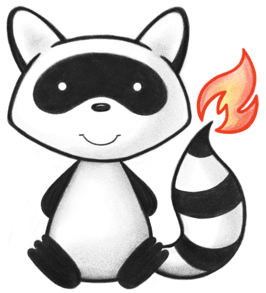
Interface Repository
This API is under-going active development, so it should be considered beta-level.
This interface is a Java rendition of the FHIR REST API. All FHIR operations are defined at the HTTP level, which is convenient from the specification point-of-view since FHIR is built on top of web standards. This does mean that a few HTTP specific considerations, such as transmitting side-band information through the HTTP headers, bleeds into this API.
One particularly odd case are FHIR Bundle links. The specification describes these as opaque to
the end-user, so a given FHIR repository implementation must be able to resolve those directly.
See link(Class, String)
This interface also chooses to ignore return headers for most cases, preferring to return the
Java objects directly. In cases where this is not possible, or the additional headers are crucial
information, HAPI's MethodOutcome
is used.
Implementations of this interface should prefer to throw the exceptions derived from
BaseServerResponseException
All operations may throw AuthenticationException
, ForbiddenOperationException
, or
InternalErrorException
in addition to operation-specific exceptions.
If a given operation is not supported, implementations should throw an
NotImplementedOperationException
. The capabilities operation, if supported, should return
the set of supported interactions. If capabilities is not supported, the components in this
repository will try to invoke operations with "sensible" defaults. For example, by using the
standard FHIR search parameters. Discussion is on-going to determine what a "sensible" minimal
level of support for interactions should be.
- See Also:
-
Method Summary
Modifier and TypeMethodDescriptiondefault <C extends IBaseConformance>
Ccapabilities
(Class<C> resourceType) Returns the CapabilityStatement/Conformance metadata for this repositorydefault <C extends IBaseConformance>
Ccapabilities
(Class<C> resourceType, Map<String, String> headers) Returns the CapabilityStatement/Conformance metadata for this repositorydefault <T extends IBaseResource>
MethodOutcomecreate
(T resource) Creates a Resource in the repository<T extends IBaseResource>
MethodOutcomeCreates a Resource in the repositorydefault <T extends IBaseResource,
I extends IIdType>
MethodOutcomeDeletes a Resource in the repository<T extends IBaseResource,
I extends IIdType>
MethodOutcomeDeletes a Resource in the repositoryReturns theFhirContext
used by the repository Practically, implementing FHIR functionality with the HAPI toolset requires a FhirContext.default <B extends IBaseBundle,
P extends IBaseParameters, I extends IIdType>
BReturns a Bundle with instance-level historydefault <B extends IBaseBundle,
P extends IBaseParameters, I extends IIdType>
BReturns a Bundle with instance-level historydefault <B extends IBaseBundle,
P extends IBaseParameters, T extends IBaseResource>
BReturns a Bundle with type-level history for this repositorydefault <B extends IBaseBundle,
P extends IBaseParameters, T extends IBaseResource>
BReturns a Bundle with type-level history for this repositorydefault <B extends IBaseBundle,
P extends IBaseParameters>
BReturns a Bundle with server-level history for this repositorydefault <B extends IBaseBundle,
P extends IBaseParameters>
BReturns a Bundle with server-level history for this repositorydefault <P extends IBaseParameters,
I extends IIdType>
MethodOutcomeInvokes an instance-level operation on this repositorydefault <R extends IBaseResource,
P extends IBaseParameters, I extends IIdType>
RInvokes an instance-level operation on this repository that returns a Resource<R extends IBaseResource,
P extends IBaseParameters, I extends IIdType>
RInvokes an instance-level operation on this repository that returns a Resourcedefault <P extends IBaseParameters,
I extends IIdType>
MethodOutcomeInvokes an instance-level operation on this repositorydefault <P extends IBaseParameters,
T extends IBaseResource>
MethodOutcomeInvokes a type-level operation on this repositorydefault <R extends IBaseResource,
P extends IBaseParameters, T extends IBaseResource>
RInvokes a type-level operation on this repository that returns a Resource<R extends IBaseResource,
P extends IBaseParameters, T extends IBaseResource>
Rinvoke
(Class<T> resourceType, String name, P parameters, Class<R> returnType, Map<String, String> headers) Invokes a type-level operation on this repository that returns a Resourcedefault <P extends IBaseParameters,
T extends IBaseResource>
MethodOutcomeInvokes a type-level operation on this repositorydefault <P extends IBaseParameters>
MethodOutcomeInvokes a server-level operation on this repositorydefault <R extends IBaseResource,
