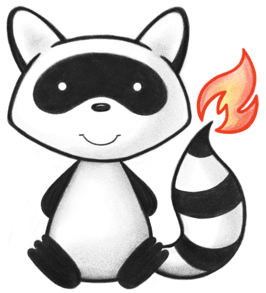
Package ca.uhn.fhir.model.dstu2.valueset
Enum Class IssueTypeEnum
- All Implemented Interfaces:
Serializable
,Comparable<IssueTypeEnum>
,Constable
-
Nested Class Summary
Nested classes/interfaces inherited from class java.lang.Enum
Enum.EnumDesc<E extends Enum<E>>
-
Enum Constant Summary
Enum ConstantsEnum ConstantDescriptionDisplay: Business Rule Violation
Code Value: business-rule The content/operation failed to pass some business rule, and so could not proceed.Display: Content not supported
Code Value: not-supported The resource or profile is not supported.Display: Content Too Long
Code Value: too-long Provided content is too long (typically, this is a denial of service protection type of error).Display: Duplicate
Code Value: duplicate An attempt was made to create a duplicate record.Display: Edit Version Conflict
Code Value: conflict Content could not be accepted because of an edit conflict (i.e. version aware updates) (In a pure RESTful environment, this would be an HTTP 404 error, but this code may be used where the conflict is discovered further into the application architecture.)Display: Element value invalid
Code Value: value An element value is invalid.Display: Exception
Code Value: exception An unexpected internal error has occurred.Display: Forbidden
Code Value: forbidden The user does not have the rights to perform this action.Display: Incomplete Results
Code Value: incomplete Not all data sources typically accessed could be reached, or responded in time, so the returned information may not be complete.Display: Information Suppressed
Code Value: suppressed Some information was not or may not have been returned due to business rules, consent or privacy rules, or access permission constraints.Display: Informational Note
Code Value: informational A message unrelated to the processing success of the completed operation (examples of the latter include things like reminders of password expiry, system maintenance times, etc.).Display: Invalid Code
Code Value: code-invalid The code or system could not be understood, or it was not valid in the context of a particular ValueSet.code.Display: Invalid Content
Code Value: invalid Content invalid against the specification or a profile.Display: Lock Error
Code Value: lock-error A resource/record locking failure (usually in an underlying database).Display: Login Required
Code Value: login The client needs to initiate an authentication process.Display: No Store Available
Code Value: no-store The persistent store is unavailable; e.g. the database is down for maintenance or similar action.Display: Not Found
Code Value: not-found The reference provided was not found.Display: Operation Too Costly
Code Value: too-costly The operation was stopped to protect server resources; e.g. a request for a value set expansion on all of SNOMED CT.Display: Processing Failure
Code Value: processing Processing issues.Display: Required element missing
Code Value: required A required element is missing.Display: Security Problem
Code Value: security An authentication/authorization/permissions issue of some kind.Display: Session Expired
Code Value: expired User session expired; a login may be required.Display: Structural Issue
Code Value: structure A structural issue in the content such as wrong namespace, or unable to parse the content completely, or invalid json syntax.Display: Throttled
Code Value: throttled The system is not prepared to handle this request due to load management.Display: Timeout
Code Value: timeout An internal timeout has occurred.Display: Transient Issue
Code Value: transient Transient processing issues.Display: Unacceptable Extension
Code Value: extension An extension was found that was not acceptable, could not be resolved, or a modifierExtension was not recognized.Display: Unknown User
Code Value: unknown The user or system was not able to be authenticated (either there is no process, or the proferred token is unacceptable).Display: Validation rule failed
Code Value: invariant A content validation rule failed - e.g. a schematron rule. -
Field Summary
FieldsModifier and TypeFieldDescriptionstatic final ca.uhn.fhir.model.api.IValueSetEnumBinder
<IssueTypeEnum> Converts codes to their respective enumerated valuesstatic final String
Identifier for this Value Set:static final String
Name for this Value Set: IssueType -
Method Summary
Modifier and TypeMethodDescriptionstatic IssueTypeEnum
Returns the enumerated value associated with this codegetCode()
Returns the code associated with this enumerated valueReturns the code system associated with this enumerated valuestatic IssueTypeEnum
Returns the enum constant of this class with the specified name.static IssueTypeEnum[]
values()
Returns an array containing the constants of this enum class, in the order they are declared.
