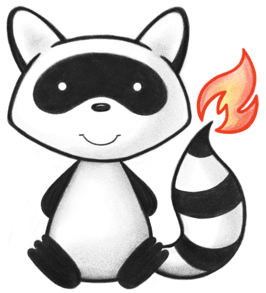
Class IdType
- All Implemented Interfaces:
ca.uhn.fhir.model.api.IElement
,Externalizable
,Serializable
,org.hl7.fhir.instance.model.api.IBase
,org.hl7.fhir.instance.model.api.IBaseDatatype
,org.hl7.fhir.instance.model.api.IBaseElement
,org.hl7.fhir.instance.model.api.IBaseHasExtensions
,org.hl7.fhir.instance.model.api.IIdType
,org.hl7.fhir.instance.model.api.IPrimitiveType<String>
Any combination of upper or lower case ASCII letters ('A'..'Z', and 'a'..'z', numerals ('0'..'9'), '-' and '.', with a length limit of 64 characters. (This might be an integer, an un-prefixed OID, UUID or any other identifier pattern that meets these constraints.)
This class contains that logical ID, and can optionally also contain a relative or absolute URL representing the resource identity. For example, the following are all valid values for IdType, and all might represent the same resource:
123
(just a resource's ID)Patient/123
(a relative identity)http://example.com/Patient/123 (an absolute identity)
-
http://example.com/Patient/123/_history/1 (an absolute identity with a version id)
-
Patient/123/_history/1 (a relative identity with a version id)
Note that the 64 character limit applies only to the ID portion ("123" in the examples above).
In most situations, you only need to populate the resource's ID (e.g.
123
) in resources you are constructing and the encoder will
infer the rest from the context in which the object is being used. On the
other hand, the parser will always try to populate the complete absolute
identity on objects it creates as a convenience.
Regex for ID: [a-z0-9\-\.]{1,36}
- See Also:
-
Field Summary
FieldsModifier and TypeFieldDescriptionstatic final int
This is the maximum length for the IDstatic final String
-
Constructor Summary
ConstructorsConstructorDescriptionIdType()
Create a new empty IDIdType
(long theId) Create a new ID using a longCreate a new ID using a string.ConstructorConstructorConstructorConstructorIdType
(String theResourceType, BigDecimal theIdPart) ConstructorIdType
(BigDecimal thePid) Create a new ID, using a BigDecimal input.Creates an ID based on a given URL -
Method Summary
Modifier and TypeMethodDescriptionvoid
applyTo
(org.hl7.fhir.instance.model.api.IBaseResource theResouce) Deprecated.copy()
boolean
boolean
equalsIgnoreBase
(IdType theId) Returns true if this IdType matches the given IdType in terms of resource type and ID, but ignores the URL basefhirType()
Returns the portion of this resource ID which corresponds to the server base URL.Returns only the logical ID part of this ID.Returns the unqualified portion of this ID as a big decimal, ornull
if the value is nullReturns the unqualified portion of this ID as aLong
, ornull
if the value is nullgetValue()
Returns the value of this ID.boolean
Returns true if this ID has a base urlint
hashCode()
boolean
boolean
boolean
boolean
Returnstrue
if this ID contains an absolute URL (in other words, a URL starting with "http://" or "https://"boolean
isEmpty()
boolean
boolean
Returnstrue
if the unqualified ID is a validLong
value (in other words, it consists only of digits)boolean
isLocal()
Returnstrue
if the ID is a local reference (in other words, it begins with the '#' character)boolean
isUrn()
boolean
static IdType
Construct a new ID with with form "urn:uuid:[UUID]" where [UUID] is a new, randomly created UUID generated byUUID.randomUUID()
static IdType
of
(org.hl7.fhir.instance.model.api.IBaseResource theResouce) Retrieves the ID from the given resource instanceorg.hl7.fhir.instance.model.api.IIdType
Set the valuevoid
setValueAsString
(String theValue) Set the valuetoString()
Returns a new IdType containing this IdType's values but with no server base URL if one is present in this IdType.withResourceType
(String theResourceName) withServerBase
(String theServerBase, String theResourceType) Returns a view of this ID as a fully qualified URL, given a server base and resource name (which will only be used if the ID does not already contain those respective parts).withVersion
