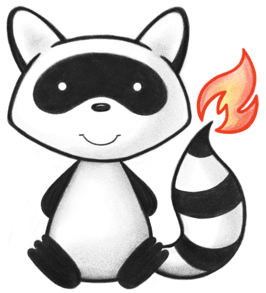
Class RestfulServer
- All Implemented Interfaces:
IRestfulServer<ServletRequestDetails>
,IRestfulServerDefaults
,jakarta.servlet.Servlet
,jakarta.servlet.ServletConfig
,Serializable
See HAPI FHIR Plain Server for information on how to use this framework.
- See Also:
-
Field Summary
FieldsModifier and TypeFieldDescriptionstatic final ETagSupportEnum
Default setting forETag Support
:ETagSupportEnum.ENABLED
static final ca.uhn.fhir.rest.api.PreferReturnEnum
Default value forsetDefaultPreferReturn(PreferReturnEnum)
static final String
All incoming requests will have an attribute added toServletRequest.getAttribute(String)
with this key.static final String
Requests will have an HttpServletRequest attribute set with this name, containing the servlet context, in order to avoid a dependency on Servlet-API 3.0+Fields inherited from class jakarta.servlet.http.HttpServlet
LEGACY_DO_HEAD
-
Constructor Summary
ConstructorsConstructorDescriptionConstructor.RestfulServer
(ca.uhn.fhir.context.FhirContext theCtx) ConstructorRestfulServer
(ca.uhn.fhir.context.FhirContext theCtx, ca.uhn.fhir.interceptor.api.IInterceptorService theInterceptorService) -
Method Summary
Modifier and TypeMethodDescriptionvoid
addHeadersToResponse
(jakarta.servlet.http.HttpServletResponse theHttpResponse) This method is called prior to sending a response to incoming requests.protected void
addRequestIdToResponse
(ServletRequestDetails theRequestDetails, String theRequestId) protected String
Subclasses may override to provide their own powered by header.protected String
Subclasses my overrideprotected String
Subclasses my overrideprotected String
Subclasses my overridevoid
destroy()
determineResourceMethod
(RequestDetails requestDetails, String requestPath) Figure out and return whichever method binding is appropriate for the given requestprotected void
doDelete
(jakarta.servlet.http.HttpServletRequest request, jakarta.servlet.http.HttpServletResponse response) protected void
doGet
(jakarta.servlet.http.HttpServletRequest request, jakarta.servlet.http.HttpServletResponse response) protected void
doOptions
(jakarta.servlet.http.HttpServletRequest theReq, jakarta.servlet.http.HttpServletResponse theResp) protected void
doPost
(jakarta.servlet.http.HttpServletRequest request, jakarta.servlet.http.HttpServletResponse response) protected void
doPut
(jakarta.servlet.http.HttpServletRequest request, jakarta.servlet.http.HttpServletResponse response) protected static int
escapedLength
(String theServletPath) Count length of URL string, but treating unescaped sequences (e.g.ca.uhn.fhir.context.api.AddProfileTagEnum
Deprecated.ca.uhn.fhir.context.api.BundleInclusionRule
org.hl7.fhir.instance.model.api.IBaseConformance
getCapabilityStatement
(ServletRequestDetails theRequestDetails) Create a CapabilityStatement based on the given requestReturns the server copyright (will be added to the CapabilityStatement).Default page size for searches.ca.uhn.fhir.rest.api.PreferReturnEnum
By default, server create/update/patch/transaction methods return a copy of the resource as it was stored.ca.uhn.fhir.rest.api.EncodingEnum
Returns the default encoding to return (XML/JSON) if an incoming request does not specify a preference (either with the_format
URL parameter, or with anAccept
header in the request.ca.uhn.fhir.context.FhirContext
Gets theFhirContext
associated with this server.Deprecated.As of HAPI FHIR 3.8.0, usegetInterceptorService()
to access the interceptor service.ca.uhn.fhir.interceptor.api.IInterceptorService
Returns the interceptor registry for this service.Maximum page size for searches.protected String
getOrCreateRequestId
(jakarta.servlet.http.HttpServletRequest theRequest) Reads a request ID from the request headers via theConstants.HEADER_REQUEST_ID
header, or generates one if none is supplied.Returns the paging provider for this serverProvides the non-resource specific providers which implement method calls on this servergetProviderMethodBindings
(Object theProvider) protected String
getRequestPath
(String requestFullPath, String servletContextPath, String servletPath) Allows users of RestfulServer to override the getRequestPath method to let them build their custom request path implementationProvides the resource providers for this serverGet the server address strategy, which is used to determine what base URL to provide clients to refer to this server.getServerBaseForRequest
