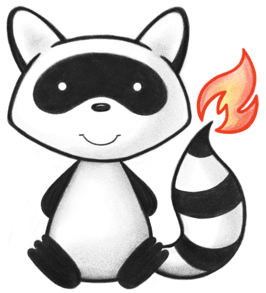
Package org.hl7.fhir.dstu3.model
Class SampledData
java.lang.Object
org.hl7.fhir.dstu3.model.Base
org.hl7.fhir.dstu3.model.Element
org.hl7.fhir.dstu3.model.Type
org.hl7.fhir.dstu3.model.SampledData
- All Implemented Interfaces:
ca.uhn.fhir.model.api.IElement
,Serializable
,org.hl7.fhir.instance.model.api.IBase
,org.hl7.fhir.instance.model.api.IBaseDatatype
,org.hl7.fhir.instance.model.api.IBaseElement
,org.hl7.fhir.instance.model.api.IBaseHasExtensions
,org.hl7.fhir.instance.model.api.ICompositeType
A series of measurements taken by a device, with upper and lower limits. There may be more than one dimension in the data.
- See Also:
-
Field Summary
FieldsModifier and TypeFieldDescriptionprotected StringType
A series of data points which are decimal values separated by a single space (character u20).protected PositiveIntType
The number of sample points at each time point.protected DecimalType
A correction factor that is applied to the sampled data points before they are added to the origin.protected DecimalType
The lower limit of detection of the measured points.protected SimpleQuantity
The base quantity that a measured value of zero represents.protected DecimalType
The length of time between sampling times, measured in milliseconds.protected DecimalType
The upper limit of detection of the measured points. -
Constructor Summary
ConstructorsConstructorDescriptionConstructorSampledData
(SimpleQuantity origin, DecimalType period, PositiveIntType dimensions, StringType data) Constructor -
Method Summary
Modifier and TypeMethodDescriptioncopy()
boolean
equalsDeep
(Base other_) boolean
equalsShallow
(Base other_) fhirType()
getData()
int
getNamedProperty
(int _hash, String _name, boolean _checkValid) Base[]
getProperty
(int hash, String name, boolean checkValid) String[]
getTypesForProperty
(int hash, String name) boolean
hasData()
boolean
boolean
boolean
boolean
boolean
boolean
boolean
boolean
boolean
boolean
boolean
boolean
boolean
isEmpty()
protected void
listChildren
(List<Property> children) makeProperty
(int hash, String name) setDataElement
(StringType value) setDimensions
(int value) setFactor
(double value) setFactor
(long value) setFactor
(BigDecimal value) setFactorElement
(DecimalType value) setLowerLimit
(double value) setLowerLimit
(long value) setLowerLimit
(BigDecimal value) setLowerLimitElement
(DecimalType value) setOrigin
(SimpleQuantity value) setPeriod
(double value) setPeriod
(long value) setPeriod
(BigDecimal value) setPeriodElement
(DecimalType value) setProperty
(int hash, String name, Base value) setProperty
(String name, Base value) setUpperLimit
(double value) setUpperLimit
(long value) setUpperLimit
(BigDecimal value) setUpperLimitElement
(DecimalType value) protected SampledData
Methods inherited from class org.hl7.fhir.dstu3.model.Element
addExtension, addExtension, addExtension, copyValues, getExtension, getExtensionByUrl, getExtensionFirstRep, getExtensionsByUrl, getExtensionString, getId, getIdBase, getIdElement, hasExtension, hasExtension, hasId, hasIdElement, setExtension, setId, setIdBase, setIdElement
Methods inherited from class org.hl7.fhir.dstu3.model.Base
castToAddress, castToAnnotation, castToAttachment, castToBase64Binary, castToBoolean, castToCode, castToCodeableConcept, castToCoding, castToContactDetail, castToContactPoint, castToContributor, castToDataRequirement, castToDate, castToDateTime, castToDecimal, castToDosage, castToDuration, castToElementDefinition, castToExtension, castToHumanName, castToId, castToIdentifier, castToInstant, castToInteger, castToMarkdown, castToMeta, castToMoney, castToNarrative, castToOid, castToParameterDefinition, castToPeriod, castToPositiveInt, castToQuantity, castToRange, castToRatio, castToReference, castToRelatedArtifact, castToResource, castToSampledData, castToSignature, castToSimpleQuantity, castToString, castToTime, castToTiming, castToTriggerDefinition, castToType, castToUnsignedInt, castToUri, castToUsageContext, castToXhtml, castToXhtmlString, children, clearUserData, compareDeep, compareDeep, compareDeep, compareValues, compareValues, equals, getChildByName, getFormatCommentsPost, getFormatCommentsPre, getNamedProperty, getUserData, getUserInt, getUserString, hasFormatComment, hasPrimitiveValue, hasType, hasUserData, isBooleanPrimitive, isMetadataBased, isPrimitive, isResource, listChildrenByName, listChildrenByName, primitiveValue, setUserData, setUserDataINN
Methods inherited from class java.lang.Object
clone, equals, finalize, getClass, hashCode, notify, notifyAll, toString, wait, wait, wait
Methods inherited from interface org.hl7.fhir.instance.model.api.IBase
getFormatCommentsPost, getFormatCommentsPre, getUserData, hasFormatComment, setUserData
Methods inherited from interface org.hl7.fhir.instance.model.api.IBaseElement
getUserData, setUserData
-
