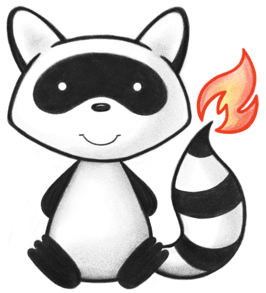
Class BaseDateTimeType
- All Implemented Interfaces:
ca.uhn.fhir.model.api.IElement
,Externalizable
,Serializable
,org.hl7.fhir.instance.model.api.IBase
,org.hl7.fhir.instance.model.api.IBaseDatatype
,org.hl7.fhir.instance.model.api.IBaseElement
,org.hl7.fhir.instance.model.api.IBaseHasExtensions
,org.hl7.fhir.instance.model.api.IPrimitiveType<Date>
- Direct Known Subclasses:
DateTimeType
,DateType
,InstantType
- See Also:
-
Field Summary
-
Constructor Summary
ConstructorsConstructorDescriptionConstructorBaseDateTimeType
(String theString) ConstructorBaseDateTimeType
(Date theDate, ca.uhn.fhir.model.api.TemporalPrecisionEnum thePrecision) ConstructorBaseDateTimeType
(Date theDate, ca.uhn.fhir.model.api.TemporalPrecisionEnum thePrecision, TimeZone theTimeZone) Constructor -
Method Summary
Modifier and TypeMethodDescriptionvoid
add
(int theField, int theValue) Adds the given amount to the field specified by theFieldboolean
after
(DateTimeType theDateTimeType) Returnstrue
if the given object represents a date/time beforethis
objectboolean
before
(DateTimeType theDateTimeType) Returnstrue
if the given object represents a date/time beforethis
objectstatic Integer
compareTimes
(BaseDateTimeType left, BaseDateTimeType right, Integer def) protected String
Subclasses must override to convert a "coerced" value into an encoded one.equalsUsingFhirPathRules
(BaseDateTimeType theOther) This method implements a datetime equality check using the rules as defined by FHIRPath.fpValue()
getDay()
Returns the month with 1-index, e.g. 1=the first day of the monthprotected abstract ca.uhn.fhir.model.api.TemporalPrecisionEnum
Returns the default precision for the given datatypegetHour()
Returns the hour of the day in a 24h clock, e.g. 13=1pmReturns the milliseconds within the current second.Returns the minute of the hour in the range 0-59getMonth()
Returns the month with 0-index, e.g. 0=JanuarygetNanos()
Returns the nanoseconds within the current secondca.uhn.fhir.model.api.TemporalPrecisionEnum
Gets the precision for this datatype (using the default for the given type if not set)Returns the second of the minute in the range 0-59float
Returns the TimeZone associated with this dateTime's value.Returns the value of this object as aGregorianCalendar
getValueAsString
(ca.uhn.fhir.model.api.TemporalPrecisionEnum thePrecision) getYear()
Returns the year, e.g. 2015boolean
hasTime()
boolean
boolean
Returns true if the timezone is set to GMT-0:00 (Z)boolean
isToday()
Returnstrue
if this object represents a date that is today's dateprotected Date
Subclasses must override to convert an encoded representation of this datatype into a "coerced" onesetDay
(int theDay) Sets the month with 1-index, e.g. 1=the first day of the monthsetHour
(int theHour) Sets the hour of the day in a 24h clock, e.g. 13=1pmsetMillis
(int theMillis) Sets the milliseconds within the current second.setMinute
(int theMinute) Sets the minute of the hour in the range 0-59setMonth
(int theMonth) Sets the month with 0-index, e.g. 0=JanuarysetNanos
(long theNanos) Sets the nanoseconds within the current secondvoid
setPrecision
(ca.uhn.fhir.model.api.TemporalPrecisionEnum thePrecision) Sets the precision for this datatypesetSecond
(int theSecond) Sets the second of the minute in the range 0-59setTimeZone
(TimeZone theTimeZone) setTimeZoneZulu
(boolean theTimeZoneZulu) Sets the value for this type using the given Java Date object as the time, and using the default precision for this datatype (unless the precision is already set), as well as the local timezone as determined by the local operating system.void
Sets the value for this type using the given Java Date object as the time, and using the specified precision, as well as the local timezone as determined by the local operating system.void
setValueAsString
(String theString) protected void
setValueAsV3String
(String theV3String) setYear
(int theYear) Sets the year, e.g. 2015Returns a view of this date/time as a Calendar object.Returns a human readable version of this date/time using the system local format.Returns a human readable version of this date/time using the system local format, converted to the local timezone if neccesary.Methods inherited from class org.hl7.fhir.r4.model.PrimitiveType
