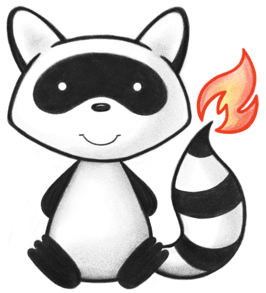
Package org.hl7.fhir.dstu2.model
Class Device
java.lang.Object
org.hl7.fhir.dstu2.model.Base
org.hl7.fhir.dstu2.model.BaseResource
org.hl7.fhir.dstu2.model.Resource
org.hl7.fhir.dstu2.model.DomainResource
org.hl7.fhir.dstu2.model.Device
- All Implemented Interfaces:
ca.uhn.fhir.model.api.IElement
,Serializable
,org.hl7.fhir.instance.model.api.IAnyResource
,org.hl7.fhir.instance.model.api.IBase
,org.hl7.fhir.instance.model.api.IBaseHasExtensions
,org.hl7.fhir.instance.model.api.IBaseHasModifierExtensions
,org.hl7.fhir.instance.model.api.IBaseResource
,org.hl7.fhir.instance.model.api.IDomainResource
This resource identifies an instance of a manufactured item that is used in
the provision of healthcare without being substantially changed through that
activity. The device may be a medical or non-medical device. Medical devices
includes durable (reusable) medical equipment, implantable devices, as well
as disposable equipment used for diagnostic, treatment, and research for
healthcare and public health. Non-medical devices may include items such as a
machine, cellphone, computer, application, etc.
- See Also:
-
Nested Class Summary
Nested ClassesModifier and TypeClassDescriptionstatic enum
static class
-
Field Summary
FieldsModifier and TypeFieldDescriptionprotected List
<ContactPoint> Contact details for an organization or a particular human that is responsible for the device.protected DateTimeType
The date and time beyond which this device is no longer valid or should not be used (if applicable).protected List
<Identifier> Unique instance identifiers assigned to a device by organizations like manufacturers or owners.protected Reference
The place where the device can be found.protected Location
The actual object that is the target of the reference (The place where the device can be found.)protected StringType
Lot number assigned by the manufacturer.protected DateTimeType
The date and time when the device was manufactured.protected StringType
A name of the manufacturer.protected StringType
The "model" is an identifier assigned by the manufacturer to identify the product by its type.protected List
<Annotation> Descriptive information, usage information or implantation information that is not captured in an existing element.protected Reference
An organization that is responsible for the provision and ongoing maintenance of the device.protected Organization
The actual object that is the target of the reference (An organization that is responsible for the provision and ongoing maintenance of the device.)protected Reference
Patient information, if the resource is affixed to a person.protected Patient
The actual object that is the target of the reference (Patient information, if the resource is affixed to a person.)static final String
static final String
static final String
static final String
static final String
static final String
static final String
static final String
static final String
protected Enumeration
<Device.DeviceStatus> Status of the Device availability.protected CodeableConcept
Code or identifier to identify a kind of device.protected StringType
United States Food and Drug Administration mandated Unique Device Identifier (UDI).protected UriType
A network address on which the device may be contacted directly.protected StringType
The version of the device, if the device has multiple releases under the same model, or if the device is software or carries firmware.Fields inherited from class org.hl7.fhir.dstu2.model.DomainResource
contained, extension, modifierExtension, text
Fields inherited from class org.hl7.fhir.dstu2.model.Resource
id, implicitRules, language, meta
Fields inherited from interface org.hl7.fhir.instance.model.api.IAnyResource
RES_ID, SP_RES_ID
Fields inherited from interface org.hl7.fhir.instance.model.api.IBaseResource
INCLUDE_ALL, WILDCARD_ALL_SET
-
Constructor Summary
Constructors -
Method Summary
Modifier and TypeMethodDescriptionaddNote()
copy()