(ServletRequestDetails theRequest) Returns the server base URL (with no trailing '/') for a given requestReturns the method bindings for this server which are not specific to any particular resource type.Returns the server conformance provider, which is the provider that is used to generate the server's conformance (metadata) statement if one has been explicitly defined.Gets the server's name, as exported in conformance profiles exported by the server.Gets the server's version, as exported in conformance profiles exported by the server.protected void
handleRequest
(ca.uhn.fhir.rest.api.RequestTypeEnum theRequestType, jakarta.servlet.http.HttpServletRequest theRequest, jakarta.servlet.http.HttpServletResponse theResponse) final void
init()
Initializes the server.protected void
This method may be overridden by subclasses to do perform initialization that needs to be performed prior to the server being used.boolean
Should the server "pretty print" responses by default (requesting clients can always override this default by supplying anAccept
header in the request, or a_pretty
parameter in the request URL.boolean
If set totrue
(the default istrue
) this server will not use the parsed request parameters (URL parameters and HTTP POST form contents) but will instead parse these values manually from the request URL and request body.boolean
Should the server attempt to decompress incoming request contents (default istrue
).protected ServletRequestDetails
Deprecated.Deprecated in HAPI FHIR 4.1.0 - Users wishing to override this method should overridenewRequestDetails(RequestTypeEnum, HttpServletRequest, HttpServletResponse)
insteadprotected ServletRequestDetails
newRequestDetails
(ca.uhn.fhir.rest.api.RequestTypeEnum theRequestType, jakarta.servlet.http.HttpServletRequest theRequest, jakarta.servlet.http.HttpServletResponse theResponse) Subclasses may override this to customize the way that the RequestDetails object is created.protected String
newRequestId
(int theRequestIdLength) Generate a new request ID string.void
populateRequestDetailsFromRequestPath
(RequestDetails theRequestDetails, String theRequestPath) void
registerInterceptor
(Object theInterceptor) Registers an interceptor.void
registerProvider
(Object provider) Register a single provider.void
registerProviders
(Object... theProviders) Register a group of providers.void
registerProviders
(Collection<?> theProviders) Register a group of theProviders.protected void
registerProviders
(Collection<?> theProviders, boolean inInit) returnResponse
(ServletRequestDetails theRequest, BaseParseAction<?> outcome, int operationStatus, boolean allowPrefer, ca.uhn.fhir.rest.api.MethodOutcome response, String resourceName) protected void
service
(jakarta.servlet.http.HttpServletRequest theReq, jakarta.servlet.http.HttpServletResponse theResp) void
setAddProfileTag
(ca.uhn.fhir.context.api.AddProfileTagEnum theAddProfileTag) Deprecated.As of HAPI FHIR 1.5, this property has been moved toFhirContext.setAddProfileTagWhenEncoding(AddProfileTagEnum)
void
setBundleInclusionRule
(ca.uhn.fhir.context.api.BundleInclusionRule theBundleInclusionRule) Set how bundle factory should decide whether referenced resources should be included in bundlesvoid
setCopyright
(String theCopyright) Sets the server copyright (will be added to the CapabilityStatement).void
setDefaultPageSize
(Integer thePageSize) Sets the default page size to use, ornull
if no default page sizevoid
setDefaultPreferReturn
(ca.uhn.fhir.rest.api.PreferReturnEnum theDefaultPreferReturn) By default, server create/update/patch/transaction methods return a copy of the resource as it was stored.void
setDefaultPrettyPrint
(boolean theDefaultPrettyPrint) Should the server "pretty print" responses by default (requesting clients can always override this default by supplying anAccept
header in the request, or a_pretty
parameter in the request URL.void
setDefaultResponseEncoding
(ca.uhn.fhir.rest.api.EncodingEnum theDefaultResponseEncoding) Sets the default encoding to return (XML/JSON) if an incoming request does not specify a preference (either with the_format
URL parameter, or with anAccept
header in the request.void
setElementsSupport
