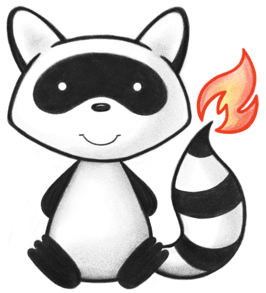
Class Device
- All Implemented Interfaces:
ca.uhn.fhir.model.api.ICompositeElement
,ca.uhn.fhir.model.api.IElement
,ca.uhn.fhir.model.api.IResource
,ca.uhn.fhir.model.api.ISupportsUndeclaredExtensions
,Serializable
,org.hl7.fhir.instance.model.api.IBase
,org.hl7.fhir.instance.model.api.IBaseResource
Definition: This resource identifies an instance of a manufactured item that is used in the provision of healthcare without being substantially changed through that activity. The device may be a medical or non-medical device. Medical devices includes durable (reusable) medical equipment, implantable devices, as well as disposable equipment used for diagnostic, treatment, and research for healthcare and public health. Non-medical devices may include items such as a machine, cellphone, computer, application, etc.
Requirements: Allows institutions to track their devices.
Profile Definition: http://hl7.org/fhir/profiles/Device
- See Also:
-
Field Summary
FieldsModifier and TypeFieldDescriptionstatic final ca.uhn.fhir.rest.gclient.TokenClientParam
Fluent Client search parameter constant for identifierstatic final ca.uhn.fhir.model.api.Include
Constant for fluent queries to be used to add include statements.static final ca.uhn.fhir.model.api.Include
Constant for fluent queries to be used to add include statements.static final ca.uhn.fhir.model.api.Include
Constant for fluent queries to be used to add include statements.static final ca.uhn.fhir.rest.gclient.ReferenceClientParam
Fluent Client search parameter constant for locationstatic final ca.uhn.fhir.rest.gclient.StringClientParam
Fluent Client search parameter constant for manufacturerstatic final ca.uhn.fhir.rest.gclient.StringClientParam
Fluent Client search parameter constant for modelstatic final ca.uhn.fhir.rest.gclient.ReferenceClientParam
Fluent Client search parameter constant for organizationstatic final ca.uhn.fhir.rest.gclient.ReferenceClientParam
Fluent Client search parameter constant for patientstatic final String
Search parameter constant for identifierstatic final String
Search parameter constant for locationstatic final String
Search parameter constant for manufacturerstatic final String
Search parameter constant for modelstatic final String
Search parameter constant for organizationstatic final String
Search parameter constant for patientstatic final String
Search parameter constant for typestatic final String
Search parameter constant for udistatic final String
Search parameter constant for urlstatic final ca.uhn.fhir.rest.gclient.TokenClientParam
Fluent Client search parameter constant for typestatic final ca.uhn.fhir.rest.gclient.StringClientParam
Fluent Client search parameter constant for udistatic final ca.uhn.fhir.rest.gclient.UriClientParam
Fluent Client search parameter constant for urlFields inherited from class ca.uhn.fhir.model.dstu2.resource.BaseResource
RES_ID, SP_RES_ID
Fields inherited from interface org.hl7.fhir.instance.model.api.IBaseResource
INCLUDE_ALL, WILDCARD_ALL_SET
-
Constructor Summary
Constructors -
Method Summary
Modifier and TypeMethodDescriptionAdds and returns a new value for contact ()addContact
(ContactPointDt theValue) Adds a given new value for contact ()Adds and returns a new value for identifier ()addIdentifier
(IdentifierDt theValue) Adds a given new value for identifier ()addNote()
Adds and returns a new value for note ()addNote
(AnnotationDt theValue) Adds a given new value for note ()<T extends ca.uhn.fhir.model.api.IElement>
List<T> getAllPopulatedChildElementsOfType
(Class<T> theType) Gets the value(s) for contact ().Gets the first repetition for contact (), creating it if it does not already exist.Gets the value(s) for expiry ().ca.uhn.fhir.model.primitive.DateTimeDt
Gets the value(s) for expiry ().Gets the value(s) for identifier ().Gets the first repetition for identifier (), creating it if it does not already exist.Gets the value(s) for location ().Gets the value(s) for lotNumber ().ca.uhn.fhir.model.primitive.StringDt
Gets the value(s) for lotNumber ().Gets the value(s) for manufactureDate ().ca.uhn.fhir.model.primitive.DateTimeDt
Gets the value(s) for manufactureDate ().Gets the value(s) for manufacturer ().ca.uhn.fhir.model.primitive.StringDt
Gets the value(s) for manufacturer ().getModel()
Gets the value(s) for model ().ca.uhn.fhir.model.primitive.StringDt
Gets the value(s) for model ().getNote()
