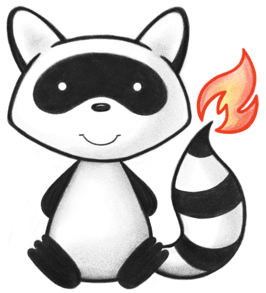
Package ca.uhn.fhir.model.dstu2.valueset
Enum LocationTypeEnum
- All Implemented Interfaces:
Serializable
,Comparable<LocationTypeEnum>
-
Enum Constant Summary
Enum ConstantsEnum ConstantDescriptionDisplay: Area
Code Value: area A defined boundary, such as a state, region, country, countyDisplay: Bed
Code Value: bd A space that is allocated for sleeping/laying on.Display: Building
Code Value: bu Any Building or structure.Display: Cabinet
Code Value: ca A container that can store goods, equipment, medications or other items.Display: Corridor
Code Value: co Any corridor within a Building, that is not within.Display: House
Code Value: ho A residential dwelling.Display: Jurisdiction
Code Value: jdn A wide scope that covers a conceptual domain, such as a Nation (Country wide community or Federal Government - e.g.Display: Level
Code Value: lvl A Level in a multi-level Building/Structure.Display: Road
Code Value: rd A defined path to travel between 2 points that has a known name.Display: Room
Code Value: ro A space that is allocated as a room, it may have walls/roof etc., but does not require these.Display: Vehicle
Code Value: ve A means of transportation.Display: Wing
Code Value: wi A Wing within a Building, this often contains levels, rooms and corridors. -
Field Summary
FieldsModifier and TypeFieldDescriptionstatic final ca.uhn.fhir.model.api.IValueSetEnumBinder
<LocationTypeEnum> Converts codes to their respective enumerated valuesstatic final String
Identifier for this Value Set:static final String
Name for this Value Set: LocationType -
Method Summary
Modifier and TypeMethodDescriptionstatic LocationTypeEnum
Returns the enumerated value associated with this codegetCode()
Returns the code associated with this enumerated valueReturns the code system associated with this enumerated valuestatic LocationTypeEnum
Returns the enum constant of this type with the specified name.static LocationTypeEnum[]
values()
Returns an array containing the constants of this enum type, in the order they are declared.
-
Enum Constant Details
-
BUILDING
Display: Building
Code Value: bu Any Building or structure. This may contain rooms, corridors, wings, etc. It may not have walls, or a roof, but is considered a defined/allocated space. -
WING
Display: Wing
Code Value: wi A Wing within a Building, this often contains levels, rooms and corridors. -
LEVEL
Display: Level
Code Value: lvl A Level in a multi-level Building/Structure. -
CORRIDOR
Display: Corridor
Code Value: co Any corridor within a Building, that is not within. -
ROOM
Display: Room
Code Value: ro A space that is allocated as a room, it may have walls/roof etc., but does not require these. -
BED
Display: Bed
Code Value: bd A space that is allocated for sleeping/laying on. -
VEHICLE
Display: Vehicle
Code Value: ve A means of transportation. -
HOUSE
Display: House
Code Value: ho A residential dwelling. Usually used to reference a location that a person/patient may reside. -
CABINET
Display: Cabinet
Code Value: ca A container that can store goods, equipment, medications or other items. -
ROAD
Display: Road
Code Value: rd A defined path to travel between 2 points that has a known name. -
JURISDICTION
Display: Jurisdiction
Code Value: jdn A wide scope that covers a conceptual domain, such as a Nation (Country wide community or Federal Government - e.g. Ministry of Health), Province or State (community or Government), Business (throughout the enterprise), Nation with a business scope of an agency (e.g. CDC, FDA etc.) or a Business segment (UK Pharmacy). -
AREA
Display: Area
Code Value: area A defined boundary, such as a state, region, country, county
-
-
Field Details
-
VALUESET_IDENTIFIER
Identifier for this Value Set:- See Also:
-
VALUESET_NAME
Name for this Value Set: LocationType- See Also:
-
VALUESET_BINDER
Converts codes to their respective enumerated values
-
-
Method Details
-
values
Returns an array containing the constants of this enum type, in the order they are declared.- Returns:
- an array containing the constants of this enum type, in the order they are declared
-
valueOf
Returns the enum constant of this type with the specified name. The string must match exactly an identifier used to declare an enum constant in this type. (Extraneous whitespace characters are not permitted.)- Parameters:
name
- the name of the enum constant to be returned.- Returns:
- the enum constant with the specified name
- Throws:
IllegalArgumentException
- if this enum type has no constant with the specified nameNullPointerException
- if the argument is null
-
getCode
Returns the code associated with this enumerated value -
getSystem
Returns the code system associated with this enumerated value -
forCode
Returns the enumerated value associated with this code
-