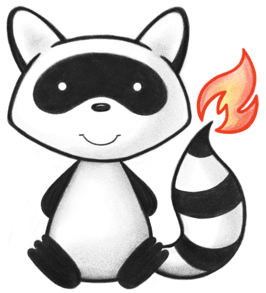
Package org.hl7.fhir.dstu3.model
Class MedicationAdministration.MedicationAdministrationDosageComponent
java.lang.Object
org.hl7.fhir.dstu3.model.Base
org.hl7.fhir.dstu3.model.Element
org.hl7.fhir.dstu3.model.BackboneElement
org.hl7.fhir.dstu3.model.MedicationAdministration.MedicationAdministrationDosageComponent
- All Implemented Interfaces:
ca.uhn.fhir.model.api.IElement
,Serializable
,org.hl7.fhir.instance.model.api.IBase
,org.hl7.fhir.instance.model.api.IBaseBackboneElement
,org.hl7.fhir.instance.model.api.IBaseElement
,org.hl7.fhir.instance.model.api.IBaseHasExtensions
,org.hl7.fhir.instance.model.api.IBaseHasModifierExtensions
- Enclosing class:
MedicationAdministration
public static class MedicationAdministration.MedicationAdministrationDosageComponent
extends BackboneElement
implements org.hl7.fhir.instance.model.api.IBaseBackboneElement
- See Also:
-
Field Summary
FieldsModifier and TypeFieldDescriptionprotected SimpleQuantity
The amount of the medication given at one administration event.protected CodeableConcept
A coded value indicating the method by which the medication is intended to be or was introduced into or on the body.protected Type
Identifies the speed with which the medication was or will be introduced into the patient.protected CodeableConcept
A code specifying the route or physiological path of administration of a therapeutic agent into or onto the patient.protected CodeableConcept
A coded specification of the anatomic site where the medication first entered the body.protected StringType
Free text dosage can be used for cases where the dosage administered is too complex to code.Fields inherited from class org.hl7.fhir.dstu3.model.BackboneElement
modifierExtension
-
Constructor Summary
Constructors -
Method Summary
Modifier and TypeMethodDescriptioncopy()
boolean
equalsDeep
(Base other_) boolean
equalsShallow
(Base other_) fhirType()
getDose()
getNamedProperty
(int _hash, String _name, boolean _checkValid) Base[]
getProperty
(int hash, String name, boolean checkValid) getRate()
getRoute()
getSite()
getText()
String[]
getTypesForProperty
(int hash, String name) boolean
hasDose()
boolean
boolean
hasRate()
boolean
boolean
boolean
hasRoute()
boolean
hasSite()
boolean
hasText()
boolean
boolean
isEmpty()
protected void
listChildren
(List<Property> children) makeProperty
(int hash, String name) setDose
(SimpleQuantity value) setMethod
(CodeableConcept value) setProperty
(int hash, String name, Base value) setProperty
(String name, Base value) setRoute
(CodeableConcept value) setSite
(CodeableConcept value) setTextElement
(StringType value) Methods inherited from class org.hl7.fhir.dstu3.model.BackboneElement
addModifierExtension, addModifierExtension, copyValues, getModifierExtension, getModifierExtensionFirstRep, hasModifierExtension, setModifierExtension
Methods inherited from class org.hl7.fhir.dstu3.model.Element
addExtension, addExtension, addExtension, copyValues, getExtension, getExtensionByUrl, getExtensionFirstRep, getExtensionsByUrl, getExtensionString, getId, getIdBase, getIdElement, hasExtension, hasExtension, hasId, hasIdElement, setExtension, setId, setIdBase, setIdElement
Methods inherited from class org.hl7.fhir.dstu3.model.Base
castToAddress, castToAnnotation, castToAttachment, castToBase64Binary, castToBoolean, castToCode, castToCodeableConcept, castToCoding, castToContactDetail, castToContactPoint, castToContributor, castToDataRequirement, castToDate, castToDateTime, castToDecimal, castToDosage, castToDuration, castToElementDefinition, castToExtension, castToHumanName, castToId, castToIdentifier, castToInstant, castToInteger, castToMarkdown, castToMeta, castToMoney, castToNarrative, castToOid, castToParameterDefinition, castToPeriod, castToPositiveInt, castToQuantity, castToRange, castToRatio, castToReference, castToRelatedArtifact, castToResource, castToSampledData, castToSignature, castToSimpleQuantity, castToString, castToTime, castToTiming, castToTriggerDefinition, castToType, castToUnsignedInt, castToUri, castToUsageContext, castToXhtml, castToXhtmlString, children, clearUserData, compareDeep, compareDeep, compareDeep, compareValues, compareValues, equals, getChildByName, getFormatCommentsPost, getFormatCommentsPre, getNamedProperty, getUserData, getUserInt, getUserString, hasFormatComment, hasPrimitiveValue, hasType, hasUserData, isBooleanPrimitive, isMetadataBased, isPrimitive, isResource, listChildrenByName, listChildrenByName, primitiveValue, setUserData, setUserDataINN
Methods inherited from class java.lang.Object
clone, equals, finalize, getClass, hashCode, notify, notifyAll, toString, wait, wait, wait
Methods inherited from interface org.hl7.fhir.instance.model.api.IBase