asStringValue, copy, equalsDeep, equalsShallow, forceStringValue, fromStringValue, getProperty, getTypesForProperty, getValue, getValueAsString, hashCode, hasPrimitiveValue, hasValue, isEmpty, isPrimitive, makeProperty, primitiveValue, readExternal, removeChild, setProperty, setProperty, toString, typedCopy, updateStringValue, writeExternal
Methods inherited from class org.hl7.fhir.r4.model.Element
addChild, addExtension, addExtension, addExtension, copyExtensions, copyNewExtensions, copyValues, fhirType, getExtension, getExtensionByUrl, getExtensionFirstRep, getExtensionsByUrl, getExtensionsByUrl, getExtensionString, getId, getIdBase, getIdElement, getNamedProperty, hasExtension, hasExtension, hasExtension, hasExtension, hasId, hasIdElement, isDisallowExtensions, listChildren, noExtensions, removeExtension, setDisallowExtensions, setExtension, setId, setIdBase, setIdElement
Methods inherited from class org.hl7.fhir.r4.model.Base
castToAddress, castToAnnotation, castToAttachment, castToBase64Binary, castToBoolean, castToCanonical, castToCode, castToCodeableConcept, castToCoding, castToContactDetail, castToContactPoint, castToContributor, castToDataRequirement, castToDate, castToDateTime, castToDecimal, castToDosage, castToDuration, castToElementDefinition, castToExpression, castToExtension, castToHumanName, castToId, castToIdentifier, castToInstant, castToInteger, castToMarkdown, castToMarketingStatus, castToMeta, castToMoney, castToNarrative, castToOid, castToParameterDefinition, castToPeriod, castToPopulation, castToPositiveInt, castToProdCharacteristic, castToProductShelfLife, castToQuantity, castToRange, castToRatio, castToReference, castToRelatedArtifact, castToResource, castToSampledData, castToSignature, castToSimpleQuantity, castToString, castToSubstanceAmount, castToTime, castToTiming, castToTriggerDefinition, castToType, castToUnsignedInt, castToUri, castToUrl, castToUsageContext, castToXhtml, castToXhtmlString, children, clearUserData, compareDeep, compareDeep, compareDeep, compareDeep, compareValues, compareValues, copyValues, equals, getChildByName, getChildValueByName, getFormatCommentsPost, getFormatCommentsPre, getNamedProperty, getUserData, getUserInt, getUserString, getXhtml, hasFormatComment, hasType, hasUserData, isBooleanPrimitive, isMetadataBased, isResource, listChildrenByName, listChildrenByName, setUserData, setUserDataINN, setXhtml
Methods inherited from class java.lang.Object
clone, equals, finalize, getClass, notify, notifyAll, wait, wait, wait
Methods inherited from interface org.hl7.fhir.instance.model.api.IBase
fhirType, getFormatCommentsPost, getFormatCommentsPre, getUserData, hasFormatComment, setUserData
Methods inherited from interface org.hl7.fhir.instance.model.api.IBaseElement
getUserData, setUserData
Methods inherited from interface org.hl7.fhir.instance.model.api.IBaseHasExtensions
addExtension, getExtension, hasExtension
-
Constructor Details
-
BaseDateTimeType
public BaseDateTimeType()Constructor -
BaseDateTimeType
Constructor- Throws:
IllegalArgumentException
- If the specified precision is not allowed for this type
-
BaseDateTimeType
public BaseDateTimeType(Date theDate, ca.uhn.fhir.model.api.TemporalPrecisionEnum thePrecision, TimeZone theTimeZone) Constructor -
BaseDateTimeType
Constructor- Throws:
IllegalArgumentException
- If the specified precision is not allowed for this type
-
-
Method Details
-
add
Adds the given amount to the field specified by theField- Parameters:
theField
- The field, uses constants fromCalendar
such asCalendar.YEAR
theValue
- The number to add (or subtract for a negative number)
-
after
Returnstrue
if the given object represents a date/time beforethis
object- Throws:
NullPointerException
- Ifthis.getValue()
ortheDateTimeType.getValue()
returnnull
-
before
Returnstrue
if the given object represents a date/time beforethis
object- Throws:
NullPointerException
- Ifthis.getValue()
ortheDateTimeType.getValue()
returnnull
-
getValueAsString
- Parameters:
thePrecision
-- Returns:
- the String value of this instance with the specified precision.