(ElementsSupportEnum theElementsSupport) Sets the elements support mode.void
setETagSupport
(ETagSupportEnum theETagSupport) Sets (enables/disables) the server support for ETags.void
setFhirContext
(ca.uhn.fhir.context.FhirContext theFhirContext) void
setIgnoreServerParsedRequestParameters
(boolean theIgnoreServerParsedRequestParameters) If set totrue
(the default istrue
) this server will not use the parsed request parameters (URL parameters and HTTP POST form contents) but will instead parse these values manually from the request URL and request body.void
setImplementationDescription
(String theImplementationDescription) void
setInterceptors
(IServerInterceptor... theInterceptors) Deprecated.As of HAPI FHIR 3.8.0, usegetInterceptorService()
to access the interceptor service.void
setInterceptors
(List<?> theList) Deprecated.As of HAPI FHIR 3.8.0, usegetInterceptorService()
to access the interceptor service.void
setInterceptorService
(ca.uhn.fhir.interceptor.api.IInterceptorService theInterceptorService) Sets the interceptor registry for this service.void
setMaximumPageSize
(Integer theMaximumPageSize) Sets the maximum page size to use, ornull
if no maximum page sizevoid
setPagingProvider
(IPagingProvider thePagingProvider) Sets the paging provider to use, ornull
to use no paging (which is the default).void
setPlainProviders
(Object... theProv) Deprecated.This method causes inconsistent behaviour depending on the order it is called in.void
setPlainProviders
(Collection<Object> theProviders) Deprecated.This method causes inconsistent behaviour depending on the order it is called in.void
setProviders
(Object... theProviders) Sets the non-resource specific providers which implement method calls on this servervoid
setResourceProviders
(IResourceProvider... theResourceProviders) Sets the resource providers for this servervoid
setResourceProviders
(Collection<IResourceProvider> theProviders) Sets the resource providers for this servervoid
setServerAddressStrategy
(IServerAddressStrategy theServerAddressStrategy) Provide a server address strategy, which is used to determine what base URL to provide clients to refer to this server.void
setServerConformanceProvider
(Object theServerConformanceProvider) Returns the server conformance provider, which is the provider that is used to generate the server's conformance (metadata) statement.void
setServerName
(String theServerName) Sets the server's name, as exported in conformance profiles exported by the server.void
setServerVersion
(String theServerVersion) Gets the server's version, as exported in conformance profiles exported by the server.void
setTenantIdentificationStrategy
(ITenantIdentificationStrategy theTenantIdentificationStrategy) If provided (default isnull
), the tenant identification strategy provides a mechanism for a multitenant server to identify which tenant a given request corresponds to.void
setUncompressIncomingContents
(boolean theUncompressIncomingContents) Should the server attempt to decompress incoming request contents (default istrue
).protected void
throwUnknownFhirOperationException
(RequestDetails requestDetails, String requestPath, ca.uhn.fhir.rest.api.RequestTypeEnum theRequestType) static void
throwUnknownFhirOperationException
(RequestDetails requestDetails, String requestPath, ca.uhn.fhir.rest.api.RequestTypeEnum theRequestType, ca.uhn.fhir.context.FhirContext theFhirContext) protected void
throwUnknownResourceTypeException
(String theResourceName) void
Unregisters all plain and resource providers (but not the conformance provider).void
unregisterInterceptor
(Object theInterceptor) Unregisters an interceptor.void
unregisterProvider
(Object provider) Unregister one provider (either a Resource provider or a plain provider)void
unregisterProviders
(Collection<?> providers) Unregister aCollection
of providersprotected void
validateRequest
(ServletRequestDetails theRequestDetails) Methods inherited from class jakarta.servlet.http.HttpServlet
doHead, doTrace, getLastModified, init, service
Methods inherited from class jakarta.servlet.GenericServlet
getInitParameter, getInitParameterNames, getServletConfig, getServletContext, getServletInfo, getServletName, log, log
Methods inherited from class java.lang.Object
clone, equals, finalize, getClass, hashCode, notify, notifyAll, toString, wait, wait, wait
