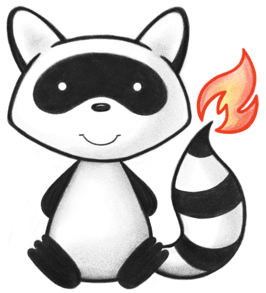
Class FhirInstanceValidator
- All Implemented Interfaces:
ca.uhn.fhir.validation.IInstanceValidatorModule
,ca.uhn.fhir.validation.IValidatorModule
-
Nested Class Summary
Nested Classes -
Constructor Summary
ConstructorsConstructorDescriptionFhirInstanceValidator
(ca.uhn.fhir.context.FhirContext theContext) ConstructorFhirInstanceValidator
(ca.uhn.fhir.context.support.IValidationSupport theValidationSupport) Constructor which uses the given validation support -
Method Summary
Modifier and TypeMethodDescriptionorg.hl7.fhir.r5.utils.validation.constants.BestPracticeWarningLevel
Returns the "best practice" warning level (default isBestPracticeWarningLevel.Hint
).ca.uhn.fhir.context.support.IValidationSupport
Returns thevalidation support
in use by this validator.org.hl7.fhir.r5.utils.validation.IValidationPolicyAdvisor
org.hl7.fhir.r5.utils.validation.IValidatorResourceFetcher
void
Clear any cached data held by the validator or any of its internal stores.boolean
If set to true (default is true) extensions which are not known to the validator (e.g.boolean
boolean
boolean
If set to true (default is false) no binding message is suppressedboolean
If set to true (default is false) no extensible warnings suppressedboolean
If set to true (default is false) the valueSet will not be validateprotected VersionSpecificWorkerContextWrapper
void
setAnyExtensionsAllowed
(boolean theAnyExtensionsAllowed) If set to true (default is true) extensions which are not known to the validator (e.g.void
setAssumeValidRestReferences
(boolean assumeValidRestReferences) void
setBestPracticeWarningLevel
(org.hl7.fhir.r5.utils.validation.constants.BestPracticeWarningLevel theBestPracticeWarningLevel) Sets the "best practice warning level".setCustomExtensionDomains
(String... extensionDomains) Every element in a resource or data type includes an optionalextension child element which is identified by it'surl attribute
.setCustomExtensionDomains
(List<String> extensionDomains) Every element in a resource or data type includes an optionalextension child element which is identified by it'surl attribute
.void
setErrorForUnknownProfiles
(boolean errorForUnknownProfiles) void
setNoBindingMsgSuppressed
(boolean theNoBindingMsgSuppressed) If set to true (default is false) no binding message is suppressedvoid
setNoExtensibleWarnings
(boolean theNoExtensibleWarnings) If set to true (default is false) no extensible warnings is suppressedvoid
setNoTerminologyChecks
(boolean theNoTerminologyChecks) If set to true (default is false) the valueSet will not be validatevoid
setValidationSupport
(ca.uhn.fhir.context.support.IValidationSupport theValidationSupport) Sets thevalidation support
in use by this validator.void
setValidatorPolicyAdvisor
(org.hl7.fhir.r5.utils.validation.IValidationPolicyAdvisor validatorPolicyAdvisor) void
setValidatorResourceFetcher
(org.hl7.fhir.r5.utils.validation.IValidatorResourceFetcher validatorResourceFetcher) protected List
<org.hl7.fhir.utilities.validation.ValidationMessage> validate
(ca.uhn.fhir.validation.IValidationContext<?> theValidationCtx) void
validateResource
(ca.uhn.fhir.validation.IValidationContext<org.hl7.fhir.instance.model.api.IBaseResource> theCtx) Methods inherited from class java.lang.Object
clone, equals, finalize, getClass, hashCode, notify, notifyAll, toString, wait, wait, wait
Methods inherited from interface ca.uhn.fhir.validation.IValidatorModule
validateResource
-
Constructor Details
-
FhirInstanceValidator
ConstructorUses
DefaultProfileValidationSupport
forvalidation support
-
FhirInstanceValidator
Constructor which uses the given validation support- Parameters:
theValidationSupport
- The validation support
-
-
Method Details
-
setCustomExtensionDomains
Every element in a resource or data type includes an optionalextension child element which is identified by it'surl attribute
. There exists a number of predefined extension urls or extension domains:- any url which contains
example.org
,nema.org
, oracme.com
. - any url which starts with
http://hl7.org/fhir/StructureDefinition/
.