getFormatCommentsPost, getFormatCommentsPre, getUserData, hasFormatComment, setUserData
Methods inherited from interface org.hl7.fhir.instance.model.api.IBaseElement
getUserData, setUserData
Methods inherited from interface org.hl7.fhir.instance.model.api.IBaseHasExtensions
addExtension, getExtension, hasExtension
Methods inherited from interface org.hl7.fhir.instance.model.api.IBaseHasModifierExtensions
addModifierExtension, getModifierExtension, hasModifierExtension
-
Field Details
-
text
Free text dosage can be used for cases where the dosage administered is too complex to code. When coded dosage is present, the free text dosage may still be present for display to humans. The dosage instructions should reflect the dosage of the medication that was administered. -
site
A coded specification of the anatomic site where the medication first entered the body. For example, "left arm". -
route
A code specifying the route or physiological path of administration of a therapeutic agent into or onto the patient. For example, topical, intravenous, etc. -
method
A coded value indicating the method by which the medication is intended to be or was introduced into or on the body. This attribute will most often NOT be populated. It is most commonly used for injections. For example, Slow Push, Deep IV. -
dose
The amount of the medication given at one administration event. Use this value when the administration is essentially an instantaneous event such as a swallowing a tablet or giving an injection. -
rate
Identifies the speed with which the medication was or will be introduced into the patient. Typically the rate for an infusion e.g. 100 ml per 1 hour or 100 ml/hr. May also be expressed as a rate per unit of time e.g. 500 ml per 2 hours. Other examples: 200 mcg/min or 200 mcg/1 minute; 1 liter/8 hours.
-
-
Constructor Details
-
MedicationAdministrationDosageComponent
Constructor
-
-
Method Details
-
getTextElement
- Returns:
text
(Free text dosage can be used for cases where the dosage administered is too complex to code. When coded dosage is present, the free text dosage may still be present for display to humans. The dosage instructions should reflect the dosage of the medication that was administered.). This is the underlying object with id, value and extensions. The accessor "getText" gives direct access to the value
-
hasTextElement
-
hasText
-
setTextElement
public MedicationAdministration.MedicationAdministrationDosageComponent setTextElement(StringType value) - Parameters:
value
-text
(Free text dosage can be used for cases where the dosage administered is too complex to code. When coded dosage is present, the free text dosage may still be present for display to humans. The dosage instructions should reflect the dosage of the medication that was administered.). This is the underlying object with id, value and extensions. The accessor "getText" gives direct access to the value
-
getText
- Returns:
- Free text dosage can be used for cases where the dosage administered is too complex to code. When coded dosage is present, the free text dosage may still be present for display to humans. The dosage instructions should reflect the dosage of the medication that was administered.
-
setText
- Parameters:
value
- Free text dosage can be used for cases where the dosage administered is too complex to code. When coded dosage is present, the free text dosage may still be present for display to humans. The dosage instructions should reflect the dosage of the medication that was administered.
-
getSite
- Returns:
site
(A coded specification of the anatomic site where the medication first entered the body. For example, "left arm".)
-
hasSite
-
setSite
public MedicationAdministration.MedicationAdministrationDosageComponent setSite(CodeableConcept value) - Parameters:
value
-site
(A coded specification of the anatomic site where the medication first entered the body. For example, "left arm".)
-
getRoute
- Returns:
route
(A code specifying the route or physiological path of administration of a therapeutic agent into or onto the patient. For example, topical, intravenous, etc.)
-
hasRoute
-
setRoute
public MedicationAdministration.MedicationAdministrationDosageComponent setRoute(CodeableConcept value) - Parameters:
value
-route
(A code specifying the route or physiological path of administration of a therapeutic agent into or onto the patient. For example, topical, intravenous, etc.)
-
getMethod
- Returns:
method
(A coded value indicating the method by which the medication is intended to be or was introduced into or on the body. This attribute will most often NOT be populated. It is most commonly used for injections. For example, Slow Push, Deep IV.)
