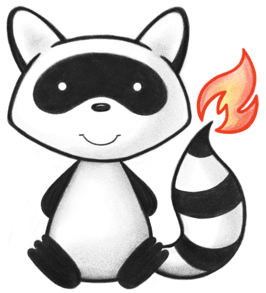
Class Bundle
- All Implemented Interfaces:
ca.uhn.fhir.model.api.ICompositeElement
,ca.uhn.fhir.model.api.IElement
,ca.uhn.fhir.model.api.IResource
,ca.uhn.fhir.model.api.ISupportsUndeclaredExtensions
,Serializable
,org.hl7.fhir.instance.model.api.IBase
,org.hl7.fhir.instance.model.api.IBaseBundle
,org.hl7.fhir.instance.model.api.IBaseResource
Definition: A container for a collection of resources.
Requirements:
Profile Definition: http://hl7.org/fhir/profiles/Bundle
- See Also:
-
Nested Class Summary
Nested ClassesModifier and TypeClassDescriptionstatic class
Block class for child element: Bundle.entry ()static class
Block class for child element: Bundle.entry.request ()static class
Block class for child element: Bundle.entry.response ()static class
Block class for child element: Bundle.entry.search ()static class
Block class for child element: Bundle.link () -
Field Summary
FieldsModifier and TypeFieldDescriptionstatic final ca.uhn.fhir.rest.gclient.ReferenceClientParam
Fluent Client search parameter constant for compositionstatic final ca.uhn.fhir.model.api.Include
Constant for fluent queries to be used to add include statements.static final ca.uhn.fhir.model.api.Include
Constant for fluent queries to be used to add include statements.static final ca.uhn.fhir.rest.gclient.ReferenceClientParam
Fluent Client search parameter constant for messagestatic final String
Search parameter constant for compositionstatic final String
Search parameter constant for messagestatic final String
Search parameter constant for typestatic final ca.uhn.fhir.rest.gclient.TokenClientParam
Fluent Client search parameter constant for typeFields inherited from class ca.uhn.fhir.model.dstu2.resource.BaseResource
RES_ID, SP_RES_ID
Fields inherited from interface org.hl7.fhir.instance.model.api.IBaseBundle
LINK_NEXT, LINK_PREV, LINK_SELF
Fields inherited from interface org.hl7.fhir.instance.model.api.IBaseResource
INCLUDE_ALL, WILDCARD_ALL_SET
-
Constructor Summary
Constructors -
Method Summary
Modifier and TypeMethodDescriptionaddEntry()
Adds and returns a new value for entry ()addEntry
(Bundle.Entry theValue) Adds a given new value for entry ()addLink()
Adds and returns a new value for link ()addLink
(Bundle.Link theValue) Adds a given new value for link ()<T extends ca.uhn.fhir.model.api.IElement>
List<T> getAllPopulatedChildElementsOfType
(Class<T> theType) getEntry()
Gets the value(s) for entry ().Gets the first repetition for entry (), creating it if it does not already exist.getLink()
Gets the value(s) for link ().Gets the first repetition for link (), creating it if it does not already exist.getLinkOrCreate
(String theRelation) Gets the value(s) for signature ().ca.uhn.fhir.context.FhirVersionEnum
getTotal()
Gets the value(s) for total ().ca.uhn.fhir.model.primitive.UnsignedIntDt
Gets the value(s) for total ().getType()
Gets the value(s) for type ().ca.uhn.fhir.model.primitive.BoundCodeDt
<BundleTypeEnum> Gets the value(s) for type ().boolean
isEmpty()
setEntry
(List<Bundle.Entry> theValue) Sets the value(s) for entry ()setLink
(List<Bundle.Link> theValue) Sets the value(s) for link ()setSignature
