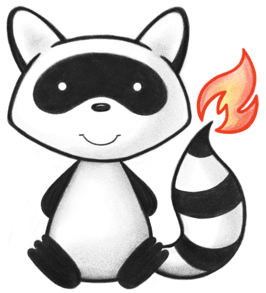
Class FhirTerser
-
Nested Class Summary
Nested Classes -
Constructor Summary
Constructors -
Method Summary
Modifier and TypeMethodDescription<T extends IBase>
TaddElement
(IBase theTarget, String thePath) Adds and returns a new element at the given path within the given structure.<T extends IBase>
TaddElement
(IBase theTarget, String thePath, String theValue) Adds and returns a new element at the given path within the given structure.void
addElements
(IBase theTarget, String thePath, Collection<String> theValues) This method has the same semantics asaddElement(IBase, String, String)
but adds a collection of primitives instead of a single one.void
clear
(IBaseResource theInput) Clear all content on a resource<T extends IBaseResource>
Tclone
(T theSource) Clones a resource object, copying all data elements from theSource into a new copy of the same type.Clones all values from a source object into the equivalent fields in a target objectcontainResources
(IBaseResource theResource, FhirTerser.ContainedResources theParentContainedResources, boolean theStoreResults) Iterate through the whole resource and identify any contained resources.getAllEmbeddedResources
(IBaseResource theResource, boolean theRecurse) Returns all embedded resources that are found embedded withintheResource
.getAllPopulatedChildElementsOfType
(IBaseResource theResource, Class<T> theType) Returns a list containing all child elements (including the resource itself) which are non-empty and are either of the exact type specified, or are a subclass of that type.getAllResourceReferences
(IBaseResource theResource) Extracts all outbound references from a resourcegetAllResourceReferencesExcluding
(IBaseResource theResource, List<String> thePathsToExclude) Extracts all outbound references from a resource, excluding any that are located on black-listed parts of the resourcegetCompartmentOwnersForResource
(String theCompartmentName, IBaseResource theSource, Set<String> theAdditionalCompartmentParamNames) Returns the owners of the compartment intheSource
is in the compartment namedtheCompartmentName
.getCompartmentReferencesForResource
(String theCompartmentName, IBaseResource theSource, Set<String> theAdditionalCompartmentParamNames) getDefinition
(Class<? extends IBaseResource> theResourceType, String thePath) getSinglePrimitiveValue
(IBase theTarget, String thePath) getSinglePrimitiveValueOrNull
(IBase theTarget, String thePath) getSingleValue
(IBase theTarget, String thePath, Class<T> theWantedType) getSingleValueOrNull
(IBase theTarget, String thePath) <T extends IBase>
TgetSingleValueOrNull
(IBase theTarget, String thePath, Class<T> theWantedType) Returns values stored in an element identified by its path.Returns values stored in an element identified by its path.Returns values stored in an element identified by its path.Returns values stored in an element identified by its path.Returns values stored in an element identified by its path.getValues
(IBase theElement, String thePath, Class<T> theWantedClass, boolean theCreate, boolean theAddExtension) Returns values stored in an element identified by its path.boolean
isSourceInCompartmentForTarget
(String theCompartmentName, IBaseResource theSource, IIdType theTarget) Returnstrue
iftheSource
is in the compartment namedtheCompartmentName
belonging to resourcetheTarget
boolean
isSourceInCompartmentForTarget
(String theCompartmentName, IBaseResource theSource, IIdType theTarget, Set<String> theAdditionalCompartmentParamNames) Returnstrue
iftheSource
is in the compartment namedtheCompartmentName
belonging to resourcetheTarget
newMap()
<T extends IBase>
TsetElement
(IBase theTarget, String thePath, String theValue) Adds and returns a new element at the given path within the given structure.void
visit
(IBase theElement, IModelVisitor2 theVisitor) Visit all elements in a given resource or elementvoid
visit
(IBaseResource theResource, IModelVisitor theVisitor) Visit all elements in a given resource
-
Constructor Details
-
FhirTerser
-
-
Method Details
-
cloneInto
Clones all values from a source object into the equivalent fields in a target object- Parameters:
theSource
