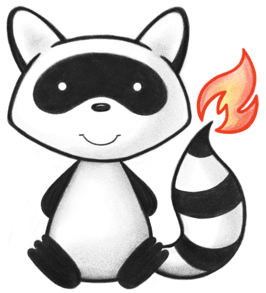
Package org.hl7.fhir.dstu3.model
Class HumanName
java.lang.Object
org.hl7.fhir.dstu3.model.Base
org.hl7.fhir.dstu3.model.Element
org.hl7.fhir.dstu3.model.Type
org.hl7.fhir.dstu3.model.HumanName
- All Implemented Interfaces:
ca.uhn.fhir.model.api.IElement
,Serializable
,org.hl7.fhir.instance.model.api.IBase
,org.hl7.fhir.instance.model.api.IBaseDatatype
,org.hl7.fhir.instance.model.api.IBaseElement
,org.hl7.fhir.instance.model.api.IBaseHasExtensions
,org.hl7.fhir.instance.model.api.ICompositeType
A human's name with the ability to identify parts and usage.
- See Also:
-
Nested Class Summary
Nested ClassesModifier and TypeClassDescriptionstatic enum
static class
-
Field Summary
FieldsModifier and TypeFieldDescriptionprotected StringType
The part of a name that links to the genealogy.protected List
<StringType> Given name.protected Period
Indicates the period of time when this name was valid for the named person.protected List
<StringType> Part of the name that is acquired as a title due to academic, legal, employment or nobility status, etc. and that appears at the start of the name.protected List
<StringType> Part of the name that is acquired as a title due to academic, legal, employment or nobility status, etc. and that appears at the end of the name.protected StringType
A full text representation of the name.protected Enumeration
<HumanName.NameUse> Identifies the purpose for this name. -
Constructor Summary
Constructors -
Method Summary
Modifier and TypeMethodDescriptioncopy()
boolean
equalsDeep
(Base other_) boolean
equalsShallow
(Base other_) fhirType()
getGiven()
/** Returns all repetitions ofgiven name
as a space separated stringReturns thetext
element value if it is not null.getNamedProperty
(int _hash, String _name, boolean _checkValid) Returns all repetitions ofprefix name
as a space separated stringBase[]
getProperty
(int hash, String name, boolean checkValid) Returns all repetitions ofsuffix
as a space separated stringgetText()
String[]
getTypesForProperty
(int hash, String name) getUse()
boolean
boolean
boolean
hasGiven()
boolean
boolean
boolean
boolean
boolean
boolean
boolean
hasText()
boolean
boolean
hasUse()
boolean
boolean
isEmpty()
protected void
listChildren
(List<Property> children) makeProperty
(int hash, String name) setFamilyElement
(StringType value) setGiven
(List<StringType> theGiven) setPrefix
(List<StringType> thePrefix) setProperty
(int hash, String name, Base value) setProperty
(String name, Base value) setSuffix
(List<StringType> theSuffix) setTextElement
(StringType value) setUse
(HumanName.NameUse value) protected HumanName
Methods inherited from class org.hl7.fhir.dstu3.model.Element
addExtension, addExtension, addExtension, copyValues, getExtension, getExtensionByUrl, getExtensionFirstRep, getExtensionsByUrl, getExtensionString, getId, getIdBase, getIdElement, hasExtension, hasExtension, hasId, hasIdElement, setExtension, setId, setIdBase, setIdElement
Methods inherited from class org.hl7.fhir.dstu3.model.Base
castToAddress, castToAnnotation, castToAttachment, castToBase64Binary, castToBoolean, castToCode, castToCodeableConcept, castToCoding, castToContactDetail, castToContactPoint, castToContributor, castToDataRequirement, castToDate, castToDateTime, castToDecimal, castToDosage, castToDuration, castToElementDefinition, castToExtension, castToHumanName, castToId, castToIdentifier, castToInstant, castToInteger, castToMarkdown, castToMeta, castToMoney, castToNarrative, castToOid, castToParameterDefinition, castToPeriod, castToPositiveInt, castToQuantity, castToRange, castToRatio, castToReference, castToRelatedArtifact, castToResource, castToSampledData, castToSignature, castToSimpleQuantity, castToString, castToTime, castToTiming, castToTriggerDefinition, castToType, castToUnsignedInt, castToUri, castToUsageContext, castToXhtml, castToXhtmlString, children, clearUserData, compareDeep, compareDeep, compareDeep, compareValues, compareValues, equals, getChildByName, getFormatCommentsPost, getFormatCommentsPre, getNamedProperty, getUserData, getUserInt, getUserString, hasFormatComment, hasPrimitiveValue, hasType, hasUserData, isBooleanPrimitive, isMetadataBased, isPrimitive, isResource, listChildrenByName, listChildrenByName, primitiveValue, setUserData, setUserDataINN
