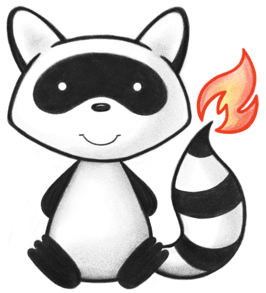
Package ca.uhn.fhir.jpa.dao.data
Interface IBatch2JobInstanceRepository
- All Superinterfaces:
org.springframework.data.repository.CrudRepository<Batch2JobInstanceEntity,
,String> IHapiFhirJpaRepository
,org.springframework.data.jpa.repository.JpaRepository<Batch2JobInstanceEntity,
,String> org.springframework.data.repository.ListCrudRepository<Batch2JobInstanceEntity,
,String> org.springframework.data.repository.ListPagingAndSortingRepository<Batch2JobInstanceEntity,
,String> org.springframework.data.repository.PagingAndSortingRepository<Batch2JobInstanceEntity,
,String> org.springframework.data.repository.query.QueryByExampleExecutor<Batch2JobInstanceEntity>
,org.springframework.data.repository.Repository<Batch2JobInstanceEntity,
String>
public interface IBatch2JobInstanceRepository
extends org.springframework.data.jpa.repository.JpaRepository<Batch2JobInstanceEntity,String>, IHapiFhirJpaRepository
-
Method Summary
Modifier and TypeMethodDescriptionfetchBatchInstanceStatus
(String theInstanceId) fetchInstancesByJobDefinitionIdAndStatus
(String theJobDefinitionId, Set<StatusEnum> theIncompleteStatuses, org.springframework.data.domain.Pageable thePageRequest) org.springframework.data.domain.Page
<Batch2JobInstanceEntity> findByJobDefinitionIdOrStatusOrIdOrCreateTime
(String theDefinitionId, StatusEnum theStatus, String theJobId, Date theFrom, Date theTo, org.springframework.data.domain.Pageable thePageable) findInstancesByJobDefinitionId
(String theJobDefinitionId, org.springframework.data.domain.Pageable thePageRequest) findInstancesByJobIdAndParams
(String theDefinitionId, String theParams, org.springframework.data.domain.Pageable thePageable) findInstancesByJobIdAndStatusAndExpiry
(String theDefinitionId, Set<StatusEnum> theStatus, Date theCutoff, org.springframework.data.domain.Pageable thePageable) findInstancesByJobIdParamsAndStatus
(String theDefinitionId, String theParams, Set<StatusEnum> theStatus, org.springframework.data.domain.Pageable thePageable) int
updateInstanceCancelled
(String theInstanceId, boolean theCancelled) int
updateInstanceStatusIfIn
(String theInstanceId, StatusEnum theNewState, Set<StatusEnum> thePriorStates) int
updateInstanceUpdateTime
(String theInstanceId, Date theUpdated) int
updateWorkChunksPurgedTrue
(String theInstanceId) Methods inherited from interface org.springframework.data.repository.CrudRepository
count, delete, deleteAll, deleteAll, deleteAllById, deleteById, existsById, findById, save
Methods inherited from interface org.springframework.data.jpa.repository.JpaRepository
deleteAllByIdInBatch, deleteAllInBatch, deleteAllInBatch, deleteInBatch, findAll, findAll, flush, getById, getOne, getReferenceById, saveAllAndFlush, saveAndFlush
Methods inherited from interface org.springframework.data.repository.ListCrudRepository
findAll, findAllById, saveAll
Methods inherited from interface org.springframework.data.repository.ListPagingAndSortingRepository
findAll
Methods inherited from interface org.springframework.data.repository.PagingAndSortingRepository
findAll
Methods inherited from interface org.springframework.data.repository.query.QueryByExampleExecutor
count, exists, findAll, findBy, findOne
-
Method Details
-
updateInstanceStatusIfIn
@Modifying @Query("UPDATE Batch2JobInstanceEntity e SET e.myStatus = :status WHERE e.myId = :id and e.myStatus IN ( :prior_states )") int updateInstanceStatusIfIn(@Param("id") String theInstanceId, @Param("status") StatusEnum theNewState, @Param("prior_states") Set<StatusEnum> thePriorStates) -
