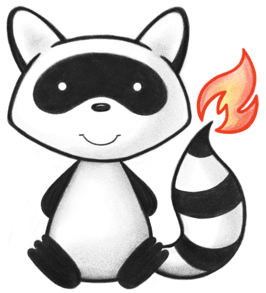
Class DateRangeParam
- All Implemented Interfaces:
IQueryParameterAnd<DateParam>
,Serializable
- Direct Known Subclasses:
HistorySearchDateRangeParam
- See Also:
-
Constructor Summary
ConstructorsConstructorDescriptionBasic constructor.DateRangeParam
(DateParam theDateParam) Sets the range from a single date param.DateRangeParam
(DateParam theLowerBound, DateParam theUpperBound) Constructor which takes two Dates representing the lower and upper bounds of the range (inclusive on both ends)DateRangeParam
(DateRangeParam theDateRangeParam) Copy constructor.DateRangeParam
(String theLowerBound, String theUpperBound) Constructor which takes two strings representing the lower and upper bounds of the range (inclusive on both ends)DateRangeParam
(Date theLowerBound, Date theUpperBound) Constructor which takes two Dates representing the lower and upper bounds of the range (inclusive on both ends)DateRangeParam
(IPrimitiveType<Date> theLowerBound, IPrimitiveType<Date> theUpperBound) Constructor which takes two Dates representing the lower and upper bounds of the range (inclusive on both ends) -
Method Summary
Modifier and TypeMethodDescriptionboolean
Return the current lower bound as an integer representative of the date.Return the current upper bound as an integer representative of the date e.g.See FHIR specification 2.2.2 Search SearchParameter Types for information on the token formatint
hashCode()
boolean
isEmpty()
setLowerBound
(DateParam theLowerBound) setLowerBound
(String theLowerBound) Sets the lower bound using a string that is compliant with FHIR dateTime format (ISO-8601).setLowerBoundExclusive
(Date theLowerBound) Sets the lower bound to be greaterthan to the given datesetLowerBoundInclusive
(Date theLowerBound) Sets the lower bound to be greaterthan or equal to the given datevoid
setRangeFromDatesInclusive
(DateParam theLowerBound, DateParam theUpperBound) Sets the range from a pair of dates, inclusive on both endsvoid
setRangeFromDatesInclusive
(String theLowerBound, String theUpperBound) Sets the range from a pair of dates, inclusive on both endsvoid
setRangeFromDatesInclusive
(Date theLowerBound, Date theUpperBound) Sets the range from a pair of dates, inclusive on both endsvoid
setRangeFromDatesInclusive
(IPrimitiveType<Date> theLowerBound, IPrimitiveType<Date> theUpperBound) Sets the range from a pair of dates, inclusive on both ends.setUpperBound
(DateParam theUpperBound) setUpperBound
(String theUpperBound) Sets the upper bound using a string that is compliant with FHIR dateTime format (ISO-8601).setUpperBoundExclusive
(Date theUpperBound) Sets the upper bound to be greaterthan to the given datesetUpperBoundInclusive
(Date theUpperBound) Sets the upper bound to be greaterthan or equal to the given datevoid
setValuesAsQueryTokens
(FhirContext theContext, String theParamName, List<QualifiedParamList> theParameters) See FHIR specification 2.2.2 Search SearchParameter Types for information on the token formattoString()
-
Constructor Details
-
DateRangeParam
public DateRangeParam()Basic constructor. Values must be supplied by callingsetLowerBound(DateParam)
andsetUpperBound(DateParam)
-
DateRangeParam
Copy constructor. -
DateRangeParam
Constructor which takes two Dates representing the lower and upper bounds of the range (inclusive on both ends)- Parameters:
theLowerBound
- A qualified date param representing the lower date bound (optionally may include time), e.g. "2011-02-22" or "2011-02-22T13:12:00Z". Will be treated inclusively. Either theLowerBound or theUpperBound may both be populated, or one may be null, but it is not valid for both to be null.theUpperBound
- A qualified date param representing the upper date bound (optionally may include time), e.g. "2011-02-22" or "2011-02-22T13:12:00Z". Will be treated inclusively. Either theLowerBound or theUpperBound may both be populated, or one may be null, but it is not valid for both to be null.