Gets the value(s) for note ().Gets the first repetition for note (), creating it if it does not already exist.getOwner()
Gets the value(s) for owner ().Gets the value(s) for patient ().Gets the value(s) for status ().ca.uhn.fhir.model.primitive.BoundCodeDt
<DeviceStatusEnum> Gets the value(s) for status ().ca.uhn.fhir.context.FhirVersionEnum
getType()
Gets the value(s) for type ().getUdi()
Gets the value(s) for udi ().ca.uhn.fhir.model.primitive.StringDt
Gets the value(s) for udi ().getUrl()
Gets the value(s) for url ().ca.uhn.fhir.model.primitive.UriDt
Gets the value(s) for url ().Gets the value(s) for version ().ca.uhn.fhir.model.primitive.StringDt
Gets the value(s) for version ().boolean
isEmpty()
setContact
(List<ContactPointDt> theValue) Sets the value(s) for contact ()setExpiry
(ca.uhn.fhir.model.primitive.DateTimeDt theValue) Sets the value(s) for expiry ()Sets the value for expiry ()setExpiryWithSecondsPrecision
(Date theDate) Sets the value for expiry ()setIdentifier
(List<IdentifierDt> theValue) Sets the value(s) for identifier ()setLocation
(ResourceReferenceDt theValue) Sets the value(s) for location ()setLotNumber
(ca.uhn.fhir.model.primitive.StringDt theValue) Sets the value(s) for lotNumber ()setLotNumber
(String theString) Sets the value for lotNumber ()setManufactureDate
(ca.uhn.fhir.model.primitive.DateTimeDt theValue) Sets the value(s) for manufactureDate ()setManufactureDate
(Date theDate, ca.uhn.fhir.model.api.TemporalPrecisionEnum thePrecision) Sets the value for manufactureDate ()Sets the value for manufactureDate ()setManufacturer
(ca.uhn.fhir.model.primitive.StringDt theValue) Sets the value(s) for manufacturer ()setManufacturer
(String theString) Sets the value for manufacturer ()setModel
(ca.uhn.fhir.model.primitive.StringDt theValue) Sets the value(s) for model ()Sets the value for model ()setNote
(List<AnnotationDt> theValue) Sets the value(s) for note ()setOwner
(ResourceReferenceDt theValue) Sets the value(s) for owner ()setPatient
(ResourceReferenceDt theValue) Sets the value(s) for patient ()setStatus
(DeviceStatusEnum theValue) Sets the value(s) for status ()setStatus
(ca.uhn.fhir.model.primitive.BoundCodeDt<DeviceStatusEnum> theValue) Sets the value(s) for status ()setType
(CodeableConceptDt theValue) Sets the value(s) for type ()setUdi
(ca.uhn.fhir.model.primitive.StringDt theValue) Sets the value(s) for udi ()Sets the value for udi ()setUrl
(ca.uhn.fhir.model.primitive.UriDt theValue) Sets the value(s) for url ()Sets the value for url ()setVersion
(ca.uhn.fhir.model.primitive.StringDt theValue) Sets the value(s) for version ()setVersion
(String theString) Sets the value for version ()Methods inherited from class ca.uhn.fhir.model.dstu2.resource.BaseResource
getContained, getId, getIdElement, getLanguage, getMeta, getResourceMetadata, getText, isBaseEmpty, setContained, setId, setId, setId, setLanguage, setResourceMetadata, setText, toString
Methods inherited from class ca.uhn.fhir.model.api.BaseElement
addUndeclaredExtension, addUndeclaredExtension, addUndeclaredExtension, getAllUndeclaredExtensions, getFormatCommentsPost, getFormatCommentsPre, getUndeclaredExtensions, getUndeclaredExtensionsByUrl, getUndeclaredModifierExtensions, getUserData, hasFormatComment, setUserData
Methods inherited from class java.lang.Object
clone, equals, finalize, getClass, hashCode, notify, notifyAll, wait, wait, wait
Methods inherited from interface org.hl7.fhir.instance.model.api.IBase
fhirType, getFormatCommentsPost, getFormatCommentsPre, getUserData, hasFormatComment, setUserData
Methods inherited from interface org.hl7.fhir.instance.model.api.IBaseResource
getIdElement, isDeleted, setId, setId
Methods inherited from interface ca.uhn.fhir.model.api.IResource
getContained, getId, getLanguage, getMeta, getResourceMetadata, getText, setId, setLanguage, setResourceMetadata
-
Field Details
-
SP_TYPE
Search parameter constant for typeDescription: The type of the device
Type: token
Path: Device.type
- See Also:
-
TYPE
Fluent Client search parameter constant for typeDescription: The type of the device
Type: token
Path: Device.type
-
SP_MANUFACTURER
Search parameter constant for manufacturerDescription: The manufacturer of the device
Type: string
Path: Device.manufacturer
- See Also:
-
MANUFACTURER
Fluent Client search parameter constant for manufacturerDescription: The manufacturer of the device
Type: string
Path: Device.manufacturer
