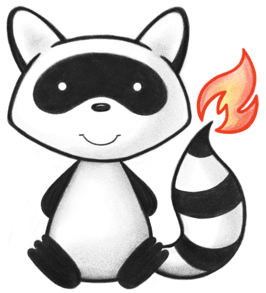
Package org.hl7.fhir.dstu2.model
Class OrderResponse
java.lang.Object
org.hl7.fhir.dstu2.model.Base
org.hl7.fhir.dstu2.model.BaseResource
org.hl7.fhir.dstu2.model.Resource
org.hl7.fhir.dstu2.model.DomainResource
org.hl7.fhir.dstu2.model.OrderResponse
- All Implemented Interfaces:
ca.uhn.fhir.model.api.IElement
,Serializable
,org.hl7.fhir.instance.model.api.IAnyResource
,org.hl7.fhir.instance.model.api.IBase
,org.hl7.fhir.instance.model.api.IBaseHasExtensions
,org.hl7.fhir.instance.model.api.IBaseHasModifierExtensions
,org.hl7.fhir.instance.model.api.IBaseResource
,org.hl7.fhir.instance.model.api.IDomainResource
A response to an order.
- See Also:
-
Nested Class Summary
Nested ClassesModifier and TypeClassDescriptionstatic enum
static class
-
Field Summary
FieldsModifier and TypeFieldDescriptionprotected DateTimeType
The date and time at which this order response was made (created/posted).protected StringType
Additional description about the response - e.g. a text description provided by a human user when making decisions about the order.Links to resources that provide details of the outcome of performing the order; e.g.The actual objects that are the target of the reference (Links to resources that provide details of the outcome of performing the order; e.g.protected List
<Identifier> Identifiers assigned to this order.protected Enumeration
<OrderResponse.OrderStatus> What this response says about the status of the original order.protected Reference
A reference to the order that this is in response to.protected Order
The actual object that is the target of the reference (A reference to the order that this is in response to.)static final String
static final String
static final String
static final String
static final String
static final String
protected Reference
The person, organization, or device credited with making the response.protected Resource
The actual object that is the target of the reference (The person, organization, or device credited with making the response.)Fields inherited from class org.hl7.fhir.dstu2.model.DomainResource
contained, extension, modifierExtension, text
Fields inherited from class org.hl7.fhir.dstu2.model.Resource
id, implicitRules, language, meta
Fields inherited from interface org.hl7.fhir.instance.model.api.IAnyResource
RES_ID, RES_LAST_UPDATED, RES_PROFILE, RES_SECURITY, RES_TAG, SP_RES_ID, SP_RES_LAST_UPDATED, SP_RES_PROFILE, SP_RES_SECURITY, SP_RES_TAG
Fields inherited from interface org.hl7.fhir.instance.model.api.IBaseResource
INCLUDE_ALL, WILDCARD_ALL_SET
-
Constructor Summary
ConstructorsConstructorDescriptionOrderResponse
(Reference request, Enumeration<OrderResponse.OrderStatus> orderStatus) -
Method Summary
Modifier and TypeMethodDescriptioncopy()
boolean
equalsDeep
(Base other) boolean
equalsShallow
(Base other) fhirType()
getDate()
getWho()
boolean
hasDate()
boolean
boolean
boolean
boolean
boolean
boolean
boolean
boolean
boolean
hasWho()
boolean
isEmpty()
protected void
listChildren
(List<Property> childrenList) setDateElement
(DateTimeType value) setDescription
(String value) setDescriptionElement
(StringType value) void
setProperty
(String name, Base value) setRequest
(Reference value) setRequestTarget
(Order value) setWhoTarget
(Resource value) protected OrderResponse
Methods inherited from class org.hl7.fhir.dstu2.model.DomainResource
addContained, addExtension, addExtension, addModifierExtension, addModifierExtension, copyValues, getContained, getExtension, getExtensionByUrl, getModifierExtension, getText, hasContained, hasExtension, hasExtension, hasModifierExtension, hasText, setText
Methods inherited from class org.hl7.fhir.dstu2.model.Resource
copyValues, getId, getIdElement, getImplicitRules, getImplicitRulesElement, getLanguage, getLanguageElement, getMeta, hasId, hasIdElement, hasImplicitRules, hasImplicitRulesElement, hasLanguage, hasLanguageElement, hasMeta, setId, setIdElement, setImplicitRules, setImplicitRulesElement, setLanguage, setLanguageElement, setMeta