(String theVersion) Creates a new instance of this ID which is identical, but refers to the specific version of this resource ID noted by theVersion.Methods inherited from class org.hl7.fhir.r4.model.UriType
encode, equals, equalsDeep, fromOid, parse
Methods inherited from class org.hl7.fhir.r4.model.PrimitiveType
equalsShallow, forceStringValue, fpValue, fromStringValue, getProperty, getTypesForProperty, hasPrimitiveValue, hasValue, isPrimitive, makeProperty, primitiveValue, readExternal, removeChild, setProperty, setProperty, typedCopy, updateStringValue, writeExternal
Methods inherited from class org.hl7.fhir.r4.model.Element
addChild, addExtension, addExtension, addExtension, copyExtensions, copyNewExtensions, copyValues, getExtension, getExtensionByUrl, getExtensionFirstRep, getExtensionsByUrl, getExtensionsByUrl, getExtensionString, getId, getIdBase, getIdElement, getNamedProperty, hasExtension, hasExtension, hasExtension, hasExtension, hasId, hasIdElement, isDisallowExtensions, listChildren, noExtensions, removeExtension, setDisallowExtensions, setExtension, setId, setIdBase, setIdElement
Methods inherited from class org.hl7.fhir.r4.model.Base
castToAddress, castToAnnotation, castToAttachment, castToBase64Binary, castToBoolean, castToCanonical, castToCode, castToCodeableConcept, castToCoding, castToContactDetail, castToContactPoint, castToContributor, castToDataRequirement, castToDate, castToDateTime, castToDecimal, castToDosage, castToDuration, castToElementDefinition, castToExpression, castToExtension, castToHumanName, castToId, castToIdentifier, castToInstant, castToInteger, castToMarkdown, castToMarketingStatus, castToMeta, castToMoney, castToNarrative, castToOid, castToParameterDefinition, castToPeriod, castToPopulation, castToPositiveInt, castToProdCharacteristic, castToProductShelfLife, castToQuantity, castToRange, castToRatio, castToReference, castToRelatedArtifact, castToResource, castToSampledData, castToSignature, castToSimpleQuantity, castToString, castToSubstanceAmount, castToTime, castToTiming, castToTriggerDefinition, castToType, castToUnsignedInt, castToUri, castToUrl, castToUsageContext, castToXhtml, castToXhtmlString, children, clearUserData, compareDeep, compareDeep, compareDeep, compareDeep, compareValues, compareValues, copyValues, dateTimeValue, equals, getChildByName, getChildValueByName, getFormatCommentsPost, getFormatCommentsPre, getNamedProperty, getUserData, getUserInt, getUserString, getXhtml, hasFormatComment, hasType, hasUserData, isBooleanPrimitive, isDateTime, isMetadataBased, isResource, listChildrenByName, listChildrenByName, setUserData, setUserDataINN
Methods inherited from class java.lang.Object
clone, finalize, getClass, notify, notifyAll, wait, wait, wait
Methods inherited from interface org.hl7.fhir.instance.model.api.IBase
getFormatCommentsPost, getFormatCommentsPre, getUserData, hasFormatComment, setUserData
Methods inherited from interface org.hl7.fhir.instance.model.api.IBaseElement
getUserData, setUserData
Methods inherited from interface org.hl7.fhir.instance.model.api.IBaseHasExtensions
addExtension, getExtension, hasExtension
Methods inherited from interface org.hl7.fhir.instance.model.api.IIdType
isUuid
Methods inherited from interface org.hl7.fhir.instance.model.api.IPrimitiveType
hasValue
-
Field Details
-
URN_PREFIX
- See Also:
-
MAX_LENGTH
This is the maximum length for the ID- See Also:
-
-
Constructor Details
-
IdType
public IdType()Create a new empty ID -
IdType
Create a new ID, using a BigDecimal input. UsesBigDecimal.toPlainString()
to generate the string representation. -
IdType
Create a new ID using a long -
IdType
Create a new ID using a string. This String may contain a simple ID (e.g. "1234") or it may contain a complete URL (http://example.com/fhir/Patient/1234).Description: A whole number in the range 0 to 2^64-1 (optionally represented in hex), a uuid, an oid, or any other combination of lowercase letters, numerals, "-" and ".", with a length limit of 36 characters.