-
SP_MODEL
Search parameter constant for modelDescription: The model of the device
Type: string
Path: Device.model
- See Also:
-
MODEL
Fluent Client search parameter constant for modelDescription: The model of the device
Type: string
Path: Device.model
-
SP_ORGANIZATION
Search parameter constant for organizationDescription: The organization responsible for the device
Type: reference
Path: Device.owner
- See Also:
-
ORGANIZATION
Fluent Client search parameter constant for organizationDescription: The organization responsible for the device
Type: reference
Path: Device.owner
-
SP_IDENTIFIER
Search parameter constant for identifierDescription: Instance id from manufacturer, owner, and others
Type: token
Path: Device.identifier
- See Also:
-
IDENTIFIER
Fluent Client search parameter constant for identifierDescription: Instance id from manufacturer, owner, and others
Type: token
Path: Device.identifier
-
SP_LOCATION
Search parameter constant for locationDescription: A location, where the resource is found
Type: reference
Path: Device.location
- See Also:
-
LOCATION
Fluent Client search parameter constant for locationDescription: A location, where the resource is found
Type: reference
Path: Device.location
-
SP_PATIENT
Search parameter constant for patientDescription: Patient information, if the resource is affixed to a person
Type: reference
Path: Device.patient
- See Also:
-
PATIENT
Fluent Client search parameter constant for patientDescription: Patient information, if the resource is affixed to a person
Type: reference
Path: Device.patient
-
SP_UDI
Search parameter constant for udiDescription: FDA mandated Unique Device Identifier
Type: string
Path: Device.udi
- See Also:
-
UDI
Fluent Client search parameter constant for udiDescription: FDA mandated Unique Device Identifier
Type: string
Path: Device.udi
-
SP_URL
Search parameter constant for urlDescription: Network address to contact device
Type: uri
Path: Device.url
- See Also:
-
URL
Fluent Client search parameter constant for urlDescription: Network address to contact device
Type: uri
Path: Device.url
-
INCLUDE_LOCATION
Constant for fluent queries to be used to add include statements. Specifies the path value of "Device:location". -
INCLUDE_ORGANIZATION
Constant for fluent queries to be used to add include statements. Specifies the path value of "Device:organization". -
INCLUDE_PATIENT
Constant for fluent queries to be used to add include statements. Specifies the path value of "Device:patient".
-
-
Constructor Details
-
Device
public Device()
-
-
Method Details
-
isEmpty
- Specified by:
isEmpty
in interfaceorg.hl7.fhir.instance.model.api.IBase
-
getAllPopulatedChildElementsOfType
public <T extends ca.uhn.fhir.model.api.IElement> List<T> getAllPopulatedChildElementsOfType(Class<T> theType) - Specified by:
getAllPopulatedChildElementsOfType
in interfaceca.uhn.fhir.model.api.ICompositeElement
-
getIdentifier
Gets the value(s) for identifier (). creating it if it does not exist. Will not returnnull
.Definition: Unique instance identifiers assigned to a device by organizations like manufacturers or owners. If the identifier identifies the type of device, Device.type should be used.
-
setIdentifier
Sets the value(s) for identifier ()Definition: Unique instance identifiers assigned to a device by organizations like manufacturers or owners. If the identifier identifies the type of device, Device.type should be used.
-
addIdentifier
Adds and returns a new value for identifier ()Definition: Unique instance identifiers assigned to a device by organizations like manufacturers or owners. If the identifier identifies the type of device, Device.type should be used.
-
addIdentifier
Adds a given new value for identifier ()Definition: Unique instance identifiers assigned to a device by organizations like manufacturers or owners. If the identifier identifies the type of device, Device.type should be used.
- Parameters:
theValue
- The identifier to add (must not benull
)
-
getIdentifierFirstRep
Gets the first repetition for identifier (), creating it if it does not already exist.Definition: Unique instance identifiers assigned to a device by organizations like manufacturers or owners. If the identifier identifies the type of device, Device.type should be used.
-
getType
Gets the value(s) for type (). creating it if it does not exist. Will not returnnull
.Definition: Code or identifier to identify a kind of device.