P extends IBaseParameters>
RInvokes a server-level operation on this repository that returns a Resourcedefault <R extends IBaseResource,
P extends IBaseParameters>
RInvokes a server-level operation on this repository that returns a Resourcedefault <P extends IBaseParameters>
MethodOutcomeInvokes a server-level operation on this repositorydefault <B extends IBaseBundle>
BReads a Bundle from a link on this repository This is typically used for paging during searchesdefault <B extends IBaseBundle>
BReads a Bundle from a link on this repository This is typically used for paging during searchesdefault <I extends IIdType,
P extends IBaseParameters>
MethodOutcomepatch
(I id, P patchParameters) Patches a Resource in the repositorydefault <I extends IIdType,
P extends IBaseParameters>
MethodOutcomePatches a Resource in the repositorydefault <T extends IBaseResource,
I extends IIdType>
TReads a resource from the repository<T extends IBaseResource,
I extends IIdType>
TReads a Resource from the repositorydefault <B extends IBaseBundle,
T extends IBaseResource>
Bsearch
(Class<B> bundleType, Class<T> resourceType, Map<String, List<IQueryParameterType>> searchParameters) Searches this repository<B extends IBaseBundle,
T extends IBaseResource>
Bsearch
(Class<B> bundleType, Class<T> resourceType, Map<String, List<IQueryParameterType>> searchParameters, Map<String, String> headers) Searches this repositorydefault <B extends IBaseBundle>
Btransaction
(B transaction) Performs a transaction or batch on this repositorydefault <B extends IBaseBundle>
Btransaction
(B transaction, Map<String, String> headers) Performs a transaction or batch on this repositorydefault <T extends IBaseResource>
MethodOutcomeupdate
(T resource) Updates a Resource in the repository<T extends IBaseResource>
MethodOutcomeUpdates a Resource in the repository
-
Method Details
-
read
Reads a resource from the repository- Type Parameters:
T
- a Resource typeI
- an Id type- Parameters:
resourceType
- the class of the Resource type to readid
- the id of the Resource to read- Returns:
- the Resource
- See Also:
-
read
<T extends IBaseResource,I extends IIdType> T read(Class<T> resourceType, I id, Map<String, String> headers) Reads a Resource from the repository- Type Parameters:
T
- a Resource typeI
- an Id type- Parameters:
resourceType
- the class of the Resource type to readid
- the id of the Resource to readheaders
- headers for this request, typically key-value pairs of HTTP headers- Returns:
- the Resource
- See Also:
-
create
Creates a Resource in the repository- Type Parameters:
T
- a Resource type- Parameters:
resource
- the Resource to create- Returns:
- a MethodOutcome with the id of the created Resource
- See Also:
-
create
Creates a Resource in the repository- Type Parameters:
T
- a Resource type- Parameters:
resource
- the Resource to createheaders
- headers for this request, typically key-value pairs of HTTP headers- Returns:
- a MethodOutcome with the id of the created Resource
- See Also:
-
patch
Patches a Resource in the repository- Type Parameters:
I
- an Id typeP
- a Parameters type- Parameters:
id
- the id of the Resource to patchpatchParameters
- parameters describing the patches to apply- Returns:
- a MethodOutcome with the id of the patched resource
- See Also:
-
patch
default <I extends IIdType,P extends IBaseParameters> MethodOutcome patch(I id, P patchParameters, Map<String, String> headers) Patches a Resource in the repository- Type Parameters:
I
- an Id typeP
- a Parameters type- Parameters:
id
- the id of the Resource to patchpatchParameters
- parameters describing the patches to applyheaders
- headers for this request, typically key-value pairs of HTTP headers- Returns:
- a MethodOutcome with the id of the patched resource
- See Also:
-
update
Updates a Resource in the repository- Type Parameters:
T
- a Resource type- Parameters:
resource
- the Resource to update- Returns:
- a MethodOutcome with the id of the updated Resource
- See Also:
-
update
Updates a Resource in the repository- Type Parameters:
T
- a Resource type- Parameters:
resource
- the Resource to updateheaders
- headers for this request, typically key-value pairs of HTTP headers- Returns:
- a MethodOutcome with the id of the updated Resource
- See Also:
-
delete
default <T extends IBaseResource,I extends IIdType> MethodOutcome delete(Class<T> resourceType, I id) Deletes a Resource in the repository- Type Parameters:
T
- a Resource typeI
- an Id type- Parameters:
resourceType
- the class of the Resource type to deleteid
- the id of the Resource to delete- Returns:
- a MethodOutcome with the id of the deleted resource
- See Also:
-
delete
