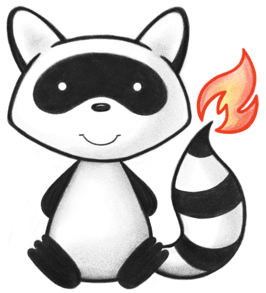
Class Account
- All Implemented Interfaces:
ca.uhn.fhir.model.api.ICompositeElement
,ca.uhn.fhir.model.api.IElement
,ca.uhn.fhir.model.api.IResource
,ca.uhn.fhir.model.api.ISupportsUndeclaredExtensions
,Serializable
,org.hl7.fhir.instance.model.api.IBase
,org.hl7.fhir.instance.model.api.IBaseResource
Definition: A financial tool for tracking value accrued for a particular purpose. In the healthcare field, used to track charges for a patient, cost centres, etc.
Requirements:
Profile Definition: http://hl7.org/fhir/profiles/Account
- See Also:
-
Field Summary
FieldsModifier and TypeFieldDescriptionstatic final ca.uhn.fhir.rest.gclient.QuantityClientParam
Fluent Client search parameter constant for balancestatic final ca.uhn.fhir.rest.gclient.TokenClientParam
Fluent Client search parameter constant for identifierstatic final ca.uhn.fhir.model.api.Include
Constant for fluent queries to be used to add include statements.static final ca.uhn.fhir.model.api.Include
Constant for fluent queries to be used to add include statements.static final ca.uhn.fhir.model.api.Include
Constant for fluent queries to be used to add include statements.static final ca.uhn.fhir.rest.gclient.StringClientParam
Fluent Client search parameter constant for namestatic final ca.uhn.fhir.rest.gclient.ReferenceClientParam
Fluent Client search parameter constant for ownerstatic final ca.uhn.fhir.rest.gclient.ReferenceClientParam
Fluent Client search parameter constant for patientstatic final ca.uhn.fhir.rest.gclient.DateClientParam
Fluent Client search parameter constant for periodstatic final String
Search parameter constant for balancestatic final String
Search parameter constant for identifierstatic final String
Search parameter constant for namestatic final String
Search parameter constant for ownerstatic final String
Search parameter constant for patientstatic final String
Search parameter constant for periodstatic final String
Search parameter constant for statusstatic final String
Search parameter constant for subjectstatic final String
Search parameter constant for typestatic final ca.uhn.fhir.rest.gclient.TokenClientParam
Fluent Client search parameter constant for statusstatic final ca.uhn.fhir.rest.gclient.ReferenceClientParam
Fluent Client search parameter constant for subjectstatic final ca.uhn.fhir.rest.gclient.TokenClientParam
Fluent Client search parameter constant for typeFields inherited from class ca.uhn.fhir.model.dstu2.resource.BaseResource
RES_ID, SP_RES_ID
Fields inherited from interface org.hl7.fhir.instance.model.api.IBaseResource
INCLUDE_ALL, WILDCARD_ALL_SET
-
Constructor Summary
Constructors -
Method Summary
Modifier and TypeMethodDescriptionAdds and returns a new value for identifier ()addIdentifier
(IdentifierDt theValue) Adds a given new value for identifier ()Gets the value(s) for activePeriod ().<T extends ca.uhn.fhir.model.api.IElement>
List<T> getAllPopulatedChildElementsOfType
(Class<T> theType) Gets the value(s) for balance ().Gets the value(s) for coveragePeriod ().Gets the value(s) for currency ().Gets the value(s) for description ().ca.uhn.fhir.model.primitive.StringDt
Gets the value(s) for description ().Gets the value(s) for identifier ().Gets the first repetition for identifier (), creating it if it does not already exist.getName()
Gets the value(s) for name ().ca.uhn.fhir.model.primitive.StringDt
Gets the value(s) for name ().getOwner()
Gets the value(s) for owner ().Gets the value(s) for status ().ca.uhn.fhir.model.primitive.BoundCodeDt
<AccountStatusEnum> Gets the value(s) for status ().ca.uhn.fhir.context.FhirVersionEnum
Gets the value(s) for subject ().getType()
Gets the value(s) for type ().boolean
isEmpty()
setActivePeriod
(PeriodDt theValue) Sets the value(s) for activePeriod ()setBalance
(MoneyDt theValue) Sets the value(s) for balance ()setCoveragePeriod
(PeriodDt theValue) Sets the value(s) for coveragePeriod ()setCurrency
(CodingDt theValue) Sets the value(s) for currency ()setDescription
(ca.uhn.fhir.model.primitive.StringDt theValue) Sets the value(s) for description ()setDescription
(String theString) Sets the value for description ()setIdentifier