-
setType
Sets the value(s) for type ()Definition: Code or identifier to identify a kind of device.
-
getNote
Gets the value(s) for note (). creating it if it does not exist. Will not returnnull
.Definition: Descriptive information, usage information or implantation information that is not captured in an existing element.
-
setNote
Sets the value(s) for note ()Definition: Descriptive information, usage information or implantation information that is not captured in an existing element.
-
addNote
Adds and returns a new value for note ()Definition: Descriptive information, usage information or implantation information that is not captured in an existing element.
-
addNote
Adds a given new value for note ()Definition: Descriptive information, usage information or implantation information that is not captured in an existing element.
- Parameters:
theValue
- The note to add (must not benull
)
-
getNoteFirstRep
Gets the first repetition for note (), creating it if it does not already exist.Definition: Descriptive information, usage information or implantation information that is not captured in an existing element.
-
getStatusElement
Gets the value(s) for status (). creating it if it does not exist. Will not returnnull
.Definition: Status of the Device availability.
-
getStatus
Gets the value(s) for status (). creating it if it does not exist. This method may returnnull
.Definition: Status of the Device availability.
-
setStatus
Sets the value(s) for status ()Definition: Status of the Device availability.
-
setStatus
Sets the value(s) for status ()Definition: Status of the Device availability.
-
getManufacturerElement
Gets the value(s) for manufacturer (). creating it if it does not exist. Will not returnnull
.Definition: A name of the manufacturer
-
getManufacturer
Gets the value(s) for manufacturer (). creating it if it does not exist. This method may returnnull
.Definition: A name of the manufacturer
-
setManufacturer
Sets the value(s) for manufacturer ()Definition: A name of the manufacturer
-
setManufacturer
Sets the value for manufacturer ()Definition: A name of the manufacturer
-
getModelElement
Gets the value(s) for model (). creating it if it does not exist. Will not returnnull
.Definition: The \"model\" is an identifier assigned by the manufacturer to identify the product by its type. This number is shared by the all devices sold as the same type.
-
getModel
Gets the value(s) for model (). creating it if it does not exist. This method may returnnull
.Definition: The \"model\" is an identifier assigned by the manufacturer to identify the product by its type. This number is shared by the all devices sold as the same type.
-
setModel
Sets the value(s) for model ()Definition: The \"model\" is an identifier assigned by the manufacturer to identify the product by its type. This number is shared by the all devices sold as the same type.
-
setModel
Sets the value for model ()Definition: The \"model\" is an identifier assigned by the manufacturer to identify the product by its type. This number is shared by the all devices sold as the same type.
-
getVersionElement
Gets the value(s) for version (). creating it if it does not exist. Will not returnnull
.Definition: The version of the device, if the device has multiple releases under the same model, or if the device is software or carries firmware.
-
getVersion
Gets the value(s) for version (). creating it if it does not exist. This method may returnnull
.Definition: The version of the device, if the device has multiple releases under the same model, or if the device is software or carries firmware.
-
setVersion
Sets the value(s) for version ()Definition: The version of the device, if the device has multiple releases under the same model, or if the device is software or carries firmware.
-
setVersion
Sets the value for version ()Definition: The version of the device, if the device has multiple releases under the same model, or if the device is software or carries firmware.
-
getManufactureDateElement
Gets the value(s) for manufactureDate (). creating it if it does not exist. Will not returnnull
.Definition: The date and time when the device was manufactured.
-
getManufactureDate
Gets the value(s) for manufactureDate (). creating it if it does not exist. This method may returnnull
.Definition: The date and time when the device was manufactured.
-
setManufactureDate
Sets the value(s) for manufactureDate ()Definition: The date and time when the device was manufactured.
-
setManufactureDateWithSecondsPrecision
Sets the value for manufactureDate ()Definition: The date and time when the device was manufactured.
-
setManufactureDate
public Device setManufactureDate(Date theDate, ca.uhn.fhir.model.api.TemporalPrecisionEnum thePrecision) Sets the value for manufactureDate ()Definition: The date and time when the device was manufactured.
