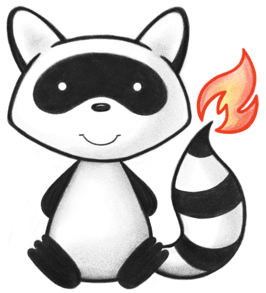
Package ca.uhn.fhir.util
Class StopWatch
java.lang.Object
ca.uhn.fhir.util.StopWatch
A multipurpose stopwatch which can be used to time tasks and produce
human readable output about task duration, throughput, estimated task completion,
etc.
Thread Safety Note: StopWatch is not intended to be thread safe.
- Since:
- HAPI FHIR 3.3.0
-
Constructor Summary
Constructors -
Method Summary
Modifier and TypeMethodDescriptionvoid
Finish the counter on the current task (which was started by callingstartTask(String)
.static String
formatEstimatedTimeRemaining
(double theCompleteToDate, double theTotal, double millis) Given an amount of something completed so far, and a total amount, calculates how long it will take for something to completestatic String
formatMillis
(double theMillis) Formats a number of milliseconds for display (e.g.static String
formatMillis
(long theMillis) Formats a number of milliseconds for display (e.g.formatMillisPerOperation
(long theNumOperations) Returns a nice human-readable display of the time taken per operation.Returns a string providing the durations of all tasks collected bystartTask(String)
static String
formatThroughput
(double throughput) Format a throughput number (output does not include units)formatThroughput
(long theNumOperations, TimeUnit theUnit) Determine the current throughput per unit of time (specified in theUnit) assuming that theNumOperations operations have happened.getEstimatedTimeRemaining
(double theCompleteToDate, double theTotal) Given an amount of something completed so far, and a total amount, calculates how long it will take for something to completelong
long
long
long
getMillisPerOperation
(long theNumOperations) static double
getThroughput
(long theNumOperations, long theMillisElapsed, TimeUnit theUnit) Calculate throughputdouble
getThroughput
(long theNumOperations, TimeUnit theUnit) Determine the current throughput per unit of time (specified in theUnit) assuming that theNumOperations operations have happened.void
restart()
static void
setNowForUnitTest
(Long theNowForUnitTest) void
Starts a counter for a sub-tasktoString()
Formats value in an appropriate format.
-
Constructor Details
-
StopWatch
public StopWatch()Constructor -
StopWatch
Constructor- Parameters:
theStart
- The time to record as the start for this timer
-
StopWatch
Constructor- Parameters:
theStart
- The time that the stopwatch was started
-
-
Method Details
-
endCurrentTask
Finish the counter on the current task (which was started by callingstartTask(String)
. This method has no effect if no task is currently started so it's ok to call it more than once. -
formatMillisPerOperation
Returns a nice human-readable display of the time taken per operation. Note that this may not actually output the number of milliseconds if the time taken per operation was very long (over 10 seconds)- See Also:
-
formatTaskDurations
Returns a string providing the durations of all tasks collected bystartTask(String)
-
formatThroughput
Determine the current throughput per unit of time (specified in theUnit) assuming that theNumOperations operations have happened.For example, if this stopwatch has 2 seconds elapsed, and this method is called for theNumOperations=30 and TimeUnit=SECONDS, this method will return 15
- See Also:
-
getEstimatedTimeRemaining
Given an amount of something completed so far, and a total amount, calculates how long it will take for something to complete- Parameters:
theCompleteToDate
- The amount so fartheTotal
- The total (must be higher than theCompleteToDate- Returns:
- A formatted amount of time
-
formatEstimatedTimeRemaining
public static String formatEstimatedTimeRemaining(double theCompleteToDate, double theTotal, double millis) Given an amount of something completed so far, and a total amount, calculates how long it will take for something to complete- Parameters:
theCompleteToDate
- The amount so fartheTotal
- The total (must be higher than theCompleteToDate- Returns:
- A formatted amount of time
-
getMillis
-
getMillis
-
getMillisAndRestart
-
getMillisPerOperation
- Parameters:
theNumOperations
- Ok for this to be 0, it will be treated as 1
-
getStartedDate
-
getThroughput
Determine the current throughput per unit of time (specified in theUnit) assuming that theNumOperations operations have happened.For example, if this stopwatch has 2 seconds elapsed, and this method is called for theNumOperations=30 and TimeUnit=SECONDS, this method will return 15
- See Also:
-
restart
-
startTask
Starts a counter for a sub-taskThread Safety Note: This method is not threadsafe! Do not use subtasks in a multithreaded environment.
- Parameters:
theTaskName
- Note that if theTaskName is blank or empty, no task is started
-
toString
Formats value in an appropriate format. SeeformatMillis(long)
} for a description of the format -
formatThroughput
Format a throughput number (output does not include units) -
getThroughput
Calculate throughput- Parameters:
theNumOperations
- The number of operations completedtheMillisElapsed
- The time elapsedtheUnit
- The unit for the throughput
-
formatMillis
Formats a number of milliseconds for display (e.g. in a log file), tailoring the output to how big the value actually is.Example outputs:
- 133ms
- 00:00:10.223
- 1.7 days
- 64 days
-
formatMillis
Formats a number of milliseconds for display (e.g. in a log file), tailoring the output to how big the value actually is.Example outputs:
- 133ms
- 00:00:10.223
- 1.7 days
- 64 days
-
setNowForUnitTest
-