-
DateRangeParam
Sets the range from a single date param. If theDateParam has no qualifier, treats it as the lower and upper bound (e.g. 2011-01-02 would match any time on that day). If theDateParam has a qualifier, treats it as either the lower or upper bound, with no opposite bound. -
DateRangeParam
Constructor which takes two Dates representing the lower and upper bounds of the range (inclusive on both ends)- Parameters:
theLowerBound
- A qualified date param representing the lower date bound (optionally may include time), e.g. "2011-02-22" or "2011-02-22T13:12:00Z". Will be treated inclusively. Either theLowerBound or theUpperBound may both be populated, or one may be null, but it is not valid for both to be null.theUpperBound
- A qualified date param representing the upper date bound (optionally may include time), e.g. "2011-02-22" or "2011-02-22T13:12:00Z". Will be treated inclusively. Either theLowerBound or theUpperBound may both be populated, or one may be null, but it is not valid for both to be null.
-
DateRangeParam
Constructor which takes two Dates representing the lower and upper bounds of the range (inclusive on both ends)- Parameters:
theLowerBound
- A qualified date param representing the lower date bound (optionally may include time), e.g. "2011-02-22" or "2011-02-22T13:12:00Z". Will be treated inclusively. Either theLowerBound or theUpperBound may both be populated, or one may be null, but it is not valid for both to be null.theUpperBound
- A qualified date param representing the upper date bound (optionally may include time), e.g. "2011-02-22" or "2011-02-22T13:12:00Z". Will be treated inclusively. Either theLowerBound or theUpperBound may both be populated, or one may be null, but it is not valid for both to be null.
-
DateRangeParam
Constructor which takes two strings representing the lower and upper bounds of the range (inclusive on both ends)- Parameters:
theLowerBound
- An unqualified date param representing the lower date bound (optionally may include time), e.g. "2011-02-22" or "2011-02-22T13:12:00Z". Either theLowerBound or theUpperBound may both be populated, or one may be null, but it is not valid for both to be null.theUpperBound
- An unqualified date param representing the upper date bound (optionally may include time), e.g. "2011-02-22" or "2011-02-22T13:12:00Z". Either theLowerBound or theUpperBound may both be populated, or one may be null, but it is not valid for both to be null.
-
-
Method Details
-
equals
-
getLowerBound
-
setLowerBound
-
setLowerBound
Sets the lower bound using a string that is compliant with FHIR dateTime format (ISO-8601).This lower bound is assumed to have a
ge
(greater than or equals) modifier.Note: An operation can take a DateRangeParam. If only a single date is provided, it will still result in a DateRangeParam where the lower and upper bounds are the same value. As such, even though the prefixes for the lower and upper bounds default to
ge
andle
respectively, the resulting prefix is effectivelyeq
where only a single date is provided - as required by the FHIR specification (i.e. "If no prefix is present, the prefixeq
is assumed"). -
setLowerBoundInclusive
Sets the lower bound to be greaterthan or equal to the given date -
setUpperBoundInclusive
Sets the upper bound to be greaterthan or equal to the given date -
setLowerBoundExclusive
Sets the lower bound to be greaterthan to the given date -
setUpperBoundExclusive
Sets the upper bound to be greaterthan to the given date -
getLowerBoundAsDateInteger
Return the current lower bound as an integer representative of the date. e.g. 2019-02-22T04:22:00-0500 -> 20120922 -
getUpperBoundAsDateInteger
Return the current upper bound as an integer representative of the date e.g. 2019-02-22T04:22:00-0500 -> 2019122 -
getLowerBoundAsInstant
-
getUpperBound
-
setUpperBound
Sets the upper bound using a string that is compliant with FHIR dateTime format (ISO-8601).This upper bound is assumed to have a
le
(less than or equals) modifier.Note: An operation can take a DateRangeParam. If only a single date is provided, it will still result in a DateRangeParam where the lower and upper bounds are the same value. As such, even though the prefixes for the lower and upper bounds default to
