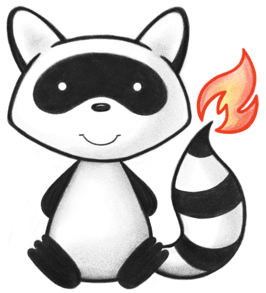
Package ca.uhn.fhir.util
Class TerserUtil
java.lang.Object
ca.uhn.fhir.util.TerserUtil
-
Field Summary
FieldsModifier and TypeFieldDescriptionstatic final String
Exclusion predicate for id, identifier, meta fields.static final Predicate
<org.apache.commons.lang3.tuple.Triple<BaseRuntimeChildDefinition, IBase, IBase>> Exclusion predicate for id/identifier, meta and fields with empty values.static final String
static final Collection
<String> Exclude for id, identifier and meta fields of a resource.Exclusion predicate for keeping all fields. -
Method Summary
Modifier and TypeMethodDescriptionstatic void
clearField
(FhirContext theFhirContext, String theFieldName, IBase theBase) Clears the specified field on the element providedstatic void
clearField
(FhirContext theFhirContext, IBaseResource theResource, String theFieldName) Clears the specified field on the resource providedstatic void
clearFieldByFhirPath
(FhirContext theFhirContext, IBaseResource theResource, String theFhirPath) Clears the specified field on the resource provided by the FHIRPath.static <T extends IBaseResource>
Tclone
(FhirContext theFhirContext, T theInstance) Clones the specified resource.static void
cloneCompositeField
(FhirContext theFhirContext, IBaseResource theFrom, IBaseResource theTo, String theField) Clones specified composite field (collection).static void
cloneIdentifierIntoResource
(FhirContext theFhirContext, BaseRuntimeChildDefinition theIdentifierDefinition, IBase theNewIdentifier, IBaseResource theResourceToCloneInto) Given an Child Definition of `identifier`, a R4/DSTU3 Identifier, and a new resource, clone the identifier into that resources' identifier list if it is not already present.static boolean
Checks if two items are equal viaEQUALS_DEEP
methodstatic boolean
fieldExists
(FhirContext theFhirContext, String theFieldName, IBaseResource theInstance) Checks if the field exists on the resourcegetFieldByFhirPath
(FhirContext theFhirContext, String theFhirPath, IBase theResource) Returns field values ant the specified FHIR path from the resource.static IBase
getFirstFieldByFhirPath
(FhirContext theFhirContext, String theFhirPath, IBase theResource) Returns the first available field value at the specified FHIR path from the resource.static IBase
getValueFirstRep
(FhirContext theFhirContext, IBaseResource theResource, String theFieldName) Gets the first available value for the specified field.getValues
(FhirContext theFhirContext, IBaseResource theResource, String theFieldName) Gets all values of the specified field.static boolean
hasValues
(FhirContext theFhirContext, IBaseResource theResource, String theFieldName) Checks if the specified fields has any valuesstatic IBaseBackboneElement
instantiateBackboneElement
(FhirContext theFhirContext, String theTargetResourceName, String theTargetFieldName) Creates a new BackboneElement.static void
mergeAllFields
(FhirContext theFhirContext, IBaseResource theFrom, IBaseResource theTo) Merges all fields on the provided instance.static void
mergeField
(FhirContext theFhirContext, FhirTerser theTerser, String theFieldName, IBaseResource theFrom, IBaseResource theTo) Merges value of the specified field fromtheFrom
resource totheTo
resource.static void
mergeField
(FhirContext theFhirContext, String theFieldName, IBaseResource theFrom, IBaseResource theTo) Merges value of the specified field fromtheFrom
resource totheTo
resource.static void
mergeFields
(FhirContext theFhirContext, IBaseResource theFrom, IBaseResource theTo, Predicate<String> inclusionStrategy) Merges values of all field fromtheFrom
resource totheTo
resource.static void
mergeFieldsExceptIdAndMeta
(FhirContext theFhirContext, IBaseResource theFrom, IBaseResource theTo) Merges values of all fields except for "identifier" and "meta" fromtheFrom
resource totheTo
resource.static <T extends IBase>
TnewElement
(FhirContext theFhirContext, String theElementType) Creates a new element instancestatic <T extends IBase>
TnewElement
(FhirContext theFhirContext, String theElementType, Object theConstructorParam) Creates a new element instancestatic <T extends IBase>
TnewResource