Methods inherited from class org.hl7.fhir.dstu2.model.BaseResource
getStructureFhirVersionEnum, setId
Methods inherited from class org.hl7.fhir.dstu2.model.Base
castToAddress, castToAnnotation, castToAttachment, castToBase64Binary, castToBoolean, castToCode, castToCodeableConcept, castToCoding, castToContactPoint, castToDate, castToDateTime, castToDecimal, castToDuration, castToElementDefinition, castToExtension, castToHumanName, castToId, castToIdentifier, castToInstant, castToInteger, castToMarkdown, castToMeta, castToMoney, castToNarrative, castToOid, castToPeriod, castToPositiveInt, castToQuantity, castToRange, castToRatio, castToReference, castToResource, castToSampledData, castToSignature, castToSimpleQuantity, castToString, castToTime, castToTiming, castToUnsignedInt, castToUri, children, compareDeep, compareDeep, compareDeep, compareValues, compareValues, equals, getChildByName, getFormatCommentsPost, getFormatCommentsPre, getUserData, getUserInt, getUserString, hasFormatComment, hasType, hasUserData, isMetadataBased, isPrimitive, listChildrenByName, primitiveValue, setUserData, setUserDataINN
Methods inherited from class java.lang.Object
clone, equals, finalize, getClass, hashCode, notify, notifyAll, toString, wait, wait, wait
Methods inherited from interface org.hl7.fhir.instance.model.api.IAnyResource
getId, getIdElement, getLanguageElement, getUserData, setId, setUserData
Methods inherited from interface org.hl7.fhir.instance.model.api.IBase
getFormatCommentsPost, getFormatCommentsPre, hasFormatComment
Methods inherited from interface org.hl7.fhir.instance.model.api.IBaseResource
getMeta, getStructureFhirVersionEnum, isDeleted, setId
-
Field Details
-
identifier
Identifiers assigned to this order. The identifiers are usually assigned by the system responding to the order, but they may be provided or added to by other systems. -
request
A reference to the order that this is in response to. -
requestTarget
The actual object that is the target of the reference (A reference to the order that this is in response to.) -
date
The date and time at which this order response was made (created/posted). -
who
The person, organization, or device credited with making the response. -
whoTarget
The actual object that is the target of the reference (The person, organization, or device credited with making the response.) -
orderStatus
What this response says about the status of the original order. -
description
Additional description about the response - e.g. a text description provided by a human user when making decisions about the order. -
fulfillment
Links to resources that provide details of the outcome of performing the order; e.g. Diagnostic Reports in a response that is made to an order that referenced a diagnostic order. -
fulfillmentTarget
The actual objects that are the target of the reference (Links to resources that provide details of the outcome of performing the order; e.g. Diagnostic Reports in a response that is made to an order that referenced a diagnostic order.) -
SP_DATE
- See Also:
-
SP_REQUEST
- See Also:
-
SP_IDENTIFIER
- See Also:
-
SP_CODE
- See Also:
-
SP_FULFILLMENT
- See Also:
-
SP_WHO
- See Also:
-
-
Constructor Details
-
OrderResponse
public OrderResponse() -
OrderResponse
-
-
Method Details
-
getIdentifier
- Returns:
identifier
(Identifiers assigned to this order. The identifiers are usually assigned by the system responding to the order, but they may be provided or added to by other systems.)
-
hasIdentifier
-
addIdentifier
- Returns:
identifier
(Identifiers assigned to this order. The identifiers are usually assigned by the system responding to the order, but they may be provided or added to by other systems.)
-
addIdentifier
-
getRequest
- Returns:
request
(A reference to the order that this is in response to.)
