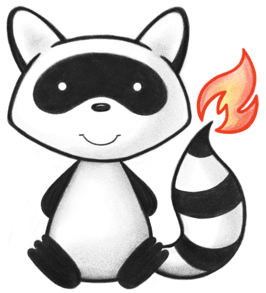
Class Medication
- All Implemented Interfaces:
ca.uhn.fhir.model.api.ICompositeElement
,ca.uhn.fhir.model.api.IElement
,ca.uhn.fhir.model.api.IResource
,ca.uhn.fhir.model.api.ISupportsUndeclaredExtensions
,Serializable
,org.hl7.fhir.instance.model.api.IBase
,org.hl7.fhir.instance.model.api.IBaseResource
Definition: This resource is primarily used for the identification and definition of a medication. It covers the ingredients and the packaging for a medication.
Requirements:
Profile Definition: http://hl7.org/fhir/profiles/Medication
- See Also:
-
Nested Class Summary
Nested ClassesModifier and TypeClassDescriptionstatic class
Block class for child element: Medication.package ()static class
Block class for child element: Medication.package.content ()static class
Block class for child element: Medication.product ()static class
Block class for child element: Medication.product.batch ()static class
Block class for child element: Medication.product.ingredient () -
Field Summary
FieldsModifier and TypeFieldDescriptionstatic final ca.uhn.fhir.rest.gclient.TokenClientParam
Fluent Client search parameter constant for codestatic final ca.uhn.fhir.rest.gclient.TokenClientParam
Fluent Client search parameter constant for containerstatic final ca.uhn.fhir.rest.gclient.ReferenceClientParam
Fluent Client search parameter constant for contentstatic final ca.uhn.fhir.rest.gclient.TokenClientParam
Fluent Client search parameter constant for formstatic final ca.uhn.fhir.model.api.Include
Constant for fluent queries to be used to add include statements.static final ca.uhn.fhir.model.api.Include
Constant for fluent queries to be used to add include statements.static final ca.uhn.fhir.model.api.Include
Constant for fluent queries to be used to add include statements.static final ca.uhn.fhir.rest.gclient.ReferenceClientParam
Fluent Client search parameter constant for ingredientstatic final ca.uhn.fhir.rest.gclient.ReferenceClientParam
Fluent Client search parameter constant for manufacturerstatic final String
Search parameter constant for codestatic final String
Search parameter constant for containerstatic final String
Search parameter constant for contentstatic final String
Search parameter constant for formstatic final String
Search parameter constant for ingredientstatic final String
Search parameter constant for manufacturerFields inherited from class ca.uhn.fhir.model.dstu2.resource.BaseResource
RES_ID, SP_RES_ID
Fields inherited from interface org.hl7.fhir.instance.model.api.IBaseResource
INCLUDE_ALL, WILDCARD_ALL_SET
-
Constructor Summary
Constructors -
Method Summary
Modifier and TypeMethodDescription<T extends ca.uhn.fhir.model.api.IElement>
List<T> getAllPopulatedChildElementsOfType
(Class<T> theType) getCode()
Gets the value(s) for code (class).Gets the value(s) for isBrand (class).ca.uhn.fhir.model.primitive.BooleanDt
Gets the value(s) for isBrand (class).Gets the value(s) for manufacturer (who.actor).Gets the value(s) for package ().Gets the value(s) for product ().ca.uhn.fhir.context.FhirVersionEnum
boolean
isEmpty()
setCode
(CodeableConceptDt theValue) Sets the value(s) for code (class)setIsBrand
(boolean theBoolean) Sets the value for isBrand (class)setIsBrand
(ca.uhn.fhir.model.primitive.BooleanDt theValue) Sets the value(s) for isBrand (class)setManufacturer
(ResourceReferenceDt theValue) Sets the value(s) for manufacturer (who.actor)setPackage
(Medication.Package theValue) Sets the value(s) for package ()setProduct
(Medication.Product theValue) Sets the value(s) for product ()Methods inherited from class ca.uhn.fhir.model.dstu2.resource.BaseResource
getContained, getId, getIdElement, getLanguage, getMeta, getResourceMetadata, getText, isBaseEmpty, setContained, setId, setId, setId, setLanguage, setResourceMetadata, setText, toString
Methods inherited from class ca.uhn.fhir.model.api.BaseElement
addUndeclaredExtension, addUndeclaredExtension, addUndeclaredExtension, getAllUndeclaredExtensions, getFormatCommentsPost, getFormatCommentsPre, getUndeclaredExtensions, getUndeclaredExtensionsByUrl, getUndeclaredModifierExtensions, getUserData, hasFormatComment, setUserData
Methods inherited from class java.lang.Object
clone, equals, finalize, getClass, hashCode, notify, notifyAll, wait, wait, wait
Methods inherited from interface org.hl7.fhir.instance.model.api.IBase
fhirType, getFormatCommentsPost, getFormatCommentsPre, getUserData, hasFormatComment, setUserData
Methods inherited from interface org.hl7.fhir.instance.model.api.IBaseResource
getIdElement, isDeleted, setId, setId
Methods inherited from interface ca.uhn.fhir.model.api.IResource
getContained, getId, getLanguage, getMeta, getResourceMetadata, getText, setId, setLanguage, setResourceMetadata
-
Field Details
-
SP_CODE
Search parameter constant for codeDescription:
Type: token
Path: Medication.code
- See Also:
-
CODE
Fluent Client search parameter constant for codeDescription:
Type: token
Path: Medication.code
-
SP_MANUFACTURER
Search parameter constant for manufacturerDescription:
Type: reference
Path: Medication.manufacturer
- See Also:
-
MANUFACTURER
Fluent Client search parameter constant for manufacturerDescription:
Type: reference
Path: Medication.manufacturer
-
SP_FORM
Search parameter constant for formDescription:
Type: token
Path: Medication.product.form
- See Also:
-
FORM
Fluent Client search parameter constant for formDescription:
Type: token
Path: Medication.product.form
-
SP_INGREDIENT
Search parameter constant for ingredientDescription:
Type: reference
Path: Medication.product.ingredient.item
- See Also:
-
INGREDIENT
Fluent Client search parameter constant for ingredientDescription:
Type: reference
Path: Medication.product.ingredient.item
-
SP_CONTAINER
Search parameter constant for containerDescription:
Type: token
Path: Medication.package.container
- See Also:
-
CONTAINER
Fluent Client search parameter constant for containerDescription:
Type: token
Path: Medication.package.container
-
SP_CONTENT
Search parameter constant for contentDescription:
Type: reference
Path: Medication.package.content.item
- See Also:
-
CONTENT
Fluent Client search parameter constant for contentDescription:
Type: reference
Path: Medication.package.content.item
-
INCLUDE_CONTENT
Constant for fluent queries to be used to add include statements. Specifies the path value of "Medication:content". -
INCLUDE_INGREDIENT
Constant for fluent queries to be used to add include statements. Specifies the path value of "Medication:ingredient". -
INCLUDE_MANUFACTURER
Constant for fluent queries to be used to add include statements. Specifies the path value of "Medication:manufacturer".
-
-
Constructor Details
-
Medication
public Medication()
-
-
Method Details
-
isEmpty
- Specified by:
isEmpty
in interfaceorg.hl7.fhir.instance.model.api.IBase
-
getAllPopulatedChildElementsOfType
public <T extends ca.uhn.fhir.model.api.IElement> List<T> getAllPopulatedChildElementsOfType(Class<T> theType) - Specified by:
getAllPopulatedChildElementsOfType
in interfaceca.uhn.fhir.model.api.ICompositeElement
-
getCode
Gets the value(s) for code (class). creating it if it does not exist. Will not returnnull
.Definition: A code (or set of codes) that specify this medication, or a textual description if no code is available. Usage note: This could be a standard medication code such as a code from RxNorm, SNOMED CT, IDMP etc. It could also be a national or local formulary code, optionally with translations to other code systems.
-
setCode
Sets the value(s) for code (class)Definition: A code (or set of codes) that specify this medication, or a textual description if no code is available. Usage note: This could be a standard medication code such as a code from RxNorm, SNOMED CT, IDMP etc. It could also be a national or local formulary code, optionally with translations to other code systems.
-
getIsBrandElement
Gets the value(s) for isBrand (class). creating it if it does not exist. Will not returnnull
.Definition: Set to true if the item is attributable to a specific manufacturer.
-
getIsBrand
Gets the value(s) for isBrand (class). creating it if it does not exist. This method may returnnull
.Definition: Set to true if the item is attributable to a specific manufacturer.
-
setIsBrand
Sets the value(s) for isBrand (class)Definition: Set to true if the item is attributable to a specific manufacturer.
-
setIsBrand
Sets the value for isBrand (class)Definition: Set to true if the item is attributable to a specific manufacturer.
-
getManufacturer
Gets the value(s) for manufacturer (who.actor). creating it if it does not exist. Will not returnnull
.Definition: Describes the details of the manufacturer
-
setManufacturer
Sets the value(s) for manufacturer (who.actor)Definition: Describes the details of the manufacturer
-
getProduct
Gets the value(s) for product (). creating it if it does not exist. Will not returnnull
.Definition: Information that only applies to products (not packages)
-
setProduct
Sets the value(s) for product ()Definition: Information that only applies to products (not packages)
-
getPackage
Gets the value(s) for package (). creating it if it does not exist. Will not returnnull
.Definition: Information that only applies to packages (not products)
-
setPackage
Sets the value(s) for package ()Definition: Information that only applies to packages (not products)
-
getResourceName
- Specified by:
getResourceName
in interfaceca.uhn.fhir.model.api.IResource
-
getStructureFhirVersionEnum
- Specified by:
getStructureFhirVersionEnum
in interfaceorg.hl7.fhir.instance.model.api.IBaseResource
- Specified by:
getStructureFhirVersionEnum
in interfaceca.uhn.fhir.model.api.IResource
-