(FhirContext theFhirContext, String theResourceName) Creates a new resource definition.static <T extends IBase>
TnewResource
(FhirContext theFhirContext, String theResourceName, Object theConstructorParam) Creates a new resource definition.static void
replaceField
(FhirContext theFhirContext, String theFieldName, IBaseResource theFrom, IBaseResource theTo) Replaces the specified fields ontheTo
resource with the value fromtheFrom
resource.static void
replaceFields
(FhirContext theFhirContext, IBaseResource theFrom, IBaseResource theTo, Predicate<String> theFieldNameInclusion) Replaces all fields that have matching field names by the given inclusion strategy.static void
replaceFieldsByPredicate
(FhirContext theFhirContext, IBaseResource theFrom, IBaseResource theTo, Predicate<org.apache.commons.lang3.tuple.Triple<BaseRuntimeChildDefinition, IBase, IBase>> thePredicate) Replaces fields on theTo resource that test positive by the given predicate.static void
setField
(FhirContext theFhirContext, FhirTerser theTerser, String theFieldName, IBaseResource theResource, IBase... theValues) Sets the provided field with the given values.static void
setField
(FhirContext theFhirContext, String theFieldName, IBaseResource theResource, IBase... theValues) Sets the provided field with the given values.static void
setFieldByFhirPath
(FhirContext theFhirContext, String theFhirPath, IBaseResource theResource, IBase theValue) Sets the specified value at the FHIR path provided.static void
setFieldByFhirPath
(FhirTerser theTerser, String theFhirPath, IBaseResource theResource, IBase theValue) Sets the specified value at the FHIR path provided.static void
setStringField
(FhirContext theFhirContext, String theFieldName, IBaseResource theResource, String theValue) Sets the provided field with the given values.static void
setStringFieldByFhirPath
(FhirContext theFhirContext, String theFhirPath, IBaseResource theResource, String theValue) Sets the specified String value at the FHIR path provided.
-
Field Details
-
FIELD_NAME_IDENTIFIER
- See Also:
-
IDS_AND_META_EXCLUDES
Exclude for id, identifier and meta fields of a resource. -
EXCLUDE_IDS_AND_META
Exclusion predicate for id, identifier, meta fields. -
EXCLUDE_IDS_META_AND_EMPTY
public static final Predicate<org.apache.commons.lang3.tuple.Triple<BaseRuntimeChildDefinition,IBase, EXCLUDE_IDS_META_AND_EMPTYIBase>> Exclusion predicate for id/identifier, meta and fields with empty values. This ensures that source / target resources, empty source fields will not results in erasure of target fields. -
INCLUDE_ALL
Exclusion predicate for keeping all fields. -
DATA_ABSENT_REASON_EXTENSION_URI
- See Also:
-
-
Method Details
-
cloneIdentifierIntoResource
public static void cloneIdentifierIntoResource(FhirContext theFhirContext, BaseRuntimeChildDefinition theIdentifierDefinition, IBase theNewIdentifier, IBaseResource theResourceToCloneInto) Given an Child Definition of `identifier`, a R4/DSTU3 Identifier, and a new resource, clone the identifier into that resources' identifier list if it is not already present. -
hasValues
public static boolean hasValues(FhirContext theFhirContext, IBaseResource theResource, String theFieldName) Checks if the specified fields has any values- Parameters:
theFhirContext
- Context holding resource definitiontheResource
- Resource to check if the specified field is settheFieldName
- name of the field to check- Returns:
- Returns true if field exists and has any values set, and false otherwise
-
getValues
public static List<IBase> getValues(FhirContext theFhirContext, IBaseResource theResource, String theFieldName) Gets all values of the specified field.- Parameters:
theFhirContext
- Context holding resource definitiontheResource
- Resource to check if the specified field is settheFieldName
- name of the field to check- Returns:
- Returns all values for the specified field or null if field with the provided name doesn't exist
-
getValueFirstRep
public static IBase getValueFirstRep(FhirContext theFhirContext, IBaseResource theResource, String theFieldName) Gets the first available value for the specified field.- Parameters:
theFhirContext
- Context holding resource definitiontheResource
- Resource to check if the specified field is settheFieldName