-
hasRequest
-
setRequest
- Parameters:
value
-request
(A reference to the order that this is in response to.)
-
getRequestTarget
- Returns:
request
The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (A reference to the order that this is in response to.)
-
setRequestTarget
- Parameters:
value
-request
The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (A reference to the order that this is in response to.)
-
getDateElement
- Returns:
date
(The date and time at which this order response was made (created/posted).). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value
-
hasDateElement
-
hasDate
-
setDateElement
- Parameters:
value
-date
(The date and time at which this order response was made (created/posted).). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value
-
getDate
- Returns:
- The date and time at which this order response was made (created/posted).
-
setDate
- Parameters:
value
- The date and time at which this order response was made (created/posted).
-
getWho
- Returns:
who
(The person, organization, or device credited with making the response.)
-
hasWho
-
setWho
- Parameters:
value
-who
(The person, organization, or device credited with making the response.)
-
getWhoTarget
- Returns:
who
The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The person, organization, or device credited with making the response.)
-
setWhoTarget
- Parameters:
value
-who
The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The person, organization, or device credited with making the response.)
-
getOrderStatusElement
- Returns:
orderStatus
(What this response says about the status of the original order.). This is the underlying object with id, value and extensions. The accessor "getOrderStatus" gives direct access to the value
-
hasOrderStatusElement
-
hasOrderStatus
-
setOrderStatusElement
- Parameters:
value
-orderStatus
(What this response says about the status of the original order.). This is the underlying object with id, value and extensions. The accessor "getOrderStatus" gives direct access to the value
-
getOrderStatus
- Returns:
- What this response says about the status of the original order.
-
setOrderStatus
- Parameters:
value
- What this response says about the status of the original order.
-
getDescriptionElement
- Returns:
description
(Additional description about the response - e.g. a text description provided by a human user when making decisions about the order.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value
-
hasDescriptionElement
-
hasDescription
-
setDescriptionElement
- Parameters:
value
-description
(Additional description about the response - e.g. a text description provided by a human user when making decisions about the order.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value
-
getDescription
- Returns:
- Additional description about the response - e.g. a text description provided by a human user when making decisions about the order.
-
setDescription
- Parameters:
value
- Additional description about the response - e.g. a text description provided by a human user when making decisions about the order.
-
getFulfillment
- Returns:
fulfillment
(Links to resources that provide details of the outcome of performing the order; e.g. Diagnostic Reports in a response that is made to an order that referenced a diagnostic order.)
-
hasFulfillment
-
addFulfillment
- Returns:
fulfillment
(Links to resources that provide details of the outcome of performing the order; e.g. Diagnostic Reports in a response that is made to an order that referenced a diagnostic order.)
-
addFulfillment
-
getFulfillmentTarget
- Returns:
fulfillment
(The actual objects that are the target of the reference. The reference library doesn't populate this, but you can use this to hold the resources if you resolvethemt. Links to resources that provide details of the outcome of performing the order; e.g. Diagnostic Reports in a response that is made to an order that referenced a diagnostic order.)
-
listChildren
- Overrides:
listChildren
in classDomainResource
-
setProperty
- Overrides:
setProperty
in classDomainResource
- Throws:
org.hl7.fhir.exceptions.FHIRException
-
addChild
- Overrides:
addChild
in classDomainResource
- Throws:
org.hl7.fhir.exceptions.FHIRException
-
fhirType
- Specified by:
fhirType
in interfaceorg.hl7.fhir.instance.model.api.IBase
- Overrides:
fhirType
in classDomainResource
-
copy
- Specified by:
copy
in classDomainResource
-
typedCopy
-
equalsDeep
- Overrides:
equalsDeep
in classDomainResource
-
equalsShallow
- Overrides:
equalsShallow
in classDomainResource
-
isEmpty
- Specified by:
isEmpty
in interfaceorg.hl7.fhir.instance.model.api.IBase
- Overrides:
isEmpty
in classDomainResource
-
getResourceType
- Specified by:
getResourceType
in classResource
-