regex: [a-z0-9\-\.]{1,36}
-
IdType
Constructor- Parameters:
theResourceType
- The resource type (e.g. "Patient")theIdPart
- The ID (e.g. "123")
-
IdType
Constructor- Parameters:
theResourceType
- The resource type (e.g. "Patient")theIdPart
- The ID (e.g. "123")
-
IdType
Constructor- Parameters:
theResourceType
- The resource type (e.g. "Patient")theId
- The ID (e.g. "123")
-
IdType
Constructor- Parameters:
theResourceType
- The resource type (e.g. "Patient")theId
- The ID (e.g. "123")theVersionId
- The version ID ("e.g. "456")
-
IdType
Constructor- Parameters:
theBaseUrl
- The server base URL (e.g. "http://example.com/fhir")theResourceType
- The resource type (e.g. "Patient")theId
- The ID (e.g. "123")theVersionId
- The version ID ("e.g. "456")
-
IdType
Creates an ID based on a given URL
-
-
Method Details
-
applyTo
- Specified by:
applyTo
in interfaceorg.hl7.fhir.instance.model.api.IIdType
-
asBigDecimal
Deprecated.UsegetIdPartAsBigDecimal()
instead (this method was deprocated because its name is ambiguous) -
copy
-
equals
-
equalsIgnoreBase
Returns true if this IdType matches the given IdType in terms of resource type and ID, but ignores the URL base -
fhirType
-
getBaseUrl
Returns the portion of this resource ID which corresponds to the server base URL. For example given the resource IDhttp://example.com/fhir/Patient/123
the base URL would behttp://example.com/fhir
.This method may return null if the ID contains no base (e.g. "Patient/123")
- Specified by:
getBaseUrl
in interfaceorg.hl7.fhir.instance.model.api.IIdType
-
getIdPart
Returns only the logical ID part of this ID. For example, given the ID "http://example,.com/fhir/Patient/123/_history/456", this method would return "123".- Specified by:
getIdPart
in interfaceorg.hl7.fhir.instance.model.api.IIdType
-
getIdPartAsBigDecimal
Returns the unqualified portion of this ID as a big decimal, ornull
if the value is null- Throws:
NumberFormatException
- If the value is not a valid BigDecimal
-
getIdPartAsLong
Returns the unqualified portion of this ID as aLong
, ornull
if the value is null- Specified by:
getIdPartAsLong
in interfaceorg.hl7.fhir.instance.model.api.IIdType
- Throws:
NumberFormatException
- If the value is not a valid Long
-
getResourceType
- Specified by:
getResourceType
in interfaceorg.hl7.fhir.instance.model.api.IIdType
-
getValue
Returns the value of this ID. Note that this value may be a fully qualified URL, a relative/partial URL, or a simple ID. UsegetIdPart()
to get just the ID portion.- Specified by:
getValue
in interfaceorg.hl7.fhir.instance.model.api.IIdType
- Specified by:
getValue
in interfaceorg.hl7.fhir.instance.model.api.IPrimitiveType<String>
- Overrides:
getValue
in classPrimitiveType<String>
- See Also:
-
setValue
Set the valueDescription: A whole number in the range 0 to 2^64-1 (optionally represented in hex), a uuid, an oid, or any other combination of lowercase letters, numerals, "-" and ".", with a length limit of 36 characters.
regex: [a-z0-9\-\.]{1,36}
- Specified by:
setValue
in interfaceorg.hl7.fhir.instance.model.api.IIdType
- Specified by:
setValue
in interfaceorg.hl7.fhir.instance.model.api.IPrimitiveType<String>
- Overrides:
setValue
in classPrimitiveType<String>
-
getValueAsString
- Specified by:
getValueAsString
in interfaceorg.hl7.fhir.instance.model.api.IPrimitiveType<String>
- Overrides:
getValueAsString
in classPrimitiveType<String>
-
asStringValue
- Overrides:
asStringValue
in classPrimitiveType<String>
-
setValueAsString
Set the valueDescription: A whole number in the range 0 to 2^64-1 (optionally represented in hex), a uuid, an oid, or any other combination of lowercase letters, numerals, "-" and ".", with a length limit of 36 characters.
