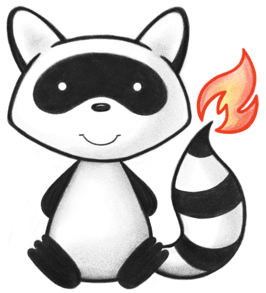
Package ca.uhn.fhir.jpa.api.svc
Interface IIdHelperService<T extends ca.uhn.fhir.rest.api.server.storage.IResourcePersistentId<?>>
public interface IIdHelperService<T extends ca.uhn.fhir.rest.api.server.storage.IResourcePersistentId<?>>
This interface is used to translate between
IResourcePersistentId
and actual resource IDs.-
Method Summary
Modifier and TypeMethodDescriptionvoid
addResolvedPidToFhirIdAfterCommit
(T theResourcePersistentId, RequestPartitionId theRequestPartitionId, String theResourceType, String theFhirId, Date theDeletedAt) This method can be called to pre-emptively add entries to the ID cache.getPidOrNull
(RequestPartitionId theRequestPartitionId, org.hl7.fhir.instance.model.api.IBaseResource theResource) default T
getPidOrThrowException
(RequestPartitionId theRequestPartitionId, org.hl7.fhir.instance.model.api.IIdType theId) getPidOrThrowException
(org.hl7.fhir.instance.model.api.IAnyResource theResource) boolean
idRequiresForcedId
(String theId) Returns true if the given resource ID should be stored in a forced ID.newPidFromStringIdAndResourceName
(String thePid, String theResourceType) Map
<org.hl7.fhir.instance.model.api.IIdType, IResourceLookup<T>> resolveResourceIdentities
(RequestPartitionId theRequestPartitionId, Collection<org.hl7.fhir.instance.model.api.IIdType> theIds, ResolveIdentityMode theMode) Given a collection of resource IDs, resolve the resource identities, including the persistent ID, deleted status, resource type, etc.resolveResourceIdentity
(RequestPartitionId theRequestPartitionId, String theResourceType, String theResourceId, ResolveIdentityMode theMode) default T
resolveResourceIdentityPid
(RequestPartitionId theRequestPartitionId, String theResourceType, String theResourceId, ResolveIdentityMode theMode) resolveResourcePids
(RequestPartitionId theRequestPartitionId, List<org.hl7.fhir.instance.model.api.IIdType> theTargetIds, ResolveIdentityMode theResolveIdentityMode) Given a collection of resource IDs, resolve the resource persistent IDs.org.hl7.fhir.instance.model.api.IIdType
resourceIdFromPidOrThrowException
(T thePid, String theResourceType) org.hl7.fhir.instance.model.api.IIdType
translatePidIdToForcedId
(ca.uhn.fhir.context.FhirContext theCtx, String theResourceType, T theId) Given a persistent ID, returns the associated resource IDtranslatePidIdToForcedIdWithCache
(T theResourcePersistentId) Value will be an empty Optional if the PID doesn't exist, or a typed resource ID if so (Patient/ABC).translatePidsToFhirResourceIds
(Set<T> thePids) Given a set of PIDs, return a set of public FHIR Resource IDs.translatePidsToForcedIds
(Set<T> theResourceIds) Values in the returned map are typed resource IDs (Patient/ABC)
-
Method Details
-
translatePidIdToForcedId
@Nonnull org.hl7.fhir.instance.model.api.IIdType translatePidIdToForcedId(ca.uhn.fhir.context.FhirContext theCtx, String theResourceType, T theId) Given a persistent ID, returns the associated resource ID -
resolveResourceIdentity
@Nonnull IResourceLookup<T> resolveResourceIdentity(@Nonnull RequestPartitionId theRequestPartitionId, @Nullable String theResourceType, @Nonnull String theResourceId, @Nonnull ResolveIdentityMode theMode) throws ca.uhn.fhir.rest.server.exceptions.ResourceNotFoundException - Parameters:
theResourceType
- Note that it is inefficient to call this method with a null resource type, so this should be avoided unless strictly necessary.- Throws:
ca.uhn.fhir.rest.server.exceptions.ResourceNotFoundException
- If the ID can not be found
-
resolveResourceIdentityPid