boolean
equalsDeep
(Base other) boolean
equalsShallow
(Base other) fhirType()
getModel()
getNote()
getOwner()
getType()
getUdi()
getUrl()
boolean
boolean
boolean
boolean
boolean
boolean
boolean
boolean
boolean
boolean
boolean
boolean
hasModel()
boolean
boolean
hasNote()
boolean
hasOwner()
boolean
boolean
boolean
boolean
hasType()
boolean
hasUdi()
boolean
boolean
hasUrl()
boolean
boolean
boolean
boolean
isEmpty()
protected void
listChildren
(List<Property> childrenList) setExpiryElement
(DateTimeType value) setLocation
(Reference value) setLocationTarget
(Location value) setLotNumber
(String value) setLotNumberElement
(StringType value) setManufactureDate
(Date value) setManufacturer
(String value) setManufacturerElement
(StringType value) setModelElement
(StringType value) setOwnerTarget
(Organization value) setPatient
(Reference value) setPatientTarget
(Patient value) void
setProperty
(String name, Base value) setStatus
(Device.DeviceStatus value) setType
(CodeableConcept value) setUdiElement
(StringType value) setUrlElement
(UriType value) setVersion
(String value) setVersionElement
(StringType value) protected Device
Methods inherited from class org.hl7.fhir.dstu2.model.DomainResource
addContained, addExtension, addExtension, addModifierExtension, addModifierExtension, copyValues, getContained, getExtension, getExtensionByUrl, getModifierExtension, getText, hasContained, hasExtension, hasExtension, hasModifierExtension, hasText, setText
Methods inherited from class org.hl7.fhir.dstu2.model.Resource
copyValues, getId, getIdElement, getImplicitRules, getImplicitRulesElement, getLanguage, getLanguageElement, getMeta, hasId, hasIdElement, hasImplicitRules, hasImplicitRulesElement, hasLanguage, hasLanguageElement, hasMeta, setId, setIdElement, setImplicitRules, setImplicitRulesElement, setLanguage, setLanguageElement, setMeta
Methods inherited from class org.hl7.fhir.dstu2.model.BaseResource
getStructureFhirVersionEnum, setId
Methods inherited from class org.hl7.fhir.dstu2.model.Base
castToAddress, castToAnnotation, castToAttachment, castToBase64Binary, castToBoolean, castToCode, castToCodeableConcept, castToCoding, castToContactPoint, castToDate, castToDateTime, castToDecimal, castToDuration, castToElementDefinition, castToExtension, castToHumanName, castToId, castToIdentifier, castToInstant, castToInteger, castToMarkdown, castToMeta, castToMoney, castToNarrative, castToOid, castToPeriod, castToPositiveInt, castToQuantity, castToRange, castToRatio, castToReference, castToResource, castToSampledData, castToSignature, castToSimpleQuantity, castToString, castToTime, castToTiming, castToUnsignedInt, castToUri, children, compareDeep, compareDeep, compareDeep, compareValues, compareValues, equals, getChildByName, getFormatCommentsPost, getFormatCommentsPre, getUserData, getUserInt, getUserString, hasFormatComment, hasType, hasUserData, isMetadataBased, isPrimitive, listChildrenByName, primitiveValue, setUserData, setUserDataINN
Methods inherited from class java.lang.Object
clone, equals, finalize, getClass, hashCode, notify, notifyAll, toString, wait, wait, wait
Methods inherited from interface org.hl7.fhir.instance.model.api.IAnyResource
getId, getIdElement, getLanguageElement, getUserData, setId, setUserData
Methods inherited from interface org.hl7.fhir.instance.model.api.IBase
getFormatCommentsPost, getFormatCommentsPre, hasFormatComment
Methods inherited from interface org.hl7.fhir.instance.model.api.IBaseResource
getMeta, getStructureFhirVersionEnum, isDeleted, setId
-
Field Details
-
identifier
Unique instance identifiers assigned to a device by organizations like manufacturers or owners. If the identifier identifies the type of device, Device.type should be used. -
type
Code or identifier to identify a kind of device. -
note
Descriptive information, usage information or implantation information that is not captured in an existing element. -
status
Status of the Device availability. -
manufacturer