Methods inherited from interface ca.uhn.fhir.rest.api.server.IRestfulServer
canStoreSearchResults
-
Field Details
-
REQUEST_START_TIME
All incoming requests will have an attribute added toServletRequest.getAttribute(String)
with this key. The value will be a JavaDate
with the time that request processing began. -
DEFAULT_ETAG_SUPPORT
Default setting forETag Support
:ETagSupportEnum.ENABLED
-
SERVLET_CONTEXT_ATTRIBUTE
Requests will have an HttpServletRequest attribute set with this name, containing the servlet context, in order to avoid a dependency on Servlet-API 3.0+- See Also:
-
DEFAULT_PREFER_RETURN
Default value forsetDefaultPreferReturn(PreferReturnEnum)
-
-
Constructor Details
-
RestfulServer
public RestfulServer()Constructor. Note that if noFhirContext
is passed in to the server (either through the constructor, or throughsetFhirContext(FhirContext)
) the server will determine which version of FHIR to support through classpath scanning. This is brittle, and it is highly recommended to explicitly specify a FHIR version. -
RestfulServer
Constructor -
RestfulServer
public RestfulServer(ca.uhn.fhir.context.FhirContext theCtx, ca.uhn.fhir.interceptor.api.IInterceptorService theInterceptorService)
-
-
Method Details
-
getServerConformanceMethod
- Since:
- 5.5.0
-
addHeadersToResponse
This method is called prior to sending a response to incoming requests. It is used to add custom headers.Use caution if overriding this method: it is recommended to call
super.addHeadersToResponse
to avoid inadvertently disabling functionality. -
createConfiguration
-
createPoweredByAttributes
-
createPoweredByHeader
Subclasses may override to provide their own powered by header. Note that if you want to be nice and still credit HAPI FHIR you could consider overridingcreatePoweredByAttributes()
instead and adding your own fragments to the list. -
createPoweredByHeaderComponentName
Subclasses my override- See Also:
-
createPoweredByHeaderProductName
Subclasses my override- See Also:
-
createPoweredByHeaderProductVersion
Subclasses my override- See Also:
-
destroy
- Specified by:
destroy
in interfacejakarta.servlet.Servlet
- Overrides:
destroy
in classjakarta.servlet.GenericServlet
-
determineResourceMethod
Figure out and return whichever method binding is appropriate for the given request -
doDelete
protected void doDelete(jakarta.servlet.http.HttpServletRequest request, jakarta.servlet.http.HttpServletResponse response) throws jakarta.servlet.ServletException, IOException - Overrides:
doDelete
in classjakarta.servlet.http.HttpServlet
- Throws:
jakarta.servlet.ServletException
IOException
-
doGet
protected void doGet(jakarta.servlet.http.HttpServletRequest request, jakarta.servlet.http.HttpServletResponse response) throws jakarta.servlet.ServletException, IOException - Overrides:
doGet
in classjakarta.servlet.http.HttpServlet
- Throws:
jakarta.servlet.ServletException
IOException
-
doOptions
protected void doOptions(jakarta.servlet.http.HttpServletRequest theReq, jakarta.servlet.http.HttpServletResponse theResp) throws jakarta.servlet.ServletException, IOException - Overrides:
doOptions
in classjakarta.servlet.http.HttpServlet
- Throws:
jakarta.servlet.ServletException
IOException
-
doPost
protected void doPost(jakarta.servlet.http.HttpServletRequest request, jakarta.servlet.http.HttpServletResponse response) throws jakarta.servlet.ServletException, IOException - Overrides:
doPost
in classjakarta.servlet.http.HttpServlet
- Throws:
jakarta.servlet.ServletException
IOException
-
doPut
protected void doPut(jakarta.servlet.http.HttpServletRequest request, jakarta.servlet.http.HttpServletResponse response) throws jakarta.servlet.ServletException, IOException - Overrides:
doPut
in classjakarta.servlet.http.HttpServlet
- Throws:
jakarta.servlet.ServletException
IOException
-
getAddProfileTag
Deprecated.As of HAPI FHIR 1.5, this property has been moved toFhirContext.setAddProfileTagWhenEncoding(AddProfileTagEnum)
- Specified by:
getAddProfileTag
in interfaceIRestfulServerDefaults
- Returns:
- Returns the setting for automatically adding profile tags
-
setAddProfileTag
@Deprecated public void setAddProfileTag(ca.uhn.fhir.context.api.AddProfileTagEnum theAddProfileTag) Deprecated.As of HAPI FHIR 1.5, this property has been moved toFhirContext.setAddProfileTagWhenEncoding(AddProfileTagEnum)