- name of the field to check- Returns:
- Returns the first value for the specified field or null if field with the provided name doesn't exist or has no values
-
cloneCompositeField
public static void cloneCompositeField(FhirContext theFhirContext, IBaseResource theFrom, IBaseResource theTo, String theField) Clones specified composite field (collection). Composite field values must conform to the collections contract.- Parameters:
theFrom
- Resource to clone the specified field fromtheTo
- Resource to clone the specified field totheField
- Field name to be copied
-
equals
Checks if two items are equal viaEQUALS_DEEP
method- Parameters:
theItem1
- First item to comparetheItem2
- Second item to compare- Returns:
- Returns true if they are equal and false otherwise
-
mergeAllFields
public static void mergeAllFields(FhirContext theFhirContext, IBaseResource theFrom, IBaseResource theTo) Merges all fields on the provided instance.theTo
will contain a union of all values fromtheFrom
instance andtheTo
instance.- Parameters:
theFhirContext
- Context holding resource definitiontheFrom
- The resource to merge the fields fromtheTo
- The resource to merge the fields into
-
replaceFields
public static void replaceFields(FhirContext theFhirContext, IBaseResource theFrom, IBaseResource theTo, Predicate<String> theFieldNameInclusion) Replaces all fields that have matching field names by the given inclusion strategy.theTo
will contain a copy of the values fromtheFrom
instance.- Parameters:
theFhirContext
- Context holding resource definitiontheFrom
- The resource to merge the fields fromtheTo
- The resource to merge the fields intotheFieldNameInclusion
- Inclusion strategy that checks if a given field should be replaced
-
replaceFieldsByPredicate
public static void replaceFieldsByPredicate(FhirContext theFhirContext, IBaseResource theFrom, IBaseResource theTo, Predicate<org.apache.commons.lang3.tuple.Triple<BaseRuntimeChildDefinition, IBase, IBase>> thePredicate) Replaces fields on theTo resource that test positive by the given predicate.theTo
will contain a copy of the values fromtheFrom
for which predicate tests positive. Please note that composite fields will be replaced fully.- Parameters:
theFhirContext
- Context holding resource definitiontheFrom
- The resource to merge the fields fromtheTo
- The resource to merge the fields intothePredicate
- Predicate that checks if a given field should be replaced
-
fieldExists
public static boolean fieldExists(FhirContext theFhirContext, String theFieldName, IBaseResource theInstance) Checks if the field exists on the resource- Parameters:
theFhirContext
- Context holding resource definitiontheFieldName
- Name of the field to checktheInstance
- Resource instance to check- Returns:
- Returns true if resource definition has a child with the specified name and false otherwise
-
replaceField
public static void replaceField(FhirContext theFhirContext, String theFieldName, IBaseResource theFrom, IBaseResource theTo) Replaces the specified fields ontheTo
resource with the value fromtheFrom
resource.- Parameters:
theFhirContext
- Context holding resource definitiontheFrom
- The resource to replace the field fromtheTo
- The resource to replace the field on
-
clearField
public static void clearField(FhirContext theFhirContext, IBaseResource theResource, String theFieldName) Clears the specified field on the resource provided- Parameters:
theFhirContext
- Context holding resource definitiontheResource
-theFieldName
-
-
clearFieldByFhirPath
public static void clearFieldByFhirPath(FhirContext theFhirContext, IBaseResource theResource, String theFhirPath) Clears the specified field on the resource provided by the FHIRPath. If more than one value matches the FHIRPath, all values will be cleared.- Parameters:
theFhirContext
-theResource
-theFhirPath
-
-
clearField
Clears the specified field on the element provided- Parameters:
theFhirContext
- Context holding resource definitiontheFieldName
- Name of the field to clear values fortheBase
- The element definition to clear values on
-
setField