regex: [a-z0-9\-\.]{1,36}
- Specified by:
setValueAsString
in interfaceorg.hl7.fhir.instance.model.api.IPrimitiveType<String>
- Overrides:
setValueAsString
in classPrimitiveType<String>
-
getVersionIdPart
- Specified by:
getVersionIdPart
in interfaceorg.hl7.fhir.instance.model.api.IIdType
-
getVersionIdPartAsLong
- Specified by:
getVersionIdPartAsLong
in interfaceorg.hl7.fhir.instance.model.api.IIdType
-
hasBaseUrl
Returns true if this ID has a base url- Specified by:
hasBaseUrl
in interfaceorg.hl7.fhir.instance.model.api.IIdType
- See Also:
-
hasIdPart
- Specified by:
hasIdPart
in interfaceorg.hl7.fhir.instance.model.api.IIdType
-
hasResourceType
- Specified by:
hasResourceType
in interfaceorg.hl7.fhir.instance.model.api.IIdType
-
hasVersionIdPart
- Specified by:
hasVersionIdPart
in interfaceorg.hl7.fhir.instance.model.api.IIdType
-
hashCode
-
isAbsolute
Returnstrue
if this ID contains an absolute URL (in other words, a URL starting with "http://" or "https://"- Specified by:
isAbsolute
in interfaceorg.hl7.fhir.instance.model.api.IIdType
-
isEmpty
- Specified by:
isEmpty
in interfaceorg.hl7.fhir.instance.model.api.IBase
- Specified by:
isEmpty
in interfaceorg.hl7.fhir.instance.model.api.IIdType
- Overrides:
isEmpty
in classPrimitiveType<String>
-
isIdPartValid
- Specified by:
isIdPartValid
in interfaceorg.hl7.fhir.instance.model.api.IIdType
-
isIdPartValidLong
Returnstrue
if the unqualified ID is a validLong
value (in other words, it consists only of digits)- Specified by:
isIdPartValidLong
in interfaceorg.hl7.fhir.instance.model.api.IIdType
-
isLocal
Returnstrue
if the ID is a local reference (in other words, it begins with the '#' character)- Specified by:
isLocal
in interfaceorg.hl7.fhir.instance.model.api.IIdType
-
isUrn
-
isVersionIdPartValidLong
- Specified by:
isVersionIdPartValidLong
in interfaceorg.hl7.fhir.instance.model.api.IIdType
-
setParts
public org.hl7.fhir.instance.model.api.IIdType setParts(String theBaseUrl, String theResourceType, String theIdPart, String theVersionIdPart) - Specified by:
setParts
in interfaceorg.hl7.fhir.instance.model.api.IIdType
-
toString
- Overrides:
toString
in classPrimitiveType<String>
-
toUnqualified
Returns a new IdType containing this IdType's values but with no server base URL if one is present in this IdType. For example, if this IdType contains the ID "http://foo/Patient/1", this method will return a new IdType containing ID "Patient/1".- Specified by:
toUnqualified
in interfaceorg.hl7.fhir.instance.model.api.IIdType
-
toUnqualifiedVersionless
- Specified by:
toUnqualifiedVersionless
in interfaceorg.hl7.fhir.instance.model.api.IIdType
-
toVersionless
- Specified by:
toVersionless
in interfaceorg.hl7.fhir.instance.model.api.IIdType
-
withResourceType
- Specified by:
withResourceType
in interfaceorg.hl7.fhir.instance.model.api.IIdType
-
withServerBase
Returns a view of this ID as a fully qualified URL, given a server base and resource name (which will only be used if the ID does not already contain those respective parts). Essentially, because IdType can contain either a complete URL or a partial one (or even jut a simple ID), this method may be used to translate into a complete URL.- Specified by:
withServerBase
in interfaceorg.hl7.fhir.instance.model.api.IIdType
- Parameters:
theServerBase
- The server base (e.g. "http://example.com/fhir")theResourceType
- The resource name (e.g. "Patient")- Returns:
- A fully qualified URL for this ID (e.g. "http://example.com/fhir/Patient/1")
-
withVersion
Creates a new instance of this ID which is identical, but refers to the specific version of this resource ID noted by theVersion.- Specified by:
withVersion
in interfaceorg.hl7.fhir.instance.model.api.IIdType
- Parameters:
theVersion
- The actual version string, e.g. "1". If theVersion is blank or null, returns the same astoVersionless()
}- Returns:
- A new instance of IdType which is identical, but refers to the specific version of this resource ID noted by theVersion.
-
newRandomUuid
Construct a new ID with with form "urn:uuid:[UUID]" where [UUID] is a new, randomly created UUID generated byUUID.randomUUID()
-
of
Retrieves the ID from the given resource instance
-
getIdPartAsBigDecimal()
instead (this method was deprocated because its name is ambiguous)