- The source object (must not be null)theTarget
- The target object to copy values into (must not be null)theIgnoreMissingFields
- The ignore fields in the target which do not exist (if false, an exception will be thrown if the target is unable to accept a value from the source)- Returns:
- Returns the target (which will be the same object that was passed into theTarget) for easy chaining
-
getAllPopulatedChildElementsOfType
public <T extends IBase> List<T> getAllPopulatedChildElementsOfType(IBaseResource theResource, Class<T> theType) Returns a list containing all child elements (including the resource itself) which are non-empty and are either of the exact type specified, or are a subclass of that type.For example, specifying a type of
StringDt
would return all non-empty string instances within the message. Specifying a type ofIResource
would return the resource itself, as well as any contained resources.Note on scope: This method will descend into any contained resources (
IResource.getContained()
) as well, but will not descend into linked resources (e.g.BaseResourceReferenceDt.getResource()
) or embedded resources (e.g. Bundle.entry.resource)- Parameters:
theResource
- The resource instance to search. Must not be null.theType
- The type to search for. Must not be null.- Returns:
- Returns a list of all matching elements
-
getAllResourceReferences
Extracts all outbound references from a resource- Parameters:
theResource
- the resource to be analyzed- Returns:
- a list of references to other resources
-
getAllResourceReferencesExcluding
public List<ResourceReferenceInfo> getAllResourceReferencesExcluding(IBaseResource theResource, List<String> thePathsToExclude) Extracts all outbound references from a resource, excluding any that are located on black-listed parts of the resource- Parameters:
theResource
- the resource to be analyzedthePathsToExclude
- a list of dot-delimited paths not to include in the result- Returns:
- a list of references to other resources
-
getDefinition
public BaseRuntimeChildDefinition getDefinition(Class<? extends IBaseResource> theResourceType, String thePath) -
getSingleValueOrNull
-
getSingleValueOrNull
public <T extends IBase> T getSingleValueOrNull(IBase theTarget, String thePath, Class<T> theWantedType) -
getSinglePrimitiveValue
-
getSinglePrimitiveValueOrNull
-
getSingleValue
-
getValues
Returns values stored in an element identified by its path. The list of values is of typeObject
.- Parameters:
theElement
- The element to be accessed. Must not be null.thePath
- The path for the element to be accessed.@param theElement The resource instance to be accessed. Must not be null.- Returns:
- A list of values of type
Object
.
-
getValues
Returns values stored in an element identified by its path. The list of values is of typeObject
.- Parameters:
theElement
- The element to be accessed. Must not be null.thePath
- The path for the element to be accessed.theCreate
- When set totrue
, the terser will create a null-valued element where none exists.- Returns:
- A list of values of type
Object
.
-
getValues
public List<IBase> getValues(IBase theElement, String thePath, boolean theCreate, boolean theAddExtension) Returns values stored in an element identified by its path. The list of values is of typeObject
.- Parameters:
theElement
- The element to be accessed. Must not be null.thePath
- The path for the element to be accessed.theCreate
- When set totrue
, the terser will create a null-valued element where none exists.theAddExtension
- When set totrue
, the terser will add a null-valued extension where one or more such extensions already exist.- Returns:
- A list of values of type
Object
.
-
getValues
public <T extends IBase> List<T> getValues(IBase theElement, String thePath, Class<T> theWantedClass) Returns values stored in an element identified by its path. The list of values is of typetheWantedClass
.- Type Parameters:
T
- Type declared bytheWantedClass
- Parameters:
theElement
- The element to be accessed. Must not be null.thePath
- The path for the element to be accessed.theWantedClass
- The desired class to be returned in a list.- Returns:
- A list of values of type
theWantedClass
.