A name of the manufacturer. -
model
The "model" is an identifier assigned by the manufacturer to identify the product by its type. This number is shared by the all devices sold as the same type. -
version
The version of the device, if the device has multiple releases under the same model, or if the device is software or carries firmware. -
manufactureDate
The date and time when the device was manufactured. -
expiry
The date and time beyond which this device is no longer valid or should not be used (if applicable). -
udi
United States Food and Drug Administration mandated Unique Device Identifier (UDI). Use the human readable information (the content that the user sees, which is sometimes different to the exact syntax represented in the barcode) - see http://www.fda.gov/MedicalDevices/DeviceRegulationandGuidance/UniqueDeviceIdentification/default.htm. -
lotNumber
Lot number assigned by the manufacturer. -
owner
An organization that is responsible for the provision and ongoing maintenance of the device. -
ownerTarget
The actual object that is the target of the reference (An organization that is responsible for the provision and ongoing maintenance of the device.) -
location
The place where the device can be found. -
locationTarget
The actual object that is the target of the reference (The place where the device can be found.) -
patient
Patient information, if the resource is affixed to a person. -
patientTarget
The actual object that is the target of the reference (Patient information, if the resource is affixed to a person.) -
contact
Contact details for an organization or a particular human that is responsible for the device. -
url
A network address on which the device may be contacted directly. -
SP_IDENTIFIER
- See Also:
-
SP_PATIENT
- See Also:
-
SP_ORGANIZATION
- See Also:
-
SP_MODEL
- See Also:
-
SP_LOCATION
- See Also:
-
SP_UDI
- See Also:
-
SP_TYPE
- See Also:
-
SP_URL
- See Also:
-
SP_MANUFACTURER
- See Also:
-
-
Constructor Details
-
Device
public Device() -
Device
-
-
Method Details
-
getIdentifier
- Returns:
identifier
(Unique instance identifiers assigned to a device by organizations like manufacturers or owners. If the identifier identifies the type of device, Device.type should be used.)
-
hasIdentifier
-
addIdentifier
- Returns:
identifier
(Unique instance identifiers assigned to a device by organizations like manufacturers or owners. If the identifier identifies the type of device, Device.type should be used.)
-
addIdentifier
-
getType
- Returns:
type
(Code or identifier to identify a kind of device.)
-
hasType
-
setType
- Parameters:
value
-type
(Code or identifier to identify a kind of device.)
-
getNote
- Returns:
note
(Descriptive information, usage information or implantation information that is not captured in an existing element.)
-
hasNote
-
addNote
- Returns:
note
(Descriptive information, usage information or implantation information that is not captured in an existing element.)
-
addNote
-
getStatusElement
- Returns:
status
(Status of the Device availability.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value
-
hasStatusElement
-
hasStatus
-
setStatusElement
- Parameters:
value
-status
(Status of the Device availability.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value
-
getStatus
- Returns:
- Status of the Device availability.
-
setStatus
- Parameters:
value
- Status of the Device availability.
-
getManufacturerElement
- Returns:
manufacturer
(A name of the manufacturer.). This is the underlying object with id, value and extensions. The accessor "getManufacturer" gives direct access to the value
-
hasManufacturerElement
-
hasManufacturer
-
setManufacturerElement
- Parameters:
value
-manufacturer
(A name of the manufacturer.). This is the underlying object with id, value and extensions. The accessor "getManufacturer" gives direct access to the value
-
getManufacturer
- Returns:
- A name of the manufacturer.
-
setManufacturer
- Parameters:
value
- A name of the manufacturer.