<T extends IBaseResource,I extends IIdType> MethodOutcome delete(Class<T> resourceType, I id, Map<String, String> headers) Deletes a Resource in the repository- Type Parameters:
T
- a Resource typeI
- an Id type- Parameters:
resourceType
- the class of the Resource type to deleteid
- the id of the Resource to deleteheaders
- headers for this request, typically key-value pairs of HTTP headers- Returns:
- a MethodOutcome with the id of the deleted resource
- See Also:
-
search
default <B extends IBaseBundle,T extends IBaseResource> B search(Class<B> bundleType, Class<T> resourceType, Map<String, List<IQueryParameterType>> searchParameters) Searches this repository- Type Parameters:
B
- a Bundle typeT
- a Resource type- Parameters:
bundleType
- the class of the Bundle type to returnresourceType
- the class of the Resource type to searchsearchParameters
- the searchParameters for this search- Returns:
- a Bundle with the results of the search
- See Also:
-
search
<B extends IBaseBundle,T extends IBaseResource> B search(Class<B> bundleType, Class<T> resourceType, Map<String, List<IQueryParameterType>> searchParameters, Map<String, String> headers) Searches this repository- Type Parameters:
B
- a Bundle typeT
- a Resource type- Parameters:
bundleType
- the class of the Bundle type to returnresourceType
- the class of the Resource type to searchsearchParameters
- the searchParameters for this searchheaders
- headers for this request, typically key-value pairs of HTTP headers- Returns:
- a Bundle with the results of the search
- See Also:
-
link
Reads a Bundle from a link on this repository This is typically used for paging during searches- Type Parameters:
B
- a Bundle type- Parameters:
url
- the url of the Bundle to load- Returns:
- a Bundle
- See Also:
-
link
Reads a Bundle from a link on this repository This is typically used for paging during searches- Type Parameters:
B
- a Bundle type- Parameters:
url
- the url of the Bundle to loadheaders
- headers for this request, typically key-value pairs of HTTP headers- Returns:
- a Bundle
- See Also:
-
capabilities
Returns the CapabilityStatement/Conformance metadata for this repository- Type Parameters:
C
- a CapabilityStatement/Conformance type- Parameters:
resourceType
- the class of the CapabilityStatement/Conformance to return- Returns:
- a CapabilityStatement/Conformance with the repository's metadata
- See Also:
-
capabilities
default <C extends IBaseConformance> C capabilities(Class<C> resourceType, Map<String, String> headers) Returns the CapabilityStatement/Conformance metadata for this repository- Type Parameters:
C
- a CapabilityStatement/Conformance type- Parameters:
resourceType
- the class of the CapabilityStatement/Conformance to returnheaders
- headers for this request, typically key-value pairs of HTTP headers- Returns:
- a CapabilityStatement/Conformance with the repository's metadata
- See Also:
-
transaction
Performs a transaction or batch on this repository- Type Parameters:
B
- a Bundle type- Parameters:
transaction
- a Bundle with the transaction/batch- Returns:
- a Bundle with the results of the transaction/batch
- See Also:
-
transaction
Performs a transaction or batch on this repository- Type Parameters:
B
- a Bundle type- Parameters:
transaction
- a Bundle with the transaction/batchheaders
- headers for this request, typically key-value pairs of HTTP headers- Returns:
- a Bundle with the results of the transaction/batch
- See Also:
-
invoke
default <R extends IBaseResource,P extends IBaseParameters> R invoke(String name, P parameters, Class<R> returnType) Invokes a server-level operation on this repository that returns a Resource- Type Parameters:
R
- a Resource type to returnP
- a Parameters type for operation parameters- Parameters:
name
- the name of the operation to invokeparameters
- the operation parametersreturnType
- the class of the Resource the operation returns- Returns:
- the results of the operation
- See Also:
-
invoke
default <R extends IBaseResource,P extends IBaseParameters> R invoke(String name, P parameters, Class<R> returnType, Map<String, String> headers) Invokes a server-level operation on this repository that returns a Resource- Type Parameters:
R
- a Resource type to returnP
- a Parameters type for operation parameters- Parameters:
name
- the name of the operation to invokeparameters
- the operation parametersreturnType
- the class of the Resource the operation returnsheaders
- headers for this request, typically key-value pairs of HTTP headers- Returns:
- the results of the operation
- See Also:
-
invoke
Invokes a server-level operation on this repository- Type Parameters:
P
- a Parameters type for operation parameters- Parameters:
name
- the name of the operation to invokeparameters
