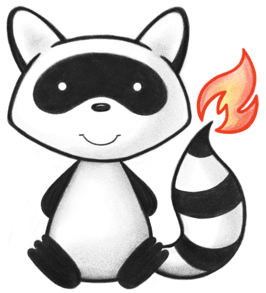
Package org.hl7.fhir.dstu2.model
Class DetectedIssue
java.lang.Object
org.hl7.fhir.dstu2.model.Base
org.hl7.fhir.dstu2.model.BaseResource
org.hl7.fhir.dstu2.model.Resource
org.hl7.fhir.dstu2.model.DomainResource
org.hl7.fhir.dstu2.model.DetectedIssue
- All Implemented Interfaces:
ca.uhn.fhir.model.api.IElement
,Serializable
,org.hl7.fhir.instance.model.api.IAnyResource
,org.hl7.fhir.instance.model.api.IBase
,org.hl7.fhir.instance.model.api.IBaseHasExtensions
,org.hl7.fhir.instance.model.api.IBaseHasModifierExtensions
,org.hl7.fhir.instance.model.api.IBaseResource
,org.hl7.fhir.instance.model.api.IDomainResource
Indicates an actual or potential clinical issue with or between one or more
active or proposed clinical actions for a patient; e.g. Drug-drug
interaction, Ineffective treatment frequency, Procedure-condition conflict,
etc.
- See Also:
-
Nested Class Summary
Nested ClassesModifier and TypeClassDescriptionstatic class
static enum
static class
-
Field Summary
FieldsModifier and TypeFieldDescriptionprotected Reference
Individual or device responsible for the issue being raised.protected Resource
The actual object that is the target of the reference (Individual or device responsible for the issue being raised.protected CodeableConcept
Identifies the general type of issue identified.protected DateTimeType
The date or date-time when the detected issue was initially identified.protected StringType
A textual explanation of the detected issue.protected Identifier
Business identifier associated with the detected issue record.Indicates the resource representing the current activity or proposed activity that is potentially problematic.The actual objects that are the target of the reference (Indicates the resource representing the current activity or proposed activity that is potentially problematic.)Indicates an action that has been taken or is committed to to reduce or eliminate the likelihood of the risk identified by the detected issue from manifesting.protected Reference
Indicates the patient whose record the detected issue is associated with.protected Patient
The actual object that is the target of the reference (Indicates the patient whose record the detected issue is associated with.)protected UriType
The literature, knowledge-base or similar reference that describes the propensity for the detected issue identified.protected Enumeration
<DetectedIssue.DetectedIssueSeverity> Indicates the degree of importance associated with the identified issue based on the potential impact on the patient.static final String
static final String
static final String
static final String
static final String
static final String
Fields inherited from class org.hl7.fhir.dstu2.model.DomainResource
contained, extension, modifierExtension, text
Fields inherited from class org.hl7.fhir.dstu2.model.Resource
id, implicitRules, language, meta
Fields inherited from interface org.hl7.fhir.instance.model.api.IAnyResource
RES_ID, RES_LAST_UPDATED, RES_PROFILE, RES_SECURITY, RES_TAG, SP_RES_ID, SP_RES_LAST_UPDATED, SP_RES_PROFILE, SP_RES_SECURITY, SP_RES_TAG
Fields inherited from interface org.hl7.fhir.instance.model.api.IBaseResource
INCLUDE_ALL, WILDCARD_ALL_SET
-
Constructor Summary
Constructors -
Method Summary
Modifier and TypeMethodDescriptioncopy()
boolean
equalsDeep
(Base other) boolean
equalsShallow
(Base other) fhirType()
getDate()
boolean
boolean
boolean
hasDate()
boolean
boolean
boolean
boolean
boolean
boolean
boolean
boolean
boolean
boolean
boolean
boolean
isEmpty()
protected void
listChildren
(List<Property> childrenList) setAuthorTarget
(Resource value) setCategory
(CodeableConcept value) setDateElement
(DateTimeType value) setDetailElement
(StringType value) setIdentifier
(Identifier value) setPatient
(Reference value) setPatientTarget
(Patient value) void
setProperty
(String name, Base value) setReference
(String value) setReferenceElement
(UriType value) protected DetectedIssue
Methods inherited from class org.hl7.fhir.dstu2.model.DomainResource
addContained, addExtension, addExtension, addModifierExtension, addModifierExtension, copyValues, getContained, getExtension, getExtensionByUrl, getModifierExtension, getText, hasContained, hasExtension, hasExtension, hasModifierExtension, hasText, setText
Methods inherited from class org.hl7.fhir.dstu2.model.Resource
copyValues, getId, getIdElement, getImplicitRules, getImplicitRulesElement, getLanguage, getLanguageElement, getMeta, hasId, hasIdElement, hasImplicitRules, hasImplicitRulesElement, hasLanguage, hasLanguageElement, hasMeta, setId, setIdElement, setImplicitRules, setImplicitRulesElement, setLanguage, setLanguageElement, setMeta
Methods inherited from class org.hl7.fhir.dstu2.model.BaseResource
getStructureFhirVersionEnum, setId
Methods inherited from class org.hl7.fhir.dstu2.model.Base