-
getValues
public <T extends IBase> List<T> getValues(IBase theElement, String thePath, Class<T> theWantedClass, boolean theCreate) Returns values stored in an element identified by its path. The list of values is of typetheWantedClass
.- Type Parameters:
T
- Type declared bytheWantedClass
- Parameters:
theElement
- The element to be accessed. Must not be null.thePath
- The path for the element to be accessed.theWantedClass
- The desired class to be returned in a list.theCreate
- When set totrue
, the terser will create a null-valued element where none exists.- Returns:
- A list of values of type
theWantedClass
.
-
getValues
public <T extends IBase> List<T> getValues(IBase theElement, String thePath, Class<T> theWantedClass, boolean theCreate, boolean theAddExtension) Returns values stored in an element identified by its path. The list of values is of typetheWantedClass
.- Type Parameters:
T
- Type declared bytheWantedClass
- Parameters:
theElement
- The element to be accessed. Must not be null.thePath
- The path for the element to be accessed.theWantedClass
- The desired class to be returned in a list.theCreate
- When set totrue
, the terser will create a null-valued element where none exists.theAddExtension
- When set totrue
, the terser will add a null-valued extension where one or more such extensions already exist.- Returns:
- A list of values of type
theWantedClass
.
-
isSourceInCompartmentForTarget
public boolean isSourceInCompartmentForTarget(String theCompartmentName, IBaseResource theSource, IIdType theTarget) Returnstrue
iftheSource
is in the compartment namedtheCompartmentName
belonging to resourcetheTarget
- Parameters:
theCompartmentName
- The name of the compartmenttheSource
- The potential member of the compartmenttheTarget
- The owner of the compartment. Note that both the resource type and ID must be filled in on this IIdType or the method will throw anIllegalArgumentException
- Returns:
true
iftheSource
is in the compartment- Throws:
IllegalArgumentException
- If theTarget does not contain both a resource type and ID
-
isSourceInCompartmentForTarget
public boolean isSourceInCompartmentForTarget(String theCompartmentName, IBaseResource theSource, IIdType theTarget, @Nullable Set<String> theAdditionalCompartmentParamNames) Returnstrue
iftheSource
is in the compartment namedtheCompartmentName
belonging to resourcetheTarget
- Parameters:
theCompartmentName
- The name of the compartmenttheSource
- The potential member of the compartmenttheTarget
- The owner of the compartment. Note that both the resource type and ID must be filled in on this IIdType or the method will throw anIllegalArgumentException
theAdditionalCompartmentParamNames
- If provided, search param names provided here will be considered as included in the given compartment for this comparison.- Returns:
true
iftheSource
is in the compartment or one of the additional parameters matched.- Throws:
IllegalArgumentException
- If theTarget does not contain both a resource type and ID
-
getCompartmentOwnersForResource
@Nonnull public List<IIdType> getCompartmentOwnersForResource(String theCompartmentName, IBaseResource theSource, Set<String> theAdditionalCompartmentParamNames) Returns the owners of the compartment intheSource
is in the compartment namedtheCompartmentName
.- Parameters:
theCompartmentName
- The name of the compartmenttheSource
- The potential member of the compartmenttheAdditionalCompartmentParamNames
- If provided, search param names provided here will be considered as included in the given compartment for this comparison.
-
getCompartmentReferencesForResource
@Nonnull public Stream<IBaseReference> getCompartmentReferencesForResource(String theCompartmentName, IBaseResource theSource, @Nullable Set<String> theAdditionalCompartmentParamNames) -
visit
Visit all elements in a given resourceNote on scope: This method will descend into any contained resources (
IResource.getContained()
) as well, but will not descend into linked resources (e.g.BaseResourceReferenceDt.getResource()
) or embedded resources (e.g. Bundle.entry.resource)- Parameters:
theResource
- The resource to visittheVisitor
- The visitor
-
newMap
-
visit
Visit all elements in a given resource or elementTHIS ALTERNATE METHOD IS STILL EXPERIMENTAL! USE WITH CAUTION
Note on scope: This method will descend into any contained resources (
IResource.getContained()
) as well, but will not descend into linked resources (e.g.BaseResourceReferenceDt.getResource()
) or embedded resources (e.g. Bundle.entry.resource)- Parameters:
theElement
- The element to visittheVisitor
- The visitor
-
getAllEmbeddedResources
public Collection<IBaseResource> getAllEmbeddedResources(IBaseResource theResource, boolean theRecurse) Returns all embedded resources that are found embedded withintheResource
. An embedded resource is a resource that can be found as a direct child within a resource, as opposed to being referenced by the resource.Examples include resources found within
Bundle.entry.resource
andParameters.parameter.resource
, as well as contained resources found withinResource.contained
- Parameters:
theRecurse
- Should embedded resources be recursively scanned for further embedded resources- Returns:
- A collection containing the embedded resources. Order is arbitrary.