@Nonnull default T resolveResourceIdentityPid(@Nonnull RequestPartitionId theRequestPartitionId, @Nullable String theResourceType, @Nonnull String theResourceId, @Nonnull ResolveIdentityMode theMode) throws ca.uhn.fhir.rest.server.exceptions.ResourceNotFoundException - Parameters:
theResourceType
- Note that it is inefficient to call this method with a null resource type, so this should be avoided unless strictly necessary.- Throws:
ca.uhn.fhir.rest.server.exceptions.ResourceNotFoundException
- If the ID can not be found
-
resolveResourceIdentities
@Nonnull Map<org.hl7.fhir.instance.model.api.IIdType,IResourceLookup<T>> resolveResourceIdentities(@Nonnull RequestPartitionId theRequestPartitionId, Collection<org.hl7.fhir.instance.model.api.IIdType> theIds, ResolveIdentityMode theMode) Given a collection of resource IDs, resolve the resource identities, including the persistent ID, deleted status, resource type, etc.- Since:
- 8.0.0
-
resolveResourcePids
default List<T> resolveResourcePids(RequestPartitionId theRequestPartitionId, List<org.hl7.fhir.instance.model.api.IIdType> theTargetIds, ResolveIdentityMode theResolveIdentityMode) Given a collection of resource IDs, resolve the resource persistent IDs.- Since:
- 8.0.0
-
idRequiresForcedId
Returns true if the given resource ID should be stored in a forced ID. Under default config (meaning client ID strategy isJpaStorageSettings.ClientIdStrategyEnum.ALPHANUMERIC
) this will return true if the ID has any non-digit characters.In
JpaStorageSettings.ClientIdStrategyEnum.ANY
mode it will always return true. -
translatePidIdToForcedIdWithCache
Value will be an empty Optional if the PID doesn't exist, or a typed resource ID if so (Patient/ABC). -
translatePidsToForcedIds
Values in the returned map are typed resource IDs (Patient/ABC) -
addResolvedPidToFhirIdAfterCommit
void addResolvedPidToFhirIdAfterCommit(@Nonnull T theResourcePersistentId, @Nonnull RequestPartitionId theRequestPartitionId, @Nonnull String theResourceType, @Nonnull String theFhirId, @Nullable Date theDeletedAt) This method can be called to pre-emptively add entries to the ID cache. It should be called by DAO methods if they are creating or changing the deleted status of a resource. This method returns immediately, but the data is not added to the internal caches until the current DB transaction is successfully committed, and nothing is added if the transaction rolls back. -
getPidOrNull
@Nullable T getPidOrNull(RequestPartitionId theRequestPartitionId, org.hl7.fhir.instance.model.api.IBaseResource theResource) -
getPidOrThrowException
@Nonnull default T getPidOrThrowException(RequestPartitionId theRequestPartitionId, org.hl7.fhir.instance.model.api.IIdType theId) -
getPidOrThrowException
@Nonnull T getPidOrThrowException(@Nonnull org.hl7.fhir.instance.model.api.IAnyResource theResource) -
resourceIdFromPidOrThrowException
org.hl7.fhir.instance.model.api.IIdType resourceIdFromPidOrThrowException(T thePid, String theResourceType) -
translatePidsToFhirResourceIds
Given a set of PIDs, return a set of public FHIR Resource IDs. This function will resolve a forced ID if it resolves, and if it fails to resolve to a forced it, will just return the pid Example: Let's say we have Patient/1(pid == 1), Patient/pat1 (pid == 2), Patient/3 (pid == 3), their pids would resolve as follows:[1,2,3] -> ["1","pat1","3"]
- Parameters:
thePids
- The Set of pids you would like to resolve to external FHIR Resource IDs.- Returns:
- A Set of strings representing the FHIR IDs of the pids.
-
newPid
-
newPidFromStringIdAndResourceName
-