castToAddress, castToAnnotation, castToAttachment, castToBase64Binary, castToBoolean, castToCode, castToCodeableConcept, castToCoding, castToContactPoint, castToDate, castToDateTime, castToDecimal, castToDuration, castToElementDefinition, castToExtension, castToHumanName, castToId, castToIdentifier, castToInstant, castToInteger, castToMarkdown, castToMeta, castToMoney, castToNarrative, castToOid, castToPeriod, castToPositiveInt, castToQuantity, castToRange, castToRatio, castToReference, castToResource, castToSampledData, castToSignature, castToSimpleQuantity, castToString, castToTime, castToTiming, castToUnsignedInt, castToUri, children, compareDeep, compareDeep, compareDeep, compareValues, compareValues, equals, getChildByName, getFormatCommentsPost, getFormatCommentsPre, getUserData, getUserInt, getUserString, hasFormatComment, hasType, hasUserData, isMetadataBased, isPrimitive, listChildrenByName, primitiveValue, setUserData, setUserDataINN
Methods inherited from class java.lang.Object
clone, equals, finalize, getClass, hashCode, notify, notifyAll, toString, wait, wait, wait
Methods inherited from interface org.hl7.fhir.instance.model.api.IAnyResource
getId, getIdElement, getLanguageElement, getUserData, setId, setUserData
Methods inherited from interface org.hl7.fhir.instance.model.api.IBase
getFormatCommentsPost, getFormatCommentsPre, hasFormatComment
Methods inherited from interface org.hl7.fhir.instance.model.api.IBaseResource
getMeta, getStructureFhirVersionEnum, isDeleted, setId
-
Field Details
-
patient
Indicates the patient whose record the detected issue is associated with. -
patientTarget
The actual object that is the target of the reference (Indicates the patient whose record the detected issue is associated with.) -
category
Identifies the general type of issue identified. -
severity
Indicates the degree of importance associated with the identified issue based on the potential impact on the patient. -
implicated
Indicates the resource representing the current activity or proposed activity that is potentially problematic. -
implicatedTarget
The actual objects that are the target of the reference (Indicates the resource representing the current activity or proposed activity that is potentially problematic.) -
detail
A textual explanation of the detected issue. -
date
The date or date-time when the detected issue was initially identified. -
author
Individual or device responsible for the issue being raised. For example, a decision support application or a pharmacist conducting a medication review. -
authorTarget
The actual object that is the target of the reference (Individual or device responsible for the issue being raised. For example, a decision support application or a pharmacist conducting a medication review.) -
identifier
Business identifier associated with the detected issue record. -
reference
The literature, knowledge-base or similar reference that describes the propensity for the detected issue identified. -
mitigation
Indicates an action that has been taken or is committed to to reduce or eliminate the likelihood of the risk identified by the detected issue from manifesting. Can also reflect an observation of known mitigating factors that may reduce/eliminate the need for any action. -
SP_DATE
- See Also:
-
SP_IDENTIFIER
- See Also:
-
SP_PATIENT
- See Also:
-
SP_AUTHOR
- See Also:
-
SP_IMPLICATED
- See Also:
-
SP_CATEGORY
- See Also:
-
-
Constructor Details
-
DetectedIssue
public DetectedIssue()
-
-
Method Details
-
getPatient
- Returns:
patient
(Indicates the patient whose record the detected issue is associated with.)
-
hasPatient
-
setPatient
- Parameters:
value
-patient
(Indicates the patient whose record the detected issue is associated with.)
-
getPatientTarget
- Returns:
patient
The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Indicates the patient whose record the detected issue is associated with.)
-
setPatientTarget
- Parameters:
value
-patient
The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Indicates the patient whose record the detected issue is associated with.)
-
getCategory
- Returns:
category
(Identifies the general type of issue identified.)
-
hasCategory
-
setCategory
- Parameters:
value
-category
(Identifies the general type of issue identified.)
-
getSeverityElement
- Returns:
severity
(Indicates the degree of importance associated with the identified issue based on the potential impact on the patient.). This is the underlying object with id, value and extensions. The accessor "getSeverity" gives direct access to the value
-
hasSeverityElement
-
hasSeverity
-
setSeverityElement
- Parameters:
value
-severity
(Indicates the degree of importance associated with the identified issue based on the potential impact on the patient.). This is the underlying object with id, value and extensions. The accessor "getSeverity" gives direct access to the value
-
getSeverity
- Returns:
- Indicates the degree of importance associated with the identified issue based on the potential impact on the patient.