(List<IdentifierDt> theValue) Sets the value(s) for identifier ()setName
(ca.uhn.fhir.model.primitive.StringDt theValue) Sets the value(s) for name ()Sets the value for name ()setOwner
(ResourceReferenceDt theValue) Sets the value(s) for owner ()setStatus
(AccountStatusEnum theValue) Sets the value(s) for status ()setStatus
(ca.uhn.fhir.model.primitive.BoundCodeDt<AccountStatusEnum> theValue) Sets the value(s) for status ()setSubject
(ResourceReferenceDt theValue) Sets the value(s) for subject ()setType
(CodeableConceptDt theValue) Sets the value(s) for type ()Methods inherited from class ca.uhn.fhir.model.dstu2.resource.BaseResource
getContained, getId, getIdElement, getLanguage, getMeta, getResourceMetadata, getText, isBaseEmpty, setContained, setId, setId, setId, setLanguage, setResourceMetadata, setText, toString
Methods inherited from class ca.uhn.fhir.model.api.BaseElement
addUndeclaredExtension, addUndeclaredExtension, addUndeclaredExtension, getAllUndeclaredExtensions, getFormatCommentsPost, getFormatCommentsPre, getUndeclaredExtensions, getUndeclaredExtensionsByUrl, getUndeclaredModifierExtensions, getUserData, hasFormatComment, setUserData
Methods inherited from class java.lang.Object
clone, equals, finalize, getClass, hashCode, notify, notifyAll, wait, wait, wait
Methods inherited from interface org.hl7.fhir.instance.model.api.IBase
fhirType, getFormatCommentsPost, getFormatCommentsPre, getUserData, hasFormatComment, setUserData
Methods inherited from interface org.hl7.fhir.instance.model.api.IBaseResource
getIdElement, isDeleted, setId, setId
Methods inherited from interface ca.uhn.fhir.model.api.IResource
getContained, getId, getLanguage, getMeta, getResourceMetadata, getText, setId, setLanguage, setResourceMetadata
-
Field Details
-
SP_IDENTIFIER
Search parameter constant for identifierDescription:
Type: token
Path: Account.identifier
- See Also:
-
IDENTIFIER
Fluent Client search parameter constant for identifierDescription:
Type: token
Path: Account.identifier
-
SP_NAME
Search parameter constant for nameDescription:
Type: string
Path: Account.name
- See Also:
-
NAME
Fluent Client search parameter constant for nameDescription:
Type: string
Path: Account.name
-
SP_TYPE
Search parameter constant for typeDescription:
Type: token
Path: Account.type
- See Also:
-
TYPE
Fluent Client search parameter constant for typeDescription:
Type: token
Path: Account.type
-
SP_STATUS
Search parameter constant for statusDescription:
Type: token
Path: Account.status
- See Also:
-
STATUS
Fluent Client search parameter constant for statusDescription:
Type: token
Path: Account.status
-
SP_BALANCE
Search parameter constant for balanceDescription:
Type: quantity
Path: Account.balance
- See Also:
-
BALANCE
Fluent Client search parameter constant for balanceDescription:
Type: quantity
Path: Account.balance
-
SP_PERIOD
Search parameter constant for periodDescription:
Type: date
Path: Account.coveragePeriod
- See Also:
-
PERIOD
Fluent Client search parameter constant for periodDescription:
Type: date
Path: Account.coveragePeriod
-
SP_SUBJECT
Search parameter constant for subjectDescription:
Type: reference
Path: Account.subject
- See Also:
-
SUBJECT
Fluent Client search parameter constant for subjectDescription:
Type: reference
Path: Account.subject
-
SP_OWNER
Search parameter constant for ownerDescription:
Type: reference
Path: Account.owner
- See Also:
-
OWNER
Fluent Client search parameter constant for ownerDescription:
Type: reference
Path: Account.owner
-
SP_PATIENT
Search parameter constant for patientDescription:
Type: reference
Path: Account.subject
- See Also:
-
PATIENT
Fluent Client search parameter constant for patientDescription:
Type: reference
Path: Account.subject
-
INCLUDE_OWNER
Constant for fluent queries to be used to add include statements. Specifies the path value of "Account:owner". -
INCLUDE_PATIENT
Constant for fluent queries to be used to add include statements. Specifies the path value of "Account:patient". -
INCLUDE_SUBJECT
Constant for fluent queries to be used to add include statements. Specifies the path value of "Account:subject".
