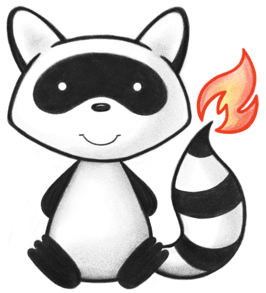
Package ca.uhn.fhir.validation
Class FhirValidator
java.lang.Object
ca.uhn.fhir.validation.FhirValidator
Resource validator, which checks resources for compliance against various validation schemes (schemas, schematrons, profiles, etc.)
To obtain a resource validator, call FhirContext.newValidator()
Thread safety note: This class is thread safe, so you may register or unregister validator modules at any time. Individual modules are not guaranteed to be thread safe however. Reconfigure them with caution.
-
Constructor Summary
ConstructorsConstructorDescriptionFhirValidator
(FhirContext theFhirContext) Constructor (this should not be called directly, but ratherFhirContext.newValidator()
should be called to obtain an instance ofFhirValidator
) -
Method Summary
Modifier and TypeMethodDescriptionboolean
If this is true, bundles will be validated in parallel threads.boolean
If this is true, any resource that has contained resources will first be deep-copied and then the contained resources remove from the copy and this copy without contained resources will be validated.boolean
Should the validator validate the resource against the base schema (the schema provided with the FHIR distribution itself)boolean
Should the validator validate the resource against the base schema (the schema provided with the FHIR distribution itself)registerValidatorModule
(IValidatorModule theValidator) Add a new validator module to this validator.setConcurrentBundleValidation
(boolean theConcurrentBundleValidation) If this is true, bundles will be validated in parallel threads.setExecutorService
(ExecutorService theExecutorService) void
setInterceptorBroadcaster
(IInterceptorBroadcaster theInterceptorBraodcaster) Optionally supplies an interceptor broadcaster that will be used to invoke validation related Pointcut eventssetSkipContainedReferenceValidation
(boolean theSkipContainedReferenceValidation) If this is true, any resource that has contained resources will first be deep-copied and then the contained resources remove from the copy and this copy without contained resources will be validated.setValidateAgainstStandardSchema
(boolean theValidateAgainstStandardSchema) Should the validator validate the resource against the base schema (the schema provided with the FHIR distribution itself)setValidateAgainstStandardSchematron
(boolean theValidateAgainstStandardSchematron) Should the validator validate the resource against the base schematron (the schematron provided with the FHIR distribution itself)void
unregisterValidatorModule
(IValidatorModule theValidator) Removes a validator module from this validator.validateWithResult
(String theResource) Validates a resource instance returning aValidationResult
which contains the results.validateWithResult
(String theResource, ValidationOptions theOptions) Validates a resource instance returning aValidationResult
which contains the results.validateWithResult
(IBaseResource theResource) Validates a resource instance returning aValidationResult
which contains the results.validateWithResult
(IBaseResource theResource, ValidationOptions theOptions) Validates a resource instance returning aValidationResult
which contains the results.
-
Constructor Details
-
FhirValidator
Constructor (this should not be called directly, but ratherFhirContext.newValidator()
should be called to obtain an instance ofFhirValidator
)
-
-
Method Details
-
isValidateAgainstStandardSchema
Should the validator validate the resource against the base schema (the schema provided with the FHIR distribution itself) -
setValidateAgainstStandardSchema
Should the validator validate the resource against the base schema (the schema provided with the FHIR distribution itself)- Returns:
- Returns a referens to
this
for method chaining
-
isValidateAgainstStandardSchematron
Should the validator validate the resource against the base schema (the schema provided with the FHIR distribution itself) -
setValidateAgainstStandardSchematron
public FhirValidator setValidateAgainstStandardSchematron(boolean theValidateAgainstStandardSchematron) Should the validator validate the resource against the base schematron (the schematron provided with the FHIR distribution itself)- Returns:
- Returns a referens to
this
for method chaining
-
registerValidatorModule
Add a new validator module to this validator. You may register as many modules as you like at any time.- Parameters:
theValidator
- The validator module. Must not be null.- Returns:
- Returns a reference to
this
for easy method chaining.
-
unregisterValidatorModule
Removes a validator module from this validator. You may register as many modules as you like, and remove them at any time.- Parameters:
theValidator
- The validator module. Must not be null.
-
validateWithResult
Validates a resource instance returning aValidationResult
which contains the results.- Parameters:
theResource
- the resource to validate- Returns:
- the results of validation
- Since:
- 0.7
-
validateWithResult
Validates a resource instance returning aValidationResult
which contains the results.- Parameters:
theResource
- the resource to validate- Returns:
- the results of validation
- Since:
- 1.1
-
validateWithResult
Validates a resource instance returning aValidationResult
which contains the results.- Parameters:
theResource
- the resource to validatetheOptions
- Optionally provides options to the validator- Returns:
- the results of validation
- Since:
- 4.0.0
-
validateWithResult
Validates a resource instance returning aValidationResult
which contains the results.- Parameters:
theResource
- the resource to validatetheOptions
- Optionally provides options to the validator- Returns:
- the results of validation
- Since:
- 4.0.0
-
setInterceptorBroadcaster
Optionally supplies an interceptor broadcaster that will be used to invoke validation related Pointcut events- Since:
- 5.5.0
-
setExecutorService
-
isConcurrentBundleValidation
If this is true, bundles will be validated in parallel threads. The bundle structure itself will not be validated, only the resources in its entries. -
setConcurrentBundleValidation
If this is true, bundles will be validated in parallel threads. The bundle structure itself will not be validated, only the resources in its entries. -
isSkipContainedReferenceValidation
If this is true, any resource that has contained resources will first be deep-copied and then the contained resources remove from the copy and this copy without contained resources will be validated. -
setSkipContainedReferenceValidation
public FhirValidator setSkipContainedReferenceValidation(boolean theSkipContainedReferenceValidation) If this is true, any resource that has contained resources will first be deep-copied and then the contained resources remove from the copy and this copy without contained resources will be validated.
-