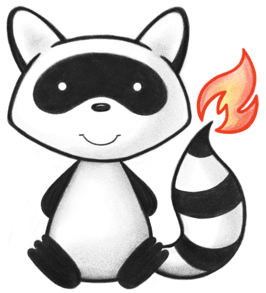
Package ca.uhn.fhir.jpa.searchparam
Class SearchParameterMap
java.lang.Object
ca.uhn.fhir.jpa.searchparam.SearchParameterMap
- All Implemented Interfaces:
Serializable
- See Also:
-
Nested Class Summary
Nested ClassesModifier and TypeClassDescriptionstatic enum
static class
static class
static class
-
Field Summary
Fields -
Constructor Summary
ConstructorsConstructorDescriptionConstructorSearchParameterMap
(String theName, ca.uhn.fhir.model.api.IQueryParameterType theParam) Constructor -
Method Summary
Modifier and TypeMethodDescriptionaddInclude
(ca.uhn.fhir.model.api.Include theInclude) addRevInclude
(ca.uhn.fhir.model.api.Include theInclude) void
clean()
clone()
Creates and returns a copy of this mapboolean
containsKey
(String theName) entrySet()
getCount()
Set
<ca.uhn.fhir.model.api.Include> If set, tells the server the maximum number of observations to return for each observation code in the result set of a lastn operationca.uhn.fhir.rest.param.DateRangeParam
Returns null if there is no last updated valueIf set, tells the server to load these results synchronously, and not to load more than X resultsca.uhn.fhir.rest.param.QuantityParam
Set
<ca.uhn.fhir.model.api.Include> ca.uhn.fhir.rest.api.SearchContainedModeEnum
ca.uhn.fhir.rest.api.SearchTotalModeEnum
ca.uhn.fhir.rest.api.SortSpec
getSort()
ca.uhn.fhir.rest.api.SummaryEnum
boolean
boolean
boolean
This will only return true if all parameters have no modifier of any kindboolean
boolean
isEmpty()
boolean
isLastN()
If set, tells the server to use an Elasticsearch query to generate a list of Resource IDs for the LastN operationboolean
If set, tells the server to load these results synchronously, and not to load more than X resultsboolean
Returns true ifgetOffset()
andgetCount()
both return a non null responseboolean
keySet()
static SearchParameterMap
static SearchParameterMap
newSynchronous
(String theName, ca.uhn.fhir.model.api.IQueryParameterType theParam) void
removeByNameAndModifier
(String theName, ca.uhn.fhir.rest.param.TokenParamModifier theModifier) removeByNameAndModifier
(String theName, String theModifier) Given a search parameter name and modifier (e.g.removeByNameUnmodified
(String theName) Variant of removeByNameAndModifier for unmodified params.removeByQualifier
(ca.uhn.fhir.rest.param.TokenParamModifier theModifier) removeByQualifier
(String theQualifier) For each search parameter in the map, extract any which have the given qualifier.setDeleteExpunge
(boolean theDeleteExpunge) void
setEverythingMode
(SearchParameterMap.EverythingModeEnum theConsolidateMatches) void
setIncludes
(Set<ca.uhn.fhir.model.api.Include> theIncludes) setLastN
(boolean theLastN) If set, tells the server to use an Elasticsearch query to generate a list of Resource IDs for the LastN operationsetLastNMax
(Integer theLastNMax) If set, tells the server the maximum number of observations to return for each observation code in the result set of a lastn operationvoid
setLastUpdated
(ca.uhn.fhir.rest.param.DateRangeParam theLastUpdated) setLoadSynchronous
(boolean theLoadSynchronous) If set, tells the server to load these results synchronously, and not to load more than X resultssetLoadSynchronousUpTo
(Integer theLoadSynchronousUpTo) If set, tells the server to load these results synchronously, and not to load more than X results.void
setNearDistanceParam
(ca.uhn.fhir.rest.param.QuantityParam theQuantityParam) void
void
setRevIncludes
(Set<ca.uhn.fhir.model.api.Include> theRevIncludes) void
setSearchContainedMode
(ca.uhn.fhir.rest.api.SearchContainedModeEnum theSearchContainedMode) void
setSearchTotalMode
(ca.uhn.fhir.rest.api.SearchTotalModeEnum theSearchTotalMode) setSort
(ca.uhn.fhir.rest.api.SortSpec theSort) void
setSummaryMode
(ca.uhn.fhir.rest.api.SummaryEnum theSummaryMode) int
size()
toNormalizedQueryString
(ca.uhn.fhir.context.FhirContext theCtx) This method creates a URL query string representation of the parameters in this object, excluding the part before the parameters, e.g.toString()
Collection
<List<List<ca.uhn.fhir.model.api.IQueryParameterType>>> values()
-
Field Details
-
INTEGER_0
-
-
Constructor Details
-
SearchParameterMap
public SearchParameterMap()Constructor -
SearchParameterMap
Constructor
-
-
Method Details
-
