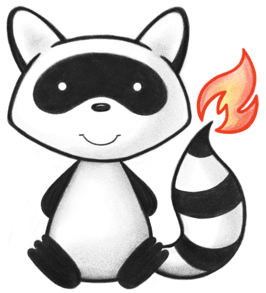
Class ResourceMetadataKeyEnum<T>
- All Implemented Interfaces:
Serializable
- Direct Known Subclasses:
ResourceMetadataKeyEnum.ExtensionResourceMetadataKey
To access or set resource metadata values, every resource has a metadata map, and this class provides convenient getters/setters for interacting with that map. For example, to get a resource's
UPDATED
value, which is the "last updated" time for that resource, use the following code:
InstantDt updated = ResourceMetadataKeyEnum.UPDATED.get(resource);
To set this value, use the following:
InstantDt update = new InstantDt("2011-01-02T11:22:33.0000Z"); // populate with the actual time
ResourceMetadataKeyEnum.UPDATED.put(resource, update);
Note that this class is not a Java Enum, and can therefore be extended (this is why it is not actually an Enum). Users of HAPI-FHIR are able to create their own classes extending this class to define their own keys for storage in resource metadata if needed.
- See Also:
-
Nested Class Summary
Nested ClassesModifier and TypeClassDescriptionstatic final class
-
Field Summary
FieldsModifier and TypeFieldDescriptionstatic final ResourceMetadataKeyEnum
<IPrimitiveType<Date>> If present and populated with a date/time (as an instance ofInstantDt
), this value is an indication that the resource is in the deleted state.static final ResourceMetadataKeyEnum
<BundleEntrySearchModeEnum> If present and populated with aBundleEntrySearchModeEnum
, contains the "bundle entry search mode", which is the value of the status field in the Bundle entry containing this resource.static final ResourceMetadataKeyEnum
<BigDecimal> If present and populated with a decimal value, contains the "bundle entry search score", which is the value of the status field in the Bundle entry containing this resource.static final ResourceMetadataKeyEnum
<BundleEntryTransactionMethodEnum> If present and populated with aBundleEntryTransactionMethodEnum
, contains the "bundle entry transaction operation", which is the value of the status field in the Bundle entry containing this resource.static final ResourceMetadataKeyEnum
<List<IdDt>> The value for this key represents aList
of profile IDs that this resource claims to conform to.static final ResourceMetadataKeyEnum
<InstantDt> The value for this key is the bundle entry Published time.static final ResourceMetadataKeyEnum
<List<BaseCodingDt>> static final ResourceMetadataKeyEnum
<TagList> The value for this key is the list of tags associated with this resourcestatic final ResourceMetadataKeyEnum
<InstantDt> The value for this key is the bundle entry Updated time.static final ResourceMetadataKeyEnum
<String> Deprecated.static final ResourceMetadataKeyEnum
<IdDt> Deprecated.TheIResource.getId()
resource ID will now be populated with the version ID via theIdDt.getVersionIdPart()
method -
Constructor Summary
Constructors -
Method Summary
-
Field Details
-
DELETED_AT
If present and populated with a date/time (as an instance ofInstantDt
), this value is an indication that the resource is in the deleted state. This key is only used in a limited number of scenarios, such as POSTing transaction bundles to a server, or returning resource history.Values for this key are of type
InstantDt
-
ENTRY_SEARCH_MODE
If present and populated with aBundleEntrySearchModeEnum
, contains the "bundle entry search mode", which is the value of the status field in the Bundle entry containing this resource. The value for this key corresponds to fieldBundle.entry.search.mode
. This value can be set to provide a status value of "include" for included resources being returned by a server, or to "match" to indicate that the resource was returned because it matched the given search criteria.Note that status is only used in FHIR DSTU2 and later.
Values for this key are of type
BundleEntrySearchModeEnum
-
ENTRY_SEARCH_SCORE
If present and populated with a decimal value, contains the "bundle entry search score", which is the value of the status field in the Bundle entry containing this resource. The value for this key corresponds to fieldBundle.entry.search.score
. This value represents the search ranking score, where 1.0 is relevant and 0.0 is irrelevant.Note that status is only used in FHIR DSTU2 and later.
-
ENTRY_TRANSACTION_METHOD
public static final ResourceMetadataKeyEnum<BundleEntryTransactionMethodEnum> ENTRY_TRANSACTION_METHODIf present and populated with aBundleEntryTransactionMethodEnum
, contains the "bundle entry transaction operation", which is the value of the status field in the Bundle entry containing this resource. The value for this key corresponds to fieldBundle.entry.transaction.operation
. This value can be set in resources being transmitted to a server to provide a status value of "create" or "update" to indicate behaviour the server should observe. It may also be set to similar values (or to "noop") in resources being returned by a server as a result of a transaction to indicate to the client what operation was actually performed.Note that status is only used in FHIR DSTU2 and later.
Values for this key are of type
BundleEntryTransactionMethodEnum
-
PROFILES
The value for this key represents aList
of profile IDs that this resource claims to conform to.Values for this key are of type List<IdDt>. Note that the returned list is unmodifiable, so you need to create a new list and call
put
to change its value. -
PUBLISHED
The value for this key is the bundle entry Published time. This is defined by FHIR as "Time resource copied into the feed", which is generally best left to the current time.Values for this key are of type
InstantDt
Server Note: In servers, it is generally advisable to leave this value
null
, in which case the server will substitute the current time automatically.- See Also:
-
SECURITY_LABELS
-
TAG_LIST
The value for this key is the list of tags associated with this resourceValues for this key are of type
TagList
- See Also:
-
UPDATED
The value for this key is the bundle entry Updated time. This is defined by FHIR as "Last Updated for resource". This value is also used for populating the "Last-Modified" header in the case of methods that return a single resource (read, vread, etc.)Values for this key are of type
InstantDt
- See Also:
-
VERSION
Deprecated.TheIResource.getId()
resource ID will now be populated with the version ID via theIdDt.getVersionIdPart()
methodThe value for this key is the version ID of the resource object.Values for this key are of type
String
-
VERSION_ID
Deprecated.TheIResource.getId()
resource ID will now be populated with the version ID via theIdDt.getVersionIdPart()
methodThe value for this key is the version ID of the resource object.Values for this key are of type
IdDt
-
-
Constructor Details
-
ResourceMetadataKeyEnum
-
-
Method Details
IResource.getId()
resource ID will now be populated with the version ID via theIdDt.getVersionIdPart()
method