Methods inherited from class java.lang.Object
clone, equals, finalize, getClass, hashCode, notify, notifyAll, toString, wait, wait, wait
Methods inherited from interface org.hl7.fhir.instance.model.api.IBase
getFormatCommentsPost, getFormatCommentsPre, getUserData, hasFormatComment, setUserData
Methods inherited from interface org.hl7.fhir.instance.model.api.IBaseElement
getUserData, setUserData
-
Field Details
-
use
Identifies the purpose for this name. -
text
A full text representation of the name. -
family
The part of a name that links to the genealogy. In some cultures (e.g. Eritrea) the family name of a son is the first name of his father. -
given
Given name. -
prefix
Part of the name that is acquired as a title due to academic, legal, employment or nobility status, etc. and that appears at the start of the name. -
suffix
Part of the name that is acquired as a title due to academic, legal, employment or nobility status, etc. and that appears at the end of the name. -
period
Indicates the period of time when this name was valid for the named person.
-
-
Constructor Details
-
HumanName
public HumanName()Constructor
-
-
Method Details
-
getUseElement
- Returns:
use
(Identifies the purpose for this name.). This is the underlying object with id, value and extensions. The accessor "getUse" gives direct access to the value
-
hasUseElement
-
hasUse
-
setUseElement
- Parameters:
value
-use
(Identifies the purpose for this name.). This is the underlying object with id, value and extensions. The accessor "getUse" gives direct access to the value
-
getUse
- Returns:
- Identifies the purpose for this name.
-
setUse
- Parameters:
value
- Identifies the purpose for this name.
-
getTextElement
- Returns:
text
(A full text representation of the name.). This is the underlying object with id, value and extensions. The accessor "getText" gives direct access to the value
-
hasTextElement
-
hasText
-
setTextElement
- Parameters:
value
-text
(A full text representation of the name.). This is the underlying object with id, value and extensions. The accessor "getText" gives direct access to the value
-
getText
- Returns:
- A full text representation of the name.
-
setText
- Parameters:
value
- A full text representation of the name.
-
getFamilyElement
- Returns:
family
(The part of a name that links to the genealogy. In some cultures (e.g. Eritrea) the family name of a son is the first name of his father.). This is the underlying object with id, value and extensions. The accessor "getFamily" gives direct access to the value
-
hasFamilyElement
-
hasFamily
-
setFamilyElement
- Parameters:
value
-family
(The part of a name that links to the genealogy. In some cultures (e.g. Eritrea) the family name of a son is the first name of his father.). This is the underlying object with id, value and extensions. The accessor "getFamily" gives direct access to the value
-
getFamily
- Returns:
- The part of a name that links to the genealogy. In some cultures (e.g. Eritrea) the family name of a son is the first name of his father.
-
setFamily
- Parameters:
value
- The part of a name that links to the genealogy. In some cultures (e.g. Eritrea) the family name of a son is the first name of his father.
-
getGiven
- Returns:
given
(Given name.)
-
setGiven
- Returns:
- Returns a reference to
this
for easy method chaining
-
hasGiven
-
addGivenElement
- Returns:
given
(Given name.)