-
getExpiryElement
Gets the value(s) for expiry (). creating it if it does not exist. Will not returnnull
.Definition: The date and time beyond which this device is no longer valid or should not be used (if applicable)
-
getExpiry
Gets the value(s) for expiry (). creating it if it does not exist. This method may returnnull
.Definition: The date and time beyond which this device is no longer valid or should not be used (if applicable)
-
setExpiry
Sets the value(s) for expiry ()Definition: The date and time beyond which this device is no longer valid or should not be used (if applicable)
-
setExpiryWithSecondsPrecision
Sets the value for expiry ()Definition: The date and time beyond which this device is no longer valid or should not be used (if applicable)
-
setExpiry
Sets the value for expiry ()Definition: The date and time beyond which this device is no longer valid or should not be used (if applicable)
-
getUdiElement
Gets the value(s) for udi (). creating it if it does not exist. Will not returnnull
.Definition: United States Food and Drug Administration mandated Unique Device Identifier (UDI). Use the human readable information (the content that the user sees, which is sometimes different to the exact syntax represented in the barcode) - see http://www.fda.gov/MedicalDevices/DeviceRegulationandGuidance/UniqueDeviceIdentification/default.htm
-
getUdi
Gets the value(s) for udi (). creating it if it does not exist. This method may returnnull
.Definition: United States Food and Drug Administration mandated Unique Device Identifier (UDI). Use the human readable information (the content that the user sees, which is sometimes different to the exact syntax represented in the barcode) - see http://www.fda.gov/MedicalDevices/DeviceRegulationandGuidance/UniqueDeviceIdentification/default.htm
-
setUdi
Sets the value(s) for udi ()Definition: United States Food and Drug Administration mandated Unique Device Identifier (UDI). Use the human readable information (the content that the user sees, which is sometimes different to the exact syntax represented in the barcode) - see http://www.fda.gov/MedicalDevices/DeviceRegulationandGuidance/UniqueDeviceIdentification/default.htm
-
setUdi
Sets the value for udi ()Definition: United States Food and Drug Administration mandated Unique Device Identifier (UDI). Use the human readable information (the content that the user sees, which is sometimes different to the exact syntax represented in the barcode) - see http://www.fda.gov/MedicalDevices/DeviceRegulationandGuidance/UniqueDeviceIdentification/default.htm
-
getLotNumberElement
Gets the value(s) for lotNumber (). creating it if it does not exist. Will not returnnull
.Definition: Lot number assigned by the manufacturer
-
getLotNumber
Gets the value(s) for lotNumber (). creating it if it does not exist. This method may returnnull
.Definition: Lot number assigned by the manufacturer
-
setLotNumber
Sets the value(s) for lotNumber ()Definition: Lot number assigned by the manufacturer
-
setLotNumber
Sets the value for lotNumber ()Definition: Lot number assigned by the manufacturer
-
getOwner
Gets the value(s) for owner (). creating it if it does not exist. Will not returnnull
.Definition: An organization that is responsible for the provision and ongoing maintenance of the device.
-
setOwner
Sets the value(s) for owner ()Definition: An organization that is responsible for the provision and ongoing maintenance of the device.
-
getLocation
Gets the value(s) for location (). creating it if it does not exist. Will not returnnull
.Definition: The place where the device can be found.
-
setLocation
Sets the value(s) for location ()Definition: The place where the device can be found.
-
getPatient
Gets the value(s) for patient (). creating it if it does not exist. Will not returnnull
.Definition: Patient information, if the resource is affixed to a person
-
setPatient
Sets the value(s) for patient ()Definition: Patient information, if the resource is affixed to a person
-
getContact
Gets the value(s) for contact (). creating it if it does not exist. Will not returnnull
.Definition: Contact details for an organization or a particular human that is responsible for the device
-
setContact
Sets the value(s) for contact ()Definition: Contact details for an organization or a particular human that is responsible for the device
-
addContact
Adds and returns a new value for contact ()Definition: Contact details for an organization or a particular human that is responsible for the device
-
addContact
Adds a given new value for contact ()Definition: Contact details for an organization or a particular human that is responsible for the device
- Parameters:
theValue
- The contact to add (must not benull
)
-
getContactFirstRep
Gets the first repetition for contact (), creating it if it does not already exist.Definition: Contact details for an organization or a particular human that is responsible for the device
-
getUrlElement
Gets the value(s) for url (). creating it if it does not exist. Will not returnnull
.Definition: A network address on which the device may be contacted directly
-
getUrl
Gets the value(s) for url (). creating it if it does not exist. This method may returnnull
.Definition: A network address on which the device may be contacted directly
-
setUrl
Sets the value(s) for url ()Definition: A network address on which the device may be contacted directly
-
setUrl
Sets the value for url ()Definition: A network address on which the device may be contacted directly
-
getResourceName
- Specified by:
getResourceName
in interfaceca.uhn.fhir.model.api.IResource
-
getStructureFhirVersionEnum
- Specified by:
getStructureFhirVersionEnum
in interfaceorg.hl7.fhir.instance.model.api.IBaseResource
- Specified by:
getStructureFhirVersionEnum
in interfaceca.uhn.fhir.model.api.IResource
-