupCode
-
Field Details
-
EMPTY_EXPANSION_OPTIONS
-
-
Constructor Details
-
ValidationSupportChain
public ValidationSupportChain()Constructor which initializes the chain with no modules (modules must subsequently be registered usingaddValidationSupport(IValidationSupport)
). The cache will be enabled usingValidationSupportChain.CacheConfiguration.defaultValues()
. -
ValidationSupportChain
public ValidationSupportChain(ca.uhn.fhir.context.support.IValidationSupport... theValidationSupportModules) Constructor which initializes the chain with the given modules. The cache will be enabled usingValidationSupportChain.CacheConfiguration.defaultValues()
. -
ValidationSupportChain
public ValidationSupportChain(List<ca.uhn.fhir.context.support.IValidationSupport> theValidationSupportModules) Constructor which initializes the chain with the given modules. The cache will be enabled usingValidationSupportChain.CacheConfiguration.defaultValues()
. -
ValidationSupportChain
public ValidationSupportChain(@Nonnull ValidationSupportChain.CacheConfiguration theCacheConfiguration, ca.uhn.fhir.context.support.IValidationSupport... theValidationSupportModules) Constructor- Parameters:
theCacheConfiguration
- The caching configurationtheValidationSupportModules
- The initial modules to add to the chain
-
ValidationSupportChain
public ValidationSupportChain(@Nonnull ValidationSupportChain.CacheConfiguration theCacheConfiguration, @Nonnull List<ca.uhn.fhir.context.support.IValidationSupport> theValidationSupportModules) Constructor- Parameters:
theCacheConfiguration
- The caching configurationtheValidationSupportModules
- The initial modules to add to the chain
-
-
Method Details
-
getName
- Specified by:
getName
in interfaceca.uhn.fhir.context.support.IValidationSupport
-
setName
Sets a name for this chain. This name will be returned bygetName()
and used by OpenTelemetry. -
start
-
stop
-
isCodeableConceptValidationSuccessfulIfNotAllCodingsAreValid
- Specified by:
isCodeableConceptValidationSuccessfulIfNotAllCodingsAreValid
in interfaceca.uhn.fhir.context.support.IValidationSupport
-
setCodeableConceptValidationSuccessfulIfNotAllCodingsAreValid
public ValidationSupportChain setCodeableConceptValidationSuccessfulIfNotAllCodingsAreValid(boolean theEnabledValidationForCodingsLogicalAnd) When validating a CodeableConcept containing multiple codings, this method can be used to control whether the validator requires all codings in the CodeableConcept to be valid in order to consider the CodeableConcept valid.See VersionSpecificWorkerContextWrapper#validateCode in hapi-fhir-validation, and the refer to the values below for the behaviour associated with each value.
- If
false
(default setting) the validation for codings will return a positive result only if ALL codings are valid. - If
true
the validation for codings will return a positive result if ANY codings are valid.
- Returns:
- true or false depending on the desired coding validation behaviour.
- If
-
translateConcept
public ca.uhn.fhir.context.support.TranslateConceptResults translateConcept(ca.uhn.fhir.context.support.IValidationSupport.TranslateCodeRequest theRequest) - Specified by:
translateConcept
in interfaceca.uhn.fhir.context.support.IValidationSupport
-
invalidateCaches
- Specified by:
invalidateCaches
in interfaceca.uhn.fhir.context.support.IValidationSupport
-
invalidateExpiringCaches
Invalidate the expiring cache, but not the permanent StructureDefinition cache- Since:
- 8.0.0
-
isValueSetSupported
public boolean isValueSetSupported(ca.uhn.fhir.context.support.ValidationSupportContext theValidationSupportContext, String theValueSetUrl) - Specified by:
isValueSetSupported
in interfaceca.uhn.fhir.context.support.IValidationSupport
-
generateSnapshot
public org.hl7.fhir.instance.model.api.IBaseResource generateSnapshot(ca.uhn.fhir.context.support.ValidationSupportContext theValidationSupportContext, org.hl7.fhir.instance.model.api.IBaseResource theInput, String theUrl, String theWebUrl, String theProfileName) - Specified by:
generateSnapshot
in interfaceca.uhn.fhir.context.support.IValidationSupport
-
getFhirContext
- Specified by:
getFhirContext
in interfaceca.uhn.fhir.context.support.IValidationSupport
-
addValidationSupport
public void addValidationSupport(ca.uhn.fhir.context.support.IValidationSupport theValidationSupport) Add a validation support module to the chain.Note that this method is not thread-safe. All validation support modules should be added prior to use.
- Parameters:
theValidationSupport
- The validation support. Must not be null, and must have aFhirContext
that is configured for the same FHIR version as other entries in the chain.
-
addValidationSupport
public void addValidationSupport(int theIndex, ca.uhn.fhir.context.support.IValidationSupport theValidationSupport) Add a validation support module to the chain at the given index.Note that this method is not thread-safe. All validation support modules should be added prior to use.
- Parameters:
theIndex
- The index to add totheValidationSupport
- The validation support. Must not be null, and must have aFhirContext
that is configured for the same FHIR version as other entries in the chain.