clone
Creates and returns a copy of this map -
getSummaryMode
-
setSummaryMode
-
getSearchTotalMode
-
setSearchTotalMode
-
add
-
add
-
add
-
values
-
add
-
addInclude
-
addRevInclude
-
getCount
-
setCount
-
getOffset
-
setOffset
-
getEverythingMode
-
setEverythingMode
-
getIncludes
-
setIncludes
-
getLastUpdated
Returns null if there is no last updated value -
setLastUpdated
-
getLoadSynchronousUpTo
If set, tells the server to load these results synchronously, and not to load more than X results -
setLoadSynchronousUpTo
If set, tells the server to load these results synchronously, and not to load more than X results. Note that setting this to a value will also setsetLoadSynchronous(boolean)
to true -
getRevIncludes
-
setRevIncludes
-
getSort
-
setSort
-
isAllParametersHaveNoModifier
This will only return true if all parameters have no modifier of any kind -
isLoadSynchronous
If set, tells the server to load these results synchronously, and not to load more than X results -
setLoadSynchronous
If set, tells the server to load these results synchronously, and not to load more than X results -
isLastN
If set, tells the server to use an Elasticsearch query to generate a list of Resource IDs for the LastN operation -
setLastN
If set, tells the server to use an Elasticsearch query to generate a list of Resource IDs for the LastN operation -
getLastNMax
If set, tells the server the maximum number of observations to return for each observation code in the result set of a lastn operation -
setLastNMax
If set, tells the server the maximum number of observations to return for each observation code in the result set of a lastn operation -
toNormalizedQueryString
This method creates a URL query string representation of the parameters in this object, excluding the part before the parameters, e.g.?name=smith&_sort=Patient:family
This method excludes the
_count
parameter, as it doesn't affect the substance of the results returned -
hasIncludes
- Since:
- 5.5.0
-
hasRevIncludes
- Since:
- 6.2.0
-
toString
-
clean
-
getNearDistanceParam
-
setNearDistanceParam
-
isWantOnlyCount
-
isDeleteExpunge
-
setDeleteExpunge
-
get
-
put
-
containsKey
-
keySet
-
isEmpty
-
entrySet
-
remove
-
removeByNameUnmodified
Variant of removeByNameAndModifier for unmodified params.- Parameters:
theName
- the query parameter key- Returns:
- an And/Or List of Query Parameters matching the name with no modifier.
-
removeByNameAndModifier
public List<List<ca.uhn.fhir.model.api.IQueryParameterType>> removeByNameAndModifier(String theName, String theModifier) Given a search parameter name and modifier (e.g. :text), get and remove all Search Parameters matching this name and modifier- Parameters:
theName
- the query parameter keytheModifier
- the qualifier you want to remove - nullable for unmodified params.- Returns:
- an And/Or List of Query Parameters matching the qualifier.
-
removeByNameAndModifier
public List<List<ca.uhn.fhir.model.api.IQueryParameterType>> removeByNameAndModifier(String theName, @Nonnull ca.uhn.fhir.rest.param.TokenParamModifier theModifier) -
removeByQualifier
public Map<String,List<List<ca.uhn.fhir.model.api.IQueryParameterType>>> removeByQualifier(String theQualifier) For each search parameter in the map, extract any which have the given qualifier. e.g. Take the url: Observation?code:text=abcinvalid input: '&code'=123invalid input: '&code':text=definvalid input: '&reason':text=somereasonIf we call this function with `:text`, it will return a map that looks like:
code -> [[code:text=abc], [code:text=def]] reason -> [[reason:text=somereason]]
and the remaining search parameters in the map will be:
code -> [[code=123]]
- Parameters:
theQualifier
-- Returns:
-
removeByQualifier
public Map<String,List<List<ca.uhn.fhir.model.api.IQueryParameterType>>> removeByQualifier(@Nonnull ca.uhn.fhir.rest.param.TokenParamModifier theModifier) -
size
-
getSearchContainedMode
-
setSearchContainedMode
public void setSearchContainedMode(ca.uhn.fhir.rest.api.SearchContainedModeEnum theSearchContainedMode) -
isOffsetQuery
Returns true ifgetOffset()
andgetCount()
both return a non null response- Since:
- 5.5.0
-
newSynchronous
-
newSynchronous
public static SearchParameterMap newSynchronous(String theName, ca.uhn.fhir.model.api.IQueryParameterType theParam)
-