public static void setField(FhirContext theFhirContext, String theFieldName, IBaseResource theResource, IBase... theValues) Sets the provided field with the given values. This method will add to the collection of existing field values in case of multiple cardinality. UseclearField(FhirContext, IBaseResource, String)
to remove values before setting- Parameters:
theFhirContext
- Context holding resource definitiontheFieldName
- Child field name of the resource to settheResource
- The resource to set the values ontheValues
- The values to set on the resource child field name
-
setField
public static void setField(FhirContext theFhirContext, FhirTerser theTerser, String theFieldName, IBaseResource theResource, IBase... theValues) Sets the provided field with the given values. This method will add to the collection of existing field values in case of multiple cardinality. UseclearField(FhirContext, IBaseResource, String)
to remove values before setting- Parameters:
theFhirContext
- Context holding resource definitiontheTerser
- Terser to be used when cloning field valuestheFieldName
- Child field name of the resource to settheResource
- The resource to set the values ontheValues
- The values to set on the resource child field name
-
setStringField
public static void setStringField(FhirContext theFhirContext, String theFieldName, IBaseResource theResource, String theValue) Sets the provided field with the given values. This method will add to the collection of existing field values in case of multiple cardinality. UseclearField(FhirContext, IBaseResource, String)
to remove values before setting- Parameters:
theFhirContext
- Context holding resource definitiontheFieldName
- Child field name of the resource to settheResource
- The resource to set the values ontheValue
- The String value to set on the resource child field name. This value is converted to the appropriate primitive type before the value is set
-
setFieldByFhirPath
public static void setFieldByFhirPath(FhirTerser theTerser, String theFhirPath, IBaseResource theResource, IBase theValue) Sets the specified value at the FHIR path provided.- Parameters:
theTerser
- The terser that should be used for cloning the field value.theFhirPath
- The FHIR path to set the field attheResource
- The resource on which the value should be settheValue
- The value to set
-
setFieldByFhirPath
public static void setFieldByFhirPath(FhirContext theFhirContext, String theFhirPath, IBaseResource theResource, IBase theValue) Sets the specified value at the FHIR path provided.- Parameters:
theFhirContext
- Context holding resource definitiontheFhirPath
- The FHIR path to set the field attheResource
- The resource on which the value should be settheValue
- The value to set
-
setStringFieldByFhirPath
public static void setStringFieldByFhirPath(FhirContext theFhirContext, String theFhirPath, IBaseResource theResource, String theValue) Sets the specified String value at the FHIR path provided.- Parameters:
theFhirContext
- Context holding resource definitiontheFhirPath
- The FHIR path to set the field attheResource
- The resource on which the value should be settheValue
- The String value to set. The string is converted to the appropriate primitive type before setting the field
-
getFieldByFhirPath
public static List<IBase> getFieldByFhirPath(FhirContext theFhirContext, String theFhirPath, IBase theResource) Returns field values ant the specified FHIR path from the resource.- Parameters:
theFhirContext
- Context holding resource definitiontheFhirPath
- The FHIR path to get the field fromtheResource
- The resource from which the value should be retrieved- Returns:
- Returns the list of field values at the given FHIR path
-
getFirstFieldByFhirPath
public static IBase getFirstFieldByFhirPath(FhirContext theFhirContext, String theFhirPath, IBase theResource) Returns the first available field value at the specified FHIR path from the resource.- Parameters:
theFhirContext
- Context holding resource definitiontheFhirPath
- The FHIR path to get the field fromtheResource
- The resource from which the value should be retrieved- Returns:
- Returns the first available value or null if no values can be retrieved
-
mergeFieldsExceptIdAndMeta