-
encode
Description copied from class:PrimitiveType
Subclasses must override to convert a "coerced" value into an encoded one.- Specified by:
encode
in classPrimitiveType<Date>
- Parameters:
theValue
- Will not be null- Returns:
- May return null if the value does not correspond to anything
-
getDay
Returns the month with 1-index, e.g. 1=the first day of the month -
getDefaultPrecisionForDatatype
Returns the default precision for the given datatype -
getHour
Returns the hour of the day in a 24h clock, e.g. 13=1pm -
getMillis
Returns the milliseconds within the current second.Note that this method returns the same value as
getNanos()
but with less precision. -
getMinute
Returns the minute of the hour in the range 0-59 -
getMonth
Returns the month with 0-index, e.g. 0=January -
getSecondsMilli
-
getNanos
Returns the nanoseconds within the current secondNote that this method returns the same value as
getMillis()
but with more precision. -
getPrecision
Gets the precision for this datatype (using the default for the given type if not set)- See Also:
-
getSecond
Returns the second of the minute in the range 0-59 -
getTimeZone
Returns the TimeZone associated with this dateTime's value. May returnnull
if no timezone was supplied. -
getValueAsCalendar
Returns the value of this object as aGregorianCalendar
-
getYear
Returns the year, e.g. 2015 -
isTimeZoneZulu
Returns true if the timezone is set to GMT-0:00 (Z) -
isToday
Returnstrue
if this object represents a date that is today's date- Throws:
NullPointerException
- ifPrimitiveType.getValue()
returnsnull
-
parse
Description copied from class:PrimitiveType
Subclasses must override to convert an encoded representation of this datatype into a "coerced" one- Specified by:
parse
in classPrimitiveType<Date>
- Parameters:
theValue
- Will not be null- Returns:
- May return null if the value does not correspond to anything
- Throws:
DataFormatException
-
setDay
Sets the month with 1-index, e.g. 1=the first day of the month -
setHour
Sets the hour of the day in a 24h clock, e.g. 13=1pm -
setMillis
Sets the milliseconds within the current second.Note that this method sets the same value as
setNanos(long)
but with less precision. -
setMinute
Sets the minute of the hour in the range 0-59 -
setMonth
Sets the month with 0-index, e.g. 0=January -
setNanos
Sets the nanoseconds within the current secondNote that this method sets the same value as
setMillis(int)
but with more precision. -
setPrecision
public void setPrecision(ca.uhn.fhir.model.api.TemporalPrecisionEnum thePrecision) throws DataFormatException Sets the precision for this datatype- Throws:
DataFormatException
-
setSecond
Sets the second of the minute in the range 0-59 -
setTimeZone
-
setTimeZoneZulu
-
setValue
Sets the value for this type using the given Java Date object as the time, and using the default precision for this datatype (unless the precision is already set), as well as the local timezone as determined by the local operating system. Both of these properties may be modified in subsequent calls if neccesary.- Specified by:
setValue
in interfaceorg.hl7.fhir.instance.model.api.IPrimitiveType<Date>
- Overrides:
setValue
in classPrimitiveType<Date>
-
setValue
public void setValue(Date theValue, ca.uhn.fhir.model.api.TemporalPrecisionEnum thePrecision) throws DataFormatException Sets the value for this type using the given Java Date object as the time, and using the specified precision, as well as the local timezone as determined by the local operating system. Both of these properties may be modified in subsequent calls if neccesary.- Parameters:
theValue
- The date valuethePrecision
- The precision- Throws:
DataFormatException
-
setValueAsString
- Specified by:
setValueAsString
in interfaceorg.hl7.fhir.instance.model.api.IPrimitiveType<Date>
- Overrides:
setValueAsString
in classPrimitiveType<Date>
- Throws:
DataFormatException
-
setValueAsV3String
-
setYear
Sets the year, e.g. 2015 -
toCalendar
Returns a view of this date/time as a Calendar object. Note that the returned Calendar object is entirely independent fromthis
object. Changes to the calendar will not affectthis
. -
toHumanDisplay
Returns a human readable version of this date/time using the system local format.Note on time zones: This method renders the value using the time zone that is contained within the value. For example, if this date object contains the value "2012-01-05T12:00:00-08:00", the human display will be rendered as "12:00:00" even if the application is being executed on a system in a different time zone. If this behaviour is not what you want, use
toHumanDisplayLocalTimezone()
instead. -
toHumanDisplayLocalTimezone
Returns a human readable version of this date/time using the system local format, converted to the local timezone if neccesary.- See Also:
-
isDateTime
- Overrides:
isDateTime
in classBase
-
dateTimeValue
- Overrides:
dateTimeValue
in classBase
-
hasTime
-
equalsUsingFhirPathRules
This method implements a datetime equality check using the rules as defined by FHIRPath. This method returns:- true if the given datetimes represent the exact same instant with the same precision (irrespective of the timezone)
- true if the given datetimes represent the exact same instant but one
includes milliseconds of
.[0]+
while the other includes only SECONDS precision (irrespecitve of the timezone) - true if the given datetimes represent the exact same year/year-month/year-month-date (if both operands have the same precision)
- false if both datetimes have equal precision of MINUTE or greater, one has no timezone specified but the other does, and could not represent the same instant in any timezone
- null if both datetimes have equal precision of MINUTE or greater, one has no timezone specified but the other does, and could potentially represent the same instant in any timezone
- false if the given datetimes have the same precision but do not represent the same instant (irrespective of timezone)
- null otherwise (since these datetimes are not comparable)
-
compareTimes
-
fpValue
- Overrides:
fpValue
in classPrimitiveType<Date>
-