-
getModelElement
- Returns:
model
(The "model" is an identifier assigned by the manufacturer to identify the product by its type. This number is shared by the all devices sold as the same type.). This is the underlying object with id, value and extensions. The accessor "getModel" gives direct access to the value
-
hasModelElement
-
hasModel
-
setModelElement
- Parameters:
value
-model
(The "model" is an identifier assigned by the manufacturer to identify the product by its type. This number is shared by the all devices sold as the same type.). This is the underlying object with id, value and extensions. The accessor "getModel" gives direct access to the value
-
getModel
- Returns:
- The "model" is an identifier assigned by the manufacturer to identify the product by its type. This number is shared by the all devices sold as the same type.
-
setModel
- Parameters:
value
- The "model" is an identifier assigned by the manufacturer to identify the product by its type. This number is shared by the all devices sold as the same type.
-
getVersionElement
- Returns:
version
(The version of the device, if the device has multiple releases under the same model, or if the device is software or carries firmware.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value
-
hasVersionElement
-
hasVersion
-
setVersionElement
- Parameters:
value
-version
(The version of the device, if the device has multiple releases under the same model, or if the device is software or carries firmware.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value
-
getVersion
- Returns:
- The version of the device, if the device has multiple releases under the same model, or if the device is software or carries firmware.
-
setVersion
- Parameters:
value
- The version of the device, if the device has multiple releases under the same model, or if the device is software or carries firmware.
-
getManufactureDateElement
- Returns:
manufactureDate
(The date and time when the device was manufactured.). This is the underlying object with id, value and extensions. The accessor "getManufactureDate" gives direct access to the value
-
hasManufactureDateElement
-
hasManufactureDate
-
setManufactureDateElement
- Parameters:
value
-manufactureDate
(The date and time when the device was manufactured.). This is the underlying object with id, value and extensions. The accessor "getManufactureDate" gives direct access to the value
-
getManufactureDate
- Returns:
- The date and time when the device was manufactured.
-
setManufactureDate
- Parameters:
value
- The date and time when the device was manufactured.
-
getExpiryElement
- Returns:
expiry
(The date and time beyond which this device is no longer valid or should not be used (if applicable).). This is the underlying object with id, value and extensions. The accessor "getExpiry" gives direct access to the value
-
hasExpiryElement
-
hasExpiry
-
setExpiryElement
- Parameters:
value
-expiry
(The date and time beyond which this device is no longer valid or should not be used (if applicable).). This is the underlying object with id, value and extensions. The accessor "getExpiry" gives direct access to the value
-
getExpiry
- Returns:
- The date and time beyond which this device is no longer valid or should not be used (if applicable).
-
setExpiry
- Parameters:
value
- The date and time beyond which this device is no longer valid or should not be used (if applicable).
-
getUdiElement
- Returns:
udi
(United States Food and Drug Administration mandated Unique Device Identifier (UDI). Use the human readable information (the content that the user sees, which is sometimes different to the exact syntax represented in the barcode) - see http://www.fda.gov/MedicalDevices/DeviceRegulationandGuidance/UniqueDeviceIdentification/default.htm.). This is the underlying object with id, value and extensions. The accessor "getUdi" gives direct access to the value
-
hasUdiElement
-
hasUdi
-
setUdiElement
- Parameters:
value
-udi
(United States Food and Drug Administration mandated Unique Device Identifier (UDI). Use the human readable information (the content that the user sees, which is sometimes different to the exact syntax represented in the barcode) - see http://www.fda.gov/MedicalDevices/DeviceRegulationandGuidance/UniqueDeviceIdentification/default.htm.). This is the underlying object with id, value and extensions. The accessor "getUdi" gives direct access to the value
-
getUdi
- Returns:
- United States Food and Drug Administration mandated Unique Device Identifier (UDI). Use the human readable information (the content that the user sees, which is sometimes different to the exact syntax represented in the barcode) - see http://www.fda.gov/MedicalDevices/DeviceRegulationandGuidance/UniqueDeviceIdentification/default.htm.