-
Enum Constant Details
-
INVALID_CONTENT
Display: Invalid Content
Code Value: invalid Content invalid against the specification or a profile. -
STRUCTURAL_ISSUE
Display: Structural Issue
Code Value: structure A structural issue in the content such as wrong namespace, or unable to parse the content completely, or invalid json syntax. -
REQUIRED_ELEMENT_MISSING
Display: Required element missing
Code Value: required A required element is missing. -
ELEMENT_VALUE_INVALID
Display: Element value invalid
Code Value: value An element value is invalid. -
VALIDATION_RULE_FAILED
Display: Validation rule failed
Code Value: invariant A content validation rule failed - e.g. a schematron rule. -
SECURITY_PROBLEM
Display: Security Problem
Code Value: security An authentication/authorization/permissions issue of some kind. -
LOGIN_REQUIRED
Display: Login Required
Code Value: login The client needs to initiate an authentication process. -
UNKNOWN_USER
Display: Unknown User
Code Value: unknown The user or system was not able to be authenticated (either there is no process, or the proferred token is unacceptable). -
SESSION_EXPIRED
Display: Session Expired
Code Value: expired User session expired; a login may be required. -
FORBIDDEN
Display: Forbidden
Code Value: forbidden The user does not have the rights to perform this action. -
INFORMATION__SUPPRESSED
Display: Information Suppressed
Code Value: suppressed Some information was not or may not have been returned due to business rules, consent or privacy rules, or access permission constraints. This information may be accessible through alternate processes. -
PROCESSING_FAILURE
Display: Processing Failure
Code Value: processing Processing issues. These are expected to be final e.g. there is no point resubmitting the same content unchanged. -
CONTENT_NOT_SUPPORTED
Display: Content not supported
Code Value: not-supported The resource or profile is not supported. -
DUPLICATE
Display: Duplicate
Code Value: duplicate An attempt was made to create a duplicate record. -
NOT_FOUND
Display: Not Found
Code Value: not-found The reference provided was not found. In a pure RESTful environment, this would be an HTTP 404 error, but this code may be used where the content is not found further into the application architecture. -
CONTENT_TOO_LONG
Display: Content Too Long
Code Value: too-long Provided content is too long (typically, this is a denial of service protection type of error). -
INVALID_CODE
Display: Invalid Code
Code Value: code-invalid The code or system could not be understood, or it was not valid in the context of a particular ValueSet.code. -
UNACCEPTABLE_EXTENSION
Display: Unacceptable Extension
Code Value: extension An extension was found that was not acceptable, could not be resolved, or a modifierExtension was not recognized. -
OPERATION_TOO_COSTLY
Display: Operation Too Costly
Code Value: too-costly The operation was stopped to protect server resources; e.g. a request for a value set expansion on all of SNOMED CT. -
BUSINESS_RULE_VIOLATION
Display: Business Rule Violation
Code Value: business-rule The content/operation failed to pass some business rule, and so could not proceed. -
EDIT_VERSION_CONFLICT
Display: Edit Version Conflict
Code Value: conflict Content could not be accepted because of an edit conflict (i.e. version aware updates) (In a pure RESTful environment, this would be an HTTP 404 error, but this code may be used where the conflict is discovered further into the application architecture.) -
INCOMPLETE_RESULTS
Display: Incomplete Results
Code Value: incomplete Not all data sources typically accessed could be reached, or responded in time, so the returned information may not be complete. -
TRANSIENT_ISSUE
Display: Transient Issue
Code Value: transient Transient processing issues. The system receiving the error may be able to resubmit the same content once an underlying issue is resolved. -
LOCK_ERROR
Display: Lock Error
Code Value: lock-error A resource/record locking failure (usually in an underlying database). -
NO_STORE_AVAILABLE
Display: No Store Available
Code Value: no-store The persistent store is unavailable; e.g. the database is down for maintenance or similar action. -
EXCEPTION
Display: Exception
Code Value: exception An unexpected internal error has occurred. -
TIMEOUT
Display: Timeout
Code Value: timeout An internal timeout has occurred. -
THROTTLED
Display: Throttled
Code Value: throttled The system is not prepared to handle this request due to load management. -
INFORMATIONAL_NOTE
Display: Informational Note
Code Value: informational A message unrelated to the processing success of the completed operation (examples of the latter include things like reminders of password expiry, system maintenance times, etc.).
-
-
Field Details
-
VALUESET_IDENTIFIER
Identifier for this Value Set:- See Also:
-
VALUESET_NAME
Name for this Value Set: IssueType- See Also:
-
VALUESET_BINDER
Converts codes to their respective enumerated values
-
-
Method Details
-
values
Returns an array containing the constants of this enum class, in the order they are declared.- Returns:
- an array containing the constants of this enum class, in the order they are declared
-
valueOf
Returns the enum constant of this class with the specified name. The string must match exactly an identifier used to declare an enum constant in this class. (Extraneous whitespace characters are not permitted.)- Parameters:
name
- the name of the enum constant to be returned.- Returns:
- the enum constant with the specified name
- Throws:
IllegalArgumentException
- if this enum class has no constant with the specified nameNullPointerException
- if the argument is null
-
getCode
Returns the code associated with this enumerated value -
getSystem
Returns the code system associated with this enumerated value -
forCode
Returns the enumerated value associated with this code
-