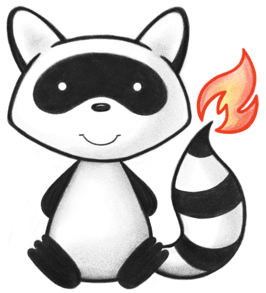
Package ca.uhn.fhir.rest.server.provider
Class ObservableSupplierSet<T extends IObservableSupplierSetObserver>
java.lang.Object
ca.uhn.fhir.rest.server.provider.ObservableSupplierSet<T>
- Type Parameters:
T
- the class of the ObserverA typical usage pattern would be:
- Create
ObservableSupplierSet
in exporter context. - Add all the suppliers in the exporter context.
- Attach the importer to the
ObservableSupplierSet
- Importer calls
getSupplierResults()
and processes all the beans - Some other service beans may add more suppliers later as a part of their initialization and the observer handlers will process them accordingly
- Those other service beans should call
removeSupplier(Supplier)
in a @PreDestroy method so they are properly cleaned up if those services are shut down or restarted
- Create
- Direct Known Subclasses:
ResourceProviderFactory
This is a generic implementation of the Observer Design Pattern.
We use this to pass sets of beans from exporting Spring application contexts to importing Spring application contexts. We defer
resolving the observed beans via a Supplier to give the exporting context a chance to initialize the beans before they are used.
-
Constructor Summary
Constructors -
Method Summary
Modifier and TypeMethodDescriptionvoid
addSupplier
(Supplier<Object> theSupplier) Add a supplier and notify all observersvoid
Attach an observer to this observableSupplierSet.void
Detach an observer from this observableSupplierSet, so it is no longer notified when suppliers are added and removed.void
removeSupplier
(Supplier<Object> theSupplier) Remove a supplier and notify all observers.
-
Constructor Details
-
ObservableSupplierSet
public ObservableSupplierSet()
-
-
Method Details
-
addSupplier
Add a supplier and notify all observers- Parameters:
theSupplier
- supplies the object to be observed
-
removeSupplier
Remove a supplier and notify all observers. CAUTION, you might think that this code would work, but it does not:observableSupplierSet.addSupplier(() -> myBean); ... observableSupplierSet.removeSupplier(() -> myBean);
the removeSupplier in this example would fail because it is a different lambda instance from the first. Instead, you need to store the supplier between the add and remove:mySupplier = () -> myBean; observableSupplierSet.addSupplier(mySupplier); ... observableSupplierSet.removeSupplier(mySupplier);
- Parameters:
theSupplier
- the supplier to be removed
-
attach
Attach an observer to this observableSupplierSet. This observer will be notified every time a supplier is added or removed.- Parameters:
theObserver
- the observer to be notified
-
detach
Detach an observer from this observableSupplierSet, so it is no longer notified when suppliers are added and removed.- Parameters:
theObserver
- the observer to be removed
-
getSupplierResults
- Returns:
- a list of get() being called on all suppliers.
-