-
setSeverity
- Parameters:
value
- Indicates the degree of importance associated with the identified issue based on the potential impact on the patient.
-
getImplicated
- Returns:
implicated
(Indicates the resource representing the current activity or proposed activity that is potentially problematic.)
-
hasImplicated
-
addImplicated
- Returns:
implicated
(Indicates the resource representing the current activity or proposed activity that is potentially problematic.)
-
addImplicated
-
getImplicatedTarget
- Returns:
implicated
(The actual objects that are the target of the reference. The reference library doesn't populate this, but you can use this to hold the resources if you resolvethemt. Indicates the resource representing the current activity or proposed activity that is potentially problematic.)
-
getDetailElement
- Returns:
detail
(A textual explanation of the detected issue.). This is the underlying object with id, value and extensions. The accessor "getDetail" gives direct access to the value
-
hasDetailElement
-
hasDetail
-
setDetailElement
- Parameters:
value
-detail
(A textual explanation of the detected issue.). This is the underlying object with id, value and extensions. The accessor "getDetail" gives direct access to the value
-
getDetail
- Returns:
- A textual explanation of the detected issue.
-
setDetail
- Parameters:
value
- A textual explanation of the detected issue.
-
getDateElement
- Returns:
date
(The date or date-time when the detected issue was initially identified.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value
-
hasDateElement
-
hasDate
-
setDateElement
- Parameters:
value
-date
(The date or date-time when the detected issue was initially identified.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value
-
getDate
- Returns:
- The date or date-time when the detected issue was initially identified.
-
setDate
- Parameters:
value
- The date or date-time when the detected issue was initially identified.
-
getAuthor
- Returns:
author
(Individual or device responsible for the issue being raised. For example, a decision support application or a pharmacist conducting a medication review.)
-
hasAuthor
-
setAuthor
- Parameters:
value
-author
(Individual or device responsible for the issue being raised. For example, a decision support application or a pharmacist conducting a medication review.)
-
getAuthorTarget
- Returns:
author
The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Individual or device responsible for the issue being raised. For example, a decision support application or a pharmacist conducting a medication review.)
-
setAuthorTarget
- Parameters:
value
-author
The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Individual or device responsible for the issue being raised. For example, a decision support application or a pharmacist conducting a medication review.)
-
getIdentifier
- Returns:
identifier
(Business identifier associated with the detected issue record.)
-
hasIdentifier
-
setIdentifier
- Parameters:
value
-identifier
(Business identifier associated with the detected issue record.)
-
getReferenceElement
- Returns:
reference
(The literature, knowledge-base or similar reference that describes the propensity for the detected issue identified.). This is the underlying object with id, value and extensions. The accessor "getReference" gives direct access to the value
-
hasReferenceElement
-
hasReference
-
setReferenceElement
- Parameters:
value
-reference
(The literature, knowledge-base or similar reference that describes the propensity for the detected issue identified.). This is the underlying object with id, value and extensions. The accessor "getReference" gives direct access to the value
-
getReference
- Returns:
- The literature, knowledge-base or similar reference that describes the propensity for the detected issue identified.
-
setReference
- Parameters:
value
- The literature, knowledge-base or similar reference that describes the propensity for the detected issue identified.
-
getMitigation
- Returns:
mitigation
(Indicates an action that has been taken or is committed to to reduce or eliminate the likelihood of the risk identified by the detected issue from manifesting. Can also reflect an observation of known mitigating factors that may reduce/eliminate the need for any action.)
-
hasMitigation
-
addMitigation
- Returns:
mitigation
(Indicates an action that has been taken or is committed to to reduce or eliminate the likelihood of the risk identified by the detected issue from manifesting. Can also reflect an observation of known mitigating factors that may reduce/eliminate the need for any action.)
-
addMitigation
-
listChildren
- Overrides:
listChildren
in classDomainResource
-
setProperty
- Overrides:
setProperty
in classDomainResource
- Throws:
org.hl7.fhir.exceptions.FHIRException
-
addChild
- Overrides:
addChild
in classDomainResource
- Throws:
org.hl7.fhir.exceptions.FHIRException
-
fhirType
- Specified by:
fhirType
in interfaceorg.hl7.fhir.instance.model.api.IBase
- Overrides:
fhirType
in classDomainResource
-
copy
- Specified by:
copy
in classDomainResource
-
typedCopy
-
equalsDeep
- Overrides:
equalsDeep
in classDomainResource
-
equalsShallow
- Overrides:
equalsShallow
in classDomainResource
-
isEmpty
- Specified by:
isEmpty
in interfaceorg.hl7.fhir.instance.model.api.IBase
- Overrides:
isEmpty
in classDomainResource
-
getResourceType
- Specified by:
getResourceType
in classResource
-