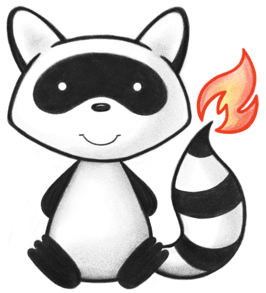
Class QuantityDt
- All Implemented Interfaces:
ca.uhn.fhir.model.api.ICompositeDatatype
,ca.uhn.fhir.model.api.ICompositeElement
,ca.uhn.fhir.model.api.IDatatype
,ca.uhn.fhir.model.api.IElement
,ca.uhn.fhir.model.api.IIdentifiableElement
,ca.uhn.fhir.model.api.IQueryParameterType
,ca.uhn.fhir.model.api.ISupportsUndeclaredExtensions
,Serializable
,org.hl7.fhir.instance.model.api.IBase
,org.hl7.fhir.instance.model.api.IBaseDatatype
,org.hl7.fhir.instance.model.api.ICompositeType
- Direct Known Subclasses:
AgeDt
,CountDt
,DistanceDt
,DurationDt
,MoneyDt
,SimpleQuantityDt
Definition: A measured amount (or an amount that can potentially be measured). Note that measured amounts include amounts that are not precisely quantified, including amounts involving arbitrary units and floating currencies
Requirements: Need to able to capture all sorts of measured values, even if the measured value are not precisely quantified. Values include exact measures such as 3.51g, customary units such as 3 tablets, and currencies such as $100.32USD
- See Also:
-
Constructor Summary
ConstructorsConstructorDescriptionConstructorQuantityDt
(double theValue) ConstructorQuantityDt
(long theValue) ConstructorQuantityDt
(QuantityComparatorEnum theComparator, double theValue, String theUnits) ConstructorQuantityDt
(QuantityComparatorEnum theComparator, double theValue, String theSystem, String theUnits) ConstructorQuantityDt
(QuantityComparatorEnum theComparator, long theValue, String theUnits) ConstructorQuantityDt
(QuantityComparatorEnum theComparator, long theValue, String theSystem, String theUnits) Constructor -
Method Summary
Modifier and TypeMethodDescription<T extends ca.uhn.fhir.model.api.IElement>
List<T> getAllPopulatedChildElementsOfType
(Class<T> theType) getCode()
Gets the value(s) for code ().ca.uhn.fhir.model.primitive.CodeDt
Gets the value(s) for code ().Gets the value(s) for comparator ().ca.uhn.fhir.model.primitive.BoundCodeDt
<QuantityComparatorEnum> Gets the value(s) for comparator ().Gets the value(s) for system ().ca.uhn.fhir.model.primitive.UriDt
Gets the value(s) for system ().getUnit()
Gets the value(s) for unit ().ca.uhn.fhir.model.primitive.StringDt
Gets the value(s) for unit ().ca.uhn.fhir.model.primitive.StringDt
Deprecated.getValue()
Gets the value(s) for value ().ca.uhn.fhir.model.primitive.DecimalDt
Gets the value(s) for value ().boolean
isEmpty()
setCode
(ca.uhn.fhir.model.primitive.CodeDt theValue) Sets the value(s) for code ()Sets the value for code ()setComparator
(QuantityComparatorEnum theValue) Sets the value(s) for comparator ()setComparator
(ca.uhn.fhir.model.primitive.BoundCodeDt<QuantityComparatorEnum> theValue) Sets the value(s) for comparator ()setSystem
(ca.uhn.fhir.model.primitive.UriDt theValue) Sets the value(s) for system ()Sets the value for system ()setUnit
(ca.uhn.fhir.model.primitive.StringDt theValue) Sets the value(s) for unit ()Sets the value for unit ()ca.uhn.fhir.model.base.composite.BaseQuantityDt
Deprecated.UsesetUnit(String)
instead - Quantity.units was renamed to Quantity.unit in DSTU2setValue
(double theValue) Sets the value for value ()setValue
(long theValue) Sets the value for value ()setValue
(ca.uhn.fhir.model.primitive.DecimalDt theValue) Sets the value(s) for value ()setValue
(BigDecimal theValue) Sets the value for value ()Methods inherited from class ca.uhn.fhir.model.base.composite.BaseQuantityDt
getMissing, getQueryParameterQualifier, getValueAsQueryToken, setMissing, setValueAsQueryToken
Methods inherited from class ca.uhn.fhir.model.api.BaseIdentifiableElement
getElementSpecificId, getId, setElementSpecificId, setId, setId
Methods inherited from class ca.uhn.fhir.model.api.BaseElement
addUndeclaredExtension, addUndeclaredExtension, addUndeclaredExtension, getAllUndeclaredExtensions, getFormatCommentsPost, getFormatCommentsPre, getUndeclaredExtensions, getUndeclaredExtensionsByUrl, getUndeclaredModifierExtensions, getUserData, hasFormatComment, isBaseEmpty, setUserData
Methods inherited from class java.lang.Object
clone, equals, finalize, getClass, hashCode, notify, notifyAll, toString, wait, wait, wait
Methods inherited from interface org.hl7.fhir.instance.model.api.IBase
fhirType, getFormatCommentsPost, getFormatCommentsPre, getUserData, hasFormatComment, setUserData
-
Constructor Details
-
QuantityDt
public QuantityDt()Constructor -
QuantityDt
Constructor -
QuantityDt
Constructor -
QuantityDt
Constructor -
QuantityDt
Constructor -
QuantityDt
public QuantityDt(QuantityComparatorEnum theComparator, double theValue, String theSystem, String theUnits) Constructor -
QuantityDt
public QuantityDt(QuantityComparatorEnum theComparator, long theValue, String theSystem, String theUnits) Constructor
-
-
Method Details
-
setUnits
Deprecated.UsesetUnit(String)
instead - Quantity.units was renamed to Quantity.unit in DSTU2- Specified by:
setUnits
in classca.uhn.fhir.model.base.composite.BaseQuantityDt
-
getUnitsElement
Deprecated.UsegetUnitElement()
- Quantity.units was renamed to Quantity.unit in DSTU2- Specified by:
getUnitsElement
in classca.uhn.fhir.model.base.composite.BaseQuantityDt
-
isEmpty