ge
andle
respectively, the resulting prefix is effectivelyeq
where only a single date is provided - as required by the FHIR specificiation (i.e. "If no prefix is present, the prefixeq
is assumed"). -
setUpperBound
-
getUpperBoundAsInstant
-
getValuesAsQueryTokens
Description copied from interface:IQueryParameterAnd
See FHIR specification 2.2.2 Search SearchParameter Types for information on the token format
- Specified by:
getValuesAsQueryTokens
in interfaceIQueryParameterAnd<DateParam>
-
hashCode
-
isEmpty
-
setRangeFromDatesInclusive
Sets the range from a pair of dates, inclusive on both ends- Parameters:
theLowerBound
- A qualified date param representing the lower date bound (optionally may include time), e.g. "2011-02-22" or "2011-02-22T13:12:00Z". Will be treated inclusively. Either theLowerBound or theUpperBound may both be populated, or one may be null, but it is not valid for both to be null.theUpperBound
- A qualified date param representing the upper date bound (optionally may include time), e.g. "2011-02-22" or "2011-02-22T13:12:00Z". Will be treated inclusively. Either theLowerBound or theUpperBound may both be populated, or one may be null, but it is not valid for both to be null.
-
setRangeFromDatesInclusive
Sets the range from a pair of dates, inclusive on both ends- Parameters:
theLowerBound
- A qualified date param representing the lower date bound (optionally may include time), e.g. "2011-02-22" or "2011-02-22T13:12:00Z". Will be treated inclusively. Either theLowerBound or theUpperBound may both be populated, or one may be null, but it is not valid for both to be null.theUpperBound
- A qualified date param representing the upper date bound (optionally may include time), e.g. "2011-02-22" or "2011-02-22T13:12:00Z". Will be treated inclusively. Either theLowerBound or theUpperBound may both be populated, or one may be null, but it is not valid for both to be null.
-
setRangeFromDatesInclusive
public void setRangeFromDatesInclusive(IPrimitiveType<Date> theLowerBound, IPrimitiveType<Date> theUpperBound) Sets the range from a pair of dates, inclusive on both ends. Note that if theLowerBound is after theUpperBound, thie method will automatically reverse the order of the arguments in order to create an inclusive range.- Parameters:
theLowerBound
- A qualified date param representing the lower date bound (optionally may include time), e.g. "2011-02-22" or "2011-02-22T13:12:00Z". Will be treated inclusively. Either theLowerBound or theUpperBound may both be populated, or one may be null, but it is not valid for both to be null.theUpperBound
- A qualified date param representing the upper date bound (optionally may include time), e.g. "2011-02-22" or "2011-02-22T13:12:00Z". Will be treated inclusively. Either theLowerBound or theUpperBound may both be populated, or one may be null, but it is not valid for both to be null.
-
setRangeFromDatesInclusive
Sets the range from a pair of dates, inclusive on both ends- Parameters:
theLowerBound
- A qualified date param representing the lower date bound (optionally may include time), e.g. "2011-02-22" or "2011-02-22T13:12:00Z". Will be treated inclusively. Either theLowerBound or theUpperBound may both be populated, or one may be null, but it is not valid for both to be null.theUpperBound
- A qualified date param representing the upper date bound (optionally may include time), e.g. "2011-02-22" or "2011-02-22T13:12:00Z". Will be treated inclusively. Either theLowerBound or theUpperBound may both be populated, or one may be null, but it is not valid for both to be null.
-
setValuesAsQueryTokens
public void setValuesAsQueryTokens(FhirContext theContext, String theParamName, List<QualifiedParamList> theParameters) throws InvalidRequestException Description copied from interface:IQueryParameterAnd
See FHIR specification 2.2.2 Search SearchParameter Types for information on the token format
- Specified by:
setValuesAsQueryTokens
in interfaceIQueryParameterAnd<DateParam>
- Parameters:
theContext
- TODOtheParamName
- TODO- Throws:
InvalidRequestException
-
toString
-