Sets the profile tagging behaviour for the server. When set to a value other thanAddProfileTagEnum.NEVER
(which is the default), the server will automatically add a profile tag based on the class of the resource(s) being returned.- Parameters:
theAddProfileTag
- The behaviour enum (must not be null)
-
getBundleInclusionRule
- Specified by:
getBundleInclusionRule
in interfaceIRestfulServer<ServletRequestDetails>
-
setBundleInclusionRule
public void setBundleInclusionRule(ca.uhn.fhir.context.api.BundleInclusionRule theBundleInclusionRule) Set how bundle factory should decide whether referenced resources should be included in bundles- Parameters:
theBundleInclusionRule
- - inclusion rule (@see BundleInclusionRule for behaviors)
-
getDefaultResponseEncoding
Returns the default encoding to return (XML/JSON) if an incoming request does not specify a preference (either with the_format
URL parameter, or with anAccept
header in the request. The default isEncodingEnum.XML
. Will not return null.- Specified by:
getDefaultResponseEncoding
in interfaceIRestfulServerDefaults
- Returns:
- Returns the default encoding to return (XML/JSON) if an incoming request does not specify a preference
(either with the
_format
URL parameter, or with anAccept
header in the request. The default isEncodingEnum.XML
. Will not return null.
-
setDefaultResponseEncoding
public void setDefaultResponseEncoding(ca.uhn.fhir.rest.api.EncodingEnum theDefaultResponseEncoding) Sets the default encoding to return (XML/JSON) if an incoming request does not specify a preference (either with the_format
URL parameter, or with anAccept
header in the request. The default isEncodingEnum.XML
.Note when testing this feature: Some browsers will include "application/xml" in their Accept header, which means that the
-
getETagSupport
- Specified by:
getETagSupport
in interfaceIRestfulServerDefaults
- Returns:
- Returns the server support for ETags (will not be
null
). Default isDEFAULT_ETAG_SUPPORT
-
setETagSupport
Sets (enables/disables) the server support for ETags. Must not benull
. Default isDEFAULT_ETAG_SUPPORT
- Parameters:
theETagSupport
- The ETag support mode
-
getElementsSupport
- Specified by:
getElementsSupport
in interfaceIRestfulServerDefaults
- Returns:
- Returns the support option for the
_elements
parameter on search and read operations. - See Also:
-
setElementsSupport
Sets the elements support mode.- See Also:
-
getFhirContext
Gets theFhirContext
associated with this server. For efficient processing, resource providers and plain providers should generally use this context if one is needed, as opposed to creating their own.- Specified by:
getFhirContext
in interfaceIRestfulServerDefaults
-
setFhirContext
-
getImplementationDescription
-
setImplementationDescription
-
getCopyright
Returns the server copyright (will be added to the CapabilityStatement). Note that FHIR allows Markdown in this string. -
setCopyright
Sets the server copyright (will be added to the CapabilityStatement). Note that FHIR allows Markdown in this string. -
getInterceptors_
Deprecated.As of HAPI FHIR 3.8.0, usegetInterceptorService()
to access the interceptor service. You can register and unregister interceptors using this service.Returns a list of all registered server interceptors- Specified by:
getInterceptors_
in interfaceIRestfulServerDefaults
-
getInterceptorService
Returns the interceptor registry for this service. Use this registry to register and unregister- Specified by:
getInterceptorService
in interfaceIRestfulServerDefaults
- Since:
- 3.8.0
-
setInterceptorService
public void setInterceptorService(@Nonnull ca.uhn.fhir.interceptor.api.IInterceptorService theInterceptorService) Sets the interceptor registry for this service. Use this registry to register and unregister- Since:
- 3.8.0
-
setInterceptors
Deprecated.As of HAPI FHIR 3.8.0, usegetInterceptorService()
to access the interceptor service. You can register and unregister interceptors using this service.Sets (or clears) the list of interceptors- Parameters:
theList
- The list of interceptors (may be null)
-
setInterceptors
Deprecated.As of HAPI FHIR 3.8.0, usegetInterceptorService()
to access the interceptor service. You can register and unregister interceptors using this service.Sets (or clears) the list of interceptors- Parameters:
theInterceptors
- The list of interceptors (may be null)
-
getPagingProvider
Description copied from interface:IRestfulServerDefaults
Returns the paging provider for this server- Specified by:
getPagingProvider
in interfaceIRestfulServer<ServletRequestDetails>
- Specified by:
getPagingProvider
in interfaceIRestfulServerDefaults
-
setPagingProvider
Sets the paging provider to use, ornull
to use no paging (which is the default). This will set defaultPageSize and maximumPageSize from the paging provider. -
getDefaultPageSize
Description copied from interface:IRestfulServerDefaults
Default page size for searches. Null means no limit (JpaStorageSettings might have size limit however)- Specified by:
getDefaultPageSize
in interfaceIRestfulServerDefaults
-
setDefaultPageSize
Sets the default page size to use, ornull
if no default page size -
getMaximumPageSize
Description copied from interface:IRestfulServerDefaults
Maximum page size for searches. Null means no upper limit.- Specified by:
getMaximumPageSize
in interfaceIRestfulServerDefaults
-
setMaximumPageSize
Sets the maximum page size to use, ornull
if no maximum page size -
getPlainProviders
Provides the non-resource specific providers which implement method calls on this server- See Also:
-
setPlainProviders
Deprecated.This method causes inconsistent behaviour depending on the order it is called in. UseregisterProviders(Object...)
instead.Sets the non-resource specific providers which implement method calls on this server.- See Also:
-
setPlainProviders
Deprecated.This method causes inconsistent behaviour depending on the order it is called in. UseregisterProviders(Object...)
instead.Sets the non-resource specific providers which implement method calls on this server.- See Also:
-
getRequestPath
protected String getRequestPath(String requestFullPath, String servletContextPath, String servletPath) Allows users of RestfulServer to override the getRequestPath method to let them build their custom request path implementation- Parameters:
requestFullPath
- the full request pathservletContextPath
- the servelet context pathservletPath
- the servelet path- Returns:
- created resource path
-
getResourceBindings
-
getProviderMethodBindings
-
getResourceProviders
Provides the resource providers for this server -
setResourceProviders
Sets the resource providers for this server -
setResourceProviders
Sets the resource providers for this server -
getServerAddressStrategy
Get the server address strategy, which is used to determine what base URL to provide clients to refer to this server. Defaults to an instance ofIncomingRequestAddressStrategy
-
setServerAddressStrategy
Provide a server address strategy, which is used to determine what base URL to provide clients to refer to this server. Defaults to an instance ofIncomingRequestAddressStrategy
-
getServerBaseForRequest
Returns the server base URL (with no trailing '/') for a given request -
getServerBindings
Returns the method bindings for this server which are not specific to any particular resource type. This method is internal to HAPI and developers generally do not need to interact with it. Use with caution, as it may change. -
getServerConformanceProvider
Returns the server conformance provider, which is the provider that is used to generate the server's conformance (metadata) statement if one has been explicitly defined.By default, the ServerConformanceProvider for the declared version of FHIR is used, but this can be changed, or set to
null
to use the appropriate one for the given FHIR version. -
setServerConformanceProvider
Returns the server conformance provider, which is the provider that is used to generate the server's conformance (metadata) statement.By default, the ServerConformanceProvider implementation for the declared version of FHIR is used, but this can be changed, or set to
This method should only be called before the server is initialized. Calling it after the server has started is allowed, but you should be very careful in this case that you only call it while no traffic is hitting the server.null
if you do not wish to export a conformance statement.- Throws:
IllegalStateException
- Note that this method can only be called prior toinitialization
and will throw anIllegalStateException
if called after that.