- Specified by:
isEmpty
in interfaceorg.hl7.fhir.instance.model.api.IBase
-
getAllPopulatedChildElementsOfType
public <T extends ca.uhn.fhir.model.api.IElement> List<T> getAllPopulatedChildElementsOfType(Class<T> theType) - Specified by:
getAllPopulatedChildElementsOfType
in interfaceca.uhn.fhir.model.api.ICompositeElement
-
getValueElement
Gets the value(s) for value (). creating it if it does not exist. Will not returnnull
.Definition: The value of the measured amount. The value includes an implicit precision in the presentation of the value
- Specified by:
getValueElement
in classca.uhn.fhir.model.base.composite.BaseQuantityDt
-
getValue
Gets the value(s) for value (). creating it if it does not exist. This method may returnnull
.Definition: The value of the measured amount. The value includes an implicit precision in the presentation of the value
-
setValue
Sets the value(s) for value ()Definition: The value of the measured amount. The value includes an implicit precision in the presentation of the value
-
setValue
Sets the value for value ()Definition: The value of the measured amount. The value includes an implicit precision in the presentation of the value
-
setValue
Sets the value for value ()Definition: The value of the measured amount. The value includes an implicit precision in the presentation of the value
-
setValue
Sets the value for value ()Definition: The value of the measured amount. The value includes an implicit precision in the presentation of the value
- Specified by:
setValue
in classca.uhn.fhir.model.base.composite.BaseQuantityDt
-
getComparatorElement
Gets the value(s) for comparator (). creating it if it does not exist. Will not returnnull
.Definition: How the value should be understood and represented - whether the actual value is greater or less than the stated value due to measurement issues; e.g. if the comparator is \"<\" , then the real value is < stated value
- Specified by:
getComparatorElement
in classca.uhn.fhir.model.base.composite.BaseQuantityDt
-
getComparator
Gets the value(s) for comparator (). creating it if it does not exist. This method may returnnull
.Definition: How the value should be understood and represented - whether the actual value is greater or less than the stated value due to measurement issues; e.g. if the comparator is \"<\" , then the real value is < stated value
-
setComparator
public QuantityDt setComparator(ca.uhn.fhir.model.primitive.BoundCodeDt<QuantityComparatorEnum> theValue) Sets the value(s) for comparator ()Definition: How the value should be understood and represented - whether the actual value is greater or less than the stated value due to measurement issues; e.g. if the comparator is \"<\" , then the real value is < stated value
-
setComparator
Sets the value(s) for comparator ()Definition: How the value should be understood and represented - whether the actual value is greater or less than the stated value due to measurement issues; e.g. if the comparator is \"<\" , then the real value is < stated value
-
getUnitElement
Gets the value(s) for unit (). creating it if it does not exist. Will not returnnull
.Definition: A human-readable form of the unit
-
getUnit
Gets the value(s) for unit (). creating it if it does not exist. This method may returnnull
.Definition: A human-readable form of the unit
-
setUnit
Sets the value(s) for unit ()Definition: A human-readable form of the unit
-
setUnit
Sets the value for unit ()Definition: A human-readable form of the unit
-
getSystemElement
Gets the value(s) for system (). creating it if it does not exist. Will not returnnull
.Definition: The identification of the system that provides the coded form of the unit
- Specified by:
getSystemElement
in classca.uhn.fhir.model.base.composite.BaseQuantityDt
-
getSystem
Gets the value(s) for system (). creating it if it does not exist. This method may returnnull
.Definition: The identification of the system that provides the coded form of the unit
-
setSystem
Sets the value(s) for system ()Definition: The identification of the system that provides the coded form of the unit
-
setSystem
Sets the value for system ()Definition: The identification of the system that provides the coded form of the unit
- Specified by:
setSystem
in classca.uhn.fhir.model.base.composite.BaseQuantityDt
-
getCodeElement
Gets the value(s) for code (). creating it if it does not exist. Will not returnnull
.Definition: A computer processable form of the unit in some unit representation system
- Specified by:
getCodeElement
in classca.uhn.fhir.model.base.composite.BaseQuantityDt
-
getCode
Gets the value(s) for code (). creating it if it does not exist. This method may returnnull
.Definition: A computer processable form of the unit in some unit representation system
-
setCode
Sets the value(s) for code ()Definition: A computer processable form of the unit in some unit representation system
-
setCode
Sets the value for code ()Definition: A computer processable form of the unit in some unit representation system
- Specified by:
setCode
in classca.uhn.fhir.model.base.composite.BaseQuantityDt
-
getUnitElement()
- Quantity.units was renamed to Quantity.unit in DSTU2