-
setUdi
- Parameters:
value
- United States Food and Drug Administration mandated Unique Device Identifier (UDI). Use the human readable information (the content that the user sees, which is sometimes different to the exact syntax represented in the barcode) - see http://www.fda.gov/MedicalDevices/DeviceRegulationandGuidance/UniqueDeviceIdentification/default.htm.
-
getLotNumberElement
- Returns:
lotNumber
(Lot number assigned by the manufacturer.). This is the underlying object with id, value and extensions. The accessor "getLotNumber" gives direct access to the value
-
hasLotNumberElement
-
hasLotNumber
-
setLotNumberElement
- Parameters:
value
-lotNumber
(Lot number assigned by the manufacturer.). This is the underlying object with id, value and extensions. The accessor "getLotNumber" gives direct access to the value
-
getLotNumber
- Returns:
- Lot number assigned by the manufacturer.
-
setLotNumber
- Parameters:
value
- Lot number assigned by the manufacturer.
-
getOwner
- Returns:
owner
(An organization that is responsible for the provision and ongoing maintenance of the device.)
-
hasOwner
-
setOwner
- Parameters:
value
-owner
(An organization that is responsible for the provision and ongoing maintenance of the device.)
-
getOwnerTarget
- Returns:
owner
The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (An organization that is responsible for the provision and ongoing maintenance of the device.)
-
setOwnerTarget
- Parameters:
value
-owner
The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (An organization that is responsible for the provision and ongoing maintenance of the device.)
-
getLocation
- Returns:
location
(The place where the device can be found.)
-
hasLocation
-
setLocation
- Parameters:
value
-location
(The place where the device can be found.)
-
getLocationTarget
- Returns:
location
The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The place where the device can be found.)
-
setLocationTarget
- Parameters:
value
-location
The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The place where the device can be found.)
-
getPatient
- Returns:
patient
(Patient information, if the resource is affixed to a person.)
-
hasPatient
-
setPatient
- Parameters:
value
-patient
(Patient information, if the resource is affixed to a person.)
-
getPatientTarget
- Returns:
patient
The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Patient information, if the resource is affixed to a person.)
-
setPatientTarget
- Parameters:
value
-patient
The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Patient information, if the resource is affixed to a person.)
-
getContact
- Returns:
contact
(Contact details for an organization or a particular human that is responsible for the device.)
-
hasContact
-
addContact
- Returns:
contact
(Contact details for an organization or a particular human that is responsible for the device.)
-
addContact
-
getUrlElement
- Returns:
url
(A network address on which the device may be contacted directly.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value
-
hasUrlElement
-
hasUrl
-
setUrlElement
- Parameters:
value
-url
(A network address on which the device may be contacted directly.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value
-
getUrl
- Returns:
- A network address on which the device may be contacted directly.
-
setUrl
- Parameters:
value
- A network address on which the device may be contacted directly.
-
listChildren
- Overrides:
listChildren
in classDomainResource
-
setProperty
- Overrides:
setProperty
in classDomainResource
- Throws:
org.hl7.fhir.exceptions.FHIRException
-
addChild
- Overrides:
addChild
in classDomainResource
- Throws:
org.hl7.fhir.exceptions.FHIRException
-
fhirType
- Specified by:
fhirType
in interfaceorg.hl7.fhir.instance.model.api.IBase
- Overrides:
fhirType
in classDomainResource
-
copy
- Specified by:
copy
in classDomainResource
-
typedCopy
-
equalsDeep
- Overrides:
equalsDeep
in classDomainResource
-
equalsShallow
- Overrides:
equalsShallow
in classDomainResource
-
isEmpty
- Specified by:
isEmpty
in interfaceorg.hl7.fhir.instance.model.api.IBase
- Overrides:
isEmpty
in classDomainResource
-
getResourceType
- Specified by:
getResourceType
in classResource
-