Any unknown extension domain will result in an information message when validating a resource.
- any url which contains
-
setCustomExtensionDomains
Every element in a resource or data type includes an optionalextension child element which is identified by it'surl attribute
. There exists a number of predefined extension urls or extension domains:- any url which contains
example.org
,nema.org
, oracme.com
. - any url which starts with
http://hl7.org/fhir/StructureDefinition/
.
Any unknown extension domain will result in an information message when validating a resource.
- any url which contains
-
getBestPracticeWarningLevel
public org.hl7.fhir.r5.utils.validation.constants.BestPracticeWarningLevel getBestPracticeWarningLevel()Returns the "best practice" warning level (default isBestPracticeWarningLevel.Hint
).The FHIR Instance Validator has a number of checks for best practices in terms of FHIR usage. If this setting is set to
BestPracticeWarningLevel.Error
, any resource data which does not meet these best practices will be reported at the ERROR level. If this setting is set toBestPracticeWarningLevel.Ignore
, best practice guielines will be ignored.- See Also:
-
setBestPracticeWarningLevel
public void setBestPracticeWarningLevel(org.hl7.fhir.r5.utils.validation.constants.BestPracticeWarningLevel theBestPracticeWarningLevel) Sets the "best practice warning level". When validating, any deviations from best practices will be reported at this level.The FHIR Instance Validator has a number of checks for best practices in terms of FHIR usage. If this setting is set to
BestPracticeWarningLevel.Error
, any resource data which does not meet these best practices will be reported at the ERROR level. If this setting is set toBestPracticeWarningLevel.Ignore
, best practice guielines will be ignored.- Parameters:
theBestPracticeWarningLevel
- The level, must not benull
-
getValidationSupport
Returns thevalidation support
in use by this validator. Default is an instance of DefaultProfileValidationSupport if the no-arguments constructor for this object was used. -
setValidationSupport
public void setValidationSupport(ca.uhn.fhir.context.support.IValidationSupport theValidationSupport) Sets thevalidation support
in use by this validator. Default is an instance of DefaultProfileValidationSupport if the no-arguments constructor for this object was used. -
isAnyExtensionsAllowed
If set to true (default is true) extensions which are not known to the validator (e.g. because they have not been explicitly declared in a profile) will be validated but will not cause an error. -
setAnyExtensionsAllowed
If set to true (default is true) extensions which are not known to the validator (e.g. because they have not been explicitly declared in a profile) will be validated but will not cause an error. -
isErrorForUnknownProfiles
-
setErrorForUnknownProfiles
-
isNoTerminologyChecks
If set to true (default is false) the valueSet will not be validate -
setNoTerminologyChecks
If set to true (default is false) the valueSet will not be validate -
isNoExtensibleWarnings
If set to true (default is false) no extensible warnings suppressed -
setNoExtensibleWarnings
If set to true (default is false) no extensible warnings is suppressed -
isNoBindingMsgSuppressed
If set to true (default is false) no binding message is suppressed -
setNoBindingMsgSuppressed
If set to true (default is false) no binding message is suppressed -
getExtensionDomains
-
validate
-
provideWorkerContext
-
getWorkerContext
-
getValidatorPolicyAdvisor
-
setValidatorPolicyAdvisor
public void setValidatorPolicyAdvisor(org.hl7.fhir.r5.utils.validation.IValidationPolicyAdvisor validatorPolicyAdvisor) -
getValidatorResourceFetcher
-
setValidatorResourceFetcher
public void setValidatorResourceFetcher(org.hl7.fhir.r5.utils.validation.IValidatorResourceFetcher validatorResourceFetcher) -
isAssumeValidRestReferences
-
setAssumeValidRestReferences
-
invalidateCaches
Clear any cached data held by the validator or any of its internal stores. This is mostly intended for unit tests, but could be used for production uses too. -
validateResource
public void validateResource(ca.uhn.fhir.validation.IValidationContext<org.hl7.fhir.instance.model.api.IBaseResource> theCtx) - Specified by:
validateResource
in interfaceca.uhn.fhir.validation.IValidatorModule
-