public static void mergeFieldsExceptIdAndMeta(FhirContext theFhirContext, IBaseResource theFrom, IBaseResource theTo) Merges values of all fields except for "identifier" and "meta" fromtheFrom
resource totheTo
resource. Fields values are compared via the equalsDeep method, or via object identity if this method is not available.- Parameters:
theFhirContext
- Context holding resource definitiontheFrom
- Resource to merge the specified field fromtheTo
- Resource to merge the specified field into
-
mergeFields
public static void mergeFields(FhirContext theFhirContext, IBaseResource theFrom, IBaseResource theTo, Predicate<String> inclusionStrategy) Merges values of all field fromtheFrom
resource totheTo
resource. Fields values are compared via the equalsDeep method, or via object identity if this method is not available.- Parameters:
theFhirContext
- Context holding resource definitiontheFrom
- Resource to merge the specified field fromtheTo
- Resource to merge the specified field intoinclusionStrategy
- Predicate to test which fields should be merged
-
mergeField
public static void mergeField(FhirContext theFhirContext, String theFieldName, IBaseResource theFrom, IBaseResource theTo) Merges value of the specified field fromtheFrom
resource totheTo
resource. Fields values are compared via the equalsDeep method, or via object identity if this method is not available.- Parameters:
theFhirContext
- Context holding resource definitiontheFieldName
- Name of the child filed to mergetheFrom
- Resource to merge the specified field fromtheTo
- Resource to merge the specified field into
-
mergeField
public static void mergeField(FhirContext theFhirContext, FhirTerser theTerser, String theFieldName, IBaseResource theFrom, IBaseResource theTo) Merges value of the specified field fromtheFrom
resource totheTo
resource. Fields values are compared via the equalsDeep method, or via object identity if this method is not available.- Parameters:
theFhirContext
- Context holding resource definitiontheTerser
- Terser to be used when cloning the field valuestheFieldName
- Name of the child filed to mergetheFrom
- Resource to merge the specified field fromtheTo
- Resource to merge the specified field into
-
clone
Clones the specified resource.- Type Parameters:
T
- Base resource type- Parameters:
theFhirContext
- Context holding resource definitiontheInstance
- The instance to be cloned- Returns:
- Returns a cloned instance
-
newElement
Creates a new element instance- Type Parameters:
T
- Base element type- Parameters:
theFhirContext
- Context holding resource definitiontheElementType
- Element type name- Returns:
- Returns a new instance of the element
-
newElement
public static <T extends IBase> T newElement(FhirContext theFhirContext, String theElementType, Object theConstructorParam) Creates a new element instance- Type Parameters:
T
- Base element type- Parameters:
theFhirContext
- Context holding resource definitiontheElementType
- Element type nametheConstructorParam
- Initialization parameter for the element- Returns:
- Returns a new instance of the element with the specified initial value
-
newResource
Creates a new resource definition.- Type Parameters:
T
- Type of the resource- Parameters:
theFhirContext
- Context holding resource definitiontheResourceName
- Name of the resource in the context- Returns:
- Returns a new instance of the resource
-
newResource
public static <T extends IBase> T newResource(FhirContext theFhirContext, String theResourceName, Object theConstructorParam) Creates a new resource definition.- Type Parameters:
T
- Type of the resource- Parameters:
theFhirContext
- Context holding resource definitiontheResourceName
- Name of the resource in the contexttheConstructorParam
- Initialization parameter for the new instance- Returns:
- Returns a new instance of the resource
-
instantiateBackboneElement
public static IBaseBackboneElement instantiateBackboneElement(FhirContext theFhirContext, String theTargetResourceName, String theTargetFieldName) Creates a new BackboneElement.- Parameters:
theFhirContext
- Context holding resource definitiontheTargetResourceName
- Name of the resource in the contexttheTargetFieldName
- Name of the backbone element in the resource- Returns:
- Returns a new instance of the element
-