-
addGiven
- Parameters:
value
-given
(Given name.)
-
hasGiven
- Parameters:
value
-given
(Given name.)
-
getPrefix
- Returns:
prefix
(Part of the name that is acquired as a title due to academic, legal, employment or nobility status, etc. and that appears at the start of the name.)
-
setPrefix
- Returns:
- Returns a reference to
this
for easy method chaining
-
hasPrefix
-
addPrefixElement
- Returns:
prefix
(Part of the name that is acquired as a title due to academic, legal, employment or nobility status, etc. and that appears at the start of the name.)
-
addPrefix
- Parameters:
value
-prefix
(Part of the name that is acquired as a title due to academic, legal, employment or nobility status, etc. and that appears at the start of the name.)
-
hasPrefix
- Parameters:
value
-prefix
(Part of the name that is acquired as a title due to academic, legal, employment or nobility status, etc. and that appears at the start of the name.)
-
getSuffix
- Returns:
suffix
(Part of the name that is acquired as a title due to academic, legal, employment or nobility status, etc. and that appears at the end of the name.)
-
setSuffix
- Returns:
- Returns a reference to
this
for easy method chaining
-
hasSuffix
-
addSuffixElement
- Returns:
suffix
(Part of the name that is acquired as a title due to academic, legal, employment or nobility status, etc. and that appears at the end of the name.)
-
addSuffix
- Parameters:
value
-suffix
(Part of the name that is acquired as a title due to academic, legal, employment or nobility status, etc. and that appears at the end of the name.)
-
hasSuffix
- Parameters:
value
-suffix
(Part of the name that is acquired as a title due to academic, legal, employment or nobility status, etc. and that appears at the end of the name.)
-
getPeriod
- Returns:
period
(Indicates the period of time when this name was valid for the named person.)
-
hasPeriod
-
setPeriod
- Parameters:
value
-period
(Indicates the period of time when this name was valid for the named person.)
-
getGivenAsSingleString
/** Returns all repetitions ofgiven name
as a space separated string- See Also:
-
getPrefixAsSingleString
Returns all repetitions ofprefix name
as a space separated string- See Also:
-
getSuffixAsSingleString
Returns all repetitions ofsuffix
as a space separated string- See Also:
-
getNameAsSingleString
Returns the
text
element value if it is not null.If the
text
element value is null, returns all the components of the name (prefix, given, family, suffix) as a single string with a single spaced string separating each part.- Returns:
- the human name as a single string
-
listChildren
- Overrides:
listChildren
in classElement
-
getNamedProperty
public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws org.hl7.fhir.exceptions.FHIRException - Overrides:
getNamedProperty
in classElement
- Throws:
org.hl7.fhir.exceptions.FHIRException
-
getProperty
public Base[] getProperty(int hash, String name, boolean checkValid) throws org.hl7.fhir.exceptions.FHIRException - Overrides:
getProperty
in classElement
- Throws:
org.hl7.fhir.exceptions.FHIRException
-
setProperty
public Base setProperty(int hash, String name, Base value) throws org.hl7.fhir.exceptions.FHIRException - Overrides:
setProperty
in classElement
- Throws:
org.hl7.fhir.exceptions.FHIRException
-
setProperty
- Overrides:
setProperty
in classElement
- Throws:
org.hl7.fhir.exceptions.FHIRException
-
makeProperty
- Overrides:
makeProperty
in classElement
- Throws:
org.hl7.fhir.exceptions.FHIRException
-
getTypesForProperty
public String[] getTypesForProperty(int hash, String name) throws org.hl7.fhir.exceptions.FHIRException - Overrides:
getTypesForProperty
in classElement
- Throws:
org.hl7.fhir.exceptions.FHIRException
-
addChild
-
fhirType
-
copy
-
typedCopy
-
equalsDeep
- Overrides:
equalsDeep
in classElement
-
equalsShallow
- Overrides:
equalsShallow
in classElement
-
isEmpty
-