(SignatureDt theValue) Sets the value(s) for signature ()setTotal
(int theInteger) Sets the value for total ()setTotal
(ca.uhn.fhir.model.primitive.UnsignedIntDt theValue) Sets the value(s) for total ()setType
(BundleTypeEnum theValue) Sets the value(s) for type ()setType
(ca.uhn.fhir.model.primitive.BoundCodeDt<BundleTypeEnum> theValue) Sets the value(s) for type ()Methods inherited from class ca.uhn.fhir.model.dstu2.resource.BaseResource
getContained, getId, getIdElement, getLanguage, getMeta, getResourceMetadata, getText, isBaseEmpty, setContained, setId, setId, setId, setLanguage, setResourceMetadata, setText, toString
Methods inherited from class ca.uhn.fhir.model.api.BaseElement
addUndeclaredExtension, addUndeclaredExtension, addUndeclaredExtension, getAllUndeclaredExtensions, getFormatCommentsPost, getFormatCommentsPre, getUndeclaredExtensions, getUndeclaredExtensionsByUrl, getUndeclaredModifierExtensions, getUserData, hasFormatComment, setUserData
Methods inherited from class java.lang.Object
clone, equals, finalize, getClass, hashCode, notify, notifyAll, wait, wait, wait
Methods inherited from interface org.hl7.fhir.instance.model.api.IBase
fhirType, getFormatCommentsPost, getFormatCommentsPre, getUserData, hasFormatComment, setUserData
Methods inherited from interface org.hl7.fhir.instance.model.api.IBaseResource
getIdElement, isDeleted, setId, setId
Methods inherited from interface ca.uhn.fhir.model.api.IResource
getContained, getId, getLanguage, getMeta, getResourceMetadata, getText, setId, setLanguage, setResourceMetadata
-
Field Details
-
SP_TYPE
Search parameter constant for typeDescription:
Type: token
Path: Bundle.type
- See Also:
-
TYPE
Fluent Client search parameter constant for typeDescription:
Type: token
Path: Bundle.type
-
SP_MESSAGE
Search parameter constant for messageDescription: The first resource in the bundle, if the bundle type is \"message\" - this is a message header, and this parameter provides access to search its contents
Type: reference
Path: Bundle.entry.resource(0)
- See Also:
-
MESSAGE
Fluent Client search parameter constant for messageDescription: The first resource in the bundle, if the bundle type is \"message\" - this is a message header, and this parameter provides access to search its contents
Type: reference
Path: Bundle.entry.resource(0)
-
SP_COMPOSITION
Search parameter constant for compositionDescription: The first resource in the bundle, if the bundle type is \"document\" - this is a composition, and this parameter provides access to searches its contents
Type: reference
Path: Bundle.entry.resource(0)
- See Also:
-
COMPOSITION
Fluent Client search parameter constant for compositionDescription: The first resource in the bundle, if the bundle type is \"document\" - this is a composition, and this parameter provides access to searches its contents
Type: reference
Path: Bundle.entry.resource(0)
-
INCLUDE_COMPOSITION
Constant for fluent queries to be used to add include statements. Specifies the path value of "Bundle:composition". -
INCLUDE_MESSAGE
Constant for fluent queries to be used to add include statements. Specifies the path value of "Bundle:message".