-
getServerName
Gets the server's name, as exported in conformance profiles exported by the server. This is informational only, but can be helpful to set with something appropriate.- See Also:
-
setServerName
Sets the server's name, as exported in conformance profiles exported by the server. This is informational only, but can be helpful to set with something appropriate. -
getServerVersion
Gets the server's version, as exported in conformance profiles exported by the server. This is informational only, but can be helpful to set with something appropriate. -
setServerVersion
Gets the server's version, as exported in conformance profiles exported by the server. This is informational only, but can be helpful to set with something appropriate. -
handleRequest
protected void handleRequest(ca.uhn.fhir.rest.api.RequestTypeEnum theRequestType, jakarta.servlet.http.HttpServletRequest theRequest, jakarta.servlet.http.HttpServletResponse theResponse) throws jakarta.servlet.ServletException, IOException - Throws:
jakarta.servlet.ServletException
IOException
-
newRequestDetails
@Nonnull protected ServletRequestDetails newRequestDetails(ca.uhn.fhir.rest.api.RequestTypeEnum theRequestType, jakarta.servlet.http.HttpServletRequest theRequest, jakarta.servlet.http.HttpServletResponse theResponse) Subclasses may override this to customize the way that the RequestDetails object is created. Generally speaking, the right way to do this is to override this method, but call the super-implementation (super.newRequestDetails
) and then customize the returned object before returning it.- Parameters:
theRequestType
- The HTTP request verbtheRequest
- The servlet requesttheResponse
- The servlet response- Returns:
- A ServletRequestDetails instance to be passed to any resource providers, interceptors, etc. that are invoked as a part of serving this request.
-
newRequestDetails
Deprecated.Deprecated in HAPI FHIR 4.1.0 - Users wishing to override this method should overridenewRequestDetails(RequestTypeEnum, HttpServletRequest, HttpServletResponse)
instead -
addRequestIdToResponse
-
getOrCreateRequestId
Reads a request ID from the request headers via theConstants.HEADER_REQUEST_ID
header, or generates one if none is supplied.Note that the generated request ID is a random 64-bit long integer encoded as hexadecimal. It is not generated using any cryptographic algorithms or a secure PRNG, so it should not be used for anything other than troubleshooting purposes.
-
newRequestId
Generate a new request ID string. Subclasses may ovrride. -
validateRequest
-
init
Initializes the server. Note that this method is final to avoid accidentally introducing bugs in implementations, but subclasses may put initialization code ininitialize()
, which is called immediately before beginning initialization of the restful server's internal init.- Overrides:
init
in classjakarta.servlet.GenericServlet
- Throws:
jakarta.servlet.ServletException
-
initialize
This method may be overridden by subclasses to do perform initialization that needs to be performed prior to the server being used.- Throws:
jakarta.servlet.ServletException
- If the initialization failed. Note that you should consider throwingUnavailableException
(which extendsServletException
), as this is a flag to the servlet container that the servlet is not usable.
-
isDefaultPrettyPrint
Should the server "pretty print" responses by default (requesting clients can always override this default by supplying anAccept
header in the request, or a_pretty
parameter in the request URL.The default is
false
Note that this setting is ignored by
ResponseHighlighterInterceptor
when streaming HTML, although even when that interceptor it used this setting will still be honoured when streaming raw FHIR.- Specified by:
isDefaultPrettyPrint
in interfaceIRestfulServerDefaults
- Returns:
- Returns the default pretty print setting
-
setDefaultPrettyPrint
Should the server "pretty print" responses by default (requesting clients can always override this default by supplying anAccept
header in the request, or a_pretty
parameter in the request URL.The default is
false
Note that this setting is ignored by
ResponseHighlighterInterceptor
when streaming HTML, although even when that interceptor it used this setting will still be honoured when streaming raw FHIR.- Parameters:
theDefaultPrettyPrint
- The default pretty print setting
-
isIgnoreServerParsedRequestParameters
If set totrue
(the default istrue
) this server will not use the parsed request parameters (URL parameters and HTTP POST form contents) but will instead parse these values manually from the request URL and request body.This is useful because many servlet containers (e.g. Tomcat, Glassfish) will use ISO-8859-1 encoding to parse escaped URL characters instead of using UTF-8 as is specified by FHIR.
-
setIgnoreServerParsedRequestParameters
If set totrue
(the default istrue
) this server will not use the parsed request parameters (URL parameters and HTTP POST form contents) but will instead parse these values manually from the request URL and request body.This is useful because many servlet containers (e.g. Tomcat, Glassfish) will use ISO-8859-1 encoding to parse escaped URL characters instead of using UTF-8 as is specified by FHIR.
