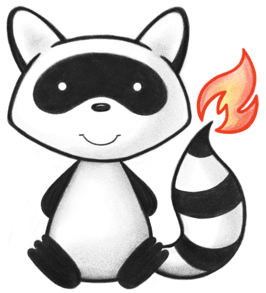
Package ca.uhn.fhir.util
Class UrlUtil
java.lang.Object
ca.uhn.fhir.util.UrlUtil
-
Nested Class Summary
Nested Classes -
Method Summary
Modifier and TypeMethodDescriptionstatic String
constructAbsoluteUrl
(String theBase, String theEndpoint) Resolve a relative URL - THIS METHOD WILL NOT FAIL but will log a warning and return theEndpoint if the input is invalid.static String
constructRelativeUrl
(String theParentExtensionUrl, String theExtensionUrl) static String
determineResourceTypeInResourceUrl
(FhirContext theFhirContext, String theUrl) Given a FHIR resource URL, extracts the associated resource type.static String
escapeUrlParam
(String theUnescaped) URL encode a value according to RFC 3986escapeUrlParams
(Collection<String> theUnescaped) Applies the same encodong asescapeUrlParam(String)
but against all values in a collectiongetAboveUriCandidates
(String theUri) Creates list of sub URIs candidates for search with :above modifier Example input: http://[host]/[pathPart1]/[pathPart2] Example output: http://[host], http://[host]/[pathPart1], http://[host]/[pathPart1]/[pathPart2]static boolean
isAbsolute
(String theValue) static boolean
isNeedsSanitization
(CharSequence theString) static boolean
static String
Normalizes canonical URLs for comparison.parseQueryString
(String theQueryString) parseQueryStrings
(String... theQueryString) static UrlUtil.UrlParts
Parse a URL in one of the following forms: [Resource Type]?[Search Params] [Resource Type]/[Resource ID] [Resource Type]/[Resource ID]/_history/[Version ID]static RuntimeResourceDefinition
parseUrlResourceType
(FhirContext theCtx, String theUrl) static String
sanitizeBaseUrl
(String theBaseUrl) static String
sanitizeHeaderValue
(String theHeader) Cleans up a value that will be serialized as an HTTP header.static String
sanitizeUrlPart
(CharSequence theString) This method specifically HTML-encodes the " and < characters in order to prevent injection attacks.static String[]
sanitizeUrlPart
(String[] theParameterValues) Applies the same logic assanitizeUrlPart(CharSequence)
but against an array, returning an array with the same strings as the input but with sanitization appliedstatic String
sanitizeUrlPart
(IPrimitiveType<?> theString) This method specifically HTML-encodes the " and < characters in order to prevent injection attacksstatic List
<org.apache.http.NameValuePair> translateMatchUrl
(String theMatchUrl) static String
-
Method Details
-
sanitizeHeaderValue
Cleans up a value that will be serialized as an HTTP header. This method:- Strips any newline (\r or \n) characters
- Since:
- 6.2.0
-
sanitizeBaseUrl
-
constructAbsoluteUrl
Resolve a relative URL - THIS METHOD WILL NOT FAIL but will log a warning and return theEndpoint if the input is invalid. -
constructRelativeUrl
-
determineResourceTypeInResourceUrl
@Nullable public static String determineResourceTypeInResourceUrl(FhirContext theFhirContext, String theUrl) Given a FHIR resource URL, extracts the associated resource type. Supported formats include the following inputs, all of which will return Patient. If no resource type can be determined, null will be returned.- Patient
- Patient?
- Patient?identifier=foo
- /Patient
- /Patient?
- /Patient?identifier=foo
- http://foo/base/Patient?identifier=foo
- http://foo/base/Patient/1
- http://foo/base/Patient/1/_history/2
- Patient/1
- Patient/1/_history/2
- /Patient/1
- /Patient/1/_history/2
-
escapeUrlParam
URL encode a value according to RFC 3986This method is intended to be applied to an individual parameter name or value. For example, if you are creating the URL
http://example.com/fhir/Patient?key=føø
it would be appropriate to pass the string "føø" to this method, but not appropriate to pass the entire URL since characters such as "/" and "?" would also be escaped. -
escapeUrlParams
Applies the same encodong asescapeUrlParam(String)
but against all values in a collection -
isAbsolute
-
isNeedsSanitization
-
isValid
-
parseUrlResourceType
public static RuntimeResourceDefinition parseUrlResourceType(FhirContext theCtx, String theUrl) throws DataFormatException - Throws:
DataFormatException
-
parseQueryString
-
parseQueryStrings
-
normalizeCanonicalUrlForComparison
Normalizes canonical URLs for comparison. Trailing "/" is stripped, and any version identifiers or fragment hash is removed -
parseUrl
Parse a URL in one of the following forms:- [Resource Type]?[Search Params]
- [Resource Type]/[Resource ID]
- [Resource Type]/[Resource ID]/_history/[Version ID]
-
sanitizeUrlPart
This method specifically HTML-encodes the " and < characters in order to prevent injection attacks -
sanitizeUrlPart
This method specifically HTML-encodes the " and < characters in order to prevent injection attacks.The following characters are escaped:
- '
- "
- <
- >
- \n (newline)
-
sanitizeUrlPart
Applies the same logic assanitizeUrlPart(CharSequence)
but against an array, returning an array with the same strings as the input but with sanitization applied -
unescape
-
translateMatchUrl
-
getAboveUriCandidates
Creates list of sub URIs candidates for search with :above modifier Example input: http://[host]/[pathPart1]/[pathPart2] Example output: http://[host], http://[host]/[pathPart1], http://[host]/[pathPart1]/[pathPart2]- Parameters:
theUri
- String URI parameter- Returns:
- List of URI candidates
-