updateInstanceUpdateTime
@Modifying @Query("UPDATE Batch2JobInstanceEntity e SET e.myUpdateTime = :updated WHERE e.myId = :id") int updateInstanceUpdateTime(@Param("id") String theInstanceId, @Param("updated") Date theUpdated) -
updateInstanceCancelled
@Modifying @Query("UPDATE Batch2JobInstanceEntity e SET e.myCancelled = :cancelled WHERE e.myId = :id") int updateInstanceCancelled(@Param("id") String theInstanceId, @Param("cancelled") boolean theCancelled) -
updateWorkChunksPurgedTrue
@Modifying @Query("UPDATE Batch2JobInstanceEntity e SET e.myWorkChunksPurged = true WHERE e.myId = :id") int updateWorkChunksPurgedTrue(@Param("id") String theInstanceId) -
findInstancesByJobIdParamsAndStatus
@Query("SELECT b from Batch2JobInstanceEntity b WHERE b.myDefinitionId = :defId AND (b.myParamsJson = :params OR b.myParamsJsonVc = :params) AND b.myStatus IN( :stats )") List<Batch2JobInstanceEntity> findInstancesByJobIdParamsAndStatus(@Param("defId") String theDefinitionId, @Param("params") String theParams, @Param("stats") Set<StatusEnum> theStatus, org.springframework.data.domain.Pageable thePageable) -
findInstancesByJobIdAndParams
@Query("SELECT b from Batch2JobInstanceEntity b WHERE b.myDefinitionId = :defId AND (b.myParamsJson = :params OR b.myParamsJsonVc = :params)") List<Batch2JobInstanceEntity> findInstancesByJobIdAndParams(@Param("defId") String theDefinitionId, @Param("params") String theParams, org.springframework.data.domain.Pageable thePageable) -
findByJobDefinitionIdOrStatusOrIdOrCreateTime
@Query("SELECT b from Batch2JobInstanceEntity b WHERE (:definitionId IS NULL OR b.myDefinitionId = :definitionId) AND (:status IS NULL OR b.myStatus = :status) AND (:jobId IS NULL OR b.myId = :jobId) AND (cast(:from as date) IS NULL OR b.myCreateTime >= :from) AND (cast(:to as date) IS NULL OR b.myCreateTime <= :to)") org.springframework.data.domain.Page<Batch2JobInstanceEntity> findByJobDefinitionIdOrStatusOrIdOrCreateTime(@Param("definitionId") String theDefinitionId, @Param("status") StatusEnum theStatus, @Param("jobId") String theJobId, @Param("from") Date theFrom, @Param("to") Date theTo, org.springframework.data.domain.Pageable thePageable) -
findInstancesByJobIdAndStatusAndExpiry
@Query("SELECT b from Batch2JobInstanceEntity b WHERE b.myDefinitionId = :defId AND b.myStatus IN( :stats ) AND b.myEndTime < :cutoff") List<Batch2JobInstanceEntity> findInstancesByJobIdAndStatusAndExpiry(@Param("defId") String theDefinitionId, @Param("stats") Set<StatusEnum> theStatus, @Param("cutoff") Date theCutoff, org.springframework.data.domain.Pageable thePageable) -
fetchInstancesByJobDefinitionIdAndStatus
@Query("SELECT e FROM Batch2JobInstanceEntity e WHERE e.myDefinitionId = :jobDefinitionId AND e.myStatus IN :statuses") List<Batch2JobInstanceEntity> fetchInstancesByJobDefinitionIdAndStatus(@Param("jobDefinitionId") String theJobDefinitionId, @Param("statuses") Set<StatusEnum> theIncompleteStatuses, org.springframework.data.domain.Pageable thePageRequest) -
findInstancesByJobDefinitionId
@Query("SELECT e FROM Batch2JobInstanceEntity e WHERE e.myDefinitionId = :jobDefinitionId") List<Batch2JobInstanceEntity> findInstancesByJobDefinitionId(@Param("jobDefinitionId") String theJobDefinitionId, org.springframework.data.domain.Pageable thePageRequest) -
fetchBatchInstanceStatus
@Query("SELECT new ca.uhn.fhir.batch2.model.BatchInstanceStatusDTO(e.myId, e.myStatus, e.myStartTime, e.myEndTime) FROM Batch2JobInstanceEntity e WHERE e.myId = :id") BatchInstanceStatusDTO fetchBatchInstanceStatus(@Param("id") String theInstanceId)
-