- the operation parameters- Returns:
- a MethodOutcome with a status code
- See Also:
-
invoke
default <P extends IBaseParameters> MethodOutcome invoke(String name, P parameters, Map<String, String> headers) Invokes a server-level operation on this repository- Type Parameters:
P
- a Parameters type for operation parameters- Parameters:
name
- the name of the operation to invokeparameters
- the operation parametersheaders
- headers for this request, typically key-value pairs of HTTP headers- Returns:
- a MethodOutcome with a status code
- See Also:
-
invoke
default <R extends IBaseResource,P extends IBaseParameters, R invokeT extends IBaseResource> (Class<T> resourceType, String name, P parameters, Class<R> returnType) Invokes a type-level operation on this repository that returns a Resource- Type Parameters:
R
- a Resource type to returnP
- a Parameters type for operation parametersT
- a Resource type to do the invocation for- Parameters:
resourceType
- the class of the Resource to do the invocation forname
- the name of the operation to invokeparameters
- the operation parametersreturnType
- the class of the Resource the operation returns- Returns:
- the results of the operation
- See Also:
-
invoke
<R extends IBaseResource,P extends IBaseParameters, R invokeT extends IBaseResource> (Class<T> resourceType, String name, P parameters, Class<R> returnType, Map<String, String> headers) Invokes a type-level operation on this repository that returns a Resource- Type Parameters:
R
- a Resource type to returnP
- a Parameters type for operation parametersT
- a Resource type to do the invocation for- Parameters:
resourceType
- the class of the Resource to do the invocation forname
- the name of the operation to invokeparameters
- the operation parametersreturnType
- the class of the Resource the operation returnsheaders
- headers for this request, typically key-value pairs of HTTP headers- Returns:
- the results of the operation
- See Also:
-
invoke
default <P extends IBaseParameters,T extends IBaseResource> MethodOutcome invoke(Class<T> resourceType, String name, P parameters) Invokes a type-level operation on this repository- Type Parameters:
P
- a Parameters type for operation parametersT
- a Resource type to do the invocation for- Parameters:
resourceType
- the class of the Resource to do the invocation forname
- the name of the operation to invokeparameters
- the operation parameters- Returns:
- a MethodOutcome with a status code
- See Also:
-
invoke
default <P extends IBaseParameters,T extends IBaseResource> MethodOutcome invoke(Class<T> resourceType, String name, P parameters, Map<String, String> headers) Invokes a type-level operation on this repository- Type Parameters:
P
- a Parameters type for operation parametersT
- a Resource type to do the invocation for- Parameters:
resourceType
- the class of the Resource to do the invocation forname
- the name of the operation to invokeparameters
- the operation parametersheaders
- headers for this request, typically key-value pairs of HTTP headers- Returns:
- a MethodOutcome with a status code
- See Also:
-
invoke
default <R extends IBaseResource,P extends IBaseParameters, R invokeI extends IIdType> (I id, String name, P parameters, Class<R> returnType) Invokes an instance-level operation on this repository that returns a Resource- Type Parameters:
R
- a Resource type to returnP
- a Parameters type for operation parametersI
- an Id type- Parameters:
id
- the id of the Resource to do the invocation onname
- the name of the operation to invokeparameters
- the operation parametersreturnType
- the class of the Resource the operation returns- Returns:
- the results of the operation
- See Also:
-
invoke
<R extends IBaseResource,P extends IBaseParameters, R invokeI extends IIdType> (I id, String name, P parameters, Class<R> returnType, Map<String, String> headers) Invokes an instance-level operation on this repository that returns a Resource- Type Parameters:
R
- a Resource type to returnP
- a Parameters type for operation parametersI
- an Id type- Parameters:
id
- the id of the Resource to do the invocation onname
- the name of the operation to invokeparameters
- the operation parametersreturnType
- the class of the Resource the operation returnsheaders
- headers for this request, typically key-value pairs of HTTP headers- Returns:
- the results of the operation
- See Also:
-
invoke
default <P extends IBaseParameters,I extends IIdType> MethodOutcome invoke(I id, String name, P parameters) Invokes an instance-level operation on this repository- Type Parameters:
P
- a Parameters type for operation parametersI
- an Id type- Parameters:
id
- the id of the Resource to do the invocation onname