Field Details
-
origin
The base quantity that a measured value of zero represents. In addition, this provides the units of the entire measurement series. -
period
The length of time between sampling times, measured in milliseconds. -
factor
A correction factor that is applied to the sampled data points before they are added to the origin. -
lowerLimit
The lower limit of detection of the measured points. This is needed if any of the data points have the value "L" (lower than detection limit). -
upperLimit
The upper limit of detection of the measured points. This is needed if any of the data points have the value "U" (higher than detection limit). -
dimensions
The number of sample points at each time point. If this value is greater than one, then the dimensions will be interlaced - all the sample points for a point in time will be recorded at once. -
data
A series of data points which are decimal values separated by a single space (character u20). The special values "E" (error), "L" (below detection limit) and "U" (above detection limit) can also be used in place of a decimal value.
-
-
Constructor Details
-
SampledData
public SampledData()Constructor -
SampledData
public SampledData(SimpleQuantity origin, DecimalType period, PositiveIntType dimensions, StringType data) Constructor
-
-
Method Details
-
getOrigin
- Returns:
origin
(The base quantity that a measured value of zero represents. In addition, this provides the units of the entire measurement series.)
-
hasOrigin
-
setOrigin
- Parameters:
value
-origin
(The base quantity that a measured value of zero represents. In addition, this provides the units of the entire measurement series.)
-
getPeriodElement
- Returns:
period
(The length of time between sampling times, measured in milliseconds.). This is the underlying object with id, value and extensions. The accessor "getPeriod" gives direct access to the value
-
hasPeriodElement
-
hasPeriod
-
setPeriodElement
- Parameters:
value
-period
(The length of time between sampling times, measured in milliseconds.). This is the underlying object with id, value and extensions. The accessor "getPeriod" gives direct access to the value
-
getPeriod
- Returns:
- The length of time between sampling times, measured in milliseconds.
-
setPeriod
- Parameters:
value
- The length of time between sampling times, measured in milliseconds.
-
setPeriod
- Parameters:
value
- The length of time between sampling times, measured in milliseconds.
-
setPeriod
- Parameters:
value
- The length of time between sampling times, measured in milliseconds.
-
getFactorElement
- Returns:
factor
(A correction factor that is applied to the sampled data points before they are added to the origin.). This is the underlying object with id, value and extensions. The accessor "getFactor" gives direct access to the value
-
hasFactorElement
-
hasFactor
-
setFactorElement
- Parameters:
value
-factor
(A correction factor that is applied to the sampled data points before they are added to the origin.). This is the underlying object with id, value and extensions. The accessor "getFactor" gives direct access to the value
-
getFactor
- Returns:
- A correction factor that is applied to the sampled data points before they are added to the origin.
-
setFactor
- Parameters:
value
- A correction factor that is applied to the sampled data points before they are added to the origin.
-
setFactor
- Parameters:
value
- A correction factor that is applied to the sampled data points before they are added to the origin.
-
setFactor
- Parameters:
value
- A correction factor that is applied to the sampled data points before they are added to the origin.
-
getLowerLimitElement
- Returns:
lowerLimit
(The lower limit of detection of the measured points. This is needed if any of the data points have the value "L" (lower than detection limit).). This is the underlying object with id, value and extensions. The accessor "getLowerLimit" gives direct access to the value
-
hasLowerLimitElement
-
hasLowerLimit
-
setLowerLimitElement
- Parameters:
value
-lowerLimit
(The lower limit of detection of the measured points. This is needed if any of the data points have the value "L" (lower than detection limit).). This is the underlying object with id, value and extensions. The accessor "getLowerLimit" gives direct access to the value
-
getLowerLimit
- Returns:
- The lower limit of detection of the measured points. This is needed if any of the data points have the value "L" (lower than detection limit).
-
setLowerLimit
- Parameters:
value
- The lower limit of detection of the measured points. This is needed if any of the data points have the value "L" (lower than detection limit).
-
setLowerLimit
- Parameters:
value
- The lower limit of detection of the measured points. This is needed if any of the data points have the value "L" (lower than detection limit).