-
-
Constructor Details
-
Account
public Account()
-
-
Method Details
-
isEmpty
- Specified by:
isEmpty
in interfaceorg.hl7.fhir.instance.model.api.IBase
-
getAllPopulatedChildElementsOfType
public <T extends ca.uhn.fhir.model.api.IElement> List<T> getAllPopulatedChildElementsOfType(Class<T> theType) - Specified by:
getAllPopulatedChildElementsOfType
in interfaceca.uhn.fhir.model.api.ICompositeElement
-
getIdentifier
Gets the value(s) for identifier (). creating it if it does not exist. Will not returnnull
.Definition: Unique identifier used to reference the account. May or may not be intended for human use (e.g. credit card number)
-
setIdentifier
Sets the value(s) for identifier ()Definition: Unique identifier used to reference the account. May or may not be intended for human use (e.g. credit card number)
-
addIdentifier
Adds and returns a new value for identifier ()Definition: Unique identifier used to reference the account. May or may not be intended for human use (e.g. credit card number)
-
addIdentifier
Adds a given new value for identifier ()Definition: Unique identifier used to reference the account. May or may not be intended for human use (e.g. credit card number)
- Parameters:
theValue
- The identifier to add (must not benull
)
-
getIdentifierFirstRep
Gets the first repetition for identifier (), creating it if it does not already exist.Definition: Unique identifier used to reference the account. May or may not be intended for human use (e.g. credit card number)
-
getNameElement
Gets the value(s) for name (). creating it if it does not exist. Will not returnnull
.Definition: Name used for the account when displaying it to humans in reports, etc.
-
getName
Gets the value(s) for name (). creating it if it does not exist. This method may returnnull
.Definition: Name used for the account when displaying it to humans in reports, etc.
-
setName
Sets the value(s) for name ()Definition: Name used for the account when displaying it to humans in reports, etc.
-
setName
Sets the value for name ()Definition: Name used for the account when displaying it to humans in reports, etc.
-
getType
Gets the value(s) for type (). creating it if it does not exist. Will not returnnull
.Definition: Categorizes the account for reporting and searching purposes
-
setType
Sets the value(s) for type ()Definition: Categorizes the account for reporting and searching purposes
-
getStatusElement
Gets the value(s) for status (). creating it if it does not exist. Will not returnnull
.Definition: Indicates whether the account is presently used/useable or not
-
getStatus
Gets the value(s) for status (). creating it if it does not exist. This method may returnnull
.Definition: Indicates whether the account is presently used/useable or not
-
setStatus
Sets the value(s) for status ()Definition: Indicates whether the account is presently used/useable or not
-
setStatus
Sets the value(s) for status ()Definition: Indicates whether the account is presently used/useable or not
-
getActivePeriod
Gets the value(s) for activePeriod (). creating it if it does not exist. Will not returnnull
.Definition: Indicates the period of time over which the account is allowed
-
setActivePeriod
Sets the value(s) for activePeriod ()Definition: Indicates the period of time over which the account is allowed
-
getCurrency
Gets the value(s) for currency (). creating it if it does not exist. Will not returnnull
.Definition: Identifies the currency to which transactions must be converted when crediting or debiting the account.
-
setCurrency
Sets the value(s) for currency ()Definition: Identifies the currency to which transactions must be converted when crediting or debiting the account.
-
getBalance
Gets the value(s) for balance (). creating it if it does not exist. Will not returnnull
.Definition: Represents the sum of all credits less all debits associated with the account. Might be positive, zero or negative.
-
setBalance
Sets the value(s) for balance ()Definition: Represents the sum of all credits less all debits associated with the account. Might be positive, zero or negative.
-
getCoveragePeriod
Gets the value(s) for coveragePeriod (). creating it if it does not exist. Will not returnnull
.Definition: Identifies the period of time the account applies to; e.g. accounts created per fiscal year, quarter, etc.
-
setCoveragePeriod
Sets the value(s) for coveragePeriod ()Definition: Identifies the period of time the account applies to; e.g. accounts created per fiscal year, quarter, etc.
-
getSubject
Gets the value(s) for subject (). creating it if it does not exist. Will not returnnull
.Definition: Identifies the patient, device, practitioner, location or other object the account is associated with
-
setSubject
Sets the value(s) for subject ()Definition: Identifies the patient, device, practitioner, location or other object the account is associated with
-
getOwner
Gets the value(s) for owner (). creating it if it does not exist. Will not returnnull
.Definition: Indicates the organization, department, etc. with responsibility for the account.
-
setOwner
Sets the value(s) for owner ()Definition: Indicates the organization, department, etc. with responsibility for the account.
-
getDescriptionElement
Gets the value(s) for description (). creating it if it does not exist. Will not returnnull
.Definition: Provides additional information about what the account tracks and how it is used
-
getDescription
Gets the value(s) for description (). creating it if it does not exist. This method may returnnull
.Definition: Provides additional information about what the account tracks and how it is used
-
setDescription
Sets the value(s) for description ()Definition: Provides additional information about what the account tracks and how it is used
-
setDescription
Sets the value for description ()Definition: Provides additional information about what the account tracks and how it is used
-
getResourceName
- Specified by:
getResourceName
in interfaceca.uhn.fhir.model.api.IResource
-
getStructureFhirVersionEnum
- Specified by:
getStructureFhirVersionEnum
in interfaceorg.hl7.fhir.instance.model.api.IBaseResource
- Specified by:
getStructureFhirVersionEnum
in interfaceca.uhn.fhir.model.api.IResource
-