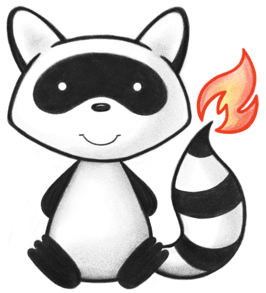
Interface IRestfulResponse
- All Known Implementing Classes:
BaseRestfulResponse
,ServletRestfulResponse
,SystemRestfulResponse
HttpServletResponse
but intended to be agnostic
of the server framework being used.
This class is a bit of an awkward abstraction given the two styles of servers it supports. Servlets work by writing to a servlet response that is provided as a parameter by the container. JAX-RS works by returning an object via a method return back to the containing framework. However using it correctly should make for compatible code across both approaches.
-
Method Summary
Modifier and TypeMethodDescriptionvoid
Adds a response header.commitResponse
(Closeable theWriterOrOutputStream) Finalizes the response streaming using the writer that was returned by calling eithergetResponseWriter(int, String, String, boolean)
orgetResponseOutputStream(int, String, Integer)
.Returns the headers added to this responsegetResponseOutputStream
(int theStatusCode, String theContentType, Integer theContentLength) Initiate a new binary response.getResponseWriter
(int theStatusCode, String theContentType, String theCharset, boolean theRespondGzip) Initiate a new textual response.
-
Method Details
-
getResponseWriter
@Nonnull Writer getResponseWriter(int theStatusCode, String theContentType, String theCharset, boolean theRespondGzip) throws IOException Initiate a new textual response. The Writer returned by this method must be finalized by callingcommitResponse(Closeable)
later.Note that the caller should not close the returned object, but should instead just return it to
commitResponse(Closeable)
upon successful completion. This is different from normal Java practice where you would request it in atry with resource
block, since in Servlets you are not actually required to close the writer/stream, and doing so automatically may prevent you from correctly handling exceptions.- Parameters:
theStatusCode
- The HTTP status code.theContentType
- The HTTP response content type.theCharset
- The HTTP response charset.theRespondGzip
- Should the response be GZip encoded?- Returns:
- Returns a
Writer
that can accept the response body. - Throws:
IOException
-
getResponseOutputStream
@Nonnull OutputStream getResponseOutputStream(int theStatusCode, String theContentType, @Nullable Integer theContentLength) throws IOException Initiate a new binary response. The OutputStream returned by this method must be finalized by callingcommitResponse(Closeable)
later. This method should only be used for non-textual responses, for those usegetResponseWriter(int, String, String, boolean)
.Note that the caller should not close the returned object, but should instead just return it to
commitResponse(Closeable)
upon successful completion. This is different from normal Java practice where you would request it in atry with resource
block, since in Servlets you are not actually required to close the writer/stream, and doing so automatically may prevent you from correctly handling exceptions.- Parameters:
theStatusCode
- The HTTP status code.theContentType
- The HTTP response content type.theContentLength
- If known, the number of bytes that will be written. null otherwise.- Returns:
- Returns an
OutputStream
that can accept the response body. - Throws:
IOException
-
commitResponse
Finalizes the response streaming using the writer that was returned by calling eithergetResponseWriter(int, String, String, boolean)
orgetResponseOutputStream(int, String, Integer)
. This method should only be called if the response writing/streaming actually completed successfully. If an error occurred you do not need to commit the response.- Parameters:
theWriterOrOutputStream
- TheWriter
orOutputStream
that was returned by this object, or a Writer/OutputStream which decorates the one returned by this object.- Returns:
- If the server style requires a returned response object (i.e. JAX-RS Server), this method
returns that object. If the server style does not require one (i.e.
RestfulServer
), this method returns null. - Throws:
IOException
-
addHeader
Adds a response header. This method must be called prior to callinggetResponseWriter(int, String, String, boolean)
orgetResponseOutputStream(int, String, Integer)
.- Parameters:
headerKey
- The header nameheaderValue
- The header value
-
getHeaders
Map<String,List<String>> getHeaders()Returns the headers added to this response
-