-
hasMethod
-
setMethod
public MedicationAdministration.MedicationAdministrationDosageComponent setMethod(CodeableConcept value) - Parameters:
value
-method
(A coded value indicating the method by which the medication is intended to be or was introduced into or on the body. This attribute will most often NOT be populated. It is most commonly used for injections. For example, Slow Push, Deep IV.)
-
getDose
- Returns:
dose
(The amount of the medication given at one administration event. Use this value when the administration is essentially an instantaneous event such as a swallowing a tablet or giving an injection.)
-
hasDose
-
setDose
public MedicationAdministration.MedicationAdministrationDosageComponent setDose(SimpleQuantity value) - Parameters:
value
-dose
(The amount of the medication given at one administration event. Use this value when the administration is essentially an instantaneous event such as a swallowing a tablet or giving an injection.)
-
getRate
- Returns:
rate
(Identifies the speed with which the medication was or will be introduced into the patient. Typically the rate for an infusion e.g. 100 ml per 1 hour or 100 ml/hr. May also be expressed as a rate per unit of time e.g. 500 ml per 2 hours. Other examples: 200 mcg/min or 200 mcg/1 minute; 1 liter/8 hours.)
-
getRateRatio
- Returns:
rate
(Identifies the speed with which the medication was or will be introduced into the patient. Typically the rate for an infusion e.g. 100 ml per 1 hour or 100 ml/hr. May also be expressed as a rate per unit of time e.g. 500 ml per 2 hours. Other examples: 200 mcg/min or 200 mcg/1 minute; 1 liter/8 hours.)- Throws:
org.hl7.fhir.exceptions.FHIRException
-
hasRateRatio
-
getRateSimpleQuantity
- Returns:
rate
(Identifies the speed with which the medication was or will be introduced into the patient. Typically the rate for an infusion e.g. 100 ml per 1 hour or 100 ml/hr. May also be expressed as a rate per unit of time e.g. 500 ml per 2 hours. Other examples: 200 mcg/min or 200 mcg/1 minute; 1 liter/8 hours.)- Throws:
org.hl7.fhir.exceptions.FHIRException
-
hasRateSimpleQuantity
-
hasRate
-
setRate
public MedicationAdministration.MedicationAdministrationDosageComponent setRate(Type value) throws org.hl7.fhir.exceptions.FHIRFormatError - Parameters:
value
-rate
(Identifies the speed with which the medication was or will be introduced into the patient. Typically the rate for an infusion e.g. 100 ml per 1 hour or 100 ml/hr. May also be expressed as a rate per unit of time e.g. 500 ml per 2 hours. Other examples: 200 mcg/min or 200 mcg/1 minute; 1 liter/8 hours.)- Throws:
org.hl7.fhir.exceptions.FHIRFormatError
-
listChildren
- Overrides:
listChildren
in classBackboneElement
-
getNamedProperty
public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws org.hl7.fhir.exceptions.FHIRException - Overrides:
getNamedProperty
in classBackboneElement
- Throws:
org.hl7.fhir.exceptions.FHIRException
-
getProperty
public Base[] getProperty(int hash, String name, boolean checkValid) throws org.hl7.fhir.exceptions.FHIRException - Overrides:
getProperty
in classBackboneElement
- Throws:
org.hl7.fhir.exceptions.FHIRException
-
setProperty
public Base setProperty(int hash, String name, Base value) throws org.hl7.fhir.exceptions.FHIRException - Overrides:
setProperty
in classBackboneElement
- Throws:
org.hl7.fhir.exceptions.FHIRException
-
setProperty
- Overrides:
setProperty
in classBackboneElement
- Throws:
org.hl7.fhir.exceptions.FHIRException
-
makeProperty
- Overrides:
makeProperty
in classBackboneElement
- Throws:
org.hl7.fhir.exceptions.FHIRException
-
getTypesForProperty
public String[] getTypesForProperty(int hash, String name) throws org.hl7.fhir.exceptions.FHIRException - Overrides:
getTypesForProperty
in classBackboneElement
- Throws:
org.hl7.fhir.exceptions.FHIRException
-
addChild
- Overrides:
addChild
in classBackboneElement
- Throws:
org.hl7.fhir.exceptions.FHIRException
-
copy
- Specified by:
copy
in classBackboneElement
-
equalsDeep
- Overrides:
equalsDeep
in classBackboneElement
-
equalsShallow
- Overrides:
equalsShallow
in classBackboneElement
-
isEmpty
- Specified by:
isEmpty
in interfaceorg.hl7.fhir.instance.model.api.IBase
- Overrides:
isEmpty
in classBackboneElement
-
fhirType
- Specified by:
fhirType
in interfaceorg.hl7.fhir.instance.model.api.IBase
- Overrides:
fhirType
in classBackboneElement
-