-
-
Constructor Details
-
Bundle
public Bundle()
-
-
Method Details
-
isEmpty
- Specified by:
isEmpty
in interfaceorg.hl7.fhir.instance.model.api.IBase
-
getAllPopulatedChildElementsOfType
public <T extends ca.uhn.fhir.model.api.IElement> List<T> getAllPopulatedChildElementsOfType(Class<T> theType) - Specified by:
getAllPopulatedChildElementsOfType
in interfaceca.uhn.fhir.model.api.ICompositeElement
-
getTypeElement
Gets the value(s) for type (). creating it if it does not exist. Will not returnnull
.Definition: Indicates the purpose of this bundle- how it was intended to be used
-
getType
Gets the value(s) for type (). creating it if it does not exist. This method may returnnull
.Definition: Indicates the purpose of this bundle- how it was intended to be used
-
setType
Sets the value(s) for type ()Definition: Indicates the purpose of this bundle- how it was intended to be used
-
setType
Sets the value(s) for type ()Definition: Indicates the purpose of this bundle- how it was intended to be used
-
getTotalElement
Gets the value(s) for total (). creating it if it does not exist. Will not returnnull
.Definition: If a set of search matches, this is the total number of matches for the search (as opposed to the number of results in this bundle)
-
getTotal
Gets the value(s) for total (). creating it if it does not exist. This method may returnnull
.Definition: If a set of search matches, this is the total number of matches for the search (as opposed to the number of results in this bundle)
-
setTotal
Sets the value(s) for total ()Definition: If a set of search matches, this is the total number of matches for the search (as opposed to the number of results in this bundle)
-
setTotal
Sets the value for total ()Definition: If a set of search matches, this is the total number of matches for the search (as opposed to the number of results in this bundle)
-
getLink
Gets the value(s) for link (). creating it if it does not exist. Will not returnnull
.Definition: A series of links that provide context to this bundle
-
setLink
Sets the value(s) for link ()Definition: A series of links that provide context to this bundle
-
addLink
Adds and returns a new value for link ()Definition: A series of links that provide context to this bundle
-
addLink
Adds a given new value for link ()Definition: A series of links that provide context to this bundle
- Parameters:
theValue
- The link to add (must not benull
)
-
getLinkFirstRep
Gets the first repetition for link (), creating it if it does not already exist.Definition: A series of links that provide context to this bundle
-
getEntry
Gets the value(s) for entry (). creating it if it does not exist. Will not returnnull
.Definition: An entry in a bundle resource - will either contain a resource, or information about a resource (transactions and history only)
-
setEntry
Sets the value(s) for entry ()Definition: An entry in a bundle resource - will either contain a resource, or information about a resource (transactions and history only)
-
addEntry
Adds and returns a new value for entry ()Definition: An entry in a bundle resource - will either contain a resource, or information about a resource (transactions and history only)
-
addEntry
Adds a given new value for entry ()Definition: An entry in a bundle resource - will either contain a resource, or information about a resource (transactions and history only)
- Parameters:
theValue
- The entry to add (must not benull
)
-
getEntryFirstRep
Gets the first repetition for entry (), creating it if it does not already exist.Definition: An entry in a bundle resource - will either contain a resource, or information about a resource (transactions and history only)
-
getSignature
Gets the value(s) for signature (). creating it if it does not exist. Will not returnnull
.Definition: Digital Signature - base64 encoded. XML DigSIg or a JWT
-
setSignature
Sets the value(s) for signature ()Definition: Digital Signature - base64 encoded. XML DigSIg or a JWT
-
getResourceName
- Specified by:
getResourceName
in interfaceca.uhn.fhir.model.api.IResource
-
getStructureFhirVersionEnum
- Specified by:
getStructureFhirVersionEnum
in interfaceorg.hl7.fhir.instance.model.api.IBaseResource
- Specified by:
getStructureFhirVersionEnum
in interfaceca.uhn.fhir.model.api.IResource
-
getLink
Returns thelink
which matches a givenrelation
. If no link is found which matches the given relation, returnsnull
. If more than one link is found which matches the given relation, returns the first matching Link.- Parameters:
theRelation
- The relation, such as "next", or "self. See the constants such asinvalid reference
IBaseBundle#LINK_SELF
invalid reference
IBaseBundle#LINK_NEXT
- Returns:
- Returns a matching Link, or
null
- See Also:
-
getLinkOrCreate
Returns thelink
which matches a givenrelation
. If no link is found which matches the given relation, creates a new Link with the given relation and adds it to this Bundle. If more than one link is found which matches the given relation, returns the first matching Link.- Parameters:
theRelation
- The relation, such as "next", or "self. See the constants such asinvalid reference
IBaseBundle#LINK_SELF
invalid reference
IBaseBundle#LINK_NEXT
- Returns:
- Returns a matching Link, or
null
- See Also:
-