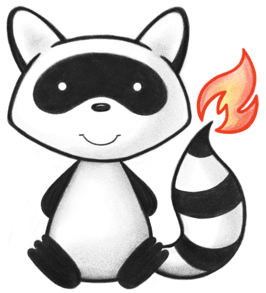
Package ca.uhn.fhir.model.dstu2.valueset
Enum Class GoalStatusEnum
- All Implemented Interfaces:
Serializable
,Comparable<GoalStatusEnum>
,Constable
-
Nested Class Summary
Nested classes/interfaces inherited from class java.lang.Enum
Enum.EnumDesc<E extends Enum<E>>
-
Enum Constant Summary
Enum ConstantsEnum ConstantDescriptionDisplay: Accepted
Code Value: accepted A proposed goal was acceptedDisplay: Achieved
Code Value: achieved The goal has been met and no further action is neededDisplay: Cancelled
Code Value: cancelled The goal is no longer being soughtDisplay: In Progress
Code Value: in-progress The goal is being sought but has not yet been reached.Display: On Hold
Code Value: on-hold The goal remains a long term objective but is no longer being actively pursued for a temporary period of time.Display: Planned
Code Value: planned A goal is planned for this patientDisplay: Proposed
Code Value: proposed A goal is proposed for this patientDisplay: Rejected
Code Value: rejected A proposed goal was rejectedDisplay: Sustaining
Code Value: sustaining The goal has been met, but ongoing activity is needed to sustain the goal objective -
Field Summary
FieldsModifier and TypeFieldDescriptionstatic final ca.uhn.fhir.model.api.IValueSetEnumBinder
<GoalStatusEnum> Converts codes to their respective enumerated valuesstatic final String
Identifier for this Value Set:static final String
Name for this Value Set: GoalStatus -
Method Summary
Modifier and TypeMethodDescriptionstatic GoalStatusEnum
Returns the enumerated value associated with this codegetCode()
Returns the code associated with this enumerated valueReturns the code system associated with this enumerated valuestatic GoalStatusEnum
Returns the enum constant of this class with the specified name.static GoalStatusEnum[]
values()
Returns an array containing the constants of this enum class, in the order they are declared.
-
Enum Constant Details
-
PROPOSED
Display: Proposed
Code Value: proposed A goal is proposed for this patient -
PLANNED
Display: Planned
Code Value: planned A goal is planned for this patient -
ACCEPTED
Display: Accepted
Code Value: accepted A proposed goal was accepted -
REJECTED
Display: Rejected
Code Value: rejected A proposed goal was rejected -
IN_PROGRESS
Display: In Progress
Code Value: in-progress The goal is being sought but has not yet been reached. (Also applies if goal was reached in the past but there has been regression and goal is being sought again) -
ACHIEVED
Display: Achieved
Code Value: achieved The goal has been met and no further action is needed -
SUSTAINING
Display: Sustaining
Code Value: sustaining The goal has been met, but ongoing activity is needed to sustain the goal objective -
ON_HOLD
Display: On Hold
Code Value: on-hold The goal remains a long term objective but is no longer being actively pursued for a temporary period of time. -
CANCELLED
Display: Cancelled
Code Value: cancelled The goal is no longer being sought
-
-
Field Details
-
VALUESET_IDENTIFIER
Identifier for this Value Set:- See Also:
-
VALUESET_NAME
Name for this Value Set: GoalStatus- See Also:
-
VALUESET_BINDER
Converts codes to their respective enumerated values
-
-
Method Details
-
values
Returns an array containing the constants of this enum class, in the order they are declared.- Returns:
- an array containing the constants of this enum class, in the order they are declared
-
valueOf
Returns the enum constant of this class with the specified name. The string must match exactly an identifier used to declare an enum constant in this class. (Extraneous whitespace characters are not permitted.)- Parameters:
name
- the name of the enum constant to be returned.- Returns:
- the enum constant with the specified name
- Throws:
IllegalArgumentException
- if this enum class has no constant with the specified nameNullPointerException
- if the argument is null
-
getCode
Returns the code associated with this enumerated value -
getSystem
Returns the code system associated with this enumerated value -
forCode
Returns the enumerated value associated with this code
-