-
isUncompressIncomingContents
Should the server attempt to decompress incoming request contents (default istrue
). Typically this should be set totrue
unless the server has other configuration to deal with decompressing request bodies (e.g. a filter applied to the whole server). -
setUncompressIncomingContents
Should the server attempt to decompress incoming request contents (default istrue
). Typically this should be set totrue
unless the server has other configuration to deal with decompressing request bodies (e.g. a filter applied to the whole server). -
populateRequestDetailsFromRequestPath
public void populateRequestDetailsFromRequestPath(RequestDetails theRequestDetails, String theRequestPath) -
registerInterceptor
Registers an interceptor. This method is a convenience method which callsgetInterceptorService().registerInterceptor(theInterceptor);
- Parameters:
theInterceptor
- The interceptor, must not be null
-
registerProvider
Register a single provider. This could be a Resource Provider or a "plain" provider not associated with any resource. -
registerProviders
Register a group of providers. These could be Resource Providers (classes implementingIResourceProvider
) or "plain" providers, or a mixture of the two.- Parameters:
theProviders
- aCollection
of theProviders. The parameter could be null or an emptyCollection
-
registerProviders
Register a group of theProviders. These could be Resource Providers, "plain" theProviders or a mixture of the two.- Parameters:
theProviders
- aCollection
of theProviders. The parameter could be null or an emptyCollection
-
registerProviders
-
returnResponse
public Object returnResponse(ServletRequestDetails theRequest, BaseParseAction<?> outcome, int operationStatus, boolean allowPrefer, ca.uhn.fhir.rest.api.MethodOutcome response, String resourceName) throws IOException - Throws:
IOException
-
service
protected void service(jakarta.servlet.http.HttpServletRequest theReq, jakarta.servlet.http.HttpServletResponse theResp) throws jakarta.servlet.ServletException, IOException - Overrides:
service
in classjakarta.servlet.http.HttpServlet
- Throws:
jakarta.servlet.ServletException
IOException
-
setProviders
Sets the non-resource specific providers which implement method calls on this server- See Also:
-
setTenantIdentificationStrategy
public void setTenantIdentificationStrategy(ITenantIdentificationStrategy theTenantIdentificationStrategy) If provided (default isnull
), the tenant identification strategy provides a mechanism for a multitenant server to identify which tenant a given request corresponds to. -
throwUnknownFhirOperationException
protected void throwUnknownFhirOperationException(RequestDetails requestDetails, String requestPath, ca.uhn.fhir.rest.api.RequestTypeEnum theRequestType) -
throwUnknownResourceTypeException
-
unregisterInterceptor
Unregisters an interceptor. This method is a convenience method which callsgetInterceptorService().unregisterInterceptor(theInterceptor);
- Parameters:
theInterceptor
- The interceptor, must not be null
-
unregisterProvider
Unregister one provider (either a Resource provider or a plain provider) -
unregisterProviders
Unregister aCollection
of providers -
unregisterAllProviders
Unregisters all plain and resource providers (but not the conformance provider). -
getDefaultPreferReturn
By default, server create/update/patch/transaction methods return a copy of the resource as it was stored. This may be overridden by the client using thePrefer
header.This setting changes the default behaviour if no Prefer header is supplied by the client. The default is
PreferReturnEnum.REPRESENTATION
- Specified by:
getDefaultPreferReturn
in interfaceIRestfulServer<ServletRequestDetails>
- See Also:
-
setDefaultPreferReturn
By default, server create/update/patch/transaction methods return a copy of the resource as it was stored. This may be overridden by the client using thePrefer
header.This setting changes the default behaviour if no Prefer header is supplied by the client. The default is
PreferReturnEnum.REPRESENTATION
- See Also:
-
getCapabilityStatement
public org.hl7.fhir.instance.model.api.IBaseConformance getCapabilityStatement(ServletRequestDetails theRequestDetails) Create a CapabilityStatement based on the given request -
escapedLength
Count length of URL string, but treating unescaped sequences (e.g. ' ') as their unescaped equivalent (%20) -
throwUnknownFhirOperationException
public static void throwUnknownFhirOperationException(RequestDetails requestDetails, String requestPath, ca.uhn.fhir.rest.api.RequestTypeEnum theRequestType, ca.uhn.fhir.context.FhirContext theFhirContext)
-
FhirContext.setAddProfileTagWhenEncoding(AddProfileTagEnum)