- the name of the operation to invokeparameters
- the operation parameters- Returns:
- a MethodOutcome with a status code
- See Also:
-
invoke
default <P extends IBaseParameters,I extends IIdType> MethodOutcome invoke(I id, String name, P parameters, Map<String, String> headers) Invokes an instance-level operation on this repository- Type Parameters:
P
- a Parameters type for operation parametersI
- an Id type- Parameters:
id
- the id of the Resource to do the invocation onname
- the name of the operation to invokeparameters
- the operation parametersheaders
- headers for this request, typically key-value pairs of HTTP headers- Returns:
- a MethodOutcome with a status code
- See Also:
-
history
default <B extends IBaseBundle,P extends IBaseParameters> B history(P parameters, Class<B> returnType) Returns a Bundle with server-level history for this repository- Type Parameters:
B
- a Bundle type to returnP
- a Parameters type for input parameters- Parameters:
parameters
- the parameters for this history interactionreturnType
- the class of the Bundle type to return- Returns:
- a Bundle with the server history
- See Also:
-
history
default <B extends IBaseBundle,P extends IBaseParameters> B history(P parameters, Class<B> returnType, Map<String, String> headers) Returns a Bundle with server-level history for this repository- Type Parameters:
B
- a Bundle type to returnP
- a Parameters type for input parameters- Parameters:
parameters
- the parameters for this history interactionreturnType
- the class of the Bundle type to returnheaders
- headers for this request, typically key-value pairs of HTTP headers- Returns:
- a Bundle with the server history
- See Also:
-
history
default <B extends IBaseBundle,P extends IBaseParameters, B historyT extends IBaseResource> (Class<T> resourceType, P parameters, Class<B> returnType) Returns a Bundle with type-level history for this repository- Type Parameters:
B
- a Bundle type to returnP
- a Parameters type for input parametersT
- a Resource type to produce history for- Parameters:
resourceType
- the class of the Resource type to produce history forparameters
- the parameters for this history interactionreturnType
- the class of the Bundle type to return- Returns:
- a Bundle with the type history
- See Also:
-
history
default <B extends IBaseBundle,P extends IBaseParameters, B historyT extends IBaseResource> (Class<T> resourceType, P parameters, Class<B> returnType, Map<String, String> headers) Returns a Bundle with type-level history for this repository- Type Parameters:
B
- a Bundle type to returnP
- a Parameters type for input parametersT
- a Resource type to produce history for- Parameters:
resourceType
- the class of the Resource type to produce history forparameters
- the parameters for this history interactionreturnType
- the class of the Bundle type to returnheaders
- headers for this request, typically key-value pairs of HTTP headers- Returns:
- a Bundle with the type history
- See Also:
-
history
default <B extends IBaseBundle,P extends IBaseParameters, B historyI extends IIdType> (I id, P parameters, Class<B> returnType) Returns a Bundle with instance-level history- Type Parameters:
B
- a Bundle type to returnP
- a Parameters type for input parametersI
- an Id type for the Resource to produce history for- Parameters:
id
- the id of the Resource type to produce history forparameters
- the parameters for this history interactionreturnType
- the class of the Bundle type to return- Returns:
- a Bundle with the instance history
- See Also:
-
history
default <B extends IBaseBundle,P extends IBaseParameters, B historyI extends IIdType> (I id, P parameters, Class<B> returnType, Map<String, String> headers) Returns a Bundle with instance-level history- Type Parameters:
B
- a Bundle type to returnP
- a Parameters type for input parametersI
- an Id type for the Resource to produce history for- Parameters:
id
- the id of the Resource type to produce history forparameters
- the parameters for this history interactionreturnType
- the class of the Bundle type to returnheaders
- headers for this request, typically key-value pairs of HTTP headers- Returns:
- a Bundle with the instance history
- See Also:
-
fhirContext
Returns theFhirContext
used by the repository Practically, implementing FHIR functionality with the HAPI toolset requires a FhirContext. In particular for things like version independent code. Ideally, a user could which FHIR version a repository was configured for using things like the CapabilityStatement. In practice, that's not widely implemented (yet) and it's expensive to create a new context with every call. We will probably revisit this in the future.- Returns:
- a FhirContext
-