-
removeValidationSupport
public void removeValidationSupport(ca.uhn.fhir.context.support.IValidationSupport theValidationSupport) Removes an item from the chain. Note that this method is mostly intended for testing. Removing items from the chain while validation is actually occurring is not an expected use case for this class. -
expandValueSet
@Nullable public ca.uhn.fhir.context.support.IValidationSupport.ValueSetExpansionOutcome expandValueSet(ca.uhn.fhir.context.support.ValidationSupportContext theValidationSupportContext, @Nullable ca.uhn.fhir.context.support.ValueSetExpansionOptions theExpansionOptions, @Nonnull String theValueSetUrlToExpand) throws ca.uhn.fhir.rest.server.exceptions.ResourceNotFoundException - Specified by:
expandValueSet
in interfaceca.uhn.fhir.context.support.IValidationSupport
- Throws:
ca.uhn.fhir.rest.server.exceptions.ResourceNotFoundException
-
expandValueSet
public ca.uhn.fhir.context.support.IValidationSupport.ValueSetExpansionOutcome expandValueSet(ca.uhn.fhir.context.support.ValidationSupportContext theValidationSupportContext, ca.uhn.fhir.context.support.ValueSetExpansionOptions theExpansionOptions, @Nonnull org.hl7.fhir.instance.model.api.IBaseResource theValueSetToExpand) - Specified by:
expandValueSet
in interfaceca.uhn.fhir.context.support.IValidationSupport
-
isRemoteTerminologyServiceConfigured
- Specified by:
isRemoteTerminologyServiceConfigured
in interfaceca.uhn.fhir.context.support.IValidationSupport
-
fetchAllConformanceResources
- Specified by:
fetchAllConformanceResources
in interfaceca.uhn.fhir.context.support.IValidationSupport
-
fetchAllStructureDefinitions
- Specified by:
fetchAllStructureDefinitions
in interfaceca.uhn.fhir.context.support.IValidationSupport
-
fetchAllNonBaseStructureDefinitions
- Specified by:
fetchAllNonBaseStructureDefinitions
in interfaceca.uhn.fhir.context.support.IValidationSupport
-
fetchAllSearchParameters
@Nullable public <T extends org.hl7.fhir.instance.model.api.IBaseResource> List<T> fetchAllSearchParameters()- Specified by:
fetchAllSearchParameters
in interfaceca.uhn.fhir.context.support.IValidationSupport
-
fetchCodeSystem
- Specified by:
fetchCodeSystem
in interfaceca.uhn.fhir.context.support.IValidationSupport
-
fetchValueSet
- Specified by:
fetchValueSet
in interfaceca.uhn.fhir.context.support.IValidationSupport
-
fetchResource
public <T extends org.hl7.fhir.instance.model.api.IBaseResource> T fetchResource(Class<T> theClass, String theUri) - Specified by:
fetchResource
in interfaceca.uhn.fhir.context.support.IValidationSupport
-
fetchBinary
- Specified by:
fetchBinary
in interfaceca.uhn.fhir.context.support.IValidationSupport
-
fetchStructureDefinition
- Specified by:
fetchStructureDefinition
in interfaceca.uhn.fhir.context.support.IValidationSupport
-
isCodeSystemSupported
public boolean isCodeSystemSupported(ca.uhn.fhir.context.support.ValidationSupportContext theValidationSupportContext, String theSystem) - Specified by:
isCodeSystemSupported
in interfaceca.uhn.fhir.context.support.IValidationSupport
-
validateCode
public ca.uhn.fhir.context.support.IValidationSupport.CodeValidationResult validateCode(@Nonnull ca.uhn.fhir.context.support.ValidationSupportContext theValidationSupportContext, @Nonnull ca.uhn.fhir.context.support.ConceptValidationOptions theOptions, String theCodeSystem, String theCode, String theDisplay, String theValueSetUrl) - Specified by:
validateCode
in interfaceca.uhn.fhir.context.support.IValidationSupport
-
validateCodeInValueSet
public ca.uhn.fhir.context.support.IValidationSupport.CodeValidationResult validateCodeInValueSet(ca.uhn.fhir.context.support.ValidationSupportContext theValidationSupportContext, ca.uhn.fhir.context.support.ConceptValidationOptions theOptions, String theCodeSystem, String theCode, String theDisplay, @Nonnull org.hl7.fhir.instance.model.api.IBaseResource theValueSet) - Specified by:
validateCodeInValueSet
in interfaceca.uhn.fhir.context.support.IValidationSupport
-
lookupCode
public ca.uhn.fhir.context.support.IValidationSupport.LookupCodeResult lookupCode(ca.uhn.fhir.context.support.ValidationSupportContext theValidationSupportContext, @Nonnull ca.uhn.fhir.context.support.LookupCodeRequest theLookupCodeRequest) - Specified by:
lookupCode
in interfaceca.uhn.fhir.context.support.IValidationSupport
-
getValidationSupports
Returns a view of theIValidationSupport
modules within this chain. The returned collection is unmodifiable and will reflect changes to the underlying list.- Since:
- 8.0.0
-
logCacheSizes
-