-
setLowerLimit
- Parameters:
value
- The lower limit of detection of the measured points. This is needed if any of the data points have the value "L" (lower than detection limit).
-
getUpperLimitElement
- Returns:
upperLimit
(The upper limit of detection of the measured points. This is needed if any of the data points have the value "U" (higher than detection limit).). This is the underlying object with id, value and extensions. The accessor "getUpperLimit" gives direct access to the value
-
hasUpperLimitElement
-
hasUpperLimit
-
setUpperLimitElement
- Parameters:
value
-upperLimit
(The upper limit of detection of the measured points. This is needed if any of the data points have the value "U" (higher than detection limit).). This is the underlying object with id, value and extensions. The accessor "getUpperLimit" gives direct access to the value
-
getUpperLimit
- Returns:
- The upper limit of detection of the measured points. This is needed if any of the data points have the value "U" (higher than detection limit).
-
setUpperLimit
- Parameters:
value
- The upper limit of detection of the measured points. This is needed if any of the data points have the value "U" (higher than detection limit).
-
setUpperLimit
- Parameters:
value
- The upper limit of detection of the measured points. This is needed if any of the data points have the value "U" (higher than detection limit).
-
setUpperLimit
- Parameters:
value
- The upper limit of detection of the measured points. This is needed if any of the data points have the value "U" (higher than detection limit).
-
getDimensionsElement
- Returns:
dimensions
(The number of sample points at each time point. If this value is greater than one, then the dimensions will be interlaced - all the sample points for a point in time will be recorded at once.). This is the underlying object with id, value and extensions. The accessor "getDimensions" gives direct access to the value
-
hasDimensionsElement
-
hasDimensions
-
setDimensionsElement
- Parameters:
value
-dimensions
(The number of sample points at each time point. If this value is greater than one, then the dimensions will be interlaced - all the sample points for a point in time will be recorded at once.). This is the underlying object with id, value and extensions. The accessor "getDimensions" gives direct access to the value
-
getDimensions
- Returns:
- The number of sample points at each time point. If this value is greater than one, then the dimensions will be interlaced - all the sample points for a point in time will be recorded at once.
-
setDimensions
- Parameters:
value
- The number of sample points at each time point. If this value is greater than one, then the dimensions will be interlaced - all the sample points for a point in time will be recorded at once.
-
getDataElement
- Returns:
data
(A series of data points which are decimal values separated by a single space (character u20). The special values "E" (error), "L" (below detection limit) and "U" (above detection limit) can also be used in place of a decimal value.). This is the underlying object with id, value and extensions. The accessor "getData" gives direct access to the value
-
hasDataElement
-
hasData
-
setDataElement
- Parameters:
value
-data
(A series of data points which are decimal values separated by a single space (character u20). The special values "E" (error), "L" (below detection limit) and "U" (above detection limit) can also be used in place of a decimal value.). This is the underlying object with id, value and extensions. The accessor "getData" gives direct access to the value
-
getData
- Returns:
- A series of data points which are decimal values separated by a single space (character u20). The special values "E" (error), "L" (below detection limit) and "U" (above detection limit) can also be used in place of a decimal value.
-
setData
- Parameters:
value
- A series of data points which are decimal values separated by a single space (character u20). The special values "E" (error), "L" (below detection limit) and "U" (above detection limit) can also be used in place of a decimal value.
-
listChildren
- Overrides:
listChildren
in classElement
-
getNamedProperty
public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws org.hl7.fhir.exceptions.FHIRException - Overrides:
getNamedProperty
in classElement
- Throws:
org.hl7.fhir.exceptions.FHIRException
-
getProperty
public Base[] getProperty(int hash, String name, boolean checkValid) throws org.hl7.fhir.exceptions.FHIRException - Overrides:
getProperty
in classElement
- Throws:
org.hl7.fhir.exceptions.FHIRException
-
setProperty
public Base setProperty(int hash, String name, Base value) throws org.hl7.fhir.exceptions.FHIRException - Overrides:
setProperty
in classElement
- Throws:
org.hl7.fhir.exceptions.FHIRException
-
setProperty
- Overrides:
setProperty
in classElement
- Throws:
org.hl7.fhir.exceptions.FHIRException
-
makeProperty
- Overrides:
makeProperty
in classElement
- Throws:
org.hl7.fhir.exceptions.FHIRException
-
getTypesForProperty
public String[] getTypesForProperty(int hash, String name) throws org.hl7.fhir.exceptions.FHIRException - Overrides:
getTypesForProperty
in classElement
- Throws:
org.hl7.fhir.exceptions.FHIRException
-
addChild
-
fhirType
-
copy
-
typedCopy
-
equalsDeep
- Overrides:
equalsDeep
in classElement
-
equalsShallow
- Overrides:
equalsShallow
in classElement
-
isEmpty
-