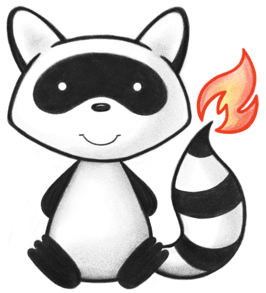
Interface IBundleProvider
- All Known Implementing Classes:
BundleProviderWithNamedPages
,SimpleBundleProvider
-
Method Summary
Modifier and TypeMethodDescriptiondefault List
<org.hl7.fhir.instance.model.api.IBaseResource> Get all resourcesdefault String
If this method is implemented, provides an ID for the current page of results.default Integer
If the results in this bundle were produced using an offset query (as opposed to a query using continuation pointers, page IDs, etc.) the page offset can be returned here.default Integer
IfgetCurrentPageOffset()
returns a non-null value, this method must also return the actual page size useddefault String
If this method is implemented, provides an ID for the next page of results.default String
If this method is implemented, provides an ID for the previous page of results.org.hl7.fhir.instance.model.api.IPrimitiveType
<Date> Returns the instant as of which this result was created.default List
<org.hl7.fhir.instance.model.api.IBaseResource> getResources
(int theFromIndex, int theToIndex) Load the given collection of resources by index, plus any additional resources per the server's processing rules (e.g.default List
<org.hl7.fhir.instance.model.api.IBaseResource> getResources
(int theFromIndex, int theToIndex, ResponsePage.ResponsePageBuilder theResponsePageBuilder) Load the given collection of resources by index, plus any additional resources per the server's processing rules (e.g.getUuid()
Returns the UUID associated with this search.default boolean
isEmpty()
This method returnsfalse
if the bundle provider knows that at least one result exists.Optionally may be used to signal a preferred page size to the server, e.g.size()
Returns the total number of results which match the given query (exclusive of any _include's or OperationOutcome).default int
-
Method Details
-
getCurrentPageId
If this method is implemented, provides an ID for the current page of results. This ID should be unique (at least within the current search as identified bygetUuid()
) so that it can be used to look up a specific page of results.This can be used in order to allow the server paging mechanism to work using completely opaque links (links that do not encode any index/offset information), which can be useful on some servers.
- Since:
- 3.5.0
-
getNextPageId
If this method is implemented, provides an ID for the next page of results. This ID should be unique (at least within the current search as identified bygetUuid()
) so that it can be used to look up a specific page of results.This can be used in order to allow the server paging mechanism to work using completely opaque links (links that do not encode any index/offset information), which can be useful on some servers.
- Since:
- 3.5.0
-
getPreviousPageId
If this method is implemented, provides an ID for the previous page of results. This ID should be unique (at least within the current search as identified bygetUuid()
) so that it can be used to look up a specific page of results.This can be used in order to allow the server paging mechanism to work using completely opaque links (links that do not encode any index/offset information), which can be useful on some servers.
- Since:
- 3.5.0
-
getCurrentPageOffset
If the results in this bundle were produced using an offset query (as opposed to a query using continuation pointers, page IDs, etc.) the page offset can be returned here. The server should then attempt to form paging links that use_offset
instead of opaque page IDs. -
getCurrentPageSize
IfgetCurrentPageOffset()
returns a non-null value, this method must also return the actual page size used -
getPublished
org.hl7.fhir.instance.model.api.IPrimitiveType<Date> getPublished()Returns the instant as of which this result was created. The result of this value is used to populate thelastUpdated
value on search result/history result bundles. -
getResources
@Nonnull default List<org.hl7.fhir.instance.model.api.IBaseResource> getResources(int theFromIndex, int theToIndex) Load the given collection of resources by index, plus any additional resources per the server's processing rules (e.g. _include'd resources, OperationOutcome, etc.). For example, if the method is invoked with index 0,10 the method might return 10 search results, plus an additional 20 resources which matched a client's _include specification.Note that if this bundle provider was loaded using a page ID (i.e. via
Note that this implementation should not be used if accurate paging is required, as page calculation depends on _include'd resource counts. For accurate paging, useIPagingProvider.retrieveResultList(RequestDetails, String, String)
becausegetNextPageId()
provided a value on the previous page, then the indexes should be ignored and the whole page returned.getResources(int, int, ResponsePage.ResponsePageBuilder)
- Parameters:
theFromIndex
- The low index (inclusive) to returntheToIndex
- The high index (exclusive) to return- Returns:
- A list of resources. The size of this list must be at least
theToIndex - theFromIndex
.
-
getResources
default List<org.hl7.fhir.instance.model.api.IBaseResource> getResources(int theFromIndex, int theToIndex, @Nonnull ResponsePage.ResponsePageBuilder theResponsePageBuilder) Load the given collection of resources by index, plus any additional resources per the server's processing rules (e.g. _include'd resources, OperationOutcome, etc.). For example, if the method is invoked with index 0,10 the method might return 10 search results, plus an additional 20 resources which matched a client's _include specification.Note that if this bundle provider was loaded using a page ID (i.e. via
IPagingProvider.retrieveResultList(RequestDetails, String, String)
becausegetNextPageId()
provided a value on the previous page, then the indexes should be ignored and the whole page returned.- Parameters:
theFromIndex
- The low index (inclusive) to returntheToIndex
- The high index (exclusive) to returntheResponsePageBuilder
- The ResponsePageBuilder. The builder will add values needed for the response page.- Returns:
- A list of resources. The size of this list must be at least
theToIndex - theFromIndex
.
-
getAllResources
Get all resources- Returns:
getResources(0, this.size())
. Return an empty list ifthis.size()
is zero.- Throws:
ca.uhn.fhir.context.ConfigurationException
- if size() is null
-
getUuid
Returns the UUID associated with this search. Note that this does not need to return a non-null value unless it a IPagingProvider is being used that requires UUIDs being returned.In other words, if you are using the default FifoMemoryPagingProvider in your server, it is fine for this method to simply return
null
since FifoMemoryPagingProvider does not use the value anyhow. On the other hand, if you are creating a custom IPagingProvider implementation you might use this method to communicate the search ID back to the provider.Note that the UUID returned by this method corresponds to the search, and not to the individual page.
-
preferredPageSize
Optionally may be used to signal a preferred page size to the server, e.g. because the implementing code recognizes that the resources which will be returned by this implementation are expensive to load so a smaller page size should be used. The value returned by this method will only be used if the client has not explicitly requested a page size.- Returns:
- Returns the preferred page size or
null
-
size
Returns the total number of results which match the given query (exclusive of any _include's or OperationOutcome). May return null if the total size is not known or would be too expensive to calculate. -
isEmpty
This method returnsfalse
if the bundle provider knows that at least one result exists. -
sizeOrThrowNpe
- Returns:
- the value of
size()
and throws aNullPointerException
of it is null
-
getAllResourceIds
- Returns:
- the list of ids of all resources in the bundle
-