-
clear
Clear all content on a resource -
containResources
public FhirTerser.ContainedResources containResources(IBaseResource theResource, FhirTerser.ContainedResources theParentContainedResources, boolean theStoreResults) Iterate through the whole resource and identify any contained resources. Optionally this method can also assign IDs and modify references where the resource link has been specified but not the reference text.- Since:
- 5.4.0
-
addElement
Adds and returns a new element at the given path within the given structure. The paths used here are not FHIRPath expressions but instead just simple dot-separated path expressions.Only the last entry in the path is always created, existing repetitions of elements before the final dot are returned if they exists (although they are created if they do not). For example, given the path
Patient.name.given
, a new repetition ofgiven
is always added to the first (index 0) repetition of the name. If an index-0 repetition ofname
already exists, it is added to. If one does not exist, it if created and then added to.If the last element in the path refers to a non-repeatable element that is already present and is not empty, a
DataFormatException
error will be thrown.- Parameters:
theTarget
- The element to add to. This will often be aresource
instance, but does not need to be.thePath
- The path.- Returns:
- The newly added element
- Throws:
DataFormatException
- If the path is invalid or does not end with either a repeatable element, or an element that is non-repeatable but not already populated.
-
addElement
@Nonnull public <T extends IBase> T addElement(@Nonnull IBase theTarget, @Nonnull String thePath, @Nullable String theValue) Adds and returns a new element at the given path within the given structure. The paths used here are not FHIRPath expressions but instead just simple dot-separated path expressions.This method follows all of the same semantics as
addElement(IBase, String)
but it requires the path to point to an element with a primitive datatype and set the value of the datatype to the given value.- Parameters:
theTarget
- The element to add to. This will often be aresource
instance, but does not need to be.thePath
- The path.theValue
- The value to set, ornull
.- Returns:
- The newly added element
- Throws:
DataFormatException
- If the path is invalid or does not end with either a repeatable element, or an element that is non-repeatable but not already populated.
-
setElement
@Nonnull public <T extends IBase> T setElement(@Nonnull IBase theTarget, @Nonnull String thePath, @Nullable String theValue) Adds and returns a new element at the given path within the given structure. The paths used here are not FHIRPath expressions but instead just simple dot-separated path expressions.This method follows all of the same semantics as
addElement(IBase, String)
but it requires the path to point to an element with a primitive datatype and set the value of the datatype to the given value.- Parameters:
theTarget
- The element to add to. This will often be aresource
instance, but does not need to be.thePath
- The path.theValue
- The value to set, ornull
.- Returns:
- The newly added element
- Throws:
DataFormatException
- If the path is invalid or does not end with either a repeatable element, or an element that is non-repeatable but not already populated.
-
addElements
This method has the same semantics asaddElement(IBase, String, String)
but adds a collection of primitives instead of a single one.- Parameters:
theTarget
- The element to add to. This will often be aresource
instance, but does not need to be.thePath
- The path.theValues
- The values to set, ornull
.
-
clone
Clones a resource object, copying all data elements from theSource into a new copy of the same type.Note that:
- Only FHIR data elements are copied (i.e. user data maps are not copied)
- If a class extending a HAPI FHIR type (e.g. an instance of a class extending the Patient class) is supplied, an instance of the base type will be returned.
- Parameters:
theSource
- The source resource- Returns:
- A copy of the source resource
- Since:
- 5.6.0
-