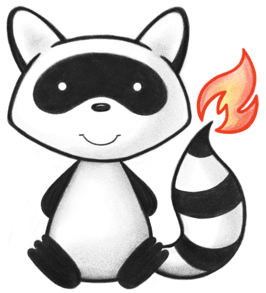
Package ca.uhn.fhir.rest.server.provider
Class HashMapResourceProvider<T extends org.hl7.fhir.instance.model.api.IBaseResource>
java.lang.Object
ca.uhn.fhir.rest.server.provider.HashMapResourceProvider<T>
- Type Parameters:
T
- The resource type to support
- All Implemented Interfaces:
IResourceProvider
public class HashMapResourceProvider<T extends org.hl7.fhir.instance.model.api.IBaseResource>
extends Object
implements IResourceProvider
This class is a simple implementation of the resource provider
interface that uses a HashMap to store all resources in memory.
This class currently supports the following FHIR operations:
- Create
- Update existing resource
- Update non-existing resource (e.g. create with client-supplied ID)
- Delete
- Search by resource type with no parameters
-
Field Summary
FieldsModifier and TypeFieldDescriptionprotected Map
<String, LinkedList<T>> protected AtomicLong
protected LinkedList
<T> -
Constructor Summary
ConstructorsConstructorDescriptionHashMapResourceProvider
(ca.uhn.fhir.context.FhirContext theFhirContext, Class<T> theResourceType) Constructor -
Method Summary
Modifier and TypeMethodDescriptionvoid
clear()
Clear all data held in this resource providervoid
Clear the counts used bygetCountRead()
and other count methodsca.uhn.fhir.rest.api.MethodOutcome
create
(T theResource, RequestDetails theRequestDetails) ca.uhn.fhir.rest.api.MethodOutcome
delete
(org.hl7.fhir.instance.model.api.IIdType theId, RequestDetails theRequestDetails) protected static <T extends org.hl7.fhir.instance.model.api.IBaseResource>
List<org.hl7.fhir.instance.model.api.IBaseResource> fireInterceptorsAndFilterAsNeeded
(List<T> theResources, RequestDetails theRequestDetails) long
This method returns a simple operation count.long
This method returns a simple operation count.long
This method returns a simple operation count.long
This method returns a simple operation count.long
This method returns a simple operation count.ca.uhn.fhir.context.FhirContext
Returns the type of resource returned by this providerReturns an unmodifiable list containing the current version of all resources stored in this providerList
<org.hl7.fhir.instance.model.api.IBaseResource> historyInstance
(org.hl7.fhir.instance.model.api.IIdType theId, RequestDetails theRequestDetails) read
(org.hl7.fhir.instance.model.api.IIdType theId, RequestDetails theRequestDetails) read
(org.hl7.fhir.instance.model.api.IIdType theId, RequestDetails theRequestDetails, boolean theDeletedOk) searchAll
(RequestDetails theRequestDetails) org.hl7.fhir.instance.model.api.IIdType
This is a utility method that can be used to store a resource without having to use the outside API.ca.uhn.fhir.rest.api.MethodOutcome
update
(T theResource, String theConditional, RequestDetails theRequestDetails)
-
Field Details
-
myIdToVersionToResourceMap
-
myIdToHistory
protected Map<String,LinkedList<T extends org.hl7.fhir.instance.model.api.IBaseResource>> myIdToHistory -
myTypeHistory
-
mySearchCount
-
-
Constructor Details
-
HashMapResourceProvider
public HashMapResourceProvider(ca.uhn.fhir.context.FhirContext theFhirContext, Class<T> theResourceType) Constructor- Parameters:
theFhirContext
- The FHIR contexttheResourceType
- The resource type to support
-
-
Method Details
-
clear
Clear all data held in this resource provider -
clearCounts
Clear the counts used bygetCountRead()
and other count methods -
create
-
delete
public ca.uhn.fhir.rest.api.MethodOutcome delete(org.hl7.fhir.instance.model.api.IIdType theId, RequestDetails theRequestDetails) -
getCountCreate
This method returns a simple operation count. This is mostly useful for testing purposes. -
getCountDelete
This method returns a simple operation count. This is mostly useful for testing purposes. -
getCountRead
This method returns a simple operation count. This is mostly useful for testing purposes. -
getCountSearch
This method returns a simple operation count. This is mostly useful for testing purposes. -
getCountUpdate
This method returns a simple operation count. This is mostly useful for testing purposes. -
getResourceType
Description copied from interface:IResourceProvider
Returns the type of resource returned by this provider- Specified by:
getResourceType
in interfaceIResourceProvider
- Returns:
- Returns the type of resource returned by this provider
-
historyInstance
public List<org.hl7.fhir.instance.model.api.IBaseResource> historyInstance(org.hl7.fhir.instance.model.api.IIdType theId, RequestDetails theRequestDetails) -
historyType
-
read
-
read
public T read(org.hl7.fhir.instance.model.api.IIdType theId, RequestDetails theRequestDetails, boolean theDeletedOk) -
searchAll
-
getAllResources
-
update
public ca.uhn.fhir.rest.api.MethodOutcome update(T theResource, String theConditional, RequestDetails theRequestDetails) - Parameters:
theConditional
- This is provided only so that subclasses can implement if they want
-
getFhirContext
-
store
This is a utility method that can be used to store a resource without having to use the outside API. In this case, the storage happens without any interaction with interceptors, etc.- Parameters:
theResource
- The resource to store. If the resource has an ID, that ID is updated.- Returns:
- Return the ID assigned to the stored resource
-
getStoredResources
Returns an unmodifiable list containing the current version of all resources stored in this provider- Since:
- 4.1.0
-
fireInterceptorsAndFilterAsNeeded
protected static <T extends org.hl7.fhir.instance.model.api.IBaseResource> List<org.hl7.fhir.instance.model.api.IBaseResource> fireInterceptorsAndFilterAsNeeded(List<T> theResources, RequestDetails theRequestDetails)
-